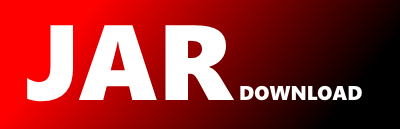
com.backendless.servercode.extension.PersistenceExtender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
/*
* ********************************************************************************************************************
*
* BACKENDLESS.COM CONFIDENTIAL
*
* ********************************************************************************************************************
*
* Copyright 2012 BACKENDLESS.COM. All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property of Backendless.com and its suppliers,
* if any. The intellectual and technical concepts contained herein are proprietary to Backendless.com and its
* suppliers and may be covered by U.S. and Foreign Patents, patents in process, and are protected by trade secret
* or copyright law. Dissemination of this information or reproduction of this material is strictly forbidden
* unless prior written permission is obtained from Backendless.com.
*
* ********************************************************************************************************************
*/
package com.backendless.servercode.extension;
import com.backendless.commons.persistence.GroupResult;
import com.backendless.persistence.BackendlessDataQuery;
import com.backendless.persistence.BackendlessGroupDataQuery;
import com.backendless.property.ObjectProperty;
import com.backendless.servercode.ExecutionResult;
import com.backendless.servercode.RunnerContext;
import com.backendless.transaction.UnitOfWork;
import com.backendless.transaction.UnitOfWorkResult;
import java.util.HashMap;
import java.util.List;
public abstract class PersistenceExtender
{
public void beforeFindById( RunnerContext context, Object objectId, String[] relations ) throws Exception
{
}
public void afterFindById( RunnerContext context, Object objectId, String[] relations,
ExecutionResult entity ) throws Exception
{
}
public void beforeCreate( RunnerContext context, T t ) throws Exception
{
}
public void afterCreate( RunnerContext context, T t, ExecutionResult entity ) throws Exception
{
}
public void beforeCreateBulk( RunnerContext context, List entities )
{
}
public void afterCreateBulk( RunnerContext context, List entities, ExecutionResult> result )
{
}
public void beforeUpdate( RunnerContext context, T t ) throws Exception
{
}
public void afterUpdate( RunnerContext context, T t, ExecutionResult entity ) throws Exception
{
}
public void beforeUpdateBulk( RunnerContext context, String tableName, String whereClause,
HashMap hashmap ) throws Exception
{
}
public void afterUpdateBulk( RunnerContext context, String tableName, String whereClause, HashMap hashmap,
ExecutionResult result ) throws Exception
{
}
public void beforeUpsert( RunnerContext context, HashMap hashmap ) throws Exception
{
}
public void afterUpsert( RunnerContext context, HashMap hashmap, ExecutionResult result ) throws Exception
{
}
public void beforeUpsertBulk( RunnerContext context, List entities)
{
}
public void afterUpsertBulk( RunnerContext context, List entities, ExecutionResult> result )
{
}
public void beforeLoadRelations( RunnerContext context, Object objectId, String entityName,
String relationName, int pageSize, int offset ) throws Exception
{
}
public void afterLoadRelations( RunnerContext context, Object objectId, String entityName,
String relationName, int pageSize, int offset,
ExecutionResult collection ) throws Exception
{
}
public void beforeRemove( RunnerContext context, Object objectId ) throws Exception
{
}
public void afterRemove( RunnerContext context, Object objectId, ExecutionResult removedVal ) throws Exception
{
}
public void beforeRemoveBulk( RunnerContext context, String tableName, String whereClause ) throws Exception
{
}
public void afterRemoveBulk( RunnerContext context, String tableName, String whereClause,
ExecutionResult result ) throws Exception
{
}
public void beforeDescribe( RunnerContext context, String entityName ) throws Exception
{
}
public void afterDescribe( RunnerContext context, String entityName,
ExecutionResult> propertiesFound ) throws Exception
{
}
public void beforeFind( RunnerContext context, BackendlessDataQuery query ) throws Exception
{
}
public void afterFind( RunnerContext context, BackendlessDataQuery query,
ExecutionResult> List ) throws Exception
{
}
public void beforeGroup( RunnerContext context, BackendlessGroupDataQuery query ) throws Exception
{
}
public void afterGroup( RunnerContext context, BackendlessGroupDataQuery query,
ExecutionResult> result ) throws Exception
{
}
public void beforeFirst( RunnerContext context, String[] relations, Integer relationsDepth, String[] properties ) throws Exception
{
}
public void afterFirst( RunnerContext context, String[] relations, Integer relationsDepth, String[] properties, ExecutionResult entity ) throws Exception
{
}
public void beforeLast( RunnerContext context, String[] relations, Integer relationsDepth, String[] properties ) throws Exception
{
}
public void afterLast( RunnerContext context, String[] relations, Integer relationsDepth, String[] properties, ExecutionResult entity ) throws Exception
{
}
public void beforeCount( RunnerContext context, BackendlessDataQuery query ) throws Exception
{
}
public void afterCount( RunnerContext context, BackendlessDataQuery query,
ExecutionResult result ) throws Exception
{
}
public void beforeCountInGroup( RunnerContext context, BackendlessGroupDataQuery query ) throws Exception
{
}
public void afterCountInGroup( RunnerContext context, BackendlessGroupDataQuery query, ExecutionResult result ) throws Exception
{
}
public void beforeAddRelation( RunnerContext context, String columnName, String parentObjectId, Object childrenArrayORWhereClause )
{
}
public void afterAddRelation( RunnerContext context, String columnName, String parentObjectId, Object childrenArrayORWhereClause, ExecutionResult result )
{
}
public void beforeSetRelation( RunnerContext context, String columnName, String parentObjectId, Object childrenArrayORWhereClause )
{
}
public void afterSetRelation( RunnerContext context, String columnName, String parentObjectId, Object childrenArrayORWhereClause, ExecutionResult result )
{
}
public void beforeDeleteRelation( RunnerContext context, String columnName, String parentObjectId, Object childrenArrayORWhereClause )
{
}
public void afterDeleteRelation( RunnerContext context, String columnName, String parentObjectId, Object childrenArrayORWhereClause, ExecutionResult result )
{
}
public void beforeTransaction( RunnerContext context, UnitOfWork unitOfWork )
{
}
public void afterTransaction( RunnerContext context, UnitOfWork unitOfWork, ExecutionResult result )
{
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy