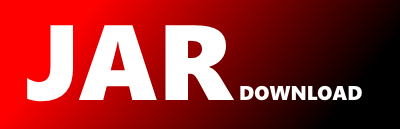
com.baomidou.mybatisplus.extension.activerecord.Model Maven / Gradle / Ivy
Show all versions of mybatis-plus-extension Show documentation
/*
* Copyright (c) 2011-2020, hubin ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.baomidou.mybatisplus.extension.activerecord;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.baomidou.mybatisplus.core.enums.SqlMethod;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.core.toolkit.Assert;
import com.baomidou.mybatisplus.core.toolkit.Constants;
import com.baomidou.mybatisplus.core.toolkit.StringUtils;
import com.baomidou.mybatisplus.core.toolkit.sql.SqlHelper;
import com.baomidou.mybatisplus.extension.toolkit.SqlRunner;
import org.apache.ibatis.session.SqlSession;
import org.springframework.transaction.annotation.Transactional;
import java.io.Serializable;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
*
* ActiveRecord 模式 CRUD
*
*
* @param
* @author hubin
* @since 2016-11-06
*/
public abstract class Model implements Serializable {
private static final long serialVersionUID = 1L;
/**
*
* 插入(字段选择插入)
*
*/
@Transactional(rollbackFor = Exception.class)
public boolean insert() {
try (SqlSession session = sqlSession()) {
return SqlHelper.retBool(session.insert(sqlStatement(SqlMethod.INSERT_ONE), this));
}
}
/**
*
* 插入 OR 更新
*
*/
@Transactional(rollbackFor = Exception.class)
public boolean insertOrUpdate() {
if (StringUtils.checkValNull(pkVal())) {
// insert
return insert();
} else {
/*
* 更新成功直接返回,失败执行插入逻辑
*/
return updateById() || insert();
}
}
/**
*
* 根据 ID 删除
*
*
* @param id 主键ID
* @return
*/
@Transactional(rollbackFor = Exception.class)
public boolean deleteById(Serializable id) {
try (SqlSession session = sqlSession()) {
return SqlHelper.delBool(session.delete(sqlStatement(SqlMethod.DELETE_BY_ID), id));
}
}
/**
*
* 根据主键删除
*
*
* @return
*/
@Transactional(rollbackFor = Exception.class)
public boolean deleteById() {
Assert.isFalse(StringUtils.checkValNull(pkVal()), "deleteById primaryKey is null.");
return deleteById(pkVal());
}
/**
*
* 删除记录
*
*
* @param wrapper
* @return
*/
@Transactional(rollbackFor = Exception.class)
public boolean delete(Wrapper wrapper) {
Map map = new HashMap<>(1);
map.put(Constants.WRAPPER, wrapper);
try (SqlSession session = sqlSession()) {
return SqlHelper.delBool(session.delete(sqlStatement(SqlMethod.DELETE), map));
}
}
/**
*
* 更新(字段选择更新)
*
*/
@Transactional(rollbackFor = Exception.class)
public boolean updateById() {
Assert.isFalse(StringUtils.checkValNull(pkVal()), "updateById primaryKey is null.");
// updateById
Map map = new HashMap<>(1);
map.put(Constants.ENTITY, this);
try(SqlSession sqlSession = sqlSession()) {
return SqlHelper.retBool(sqlSession.update(sqlStatement(SqlMethod.UPDATE_BY_ID), map));
}
}
/**
*
* 执行 SQL 更新
*
*
* @param wrapper
* @return
*/
@Transactional(rollbackFor = Exception.class)
public boolean update(Wrapper wrapper) {
Map map = new HashMap<>(2);
map.put(Constants.ENTITY, this);
map.put(Constants.WRAPPER, wrapper);
// update
try (SqlSession session = sqlSession()) {
return SqlHelper.retBool(session.update(sqlStatement(SqlMethod.UPDATE), map));
}
}
/**
*
* 查询所有
*
*
* @return
*/
public List selectAll() {
try (SqlSession session = sqlSession()) {
return session.selectList(sqlStatement(SqlMethod.SELECT_LIST));
}
}
/**
*
* 根据 ID 查询
*
*
* @param id 主键ID
* @return
*/
public T selectById(Serializable id) {
try (SqlSession session = sqlSession()) {
return session.selectOne(sqlStatement(SqlMethod.SELECT_BY_ID), id);
}
}
/**
*
* 根据主键查询
*
*
* @return
*/
public T selectById() {
Assert.isFalse(StringUtils.checkValNull(pkVal()), "selectById primaryKey is null.");
return selectById(pkVal());
}
/**
*
* 查询总记录数
*
*
* @param wrapper
* @return
*/
public List selectList(Wrapper wrapper) {
Map map = new HashMap<>(1);
map.put(Constants.WRAPPER, wrapper);
try (SqlSession session = sqlSession()) {
return session.selectList(sqlStatement(SqlMethod.SELECT_LIST), map);
}
}
/**
*
* 查询一条记录
*
*
* @param wrapper
* @return
*/
public T selectOne(Wrapper wrapper) {
return SqlHelper.getObject(selectList(wrapper));
}
/**
*
* 翻页查询
*
*
* @param page 翻页查询条件
* @param wrapper
* @return
*/
public IPage selectPage(IPage page, Wrapper wrapper) {
Map map = new HashMap<>(2);
map.put(Constants.WRAPPER, SqlHelper.fillWrapper(page, wrapper));
map.put("page", page);
try (SqlSession session = sqlSession()) {
page.setRecords(session.selectList(sqlStatement(SqlMethod.SELECT_PAGE), map));
}
return page;
}
/**
*
* 查询总数
*
*
* @param wrapper
* @return
*/
public int selectCount(Wrapper wrapper) {
Map map = new HashMap<>(1);
map.put(Constants.WRAPPER, wrapper);
try (SqlSession session = sqlSession()) {
return SqlHelper.retCount(session.selectOne(sqlStatement(SqlMethod.SELECT_COUNT), map));
}
}
/**
*
* 执行 SQL
*
*/
public SqlRunner sql() {
return new SqlRunner(getClass());
}
/**
*
* 获取Session 默认自动提交
*
*/
protected SqlSession sqlSession() {
return SqlHelper.sqlSession(getClass());
}
/**
* 获取SqlStatement
*
* @param sqlMethod
* @return
*/
protected String sqlStatement(SqlMethod sqlMethod) {
return sqlStatement(sqlMethod.getMethod());
}
/**
* 获取SqlStatement
*
* @param sqlMethod
* @return
*/
protected String sqlStatement(String sqlMethod) {
return SqlHelper.table(getClass()).getSqlStatement(sqlMethod);
}
/**
* 主键值
*/
protected abstract Serializable pkVal();
}