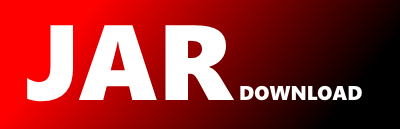
com.basho.riak.client.raw.RawClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
/*
* This file is provided to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.basho.riak.client.raw;
import com.basho.riak.client.IndexEntry;
import com.basho.riak.client.query.StreamingOperation;
import java.io.IOException;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import com.basho.riak.client.IRiakObject;
import com.basho.riak.client.bucket.BucketProperties;
import com.basho.riak.client.query.MapReduceResult;
import com.basho.riak.client.query.NodeStats;
import com.basho.riak.client.query.WalkResult;
import com.basho.riak.client.raw.query.IndexSpec;
import com.basho.riak.client.raw.query.LinkWalkSpec;
import com.basho.riak.client.raw.query.MapReduceSpec;
import com.basho.riak.client.raw.query.MapReduceTimeoutException;
import com.basho.riak.client.raw.query.indexes.IndexQuery;
/**
* Common interface for low-level clients.
*
*
* If you have the urge to write a client to Riak, please, please implement this
* interface so it can drop straight into all the higher level API code
*
*
* @author russell
*
*/
public interface RawClient {
/**
* Fetch only the meta-data from bucket/key
*
* @param bucket
* the bucket
* @param key
* the key
* @param fetchMeta
* the fetch options
* @return a {@link RiakResponse} that, if it has an {@link IRiakObject} it
* will have an empty value
* @throws IOException
*/
RiakResponse head(String bucket, String key, FetchMeta fetchMeta) throws IOException;
/**
* Fetch data from bucket/key
*
* @param bucket
* the bucket
* @param key
* the key
* @return a {@link RiakResponse}
* @throws IOException
*/
RiakResponse fetch(String bucket, String key) throws IOException;
/**
* Fetch data from the given bukcet/key
with read quorum
* readQuorum
*
* @param bucket
* the bucket
* @param key
* the key
* @param readQuorum
* readQuorum, needs to be =< the buckets n_val
* @return a {@link RiakResponse}
* @throws IOException
*/
RiakResponse fetch(String bucket, String key, int readQuorum) throws IOException;
/**
* Fetch data from the given bucket/key
with
* fetchMeta
*
* @param bucket
* the bucket
* @param key
* the key
* @param fetchMeta
* the extra fetch parameters {@link FetchMeta}
* @return a {@link RiakResponse}
* @throws IOException
*/
RiakResponse fetch(String bucket, String key, FetchMeta fetchMeta) throws IOException;
/**
* Store the given {@link IRiakObject} in Riak at the location
* bucket/key
*
* @param object
* the data to store
* @param storeMeta
* meta data for the store operation as a {@link StoreMeta}
* @return a {@link RiakResponse} if {@link StoreMeta#getReturnBody()} is
* true, or null
* @throws IOException
*/
RiakResponse store(IRiakObject object, StoreMeta storeMeta) throws IOException;
/**
* Store the given {@link IRiakObject} in Riak using the bucket default w/dw
* and false for returnBody
*
* @param object
* the data to store as an {@link IRiakObject}
* @throws IOException
*/
void store(IRiakObject object) throws IOException;
/**
* Delete the data at bucket/key
*
* @param bucket
* @param key
* @throws IOException
*/
void delete(String bucket, String key) throws IOException;
/**
* Delete the data at bucket/key
using
* deleteQuorum
as the rw param
*
* @param bucket
* @param key
* @param deleteQuorum
* an int that is less than or equal to the bucket's n_val
* @throws IOException
*/
void delete(String bucket, String key, int deleteQuorum) throws IOException;
/**
* Delete the data at bucket/key
using the parameters in
* deleteMeta
*
* @param bucket
* @param key
* @param deleteMeta
* the {@link DeleteMeta} containing the operation parameters
* @throws IOException
*/
void delete(String bucket, String key, DeleteMeta deleteMeta) throws IOException;
// Bucket
/**
* An Unmodifiable {@link Iterator} view of the all the Buckets in Riak
*/
Set listBuckets() throws IOException;
/**
* Iterate over the bucket names in Riak. This is a streaming operation.
*
* This is only available in Riak 1.4 or later.
*
* * You *must* call {@link StreamingOperation#cancel() } on the returned
* {@link StreamingOperation} if you do not iterate through the entire set.
*
* As a safeguard the stream is closed automatically when the iterator is
* weakly reachable but due to the nature of the GC it is inadvisable to
* rely on this to close the iterator. Do not retain a reference to this
* after you have used it.
*
* @return An iterable that is backed by a TCP connection
* @throws IOException
* */
StreamingOperation listBucketsStreaming() throws IOException;
/**
* The set of properties for the given bucket
*
* @param bucketName
* the name of the bucket
* @return a populated {@link BucketProperties} (by populated, as populated
* as the underlying API allows)
* @throws IOException
*/
BucketProperties fetchBucket(String bucketName) throws IOException;
/**
* Update a buckets properties from the {@link BucketProperties} provided.
* No guarantees that the underlying API is able to set all the properties
* passed.
*
* @param name
* the bucket to be updated
* @param bucketProperties
* the set of properties to be writen
* @throws IOException
*/
void updateBucket(String name, BucketProperties bucketProperties) throws IOException;
/**
* Reset the bucket properties for this bucket back to the default values
* @param bucketName
*/
void resetBucketProperties(String bucketName) throws IOException;
/**
* An unmodifiable view of the keys for the bucket named
* bucketName
*
* * You *must* call {@link StreamingOperation#cancel() } on the returned
* {@link StreamingOperation} if you do not iterate through the entire set.
*
* As a safeguard the stream is closed automatically when the iterator is
* weakly reachable but due to the nature of the GC it is inadvisable to
* rely on this to close the iterator. Do not retain a reference to this
* after you have used it.
*
* Be careful, expensive.
*
* @param bucketName
* @return an unmodifiable, iterable view of the keys for that bucket backed by a TCP connection
* @throws IOException
*/
StreamingOperation listKeys(String bucketName) throws IOException;
// Query
/**
* Performs a link walk operation described by the {@link LinkWalkSpec}
* provided.
*
* The underlying API may not support Link Walking directly but will
* approximate it at some cost.
*
* @param linkWalkSpec
* @return a {@link WalkResult}
* @throws IOException
*/
WalkResult linkWalk(final LinkWalkSpec linkWalkSpec) throws IOException;
/**
* Perform a map/reduce query defined by {@link MapReduceSpec}
* @param spec the m/r job specification
* @return A {@link MapReduceResult}
* @throws IOException
* @throws MapReduceTimeoutException
*/
MapReduceResult mapReduce(final MapReduceSpec spec) throws IOException, MapReduceTimeoutException;
/**
* If you don't set a client id explicitly at least call this to set one. It
* generates the 4 byte ID and sets that Id on the client IE you *don't*
* need to call setClientId() with the result of generate.
*
* @return the generated clientId for the client
*/
byte[] generateAndSetClientId() throws IOException;
/**
* Set a client id, currently must be a 4 bytes exactly
* @param clientId any 4 bytes
* @throws IOException
*/
void setClientId(byte[] clientId) throws IOException;
/**
* Ask Riak for the client id for the current connection.
* @return whatever 4 bytes Riak uses to identify this client
* @throws IOException
*/
byte[] getClientId() throws IOException;
/**
* Riak connection health check, is Riak reachable. Can be used for
* diagnostics/monitoring
*
* @throws IOException
* if Riak is not reachable or returns anything other than OK
*/
void ping() throws IOException;
/**
* Performs an 2i index query
*
* @param
* @param indexQuery
* the query to perform
* @return a List of keys (or empty list)
* @throws IOException
*/
List fetchIndex(IndexQuery indexQuery) throws IOException;
/**
* Performs a 2i query as a streaming operation
*/
StreamingOperation fetchIndex(IndexSpec indexSpec) throws IOException;
/**
* Increments a counter by the specified increment
*
* @param bucket the name of the bucket
* @param counter the name (key) of the counter
* @param increment the amount to increment. Note this can be a negative value.
* @param meta the query parameters
* @return null or the new value for the counter if returnBody is set to true in the StoreMeta
*/
Long incrementCounter(String bucket, String counter, long increment, StoreMeta meta) throws IOException;
/**
* Fetch the value for this counter
*
* @param bucket the name of the bucket
* @param counter the name (key) of the counter
* @param meta query parameters
* @return the value of the counter or null if it does not exist
*/
Long fetchCounter(String bucket, String counter, FetchMeta meta) throws IOException;
/**
* The raw transport name.
* @return the {@link Transport} for the client or null if not implemented.
*/
Transport getTransport();
void shutdown();
/**
* Performs a Riak /stats
operation on the node(s) this client
* is connected to. The {@link NodeStats} object that is returned contains one
* or more sets of stats and can be Iterated over.
*
* @return a {@link NodeStats} object that represents one or more sets of stats.
*/
NodeStats stats() throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy