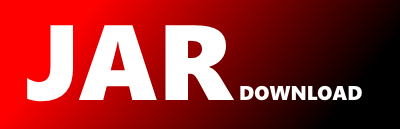
com.backendless.utils.MapEntityUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-common Show documentation
Show all versions of java-sdk-common Show documentation
Provides access to Backendless API
The newest version!
package com.backendless.utils;
import com.backendless.Persistence;
import com.backendless.commons.persistence.EntityDescription;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
/**
* Operations on a data entity represented by {@link Map}
* (e.g. a serialized object).
*/
public final class MapEntityUtil
{
/**
* Removes relations and {@code null} fields (except system ones) from {@code entityMap}.
* System fields like "created", "updated", "__meta" etc. are not removed if {@code null}.
*
* @param entityMap entity object to clean up
*/
public static void removeNullsAndRelations( Map entityMap )
{
Iterator> entryIterator = entityMap.entrySet().iterator();
while( entryIterator.hasNext() )
{
Map.Entry entry = entryIterator.next();
if( !isSystemField( entry ) )
{
if( isNullField( entry ) || isRelationField( entry ) )
{
entryIterator.remove();
}
}
}
}
private static boolean isNullField( Map.Entry entry )
{
return entry.getValue() == null;
}
private static boolean isRelationField( Map.Entry entry )
{
return entry.getValue() instanceof Map
|| entry.getValue() instanceof EntityDescription
|| entry.getValue() instanceof Collection
|| entry.getValue().getClass().isArray();
}
private static boolean isSystemField( Map.Entry entry )
{
return entry.getKey().equals( Persistence.DEFAULT_OBJECT_ID_FIELD )
|| entry.getKey().equals( Persistence.DEFAULT_CREATED_FIELD )
|| entry.getKey().equals( Persistence.DEFAULT_UPDATED_FIELD )
|| entry.getKey().equals( Persistence.DEFAULT_META_FIELD );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy