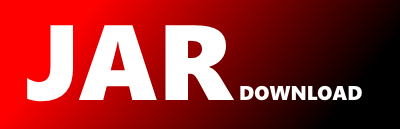
com.basho.riak.client.bucket.Bucket Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
/*
* This file is provided to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.basho.riak.client.bucket;
import com.basho.riak.client.IRiakObject;
import com.basho.riak.client.RiakException;
import com.basho.riak.client.convert.RiakKey;
import com.basho.riak.client.operations.CounterObject;
import com.basho.riak.client.operations.DeleteObject;
import com.basho.riak.client.operations.FetchObject;
import com.basho.riak.client.operations.MultiFetchObject;
import com.basho.riak.client.operations.RiakOperation;
import com.basho.riak.client.operations.StoreObject;
import com.basho.riak.client.query.indexes.FetchIndex;
import com.basho.riak.client.query.indexes.RiakIndex;
import com.basho.riak.client.query.StreamingOperation;
import java.util.List;
/**
* The primary interface for working with Key/Value data in Riak, a factory for key/value {@link RiakOperation}s.
*
* Provides convenience methods for creating {@link RiakOperation}s for storing
* byte[]
and String
data in Riak. Also provides
* methods for creating {@link RiakOperation}s for storing Java Bean style POJOs
* in Riak. A Bucket is a factory for {@link RiakOperation}s on Key/Value
* data.
*
*
* Gives access to all the {@link BucketProperties} that the underlying API
* transport exposes. NOTE: soon this will be *all* the {@link BucketProperties}
*
*
* Provides access to an {@link Iterable} for the keys in the bucket.
*
*
* @see StoreObject
* @see FetchObject
* @see DeleteObject
*
* @author russell
*/
public interface Bucket extends BucketProperties {
/**
* Get this Buckets name.
* @return the name of the bucket
*/
String getName();
/**
* Creates a {@link StoreObject} that will store a new {@link IRiakObject}.
*
* @param key the key to store the data under.
* @param value the data as a byte[]
* @return a {@link StoreObject}
* @see StoreObject
*/
StoreObject store(String key, byte[] value);
/**
* Creates a {@link StoreObject} that will store a new {@link IRiakObject}.
*
* @param key the key to store the data under.
* @param value the data as a string
* @return a {@link StoreObject}
* @see StoreObject
*/
StoreObject store(String key, String value);
/**
* Creates a {@link StoreObject} for storing o
of type
* T
on execute()
. T
must have
* a field annotated with {@link RiakKey}.
*
* @param the Type of o
* @param o the data to store
* @return a {@link StoreObject}
* @see StoreObject
*/
StoreObject store(T o);
/**
* Creates a {@link StoreObject} for storing o
of type
* T
at key
on execute()
.
*
* @param the Type of o
* @param o the data to store
* @param key the key
* @return a {@link StoreObject}
* @see StoreObject
*/
StoreObject store(String key, T o);
/**
* Creates a {@link FetchObject} that returns the data at key
* as an {@link IRiakObject} on execute()
.
*
* @param key the key
* @return a {@link FetchObject}
* @see FetchObject
*/
FetchObject fetch(String key);
/**
* Creates a {@link FetchObject} operation that returns the data at
* key
as an instance of type T
on
* execute()
.
*
* @param
* the Type to return
* @param key
* the key under which the data is stored
* @param type
* the Class of the type to return
* @return a {@link FetchObject}
* @see FetchObject
*/
FetchObject fetch(String key, Class type);
/**
* Creates a {@link FetchObject} operation that returns the data at
* o
's annotated {@link RiakKey} field as an instance of type
* T
on execute()
.
*
* @param
* the Type to return
* @param o
* an instance ot T
that has the key annotated with
* {@link RiakKey}
* @return a {@link FetchObject}
* @see FetchObject
*/
FetchObject fetch(T o);
/**
* Creates a {@link MultiFetchObject} that returns the datum at keys
* as a list of {@link IRiakObject} futures on execute()
.
*
* @param keys the keys
* @return a {@link MultiFetchObject}
* @see MultiFetchObject
*/
MultiFetchObject multiFetch(String[] keys);
/**
* Creates a {@link MultiFetchObject} operation that returns the datum at
* keys
as a list of type T
futures on
* execute()
.
*
* @param
* the Type to return
* @param keys
* the list of keys under which the data is stored
* @param type
* the Class of the type to return
* @return a {@link MultiFetchObject}
* @see MultiFetchObject
*/
MultiFetchObject multiFetch(List keys, Class type);
/**
* Creates a {@link MultiFetchObject} operation that returns the data at
* o
's annotated {@link RiakKey} field as a list of type
* T
futures on execute()
.
*
* @param
* the Type to return
* @param o
* a List of T
that has the keys annotated with
* {@link RiakKey}
* @return a {@link MultiFetchObject}
* @see MultiFetchObject
*/
MultiFetchObject multiFetch(List o);
/**
* Creates a {@link CounterObject} operation
* @param counter the name (key) for the counter
* @return a new {@link CounterObject}
*/
CounterObject counter(String counter);
/**
* Creates a {@link DeleteObject} operation that will delete the data at
* o
's {@link RiakKey} annotated field value on
* execute()
.
*
* @param
* the Type of o
* @param o
* an instance of T
with a value for the key in the
* field annotated by {@link RiakKey}
* @return a {@link DeleteObject}
* @see DeleteObject
*/
DeleteObject delete(T o);
/**
* Creates a {@link DeleteObject} operation that will delete the data at
* key
on execute()
.
*
* @param
* the Type of o
* @param o
* an instance of T
with a value for the key in the
* field annotated by {@link RiakKey}
* @return a {@link DeleteObject}
* @see DeleteObject
*/
DeleteObject delete(String key);
/**
* An {@link Iterable} view of the keys stored in this bucket.
* @return an {@link Iterable} of Strings.
* @throws RiakException
*/
StreamingOperation keys() throws RiakException;
/**
* Creates a {@link FetchIndex} operation for the given index name and type
*
* @param
* the index type (currently String or Long)
* @param index
* an index
* @return a {@link FetchIndex} operation for further configuration and
* execution
*/
FetchIndex fetchIndex(RiakIndex index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy