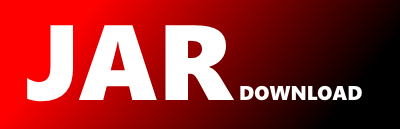
com.badlogic.gdx.pay.server.impl.Security Maven / Gradle / Ivy
package com.badlogic.gdx.pay.server.impl;
import com.badlogic.gdx.pay.server.util.Base64Util;
import java.security.InvalidKeyException;
import java.security.KeyFactory;
import java.security.NoSuchAlgorithmException;
import java.security.PublicKey;
import java.security.Signature;
import java.security.SignatureException;
import java.security.spec.InvalidKeySpecException;
import java.security.spec.X509EncodedKeySpec;
public class Security {
private static final String KEY_FACTORY_ALGORITHM = "RSA";
private static final String SIGNATURE_ALGORITHM = "SHA1withRSA";
private static final SecurityLogger logger = new SecurityLogger() {
@Override public void log (String message) {
System.out.println(message);
}
};
/**
* Generates a PublicKey instance from a string containing the
* Base64-encoded public key.
*
* @param encodedPublicKey Base64-encoded public key
* @throws IllegalArgumentException if encodedPublicKey is invalid
*/
public static PublicKey generatePublicKey(String encodedPublicKey) {
try {
byte[] decodedKey = Base64Util.decode(encodedPublicKey);
KeyFactory keyFactory = KeyFactory.getInstance(KEY_FACTORY_ALGORITHM);
return keyFactory.generatePublic(new X509EncodedKeySpec(decodedKey));
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
} catch (InvalidKeySpecException e) {
System.out.println("Invalid key specification.");
throw new IllegalArgumentException(e);
}
}
/**
* Verifies that the signature from the server matches the computed
* signature on the data. Returns true if the data is correctly signed.
*
* @param publicKey public key associated with the developer account
* @param signedData signed data from server
* @param signature server signature
* @return true if the data and signature match
*/
public static boolean verify(PublicKey publicKey, String signedData, String signature) {
return verify(publicKey, signedData, signature, logger);
}
/**
* Verifies that the signature from the server matches the computed
* signature on the data. Returns true if the data is correctly signed.
*
* @param publicKey public key associated with the developer account
* @param signedData signed data from server
* @param signature server signature
* @param logger logger that will log any issues
* @return true if the data and signature match
*/
public static boolean verify(PublicKey publicKey, String signedData, String signature, SecurityLogger logger) {
byte[] signatureBytes;
try {
signatureBytes = Base64Util.decode(signature);
Signature sig = Signature.getInstance(SIGNATURE_ALGORITHM);
sig.initVerify(publicKey);
sig.update(signedData.getBytes());
if (!sig.verify(signatureBytes)) {
logger.log("Signature verification failed.");
return false;
}
return true;
} catch (NoSuchAlgorithmException e) {
logger.log("NoSuchAlgorithmException.");
} catch (InvalidKeyException e) {
logger.log("Invalid key specification.");
} catch (SignatureException e) {
logger.log("Signature exception.");
}
return false;
}
public interface SecurityLogger {
void log(String message);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy