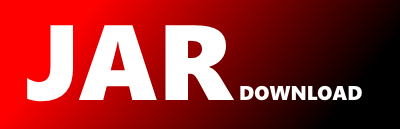
com.barchart.util.concurrent.FutureCallback Maven / Gradle / Ivy
/**
* Copyright (C) 2011-2012 Barchart, Inc.
*
* All rights reserved. Licensed under the OSI BSD License.
*
* http://www.opensource.org/licenses/bsd-license.php
*/
package com.barchart.util.concurrent;
import java.util.concurrent.Future;
/**
*
* Adds listener notification methods to {@link Future} to allow immediate
* notification when a result is available.
*
*
*
* Example:
*
*
*
*
*
* FutureListener<Employee> listener = new FutureListener<Employee> {
* public void resultAvailable(Future<Employee> result) {
* System.out.println("Got deferred result: " + result.get());
* }
* }
*
* FutureCallback<Employee> result = myDataStore.getEmployee(id);
* result.addResultListener(listener);
*
*
*
*
*
* In this example, getEmployee() returns a FutureCallback object that it will
* later use to return a result asynchronously. When it has a result, it calls
* result.succeed(Employee e), which in turn notifies any listeners registered
* with result.addResultListener(). If the call fails, the owner may call
* result.fail(Exception ex) to report the error. If a FutureCallback has
* failed, any future calls to result.get() throw an ExecutionException with the
* original exception as its cause.
*
*
*
* This class is thread-safe.
*
*
* @author jeremy
* @see Future
* @see FutureListener
* @see FutureCallbackTask
* @param
* The result type
*/
public interface FutureCallback> extends
Future {
/**
* Register a listener for this deferred result. If the result is already
* available, the listener may be called synchronously, so you should
* generally avoid any blocking code in the callback.
*
* @param listener
* The listener object
*/
public T addResultListener(FutureListener listener);
/**
* Notify listeners that a result is available. Returns the current
* FutureCallbackTask instance to allow simple synchronous returns when the
* result is already available:
* return new FutureCallbackTask
*
* @param result_
* The deferred result
* @return This FutureCallbackTask object (for chaining calls)
*/
public T succeed(V result);
/**
* Notify listeners that an error occurred. Returns the current
* FutureCallbackTask instance to allow simple synchronous returns when an
* error has already occurred:
* return new FutureCallbackTask
*
* @param error_
* The deferred error
*/
public T fail(Throwable error);
/**
* Get the return value, or null if a checked exception was caught.
*/
public V getUnchecked();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy