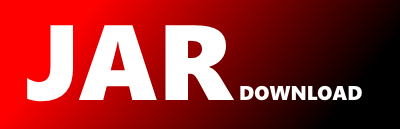
com.baomidou.framework.common.Pinyin4jHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-wind Show documentation
Show all versions of spring-wind Show documentation
spring-wind extension of spring framework.
The newest version!
/**
* Copyright (c) 2011-2014, [email protected].
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.baomidou.framework.common;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
import com.baomidou.framework.exception.SpringWindException;
import net.sourceforge.pinyin4j.PinyinHelper;
import net.sourceforge.pinyin4j.format.HanyuPinyinCaseType;
import net.sourceforge.pinyin4j.format.HanyuPinyinOutputFormat;
import net.sourceforge.pinyin4j.format.HanyuPinyinToneType;
import net.sourceforge.pinyin4j.format.exception.BadHanyuPinyinOutputFormatCombination;
/**
*
* Pinyin4j 简繁体转拼音辅助类
*
*
* @author [email protected]
* @Date 2016-04-12
*/
public class Pinyin4jHelper {
/**
*
* 拼音首字母,获取第一个结果。 (北京市长:bjsz,bjsc 返回 bjsz)
*
*
* @param chines
* 汉字
* @return 拼音首字母
*/
public static String converterToFirstSpell( String chines ) {
String pinyin = converterToAllFirstSpell(chines);
if ( pinyin != null && pinyin.contains(",") ) {
return pinyin.split(",")[0];
}
return pinyin;
}
/**
*
* 汉字转换位汉语拼音首字母,英文字符不变,特殊字符丢失 支持多音字。 (北京市长:bjsz,bjsc)
*
*
* @param chines
* 汉字
* @return 拼音首字母
*/
public static String converterToAllFirstSpell( String chines ) {
StringBuffer pinyinName = new StringBuffer();
char[] nameChar = chines.toCharArray();
HanyuPinyinOutputFormat defaultFormat = new HanyuPinyinOutputFormat();
defaultFormat.setCaseType(HanyuPinyinCaseType.LOWERCASE);
defaultFormat.setToneType(HanyuPinyinToneType.WITHOUT_TONE);
for ( int i = 0 ; i < nameChar.length ; i++ ) {
if ( nameChar[i] > 128 ) {
try {
/* 取得当前汉字的所有全拼 */
String[] strs = PinyinHelper.toHanyuPinyinStringArray(nameChar[i], defaultFormat);
if ( strs != null ) {
for ( int j = 0 ; j < strs.length ; j++ ) {
// 取首字母
pinyinName.append(strs[j].charAt(0));
if ( j != strs.length - 1 ) {
pinyinName.append(",");
}
}
}
} catch ( BadHanyuPinyinOutputFormatCombination e ) {
throw new SpringWindException(e);
}
} else {
pinyinName.append(nameChar[i]);
}
pinyinName.append(" ");
}
return parseTheChineseByObject(discountTheChinese(pinyinName.toString()));
}
/**
*
* 汉语全拼,获取第一个结果。(北京市长:beijingshizhang,beijingshichang 返回 beijingshizhang)
*
*
* @param chines
* 汉字
* @return 拼音
*/
public static String converterToSpell( String chines ) {
String pinyin = converterToAllSpell(chines);
if ( pinyin != null && pinyin.contains(",") ) {
return pinyin.split(",")[0];
}
return pinyin;
}
/**
*
* 汉字转换位汉语全拼,英文字符不变,特殊字符丢失
* 支持多音字,生成方式如(北京市长:beijingshizhang,beijingshichang)
*
*
* @param chines
* 汉字
* @return 拼音
*/
public static String converterToAllSpell( String chines ) {
StringBuffer pinyinName = new StringBuffer();
char[] nameChar = chines.toCharArray();
HanyuPinyinOutputFormat defaultFormat = new HanyuPinyinOutputFormat();
defaultFormat.setCaseType(HanyuPinyinCaseType.LOWERCASE);
defaultFormat.setToneType(HanyuPinyinToneType.WITHOUT_TONE);
for ( int i = 0 ; i < nameChar.length ; i++ ) {
if ( nameChar[i] > 128 ) {
try {
/* 取得当前汉字的所有全拼 */
String[] strs = PinyinHelper.toHanyuPinyinStringArray(nameChar[i], defaultFormat);
if ( strs != null ) {
for ( int j = 0 ; j < strs.length ; j++ ) {
pinyinName.append(strs[j]);
if ( j != strs.length - 1 ) {
pinyinName.append(",");
}
}
}
} catch ( BadHanyuPinyinOutputFormatCombination e ) {
e.printStackTrace();
}
} else {
pinyinName.append(nameChar[i]);
}
pinyinName.append(" ");
}
return parseTheChineseByObject(discountTheChinese(pinyinName.toString()));
}
/**
*
* 去除多音字重复数据
*
*
* @param theStr
* 多音字
* @return
*/
protected static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy