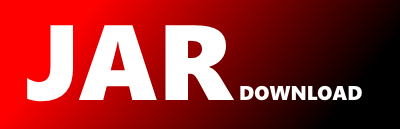
com.basho.riak.pbc.RiakStreamClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
/**
* This file is part of riak-java-pb-client
*
* Copyright (c) 2010 by Trifork
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
**/
package com.basho.riak.pbc;
import com.google.protobuf.ByteString;
import java.io.IOException;
import java.lang.ref.WeakReference;
import java.util.Iterator;
import java.util.Timer;
import java.util.TimerTask;
/**
* A general purpose stream -> iterator adaptor.
* @param
*/
public abstract class RiakStreamClient implements Iterable {
static Timer TIMER = new Timer("riak-stream-timeout-thread", true);
private RiakClient client;
protected RiakConnection conn;
protected ByteString continuation;
private ReaperTask reaper;
protected RiakStreamClient(RiakClient client, RiakConnection conn) {
this.client = client;
this.conn = conn;
this.reaper = new ReaperTask(this, conn);
}
public boolean hasContinuation()
{
return continuation != null;
}
public ByteString getContinuation()
{
return continuation;
}
static class ReaperTask extends TimerTask {
private final RiakConnection conn;
private WeakReference> ref;
ReaperTask (Object holder, RiakConnection conn) {
this.conn = conn;
this.ref = new WeakReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy