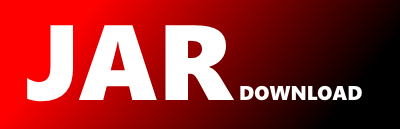
com.bandwidth.messaging.models.BandwidthCallbackMessage Maven / Gradle / Ivy
/*
* BandwidthLib
*
* This file was automatically generated by APIMATIC v3.0 ( https://www.apimatic.io ).
*/
package com.bandwidth.messaging.models;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonSetter;
/**
* This is a model class for BandwidthCallbackMessage type.
*/
public class BandwidthCallbackMessage {
@JsonInclude(JsonInclude.Include.NON_NULL)
private String time;
@JsonInclude(JsonInclude.Include.NON_NULL)
private String type;
@JsonInclude(JsonInclude.Include.NON_NULL)
private String to;
@JsonInclude(JsonInclude.Include.NON_NULL)
private String errorCode;
@JsonInclude(JsonInclude.Include.NON_NULL)
private String description;
@JsonInclude(JsonInclude.Include.NON_NULL)
private BandwidthMessage message;
/**
* Default constructor.
*/
public BandwidthCallbackMessage() {
}
/**
* Initialization constructor.
* @param time String value for time.
* @param type String value for type.
* @param to String value for to.
* @param errorCode String value for errorCode.
* @param description String value for description.
* @param message BandwidthMessage value for message.
*/
public BandwidthCallbackMessage(
String time,
String type,
String to,
String errorCode,
String description,
BandwidthMessage message) {
this.time = time;
this.type = type;
this.to = to;
this.errorCode = errorCode;
this.description = description;
this.message = message;
}
/**
* Getter for Time.
* @return Returns the String
*/
@JsonGetter("time")
public String getTime() {
return time;
}
/**
* Setter for Time.
* @param time Value for String
*/
@JsonSetter("time")
public void setTime(String time) {
this.time = time;
}
/**
* Getter for Type.
* @return Returns the String
*/
@JsonGetter("type")
public String getType() {
return type;
}
/**
* Setter for Type.
* @param type Value for String
*/
@JsonSetter("type")
public void setType(String type) {
this.type = type;
}
/**
* Getter for To.
* @return Returns the String
*/
@JsonGetter("to")
public String getTo() {
return to;
}
/**
* Setter for To.
* @param to Value for String
*/
@JsonSetter("to")
public void setTo(String to) {
this.to = to;
}
/**
* Getter for ErrorCode.
* @return Returns the String
*/
@JsonGetter("errorCode")
public String getErrorCode() {
return errorCode;
}
/**
* Setter for ErrorCode.
* @param errorCode Value for String
*/
@JsonSetter("errorCode")
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
/**
* Getter for Description.
* @return Returns the String
*/
@JsonGetter("description")
public String getDescription() {
return description;
}
/**
* Setter for Description.
* @param description Value for String
*/
@JsonSetter("description")
public void setDescription(String description) {
this.description = description;
}
/**
* Getter for Message.
* @return Returns the BandwidthMessage
*/
@JsonGetter("message")
public BandwidthMessage getMessage() {
return message;
}
/**
* Setter for Message.
* @param message Value for BandwidthMessage
*/
@JsonSetter("message")
public void setMessage(BandwidthMessage message) {
this.message = message;
}
/**
* Converts this BandwidthCallbackMessage into string format.
* @return String representation of this class
*/
@Override
public String toString() {
return "BandwidthCallbackMessage [" + "time=" + time + ", type=" + type + ", to=" + to
+ ", errorCode=" + errorCode + ", description=" + description + ", message="
+ message + "]";
}
/**
* Builds a new {@link BandwidthCallbackMessage.Builder} object.
* Creates the instance with the state of the current model.
* @return a new {@link BandwidthCallbackMessage.Builder} object
*/
public Builder toBuilder() {
Builder builder = new Builder()
.time(getTime())
.type(getType())
.to(getTo())
.errorCode(getErrorCode())
.description(getDescription())
.message(getMessage());
return builder;
}
/**
* Class to build instances of {@link BandwidthCallbackMessage}.
*/
public static class Builder {
private String time;
private String type;
private String to;
private String errorCode;
private String description;
private BandwidthMessage message;
/**
* Setter for time.
* @param time String value for time.
* @return Builder
*/
public Builder time(String time) {
this.time = time;
return this;
}
/**
* Setter for type.
* @param type String value for type.
* @return Builder
*/
public Builder type(String type) {
this.type = type;
return this;
}
/**
* Setter for to.
* @param to String value for to.
* @return Builder
*/
public Builder to(String to) {
this.to = to;
return this;
}
/**
* Setter for errorCode.
* @param errorCode String value for errorCode.
* @return Builder
*/
public Builder errorCode(String errorCode) {
this.errorCode = errorCode;
return this;
}
/**
* Setter for description.
* @param description String value for description.
* @return Builder
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* Setter for message.
* @param message BandwidthMessage value for message.
* @return Builder
*/
public Builder message(BandwidthMessage message) {
this.message = message;
return this;
}
/**
* Builds a new {@link BandwidthCallbackMessage} object using the set fields.
* @return {@link BandwidthCallbackMessage}
*/
public BandwidthCallbackMessage build() {
return new BandwidthCallbackMessage(time, type, to, errorCode, description, message);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy