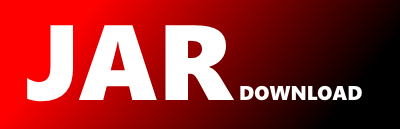
com.basho.riak.client.api.commands.Search Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
/*
* Copyright 2013 Basho Technologies Inc
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basho.riak.client.api.commands;
import com.basho.riak.client.api.RiakCommand;
import com.basho.riak.client.core.RiakCluster;
import com.basho.riak.client.core.RiakFuture;
import com.basho.riak.client.core.operations.SearchOperation;
import com.basho.riak.client.core.util.BinaryValue;
import java.util.*;
/*
* @author Dave Rusek
* @since 2.0
*/
public final class Search extends RiakCommand
{
public static enum Presort
{
KEY("key"), SCORE("score");
final String sortStr;
Presort(String sortStr)
{
this.sortStr = sortStr;
}
}
private final String index;
private final String query;
private final int start;
private final int rows;
private final Presort presort;
private final String filterQuery;
private final String sortField;
private final List returnFields;
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy