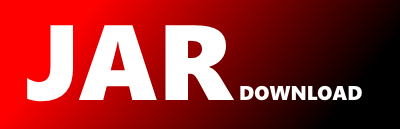
com.basho.riak.client.api.commands.search.Search Maven / Gradle / Ivy
/*
* Copyright 2013 Basho Technologies Inc
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basho.riak.client.api.commands.search;
import com.basho.riak.client.api.RiakCommand;
import com.basho.riak.client.api.commands.CoreFutureAdapter;
import com.basho.riak.client.api.commands.RiakOption;
import com.basho.riak.client.core.RiakCluster;
import com.basho.riak.client.core.RiakFuture;
import com.basho.riak.client.core.operations.SearchOperation;
import com.basho.riak.client.core.util.BinaryValue;
import java.util.*;
/**
* Command used to perform a search in Riak.
*
*
* To do a search in Riak, you must have search turned on, indexes created, and documents/objects indexed.
* Please see http://docs.basho.com/riak/latest/dev/using/search/ for more information.
*
* To use the Search class, you need a minimum of an Index to search, and a query to execute.
* Other options are available for pagination, sorting, filtering and what fields to return.
*
*
* {@code
* String index = "Author_Biographies";
* String query = "name_s:Al* AND bio_tsd:awesome";
*
* Search sch =
* new Search.Builder(index, query).withRows(10).build();
* SearchOperation.Response response = client.execute(sch);}
*
*
* All operations can called async as well.
*
* {@code
* ...
* RiakFuture future = client.executeAsync(sv);
* ...
* future.await();
* if (future.isSuccess())
* {
* ...
* }}
*
*
* @author Dave Rusek
* @since 2.0
*/
public final class Search extends RiakCommand
{
/**
* Enum that encapsulates the possible settings for a search command's presort setting.
* Presort results by the key, or search score.
*/
public enum Presort
{
KEY("key"), SCORE("score");
final String sortStr;
Presort(String sortStr)
{
this.sortStr = sortStr;
}
}
private final String index;
private final String query;
private final int start;
private final int rows;
private final Presort presort;
private final String filterQuery;
private final String sortField;
private final List returnFields;
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy