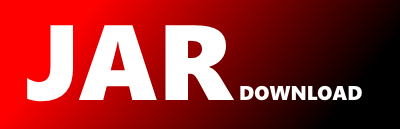
com.badlogic.ashley.core.ComponentOperationHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ashley Show documentation
Show all versions of ashley Show documentation
Ashley, a minimal entity framework inspired by Ash and Artemis
package com.badlogic.ashley.core;
import com.badlogic.gdx.utils.Array;
import com.badlogic.gdx.utils.Pool;
class ComponentOperationHandler {
private BooleanInformer delayed;
private ComponentOperationPool operationPool = new ComponentOperationPool();;
private Array operations = new Array();;
public ComponentOperationHandler(BooleanInformer delayed) {
this.delayed = delayed;
}
public void add(Entity entity) {
if (delayed.value()) {
ComponentOperation operation = operationPool.obtain();
operation.makeAdd(entity);
operations.add(operation);
}
else {
entity.notifyComponentAdded();
}
}
public void remove(Entity entity) {
if (delayed.value()) {
ComponentOperation operation = operationPool.obtain();
operation.makeRemove(entity);
operations.add(operation);
}
else {
entity.notifyComponentRemoved();
}
}
public void processOperations() {
for (int i = 0; i < operations.size; ++i) {
ComponentOperation operation = operations.get(i);
switch(operation.type) {
case Add:
operation.entity.notifyComponentAdded();
break;
case Remove:
operation.entity.notifyComponentRemoved();
break;
default: break;
}
operationPool.free(operation);
}
operations.clear();
}
private static class ComponentOperation implements Pool.Poolable {
public enum Type {
Add,
Remove,
}
public Type type;
public Entity entity;
public void makeAdd(Entity entity) {
this.type = Type.Add;
this.entity = entity;
}
public void makeRemove(Entity entity) {
this.type = Type.Remove;
this.entity = entity;
}
@Override
public void reset() {
entity = null;
}
}
private static class ComponentOperationPool extends Pool {
@Override
protected ComponentOperation newObject() {
return new ComponentOperation();
}
}
interface BooleanInformer {
public boolean value();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy