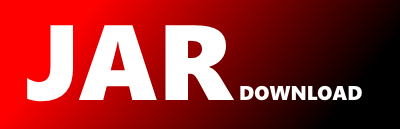
com.baomidou.mybatisplus.extension.service.IService Maven / Gradle / Ivy
Show all versions of mybatis-plus-extension Show documentation
/*
* Copyright (c) 2011-2016, hubin ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.baomidou.mybatisplus.extension.service;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
/**
*
* 顶级 Service
*
*
* @author hubin
* @since 2018-06-23
*/
public interface IService {
/**
*
* 插入一条记录(选择字段,策略插入)
*
*
* @param entity 实体对象
* @return boolean
*/
boolean save(T entity);
/**
*
* 插入(批量),该方法不适合 Oracle
*
*
* @param entityList 实体对象集合
* @return boolean
*/
boolean saveBatch(Collection entityList);
/**
*
* 插入(批量)
*
*
* @param entityList 实体对象集合
* @param batchSize 插入批次数量
* @return boolean
*/
boolean saveBatch(Collection entityList, int batchSize);
/**
*
* 批量修改插入
*
*
* @param entityList 实体对象集合
* @return boolean
*/
boolean saveOrUpdateBatch(Collection entityList);
/**
*
* 批量修改插入
*
*
* @param entityList 实体对象集合
* @param batchSize
* @return boolean
*/
boolean saveOrUpdateBatch(Collection entityList, int batchSize);
/**
*
* 根据 ID 删除
*
*
* @param id 主键ID
* @return boolean
*/
boolean removeById(Serializable id);
/**
*
* 根据 columnMap 条件,删除记录
*
*
* @param columnMap 表字段 map 对象
* @return boolean
*/
boolean removeByMap(Map columnMap);
/**
*
* 根据 entity 条件,删除记录
*
*
* @param wrapper 实体包装类 {@link Wrapper}
* @return boolean
*/
boolean remove(Wrapper wrapper);
/**
*
* 删除(根据ID 批量删除)
*
*
* @param idList 主键ID列表
* @return boolean
*/
boolean removeByIds(Collection extends Serializable> idList);
/**
*
* 根据 ID 选择修改
*
*
* @param entity 实体对象
* @return boolean
*/
boolean updateById(T entity);
/**
*
* 根据 whereEntity 条件,更新记录
*
*
* @param entity 实体对象
* @param wrapper 实体包装类 {@link Wrapper}
* @return boolean
*/
boolean update(T entity, Wrapper wrapper);
/**
*
* 根据ID 批量更新
*
*
* @param entityList 实体对象集合
* @return boolean
*/
boolean updateBatchById(Collection entityList);
/**
*
* 根据ID 批量更新
*
*
* @param entityList 实体对象集合
* @param batchSize 更新批次数量
* @return boolean
*/
boolean updateBatchById(Collection entityList, int batchSize);
/**
*
* TableId 注解存在更新记录,否插入一条记录
*
*
* @param entity 实体对象
* @return boolean
*/
boolean saveOrUpdate(T entity);
/**
*
* 根据 ID 查询
*
*
* @param id 主键ID
* @return T
*/
T getById(Serializable id);
/**
*
* 查询(根据ID 批量查询)
*
*
* @param idList 主键ID列表
* @return Collection
*/
Collection listByIds(Collection extends Serializable> idList);
/**
*
* 查询(根据 columnMap 条件)
*
*
* @param columnMap 表字段 map 对象
* @return Collection
*/
Collection listByMap(Map columnMap);
/**
*
* 根据 Wrapper,查询一条记录
*
*
* @param wrapper 实体对象
* @return T
*/
T getOne(Wrapper wrapper);
/**
*
* 根据 Wrapper,查询一条记录
*
*
* @param wrapper {@link Wrapper}
* @return
*/
Map getMap(Wrapper wrapper);
/**
*
* 根据 Wrapper,查询一条记录
*
*
* @param wrapper {@link Wrapper}
* @return Object
*/
Object getObj(Wrapper wrapper);
/**
*
* 根据 Wrapper 条件,查询总记录数
*
*
* @param wrapper 实体对象
* @return int
*/
int count(Wrapper wrapper);
/**
*
* 查询列表
*
*
* @param wrapper 实体包装类 {@link Wrapper}
* @return
*/
List list(Wrapper wrapper);
/**
*
* 翻页查询
*
*
* @param page 翻页对象
* @param wrapper 实体包装类 {@link Wrapper}
* @return
*/
IPage page(IPage page, Wrapper wrapper);
/**
*
* 查询列表
*
*
* @param wrapper {@link Wrapper}
* @return
*/
List