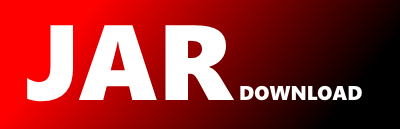
com.basho.riak.client.query.NodeStats Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
/*
* Copyright 2012 roach.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basho.riak.client.query;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.JsonToken;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.io.IOException;
import java.math.BigInteger;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* The encapsulation of the data returned by the Riak /stats
* operation.
*
* By implementing the {@link Iterable} interface it contains N sets
* of data where N is the number of connections the current client holds.
*
* Worth noting is that integer values are returned as {@link BigInteger} objects.
* This is done to match the unconstrained size provided by Riak.
*
* For example, using the HTTPClusterClient you can retrieve stats from all of your nodes:
*
*
* HTTPClusterConfig c = new HTTPClusterConfig(10);
* HTTPClientConfig cc HTTPClientConfig.defaults();
* c.addHosts(cc,"192.168.1.5:8098","192.168.1.6:8098","192.168.1.7:8098");
*
* IRiakClient riakClient = RiakFactory.newClient(c);
*
* for (NodeStats ns : riakClient.stats())
* {
* System.out.println(ns.nodename());
* Syste.out.println(ns.vnodeGets());
* }
*
*
{
/*
* This got rather tricky due to the HTTPClusterClient implementing RawClient
* and then using a pool of HTTClientAdapter objects which also implemented RawClient.
* I either had to make both return a List which meant the HTTPClientAdapter
* would always return a List containing one thing, then I'd have to combine
* those lists into one List in the HTTPClusterClient, or ... I could make
* this object Iterable. I went with the latter, implementing it as a
* psudo double-linked list
*/
private NodeStats next;
private NodeStats previous;
@JsonProperty private BigInteger vnode_gets;
@JsonProperty private BigInteger vnode_puts;
@JsonProperty private BigInteger vnode_index_reads;
@JsonProperty private BigInteger vnode_index_writes;
@JsonProperty private BigInteger vnode_index_writes_postings;
@JsonProperty private BigInteger vnode_index_deletes;
@JsonProperty private BigInteger vnode_index_deletes_postings;
@JsonProperty private BigInteger read_repairs;
@JsonProperty private BigInteger vnode_gets_total;
@JsonProperty private BigInteger vnode_puts_total;
@JsonProperty private BigInteger vnode_index_reads_total;
@JsonProperty private BigInteger vnode_index_writes_total;
@JsonProperty private BigInteger vnode_index_writes_postings_total;
@JsonProperty private BigInteger vnode_index_deletes_total;
@JsonProperty private BigInteger vnode_index_deletes_postings_total;
@JsonProperty private BigInteger node_gets;
@JsonProperty private BigInteger node_gets_total;
@JsonProperty private BigInteger node_get_fsm_time_mean;
@JsonProperty private BigInteger node_get_fsm_time_median;
@JsonProperty private BigInteger node_get_fsm_time_95;
@JsonProperty private BigInteger node_get_fsm_time_99;
@JsonProperty private BigInteger node_get_fsm_time_100;
@JsonProperty private BigInteger node_puts;
@JsonProperty private BigInteger node_puts_total;
@JsonProperty private BigInteger node_put_fsm_time_mean;
@JsonProperty private BigInteger node_put_fsm_time_median;
@JsonProperty private BigInteger node_put_fsm_time_95;
@JsonProperty private BigInteger node_put_fsm_time_99;
@JsonProperty private BigInteger node_put_fsm_time_100;
@JsonProperty private BigInteger node_get_fsm_siblings_mean;
@JsonProperty private BigInteger node_get_fsm_siblings_median;
@JsonProperty private BigInteger node_get_fsm_siblings_95;
@JsonProperty private BigInteger node_get_fsm_siblings_99;
@JsonProperty private BigInteger node_get_fsm_siblings_100;
@JsonProperty private BigInteger node_get_fsm_objsize_mean;
@JsonProperty private BigInteger node_get_fsm_objsize_median;
@JsonProperty private BigInteger node_get_fsm_objsize_95;
@JsonProperty private BigInteger node_get_fsm_objsize_99;
@JsonProperty private BigInteger node_get_fsm_objsize_100;
@JsonProperty private BigInteger read_repairs_total;
@JsonProperty private BigInteger coord_redirs_total;
@JsonProperty private BigInteger precommit_fail; // Riak 1.1
@JsonProperty private BigInteger postcommit_fail; // Riak 1.1
@JsonProperty private BigInteger cpu_nprocs;
@JsonProperty private BigInteger cpu_avg1;
@JsonProperty private BigInteger cpu_avg5;
@JsonProperty private BigInteger cpu_avg15;
@JsonProperty private BigInteger mem_total;
@JsonProperty private BigInteger mem_allocated;
@JsonProperty private String nodename;
@JsonProperty private String[] connected_nodes;
@JsonProperty private String sys_driver_version;
@JsonProperty private BigInteger sys_global_heaps_size;
@JsonProperty private String sys_heap_type;
@JsonProperty private BigInteger sys_logical_processors;
@JsonProperty private String sys_otp_release;
@JsonProperty private BigInteger sys_process_count;
@JsonProperty private boolean sys_smp_support;
@JsonProperty private String sys_system_version;
@JsonProperty private String sys_system_architecture;
@JsonProperty private boolean sys_threads_enabled;
@JsonProperty private BigInteger sys_thread_pool_size;
@JsonProperty private BigInteger sys_wordsize;
@JsonProperty private String[] ring_members;
@JsonProperty private BigInteger ring_num_partitions;
@JsonProperty private String ring_ownership;
@JsonProperty private BigInteger ring_creation_size;
@JsonProperty private String storage_backend;
@JsonProperty private BigInteger pbc_connects_total;
@JsonProperty private BigInteger pbc_connects;
@JsonProperty private BigInteger pbc_active;
@JsonProperty private String ssl_version;
@JsonProperty private String public_key_version;
@JsonProperty private String runtime_tools_version;
@JsonProperty private String basho_stats_version;
@JsonProperty private String riak_search_version;
@JsonProperty private String merge_index_version;
@JsonProperty private String luwak_version;
@JsonProperty private String skerl_version;
@JsonProperty private String riak_kv_version;
@JsonProperty private String bitcask_version;
@JsonProperty private String luke_version;
@JsonProperty private String erlang_js_version;
@JsonProperty private String mochiweb_version;
@JsonProperty private String inets_version;
@JsonProperty private String riak_pipe_version;
@JsonProperty private String riak_core_version;
@JsonProperty private String riak_sysmon_version;
@JsonProperty private String webmachine_version;
@JsonProperty private String crypto_version;
@JsonProperty private String os_mon_version;
@JsonProperty private String cluster_info_version;
@JsonProperty private String sasl_version;
@JsonProperty private String lager_version;
@JsonProperty private String basho_metrics_version;
@JsonProperty private String riak_control_version; // Riak 1.1
@JsonProperty private String stdlib_version;
@JsonProperty private String kernel_version;
@JsonProperty private BigInteger executing_mappers;
@JsonProperty private BigInteger memory_total;
@JsonProperty private BigInteger memory_processes;
@JsonProperty private BigInteger memory_processes_used;
@JsonProperty private BigInteger memory_system;
@JsonProperty private BigInteger memory_atom;
@JsonProperty private BigInteger memory_atom_used;
@JsonProperty private BigInteger memory_binary;
@JsonProperty private BigInteger memory_code;
@JsonProperty private BigInteger memory_ets;
@JsonProperty private BigInteger ignored_gossip_total; // Riak 1.1
@JsonProperty private BigInteger rings_reconciled_total; // Riak 1.1
@JsonProperty private BigInteger rings_reconciled; // Riak 1.1
@JsonProperty private BigInteger gossip_received; // Riak 1.1
@JsonProperty private BigInteger converge_delay_min; // Riak 1.1
@JsonProperty private BigInteger converge_delay_max; // Riak 1.1
@JsonProperty private BigInteger converge_delay_mean; // Riak 1.1
@JsonProperty private BigInteger converge_delay_last; // Riak 1.1
@JsonProperty private BigInteger rebalance_delay_min; // Riak 1.1
@JsonProperty private BigInteger rebalance_delay_max; // Riak 1.1
@JsonProperty private BigInteger rebalance_delay_mean; // Riak 1.1
@JsonProperty private BigInteger rebalance_delay_last; // Riak 1.1
@JsonProperty private BigInteger riak_kv_vnodes_running; // Riak 1.1
@JsonProperty private BigInteger riak_kv_vnodeq_min; // Riak 1.1
@JsonProperty private BigInteger riak_kv_vnodeq_median; // Riak 1.1
@JsonProperty private BigInteger riak_kv_vnodeq_mean; // Riak 1.1
@JsonProperty private BigInteger riak_kv_vnodeq_max; // Riak 1.1
@JsonProperty private BigInteger riak_kv_vnodeq_total; // Riak 1.1
@JsonProperty private BigInteger riak_pipe_vnodes_running; // Riak 1.1
@JsonProperty private BigInteger riak_pipe_vnodeq_min; // Riak 1.1
@JsonProperty private BigInteger riak_pipe_vnodeq_median; // Riak 1.1
@JsonProperty private BigInteger riak_pipe_vnodeq_mean; // Riak 1.1
@JsonProperty private BigInteger riak_pipe_vnodeq_max; // Riak 1.1
@JsonProperty private BigInteger riak_pipe_vnodeq_total; // Riak 1.1
/**
* Returns the vnode_gets
value from the Riak stats reply
* @return int value
*/
public BigInteger vnodeGets()
{
return vnode_gets;
}
/**
* Returns the vnode_gets
value from the Riak stats reply
* @return int
value
*/
public BigInteger vnodePuts()
{
return vnode_puts;
}
/**
* Returns the vnode_index_reads
value from the Riak stats reply
* @return int
value
*/
public BigInteger vnodeIndexReads()
{
return vnode_index_reads;
}
/**
* Returns the vnode_index_writes
value from the Riak stats reply
* @return int
value
*/
public BigInteger vnodeIndexWrites()
{
return vnode_index_writes;
}
/**
* Returns the vnode_index_writes_postings
value from the Riak stats reply
* @return int
value
*/
public BigInteger vnodeIndexWritePostings()
{
return vnode_index_writes_postings;
}
/**
* Returns the vnode_index_deletes
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodeIndexDeletes()
{
return vnode_index_deletes;
}
/**
* Returns the vnode_index_deletes_postings
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodesIndexDeletesPostings()
{
return vnode_index_deletes_postings;
}
/**
* Returns the read_repairs
value from the Riak Stats reply
* @return int
value
*/
public BigInteger readRepairs()
{
return read_repairs;
}
/**
* Returns the vnode_gets_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodeGetsTotal()
{
return vnode_gets_total;
}
/**
* Returns the vnode_puts_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodePutsTotal()
{
return vnode_puts_total;
}
/**
* Returns the vnode_index_reads_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodeIndexReadsTotal()
{
return vnode_index_reads_total;
}
/**
* Returns the vnode_index_writes_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodeIndexWritesTotal()
{
return vnode_index_writes_total;
}
/**
* Returns the vnode_index_writes_postings_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodeIndexWritesPostingsTotal()
{
return vnode_index_writes_postings_total;
}
/**
* Returns the vnode_index_deletes_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodeIndexDeletesTotal()
{
return vnode_index_deletes_total;
}
/**
* Returns the vnode_index_deletes_postings_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger vnodeIndexDeletesPostingsTotal()
{
return vnode_index_deletes_postings_total;
}
/**
* Returns the node_gets
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGets()
{
return node_gets;
}
/**
* Returns the node_gets_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetsTotal()
{
return node_gets_total;
}
/**
* Returns the node_get_fsm_time_mean
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmTimeMean()
{
return node_get_fsm_time_mean;
}
/**
* Returns the node_get_fsm_time_median
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmTimeMedian()
{
return node_get_fsm_time_median;
}
/**
* Returns the node_get_fsm_time_95
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmTime95()
{
return node_get_fsm_time_95;
}
/**
* Returns the node_get_fsm_time_99
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmTime99()
{
return node_get_fsm_time_99;
}
/**
* Returns the node_get_fsm_time_100
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmTime100()
{
return node_get_fsm_time_100;
}
/**
* Returns the node_puts
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodePuts()
{
return node_puts;
}
/**
* Returns the node_puts_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodePutsTotal()
{
return node_puts_total;
}
/**
* Returns the node_get_fsm_time_mean
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodePutFsmTimeMean()
{
return node_put_fsm_time_mean;
}
/**
* Returns the node_put_fsm_time_median
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodePutFsmTimeMedian()
{
return node_put_fsm_time_median;
}
/**
* Returns the node_put_fsm_time_95
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodePutFsmTime95()
{
return node_put_fsm_time_95;
}
/**
* Returns the node_put_fsm_time_99
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodePutFsmTime99()
{
return node_put_fsm_time_99;
}
/**
* Returns the node_put_fsm_time_100
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodePutFsmTime100()
{
return node_put_fsm_time_100;
}
/**
* Returns the node_get_fsm_siblings_mean
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmSiblingsMean()
{
return node_get_fsm_siblings_mean;
}
/**
* Returns the node_put_fsm_siblings_median
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmSiblingsMedian()
{
return node_get_fsm_siblings_median;
}
/**
* Returns the node_put_fsm_siblings_95
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmSiblings95()
{
return node_get_fsm_siblings_95;
}
/**
* Returns the node_put_fsm_siblings_99
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmSiblings99()
{
return node_get_fsm_siblings_99;
}
/**
* Returns the node_put_fsm_siblings_100
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmSiblings100()
{
return node_get_fsm_siblings_100;
}
/**
* Returns the node_get_fsm_objsize_mean
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmObjsizeMean()
{
return node_get_fsm_objsize_mean;
}
/**
* Returns the node_put_fsm_objsize_median
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmObjsizeMedian()
{
return node_get_fsm_objsize_median;
}
/**
* Returns the node_put_fsm_objsize_95
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmObjsize95()
{
return node_get_fsm_objsize_95;
}
/**
* Returns the node_put_fsm_objsize_99
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodeGetFsmObjsize99()
{
return node_get_fsm_objsize_99;
}
/**
* Returns the node_put_fsm_objsize_100
value from the Riak Stats reply
* @return int
value
*/
public BigInteger nodePutFsmSObjsize100()
{
return node_get_fsm_objsize_100;
}
/**
* Returns the read_repairs_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger readRepairsTotal()
{
return read_repairs_total;
}
/**
* Returns the coord_redirs_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger coordRedirsTotal()
{
return coord_redirs_total;
}
/**
* Returns the cpu_nprocs
value from the Riak Stats reply
* @return int
value
*/
public BigInteger cpuNumProcs()
{
return cpu_nprocs;
}
/**
* Returns the cpu_avg1
value from the Riak Stats reply
* @return int
value
*/
public BigInteger cpuAvg1()
{
return cpu_avg1;
}
/**
* Returns the cpu_avg5
value from the Riak Stats reply
* @return int
value
*/
public BigInteger cpuAvg5()
{
return cpu_avg5;
}
/**
* Returns the cpu_avg15
value from the Riak Stats reply
* @return int
value
*/
public BigInteger cpuAvg15()
{
return cpu_avg15;
}
/**
* Returns the mem_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memTotal()
{
return mem_total;
}
/**
* Returns the mem_allocated
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memAllocated()
{
return mem_allocated;
}
/**
* Returns the nodename
value from the Riak stats reply
* @return String
value
*/
public String nodename()
{
return nodename;
}
/**
* Returns the connected_nodes
value from the Riak Stats reply
* @return String[]
of node names
*/
public String[] connectedNodes()
{
return connected_nodes;
}
/**
* Returns the sys_driver_version
value from the Riak stats reply
* @return String
value
*/
public String sysDriverVersion()
{
return sys_driver_version;
}
/**
* Returns the sys_global_heaps_size
value from the Riak Stats reply
* @return int
value
*/
public BigInteger sysGlobalHeapsSize()
{
return sys_global_heaps_size;
}
/**
* Returns the sys_heap_type
value from the Riak stats reply
* @return String
value
*/
public String sysHeapType()
{
return sys_heap_type;
}
/**
* Returns the sys_logical_processors
value from the Riak Stats reply
* @return int
value
*/
public BigInteger sysLogicalProcessors()
{
return sys_logical_processors;
}
/**
* Returns the sys_otp_release
value from the Riak stats reply
* @return String
value
*/
public String sysOtpRelease()
{
return sys_otp_release;
}
/**
* Returns the sys_process_count
value from the Riak Stats reply
* @return int
value
*/
public BigInteger sysProcessCount()
{
return sys_process_count;
}
/**
* Returns the sys_smp_support
value from the Riak Stats reply
* @return boolean
value
*/
public boolean sysSmpSupport()
{
return sys_smp_support;
}
/**
* Returns the sys_system_version
value from the Riak stats reply
* @return String
value
*/
public String sysSystemVersion()
{
return sys_system_version;
}
/**
* Returns the sys_system_architecture
value from the Riak stats reply
* @return String
value
*/
public String sysSystemArchitecture()
{
return sys_system_architecture;
}
/**
* Returns the sys_threads_enabled
value from the Riak Stats reply
* @return boolean
value
*/
public boolean sysThreadsEnabled()
{
return sys_threads_enabled;
}
/**
* Returns the sys_thread_pool_size
value from the Riak Stats reply
* @return int
value
*/
public BigInteger sysThreadPoolSize()
{
return sys_thread_pool_size;
}
/**
* Returns the sys_wordsize
value from the Riak Stats reply
* @return int
value
*/
public BigInteger sysWordSize()
{
return sys_wordsize;
}
/**
* Returns the ring_members
value from the Riak Stats reply
* @return String[]
of node names
*/
public String[] ringMembers()
{
return ring_members;
}
/**
* Returns the ring_num_partitions
value from the Riak Stats reply
* @return int
value
*/
public BigInteger ringNumPartitions()
{
return ring_num_partitions;
}
/**
* Returns the ring_ownership
value from the Riak stats reply
* @return String
value
*/
public String ringOwnership()
{
return ring_ownership;
}
/**
* Returns the ring_creation_size
value from the Riak Stats reply
* @return int
value
*/
public BigInteger ringCreationSize()
{
return ring_creation_size;
}
/**
* Returns the storage_backend
value from the Riak stats reply
* @return String
value
*/
public String storageBackend()
{
return storage_backend;
}
/**
* Returns the pbc_connects_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger pbcConnectsTotal()
{
return pbc_connects_total;
}
/**
* Returns the pbc_connects
value from the Riak Stats reply
* @return int
value
*/
public BigInteger pbcConnects()
{
return pbc_connects;
}
/**
* Returns the pbc_active
value from the Riak Stats reply
* @return int
value
*/
public BigInteger pbcActive()
{
return pbc_active;
}
/**
* Returns the ssl_version
value from the Riak stats reply
* @return String
value
*/
public String sslVeriosn()
{
return ssl_version;
}
/**
* Returns the public_key_version
value from the Riak stats reply
* @return String
value
*/
public String publicKeyVersion()
{
return public_key_version;
}
/**
* Returns the runtime_tools_version
value from the Riak stats reply
* @return String
value
*/
public String runtimeToolsVersion()
{
return runtime_tools_version;
}
/**
* Returns the basho_stats_version
value from the Riak stats reply
* @return String
value
*/
public String bashoStatsVersion()
{
return basho_stats_version;
}
/**
* Returns the riak_search_version
value from the Riak stats reply
* @return String
value
*/
public String riakSearchVersion()
{
return riak_search_version;
}
/**
* Returns the merge_index_version
value from the Riak stats reply
* @return String
value
*/
public String mergeIndexVersion()
{
return merge_index_version;
}
/**
* Returns the luwak_version
value from the Riak stats reply
* @return String
value
*/
public String luwakVersion()
{
return luwak_version;
}
/**
* Returns the skerl_version
value from the Riak stats reply
* @return String
value
*/
public String skerlVersion()
{
return skerl_version;
}
/**
* Returns the riak_kv_version
value from the Riak stats reply
* @return String
value
*/
public String riakKvVersion()
{
return riak_kv_version;
}
/**
* Returns the bitcask_version
value from the Riak stats reply
* @return String
value
*/
public String bitcaskVersion()
{
return bitcask_version;
}
/**
* Returns the luke_version
value from the Riak stats reply
* @return String
value
*/
public String lukeVeriosn()
{
return luke_version;
}
/**
* Returns the erlang_js_version
value from the Riak stats reply
* @return String
value
*/
public String erlangJsVersion()
{
return erlang_js_version;
}
/**
* Returns the mochiweb_value
value from the Riak stats reply
* @return String
value
*/
public String mochiwebVersion()
{
return mochiweb_version;
}
/**
* Returns the inets_version
value from the Riak stats reply
* @return String
value
*/
public String inetsVersion()
{
return inets_version;
}
/**
* Returns the riak_pipe_version
value from the Riak stats reply
* @return String
value
*/
public String riakPipeVersion()
{
return riak_pipe_version;
}
/**
* Returns the riak_core_version
value from the Riak stats reply
* @return String
value
*/
public String riakCoreVersion()
{
return riak_core_version;
}
/**
* Returns the riak_sysmon_version
value from the Riak stats reply
* @return String
value
*/
public String riak_sysmon_version()
{
return riak_sysmon_version;
}
/**
* Returns the webmachine_version
value from the Riak stats reply
* @return String
value
*/
public String webmachineVersion()
{
return webmachine_version;
}
/**
* Returns the crypto_version
value from the Riak stats reply
* @return String
value
*/
public String cryptoVersion()
{
return crypto_version;
}
/**
* Returns the os_mon_version
value from the Riak stats reply
* @return String
value
*/
public String osMonVersion()
{
return os_mon_version;
}
/**
* Returns the cluster_info_version
value from the Riak stats reply
* @return String
value
*/
public String clusterInfoVersion()
{
return cluster_info_version;
}
/**
* Returns the sasl_version
value from the Riak stats reply
* @return String
value
*/
public String saslVersion()
{
return sasl_version;
}
/**
* Returns the lager_version
value from the Riak stats reply
* @return String
value
*/
public String lagerVersion()
{
return lager_version;
}
/**
* Returns the basho_lager_version
value from the Riak stats reply
* @return String
value
*/
public String bashoMetricsVersion()
{
return basho_metrics_version;
}
/**
* Returns the stdlib_version
value from the Riak stats reply
* @return String
value
*/
public String stdlibVersion()
{
return stdlib_version;
}
/**
* Returns the kernel_version
value from the Riak stats reply
* @return String
value
*/
public String kernelVersion()
{
return kernel_version;
}
/**
* Returns the executing_mappers
value from the Riak Stats reply
* @return int
value
*/
public BigInteger executingMappers()
{
return executing_mappers;
}
/**
* Returns the memory_total
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memoryTotal()
{
return memory_total;
}
/**
* Returns the memory_processes
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memoryProcesses()
{
return memory_processes;
}
/**
* Returns the memory_processes_used
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memoryProcessesUsed()
{
return memory_processes_used;
}
/**
* Returns the memory_system
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memorySystem()
{
return memory_system;
}
/**
* Returns the memory_atom
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memoryAtom()
{
return memory_atom;
}
/**
* Returns the memory_atom_used
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memoryAtomUsed()
{
return memory_atom_used;
}
/**
* Returns the memory_binary
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memoryBinary()
{
return memory_binary;
}
/**
* Returns the memory_code
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memoryCode()
{
return memory_code;
}
/**
* Returns the memory_ets
value from the Riak Stats reply
* @return int
value
*/
public BigInteger memoryEts()
{
return memory_ets;
}
/**
* @return the precommit_fail
*/
public BigInteger precommitFail()
{
return precommit_fail;
}
/**
* @return the postcommit_fail
*/
public BigInteger postcommitFail()
{
return postcommit_fail;
}
/**
* @return the ignored_gossip_total
*/
public BigInteger ignoredGossipTotal()
{
return ignored_gossip_total;
}
/**
* @return the rings_reconciled_total
*/
public BigInteger ringsReconciledTotal()
{
return rings_reconciled_total;
}
/**
* @return the rings_reconciled
*/
public BigInteger ringsReconciled()
{
return rings_reconciled;
}
/**
* @return the gossip_received
*/
public BigInteger gossipReceived()
{
return gossip_received;
}
/**
* @return the converge_delay_min
*/
public BigInteger convergeDelayMin()
{
return converge_delay_min;
}
/**
* @return the converge_delay_max
*/
public BigInteger convergeDelayMax()
{
return converge_delay_max;
}
/**
* @return the converge_delay_mean
*/
public BigInteger convergeDelayMean()
{
return converge_delay_mean;
}
/**
* @return the converge_delay_last
*/
public BigInteger convergeDelayLast()
{
return converge_delay_last;
}
/**
* @return the rebalance_delay_min
*/
public BigInteger rebalanceDelayMin()
{
return rebalance_delay_min;
}
/**
* @return the rebalance_delay_max
*/
public BigInteger rebalanceDelayMax()
{
return rebalance_delay_max;
}
/**
* @return the rebalance_delay_mean
*/
public BigInteger rebalanceDelayMean()
{
return rebalance_delay_mean;
}
/**
* @return the rebalanceDelay_last
*/
public BigInteger rebalanceDelayLast()
{
return rebalance_delay_last;
}
/**
* @return the riak_kv_vnodes_running
*/
public BigInteger riakKvVnodesRunning()
{
return riak_kv_vnodes_running;
}
/**
* @return the riak_kv_vnodeq_min
*/
public BigInteger riakKvVnodeqMin()
{
return riak_kv_vnodeq_min;
}
/**
* @return the riak_kv_vnodeq_median
*/
public BigInteger riakKvVnodeqMedian()
{
return riak_kv_vnodeq_median;
}
/**
* @return the riak_kv_vnodeq_mean
*/
public BigInteger riakKvVnodeqMean()
{
return riak_kv_vnodeq_mean;
}
/**
* @return the riak_kv_vnodeq_max
*/
public BigInteger riakKvVnodeqMax()
{
return riak_kv_vnodeq_max;
}
/**
* @return the riak_kv_vnodeq_total
*/
public BigInteger riakKvVnodeqTotal()
{
return riak_kv_vnodeq_total;
}
/**
* @return the riak_pipe_vnodes_running
*/
public BigInteger riakPipeVnodesRunning()
{
return riak_pipe_vnodes_running;
}
/**
* @return the riak_pipe_vnodeq_min
*/
public BigInteger riakPipeVnodeqMin()
{
return riak_pipe_vnodeq_min;
}
/**
* @return the riak_pipe_vnodeq_median
*/
public BigInteger riakPipeVnodeqMedian()
{
return riak_pipe_vnodeq_median;
}
/**
* @return the riak_pipe_vnodeq_mean
*/
public BigInteger riakPipeVnodeqMean()
{
return riak_pipe_vnodeq_mean;
}
/**
* @return the riak_pipe_vnodeq_max
*/
public BigInteger riakPipeVnodeqMax()
{
return riak_pipe_vnodeq_max;
}
/**
* @return the riak_pipe_vnodeq_total
*/
public BigInteger riakPipeVnodeqTotal()
{
return riak_pipe_vnodeq_total;
}
NodeStats getPrevios()
{
return previous;
}
NodeStats getNext()
{
return next;
}
void setNext(NodeStats anotherStats)
{
this.next = anotherStats;
}
void setPrevious(NodeStats anotherStats)
{
this.previous = anotherStats;
}
/**
* Adds a set of stats to the end of the collection. Using the iterator
* you can retrieve each set in order
* @param anotherStats an additional NodeStats
*/
public void add(NodeStats anotherStats)
{
if (next == null)
{
next = anotherStats;
anotherStats.setPrevious(this);
}
else
{
NodeStats tmp = next;
while (tmp.getNext() != null)
{
tmp = tmp.getNext();
}
tmp.setNext(anotherStats);
anotherStats.setPrevious(tmp);
}
}
public Iterator iterator()
{
NodeStats first;
if (previous == null)
{
first = this;
}
else
{
first = previous;
while (first.getPrevios() != null)
{
first = first.getPrevios();
}
}
return new NodeStatsIterator(first);
}
private class NodeStatsIterator implements Iterator
{
private NodeStats current;
public NodeStatsIterator(NodeStats first)
{
super();
current = first;
}
public boolean hasNext()
{
return current != null;
}
public NodeStats next()
{
if (!hasNext())
throw new NoSuchElementException();
NodeStats stats = current;
current = current.getNext();
return stats;
}
public void remove()
{
throw new UnsupportedOperationException();
}
}
/* There's a few stats that are currently not being properly serialized
* to JSON by Riak that have a string value ("undefined") instead of 0 (or
* any integer value). This fixes those.
*/
public static class UndefinedStatDeserializer extends JsonDeserializer
{
@Override
public BigInteger deserialize(JsonParser jp, DeserializationContext dc) throws IOException, JsonProcessingException
{
if (jp.getCurrentToken() == JsonToken.VALUE_STRING)
{
return BigInteger.valueOf(0L);
}
else
return BigInteger.valueOf(jp.getLongValue());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy