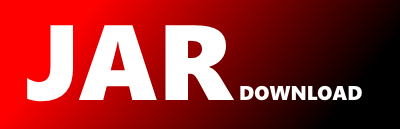
com.barchart.udt.nio.SelectorProviderUDT Maven / Gradle / Ivy
/**
* Copyright (C) 2009-2012 Barchart, Inc.
*
* All rights reserved. Licensed under the OSI BSD License.
*
* http://www.opensource.org/licenses/bsd-license.php
*/
package com.barchart.udt.nio;
import java.io.IOException;
import java.nio.channels.DatagramChannel;
import java.nio.channels.Pipe;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.nio.channels.spi.AbstractSelector;
import java.nio.channels.spi.SelectorProvider;
import com.barchart.udt.SocketUDT;
import com.barchart.udt.TypeUDT;
/**
* you must use the same system-wide provider instance for the same
* {@link TypeUDT} of UDT channels and UDT selectors;
*/
public class SelectorProviderUDT extends SelectorProvider {
/**
* system-wide provider instance, for {@link TypeUDT#DATAGRAM} UDT sockets
*/
public static final SelectorProviderUDT DATAGRAM = //
new SelectorProviderUDT(TypeUDT.DATAGRAM);
/**
* system-wide provider instance, for {@link TypeUDT#STREAM} UDT sockets
*/
public static final SelectorProviderUDT STREAM = //
new SelectorProviderUDT(TypeUDT.STREAM);
/**
* {@link TypeUDT} of UDT sockets generated by this provider
*/
public final TypeUDT type;
protected SelectorProviderUDT(TypeUDT type) {
this.type = type;
}
protected volatile int acceptQueueSize = SocketUDT.DEFAULT_ACCEPT_QUEUE_SIZE;
public int getAcceptQueueSize() {
return acceptQueueSize;
}
public void setAcceptQueueSize(int queueSize) {
acceptQueueSize = queueSize;
}
protected volatile int maxSelectorSize = SocketUDT.DEFAULT_MAX_SELECTOR_SIZE;
public int getMaxSelectorSize() {
return maxSelectorSize;
}
public void setMaxSelectorSize(int selectorSize) {
maxSelectorSize = selectorSize;
}
protected volatile int maxConnectorSize = SocketUDT.DEFAULT_CONNECTOR_POOL_SIZE;
public int getMaxConnectorSize() {
return maxConnectorSize;
}
public void setMaxConnectorSize(int connectorSize) {
maxConnectorSize = connectorSize;
}
//
/**
* feature not available
*/
@Override
public DatagramChannel openDatagramChannel() throws IOException {
throw new RuntimeException("feature not available");
}
/**
* feature not available
*/
@Override
public Pipe openPipe() throws IOException {
throw new RuntimeException("feature not available");
}
@Override
public AbstractSelector openSelector() throws IOException {
return new SelectorUDT(this, maxSelectorSize, maxConnectorSize);
}
@Override
public ServerSocketChannel openServerSocketChannel() throws IOException {
SocketUDT serverSocketUDT = new SocketUDT(type);
return new ChannelServerSocketUDT(this, serverSocketUDT);
}
@Override
public SocketChannel openSocketChannel() throws IOException {
SocketUDT socketUDT = new SocketUDT(type);
return new ChannelSocketUDT(this, socketUDT);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy