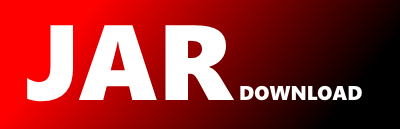
com.baomidou.mybatisplus.extension.toolkit.SqlRunner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-plus-extension Show documentation
Show all versions of mybatis-plus-extension Show documentation
An enhanced toolkit of Mybatis to simplify development.
/*
* Copyright (c) 2011-2023, baomidou ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.baomidou.mybatisplus.extension.toolkit;
import com.baomidou.mybatisplus.core.assist.ISqlRunner;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.core.toolkit.CollectionUtils;
import com.baomidou.mybatisplus.core.toolkit.GlobalConfigUtils;
import com.baomidou.mybatisplus.core.toolkit.StringUtils;
import org.apache.ibatis.logging.Log;
import org.apache.ibatis.logging.LogFactory;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionUtils;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
import java.util.Map;
import java.util.Optional;
/**
* SqlRunner 执行 SQL
*
* @author Caratacus
* @since 2016-12-11
*/
public class SqlRunner implements ISqlRunner {
private final Log log = LogFactory.getLog(SqlRunner.class);
// 单例Query
public static final SqlRunner DEFAULT = new SqlRunner();
private Class> clazz;
public SqlRunner() {
}
public SqlRunner(Class> clazz) {
this.clazz = clazz;
}
/**
* 获取默认的SqlQuery(适用于单库)
*
* @return ignore
*/
public static SqlRunner db() {
return DEFAULT;
}
/**
* 根据当前class对象获取SqlQuery(适用于多库)
*
* @param clazz ignore
* @return ignore
*/
public static SqlRunner db(Class> clazz) {
return new SqlRunner(clazz);
}
@Transactional
@Override
public boolean insert(String sql, Object... args) {
SqlSession sqlSession = sqlSession();
try {
return SqlHelper.retBool(sqlSession.insert(INSERT, sqlMap(sql, args)));
} finally {
closeSqlSession(sqlSession);
}
}
@Transactional
@Override
public boolean delete(String sql, Object... args) {
SqlSession sqlSession = sqlSession();
try {
return SqlHelper.retBool(sqlSession.delete(DELETE, sqlMap(sql, args)));
} finally {
closeSqlSession(sqlSession);
}
}
/**
* 获取sqlMap参数
*
* @param sql 指定参数的格式: {0}, {1}
* @param args 仅支持String
* @return ignore
*/
private Map sqlMap(String sql, Object... args) {
Map sqlMap = CollectionUtils.newHashMapWithExpectedSize(1);
sqlMap.put(SQL, StringUtils.sqlArgsFill(sql, args));
return sqlMap;
}
/**
* 获取sqlMap参数
*
* @param sql 指定参数的格式: {0}, {1}
* @param page 分页模型
* @param args 仅支持String
* @return ignore
*/
private Map sqlMap(String sql, IPage> page, Object... args) {
Map sqlMap = CollectionUtils.newHashMapWithExpectedSize(2);
sqlMap.put(PAGE, page);
sqlMap.put(SQL, StringUtils.sqlArgsFill(sql, args));
return sqlMap;
}
@Transactional
@Override
public boolean update(String sql, Object... args) {
SqlSession sqlSession = sqlSession();
try {
return SqlHelper.retBool(sqlSession.update(UPDATE, sqlMap(sql, args)));
} finally {
closeSqlSession(sqlSession);
}
}
/**
* 根据sql查询Map结果集
* SqlRunner.db().selectList("select * from tbl_user where name={0}", "Caratacus")
*
* @param sql sql语句,可添加参数,格式:{0},{1}
* @param args 只接受String格式
* @return ignore
*/
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy