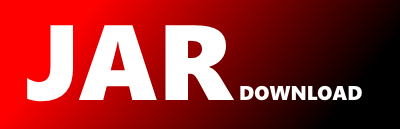
com.bakdata.fluent_kafka_streams_tests.Expectation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-kafka-streams-tests Show documentation
Show all versions of fluent-kafka-streams-tests Show documentation
Provides the fluent Kafka Streams test framework.
/*
* MIT License
*
* Copyright (c) 2019 bakdata GmbH
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package com.bakdata.fluent_kafka_streams_tests;
import lombok.RequiredArgsConstructor;
import org.apache.kafka.clients.producer.ProducerRecord;
/**
* Represents a single output {@link ProducerRecord} from {@link TestOutput} to be tested.
*
* @param the key type of the record under test
* @param the value type of the record under test
*/
@RequiredArgsConstructor
public class Expectation {
private final ProducerRecord record;
private final TestOutput output;
/**
* Asserts whether a record exists.
*/
public Expectation isPresent() {
if (this.record == null) {
throw new AssertionError("No more records found");
}
return this.and();
}
/**
* Checks for the equality of the {@link ProducerRecord#key()} and {@code expectedKey}.
*/
public Expectation hasKey(final K expectedKey) {
this.isPresent();
if (!this.record.key().equals(expectedKey)) {
throw new AssertionError("Record key does not match");
}
return this.and();
}
/**
* Checks for the equality of the {@link ProducerRecord#value()} and {@code expectedValue}.
*/
public Expectation hasValue(final V expectedValue) {
this.isPresent();
if (!this.record.value().equals(expectedValue)) {
throw new AssertionError("Record value does not match");
}
return this.and();
}
/**
* Concatenates calls to this Expectation. It is not necessary to call this method, but it can be seen as a more
* readable alternative to simple chaining.
*/
public Expectation and() {
return this;
}
/**
* Reads the next record as creates an {@link Expectation} for it.
* This is logically equivalent to {@link TestOutput#expectNextRecord()}.
* This methods main purpose is to allow chaining:
* {@code
* myOutput.expectNextRecord()
* .expectNextRecord()
* .expectNoMoreRecord();
* }
*
* @return An {@link Expectation} containing the next record from the output.
*/
public Expectation expectNextRecord() {
return this.output.expectNextRecord();
}
/**
* Reads the next record from the output and expects it to be the end of output.
* This is logically equivalent to {@link TestOutput#expectNoMoreRecord()}.
* This methods main purpose is to allow chaining:
* {@code
* myOutput.expectNextRecord()
* .expectNextRecord()
* .expectNoMoreRecord();
* }
*
* @return An {@link Expectation} containing the next record from the output.
*/
public Expectation expectNoMoreRecord() {
return this.output.expectNoMoreRecord();
}
/**
* Asserts that there is no records present, i.e., the end of the output has been reached.
* This method should be used when there are no records at all expected.
*/
public Expectation toBeEmpty() {
if (this.record != null) {
throw new AssertionError("More records found");
}
return this.and();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy