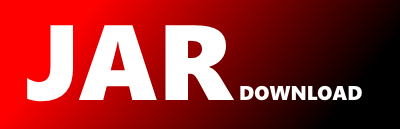
com.basho.riak.client.api.commands.kv.MultiFetch Maven / Gradle / Ivy
Show all versions of riak-client Show documentation
/*
* Copyright 2013 Basho Technologies Inc
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basho.riak.client.api.commands.kv;
import com.basho.riak.client.core.RiakCluster;
import com.basho.riak.client.api.RiakCommand;
import com.basho.riak.client.core.RiakFuture;
import com.basho.riak.client.core.RiakFutureListener;
import com.basho.riak.client.api.commands.ListenableFuture;
import com.basho.riak.client.api.commands.kv.FetchValue.Option;
import com.basho.riak.client.core.query.Location;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import static java.util.Collections.unmodifiableList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.Semaphore;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicInteger;
/**
* Command used to fetch multiple values from Riak.
*
*
* Riak itself does not support pipelining of requests. MutliFetch addresses this issue by using a thread to
* parallelize and manage a set of async fetch operations for a given set of keys.
*
*
* The result of executing this command is a {@code List} of {@link RiakFuture} objects, each one representing a single
* fetch operation. The returned {@code RiakFuture} that contains that list completes
* when all the FetchValue operations contained have finished.
*
*
* {@code
* MultiFetch multifetch = ...;
* MultiFetch.Response response = client.execute(multifetch);
* List myResults = new ArrayList();
* for (RiakFuture f : response)
* {
* try
* {
* FetchValue.Response response = f.get();
* myResults.add(response.getValue(MyPojo.class));
* }
* catch (ExecutionException e)
* {
* // log error, etc.
* }
* }}
*
*
* The maximum number of concurrent requests defaults to 10. This can be changed
* when constructing the operation.
*
*
* Be aware that because requests are being parallelized performance is also
* dependent on the client's underlying connection pool. If there are no connections
* available performance will suffer initially as connections will need to be established
* or worse they could time out.
*
*
* @author Dave Rusek
* @since 2.0
*/
public final class MultiFetch extends RiakCommand>
{
public static final int DEFAULT_MAX_IN_FLIGHT = 10;
private final ArrayList locations = new ArrayList();
private final Map