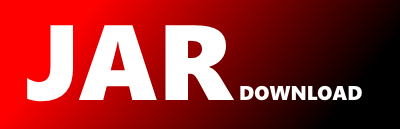
com.backendless.persistence.BackendlessDataQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
/*
* ********************************************************************************************************************
*
* BACKENDLESS.COM CONFIDENTIAL
*
* ********************************************************************************************************************
*
* Copyright 2012 BACKENDLESS.COM. All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of Backendless.com and its suppliers,
* if any. The intellectual and technical concepts contained herein are proprietary to Backendless.com and its
* suppliers and may be covered by U.S. and Foreign Patents, patents in process, and are protected by trade secret
* or copyright law. Dissemination of this information or reproduction of this material is strictly forbidden
* unless prior written permission is obtained from Backendless.com.
*
* ********************************************************************************************************************
*/
package com.backendless.persistence;
import java.util.ArrayList;
import java.util.List;
public class BackendlessDataQuery extends AbstractBackendlessQuery
{
public static final int DEFAULT_PAGE_SIZE = 10;
public static final int DEFAULT_OFFSET = 0;
private boolean distinct = false;
private final ArrayList properties = new ArrayList<>();
private final ArrayList excludeProperties = new ArrayList<>();
private String whereClause;
private QueryOptions queryOptions;
private final List groupBy = new ArrayList<>();
private String havingClause = "";
public BackendlessDataQuery()
{
}
public BackendlessDataQuery( List properties )
{
this.properties.addAll( properties );
}
public BackendlessDataQuery( String whereClause )
{
this.whereClause = whereClause;
}
public BackendlessDataQuery( QueryOptions queryOptions )
{
this.queryOptions = queryOptions;
}
public BackendlessDataQuery( List properties, String whereClause, QueryOptions queryOptions,
List groupBy, String havingClause )
{
this.setProperties( properties );
this.whereClause = whereClause;
this.queryOptions = queryOptions;
this.setGroupBy( groupBy );
this.havingClause = havingClause;
}
public boolean getDistinct()
{
return distinct;
}
public BackendlessDataQuery setDistinct(boolean distinct)
{
this.distinct = distinct;
return this;
}
public List getProperties()
{
return (List) this.properties.clone();
}
public void setProperties( List properties )
{
this.properties.clear();
if (properties != null)
for( String prop: properties )
this.addProperty( prop );
}
public void addProperties( String... properties )
{
if( properties != null )
for( String prop : properties )
this.addProperty( prop );
}
public void addProperty( String property )
{
if( property != null && !property.equals( "" ) )
properties.add( property );
}
public ArrayList getExcludeProperties()
{
return (ArrayList) excludeProperties.clone();
}
public void setExcludeProperties( ArrayList excludeProperties )
{
this.excludeProperties.clear();
if( excludeProperties != null )
for( String exclProp: excludeProperties )
this.setExcludeProperty( exclProp );
}
public void setExcludeProperties( String... excludeProperties )
{
this.excludeProperties.clear();
if( excludeProperties != null )
for( String exclProp: excludeProperties )
this.setExcludeProperty( exclProp );
}
public void setExcludeProperty( String excludeProperty )
{
if( excludeProperty != null && !excludeProperty.isEmpty() )
excludeProperties.add( excludeProperty );
}
public String getWhereClause()
{
return whereClause;
}
public void setWhereClause( String whereClause )
{
this.whereClause = whereClause;
}
public QueryOptions getQueryOptions()
{
if( queryOptions == null )
return null;
return queryOptions.newInstance();
}
public void setQueryOptions( QueryOptions queryOptions )
{
this.queryOptions = queryOptions;
}
public List getGroupBy()
{
return new ArrayList<>( this.groupBy );
}
public void setGroupBy( List groupBy )
{
this.groupBy.clear();
for( String grb : groupBy )
{
if( grb != null && !grb.equals( "" ) )
this.groupBy.add( grb );
}
}
public String getHavingClause()
{
return havingClause;
}
public void setHavingClause( String havingClause )
{
this.havingClause = havingClause;
}
@Override
public BackendlessDataQuery newInstance()
{
BackendlessDataQuery result = new BackendlessDataQuery();
result.setDistinct( getDistinct() );
result.setProperties( getProperties() );
result.setWhereClause( whereClause );
result.setQueryOptions( getQueryOptions() );
result.setGroupBy( groupBy );
result.setHavingClause( havingClause );
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy