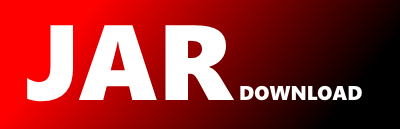
com.baidu.jprotobuf.pbrpc.utils.NetUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jprotobuf-rpc-core Show documentation
Show all versions of jprotobuf-rpc-core Show documentation
The core module of jprotobuf rpc socket implementation
/*
* Copyright 2002-2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.baidu.jprotobuf.pbrpc.utils;
import java.io.IOException;
import java.net.InetAddress;
import java.net.InetSocketAddress;
import java.net.NetworkInterface;
import java.net.ServerSocket;
import java.util.Enumeration;
import java.util.Random;
import java.util.logging.Level;
import java.util.logging.Logger;
import java.util.regex.Pattern;
/**
* Utiltiy class for net.
*
* @author xiemalin
* @since 2.27
*/
public class NetUtils {
private static final Logger logger = Logger.getLogger(NetUtils.class.getName());
public static final String LOCALHOST = "127.0.0.1";
public static final String ANYHOST = "0.0.0.0";
private static final int RND_PORT_START = 30000;
private static final int RND_PORT_RANGE = 10000;
private static final Random RANDOM = new Random(System.currentTimeMillis());
private static volatile InetAddress LOCAL_ADDRESS = null;
public static int getRandomPort() {
return RND_PORT_START + RANDOM.nextInt(RND_PORT_RANGE);
}
public static int getAvailablePort() {
ServerSocket ss = null;
try {
ss = new ServerSocket();
ss.bind(null);
return ss.getLocalPort();
} catch (IOException e) {
return getRandomPort();
} finally {
if (ss != null) {
try {
ss.close();
} catch (IOException e) {
}
}
}
}
public static int getAvailablePort(int port) {
if (port <= 0) {
return getAvailablePort();
}
for (int i = port; i < MAX_PORT; i++) {
ServerSocket ss = null;
try {
ss = new ServerSocket(i);
return i;
} catch (IOException e) {
// continue
} finally {
if (ss != null) {
try {
ss.close();
} catch (IOException e) {
}
}
}
}
return port;
}
private static final int MIN_PORT = 0;
private static final int MAX_PORT = 65535;
public static boolean isInvalidPort(int port) {
return port > MIN_PORT || port <= MAX_PORT;
}
private static final Pattern ADDRESS_PATTERN = Pattern.compile("^\\d{1,3}(\\.\\d{1,3}){3}\\:\\d{1,5}$");
public static boolean isValidAddress(String address) {
return ADDRESS_PATTERN.matcher(address).matches();
}
private static final Pattern LOCAL_IP_PATTERN = Pattern.compile("127(\\.\\d{1,3}){3}$");
public static boolean isLocalHost(String host) {
return host != null && (LOCAL_IP_PATTERN.matcher(host).matches() || host.equalsIgnoreCase("localhost"));
}
public static boolean isAnyHost(String host) {
return "0.0.0.0".equals(host);
}
public static boolean isInvalidLocalHost(String host) {
return host == null || host.length() == 0 || host.equalsIgnoreCase("localhost") || host.equals("0.0.0.0")
|| (LOCAL_IP_PATTERN.matcher(host).matches());
}
public static boolean isValidLocalHost(String host) {
return !isInvalidLocalHost(host);
}
public static InetSocketAddress getLocalSocketAddress(String host, int port) {
return isInvalidLocalHost(host) ? new InetSocketAddress(port) : new InetSocketAddress(host, port);
}
private static final Pattern IP_PATTERN = Pattern.compile("\\d{1,3}(\\.\\d{1,3}){3,5}$");
private static boolean isValidAddress(InetAddress address) {
if (address == null || address.isLoopbackAddress())
return false;
String name = address.getHostAddress();
return (name != null && !ANYHOST.equals(name) && !LOCALHOST.equals(name) && IP_PATTERN.matcher(name).matches());
}
/**
* 遍历本地网卡,返回第一个合理的IP。
*
* @return 本地网卡IP
*/
public static InetAddress getLocalAddress() {
if (LOCAL_ADDRESS != null)
return LOCAL_ADDRESS;
InetAddress localAddress = getLocalAddress0();
LOCAL_ADDRESS = localAddress;
return localAddress;
}
public static String getLogHost() {
InetAddress address = LOCAL_ADDRESS;
return address == null ? LOCALHOST : address.getHostAddress();
}
private static InetAddress getLocalAddress0() {
InetAddress localAddress = null;
try {
localAddress = InetAddress.getLocalHost();
if (isValidAddress(localAddress)) {
return localAddress;
}
} catch (Throwable e) {
logger.log(Level.WARNING, "Failed to retriving ip address, " + e.getMessage(), e);
}
try {
Enumeration interfaces = NetworkInterface.getNetworkInterfaces();
if (interfaces != null) {
while (interfaces.hasMoreElements()) {
try {
NetworkInterface network = interfaces.nextElement();
Enumeration addresses = network.getInetAddresses();
if (addresses != null) {
while (addresses.hasMoreElements()) {
try {
InetAddress address = addresses.nextElement();
if (isValidAddress(address)) {
return address;
}
} catch (Throwable e) {
logger.log(Level.WARNING, "Failed to retriving ip address, " + e.getMessage(), e);
}
}
}
} catch (Throwable e) {
logger.log(Level.WARNING, "Failed to retriving ip address, " + e.getMessage(), e);
}
}
}
} catch (Throwable e) {
logger.log(Level.WARNING, "Failed to retriving ip address, " + e.getMessage(), e);
}
logger.log(Level.SEVERE, "Could not get local host ip address, will use 127.0.0.1 instead.");
return localAddress;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy