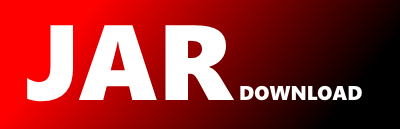
com.baomidou.framework.common.util.DateUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-wind Show documentation
Show all versions of spring-wind Show documentation
spring-wind extension of spring framework.
The newest version!
/**
* Copyright (c) 2011-2014, [email protected].
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.baomidou.framework.common.util;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.Locale;
import org.apache.commons.lang.time.DateUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* 日期通用处理工具类
*
*
* @author hubin
* @Date 2016-04-16
*/
public class DateUtil extends DateUtils {
private static Logger logger = LoggerFactory.getLogger(DateUtil.class);
/** 毫秒 */
public final static long MS = 1;
/** 每秒钟的毫秒数 */
public final static long SECOND_MS = MS * 1000;
/** 每分钟的毫秒数 */
public final static long MINUTE_MS = SECOND_MS * 60;
/** 每小时的毫秒数 */
public final static long HOUR_MS = MINUTE_MS * 60;
/** 每天的毫秒数 */
public final static long DAY_MS = HOUR_MS * 24;
/** 标准日期(不含时间)格式化器 */
private final static SimpleDateFormat NORM_DATE_FORMAT = new SimpleDateFormat("yyyy-MM-dd");
/** 标准日期时间格式化器 */
private final static SimpleDateFormat NORM_DATETIME_FORMAT = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
/** HTTP日期时间格式化器 */
private final static SimpleDateFormat HTTP_DATETIME_FORMAT = new SimpleDateFormat("EEE, dd MMM yyyy HH:mm:ss z",
Locale.US);
/**
*
* 当前时间,格式 yyyy-MM-dd HH:mm:ss
*
* @return 当前时间的标准形式字符串
*
*/
public static String now() {
return formatDateTime(new Date());
}
/**
*
* 当前日期,格式 yyyy-MM-dd
*
* @return 当前日期的标准形式字符串
*
*/
public static String today() {
return formatDate(new Date());
}
// ------------------------------------ Format start
// ----------------------------------------------
/**
*
* 根据特定格式格式化日期
*
* @param date
* 被格式化的日期
*
* @param format
* 格式
*
* @return 格式化后的字符串
*
*/
public static String format(Date date, String format) {
return new SimpleDateFormat(format).format(date);
}
/**
*
* 格式 yyyy-MM-dd HH:mm:ss
*
* @param date
* 被格式化的日期
*
* @return 格式化后的日期
*
*/
public static String formatDateTime(Date date) {
// return format(d, "yyyy-MM-dd HH:mm:ss");
return NORM_DATETIME_FORMAT.format(date);
}
/**
*
* 格式化为Http的标准日期格式
*
* @param date
* 被格式化的日期
*
* @return HTTP标准形式日期字符串
*
*/
public static String formatHttpDate(Date date) {
// return new SimpleDateFormat("EEE, dd MMM yyyy HH:mm:ss z",
// Locale.US).format(date);
return HTTP_DATETIME_FORMAT.format(date);
}
/**
*
* 格式 yyyy-MM-dd
*
* @param date
* 被格式化的日期
*
* @return 格式化后的字符串
*
*/
public static String formatDate(Date date) {
// return format(d, "yyyy-MM-dd");
return NORM_DATE_FORMAT.format(date);
}
// ------------------------------------ Format end
// ----------------------------------------------
// ------------------------------------ Parse start
// ----------------------------------------------
/**
*
* 将特定格式的日期转换为Date对象
*
* @param dateString
* 特定格式的日期
*
* @param format
* 格式,例如yyyy-MM-dd
*
* @return 日期对象
*
*/
public static Date parse(String dateString, String format) {
try {
return (new SimpleDateFormat(format)).parse(dateString);
} catch (ParseException e) {
logger.error("Parse " + dateString + " with format " + format + " error!", e);
}
return null;
}
/**
*
* 格式yyyy-MM-dd HH:mm:ss
*
* @param dateString
* 标准形式的时间字符串
*
* @return 日期对象
*
*/
public static Date parseDateTime(String dateString) {
// return parse(s, "yyyy-MM-dd HH:mm:ss");
try {
return NORM_DATETIME_FORMAT.parse(dateString);
} catch (ParseException e) {
logger.error("Parse " + dateString + " with format " + NORM_DATETIME_FORMAT.toPattern() + " error!", e);
}
return null;
}
/**
*
* 格式yyyy-MM-dd
*
* @param dateString
* 标准形式的日期字符串
*
* @return 日期对象
*
*/
public static Date parseDate(String dateString) {
// return parse(s, "yyyy-MM-dd");
try {
return NORM_DATE_FORMAT.parse(dateString);
} catch (ParseException e) {
logger.error("Parse " + dateString + " with format " + NORM_DATE_FORMAT.toPattern() + " error!", e);
}
return null;
}
// ------------------------------------ Parse end
// ----------------------------------------------
/**
*
* 获取指定日期偏移指定时间后的时间
*
* @param date
* 基准日期
*
* @param calendarField
* 偏移的粒度大小(小时、天、月等)使用Calendar中的常数
*
* @param offsite
* 偏移量,正数为向后偏移,负数为向前偏移
*
* @return 偏移后的日期
*
*/
public static Date getOffsiteDate(Date date, int calendarField, int offsite) {
Calendar cal = Calendar.getInstance();
cal.setTime(date);
cal.add(calendarField, offsite);
return cal.getTime();
}
/**
*
* 判断两个日期相差的时长
*
* 返回 minuend - subtrahend 的差
*
* @param subtrahend
* 减数日期
*
* @param minuend
* 被减数日期
*
* @param diffField
* 相差的选项:相差的天、小时
*
* @return 日期差
*
*/
public static long dateDiff(Date subtrahend, Date minuend, long diffField) {
long diff = minuend.getTime() - subtrahend.getTime();
return diff / diffField;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy