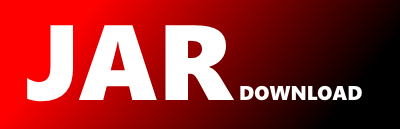
com.baomidou.kisso.common.util.HttpUtil Maven / Gradle / Ivy
Show all versions of kisso Show documentation
/*
* Copyright (c) 2011-2020, hubin ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.baomidou.kisso.common.util;
import com.baomidou.kisso.SSOConfig;
import lombok.extern.slf4j.Slf4j;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.util.Map;
/**
*
* HTTP工具类
*
*
* @author hubin
* @since 2017-07-17
*/
@Slf4j
public class HttpUtil {
public static final String XML_HTTP_REQUEST = "XMLHttpRequest";
public static final String X_REQUESTED_WITH = "X-Requested-With";
/**
*
* 判断请求是否为 AJAX
*
*
* @param request 当前请求
* @return
*/
public static boolean isAjax(HttpServletRequest request) {
return XML_HTTP_REQUEST.equals(request.getHeader(X_REQUESTED_WITH));
}
/**
*
* AJAX 设置 response 返回状态
*
*
* @param response
* @param status HTTP 状态码
* @param tip
*/
public static void ajaxStatus(HttpServletResponse response, int status, String tip) {
try {
response.setContentType("text/html;charset=" + SSOConfig.getSSOEncoding());
response.setStatus(status);
PrintWriter out = response.getWriter();
out.print(tip);
out.flush();
} catch (IOException e) {
log.error(e.getMessage());
}
}
/**
*
* 获取当前 URL 包含查询条件
*
*
* @param request
* @param encode URLEncoder编码格式
* @return
* @throws IOException
*/
public static String getQueryString(HttpServletRequest request, String encode) throws IOException {
String url = request.getRequestURL().toString();
StringBuffer sb = new StringBuffer(url);
String query = request.getQueryString();
if (query != null && query.length() > 0) {
sb.append(url.contains("?") ? "&" : "?").append(query);
}
return URLEncoder.encode(sb.toString(), encode);
}
/**
*
* getRequestURL是否包含在URL之内
*
*
* @param request
* @param url 参数为以';'分割的URL字符串
* @return
*/
public static boolean inContainURL(HttpServletRequest request, String url) {
boolean result = false;
if (url != null && !"".equals(url.trim())) {
String[] urlArr = url.split(";");
StringBuffer reqUrl = new StringBuffer(request.getRequestURL());
for (int i = 0; i < urlArr.length; i++) {
if (reqUrl.indexOf(urlArr[i]) > 1) {
result = true;
break;
}
}
}
return result;
}
/**
*
* URLEncoder 返回地址
*
*
* @param url 跳转地址
* @param retParam 返回地址参数名
* @param retUrl 返回地址
* @return
*/
public static String encodeRetURL(String url, String retParam, String retUrl) {
return encodeRetURL(url, retParam, retUrl, null);
}
/**
*
* URLEncoder 返回地址
*
*
* @param url 跳转地址
* @param retParam 返回地址参数名
* @param retUrl 返回地址
* @param data 携带参数
* @return
*/
public static String encodeRetURL(String url, String retParam, String retUrl, Map data) {
if (url == null) {
return null;
}
StringBuffer retStr = new StringBuffer(url);
retStr.append(url.contains("?") ? "&" : "?");
retStr.append(retParam);
retStr.append("=");
try {
retStr.append(URLEncoder.encode(retUrl, SSOConfig.getSSOEncoding()));
} catch (UnsupportedEncodingException e) {
log.error("encodeRetURL error.{} , {}", url, e.getMessage());
}
if (data != null) {
for (Map.Entry entry : data.entrySet()) {
retStr.append("&").append(entry.getKey()).append("=").append(entry.getValue());
}
}
return retStr.toString();
}
/**
*
* URLDecoder 解码地址
*
*
* @param url 解码地址
* @return
*/
public static String decodeURL(String url) {
if (url == null) {
return null;
}
String retUrl = "";
try {
retUrl = URLDecoder.decode(url, SSOConfig.getSSOEncoding());
} catch (UnsupportedEncodingException e) {
log.error("encodeRetURL error.{} ,{}", url, e.getMessage());
}
return retUrl;
}
/**
*
* GET 请求
*
*
* @param request
* @return boolean
*/
public static boolean isGet(HttpServletRequest request) {
if ("GET".equalsIgnoreCase(request.getMethod())) {
return true;
}
return false;
}
/**
*
* POST 请求
*
*
* @param request
* @return boolean
*/
public static boolean isPost(HttpServletRequest request) {
if ("POST".equalsIgnoreCase(request.getMethod())) {
return true;
}
return false;
}
/**
*
* 请求重定向至地址 location
*
*
* @param response 请求响应
* @param location 重定向至地址
*/
public static void sendRedirect(HttpServletResponse response, String location) {
try {
response.sendRedirect(location);
} catch (IOException e) {
log.error("sendRedirect location:{} ,{}", location, e.getMessage());
}
}
/**
*
* 获取Request Playload 内容
*
*
* @param request
* @return Request Playload 内容
*/
public static String requestPlayload(HttpServletRequest request) throws IOException {
StringBuilder stringBuilder = new StringBuilder();
BufferedReader bufferedReader = null;
try {
InputStream inputStream = request.getInputStream();
if (inputStream != null) {
bufferedReader = new BufferedReader(new InputStreamReader(inputStream));
char[] charBuffer = new char[128];
int bytesRead = -1;
while ((bytesRead = bufferedReader.read(charBuffer)) > 0) {
stringBuilder.append(charBuffer, 0, bytesRead);
}
} else {
stringBuilder.append("");
}
} catch (IOException ex) {
throw ex;
} finally {
if (bufferedReader != null) {
try {
bufferedReader.close();
} catch (IOException ex) {
throw ex;
}
}
}
return stringBuilder.toString();
}
/**
*
* 获取当前完整请求地址
*
*
* @param request
* @return 请求地址
*/
public static String getRequestUrl(HttpServletRequest request) {
StringBuffer url = new StringBuffer(request.getScheme());
// 请求协议 http,https
url.append("://");
// 请求服务器
url.append(request.getHeader("host"));
// 工程名
url.append(request.getRequestURI());
if (request.getQueryString() != null) {
// 请求参数
url.append("?").append(request.getQueryString());
}
return url.toString();
}
}