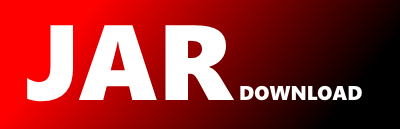
com.basho.riak.client.api.cap.ConflictResolverFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
The newest version!
/*
* Copyright 2014 Basho Technologies Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basho.riak.client.api.cap;
import com.fasterxml.jackson.core.type.TypeReference;
import java.lang.reflect.Type;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Simple factory for ConflictResolver objects.
*
* When you have multiple writers there may be multiple versions of the same
* object stored in Riak. When fetching all of these will be returned and you
* will need to resolve the conflict.
*
*
* To facilitate this, you can store an instance of the {@link ConflictResolver}
* in this factory for a class. It will then be used by the {@link com.basho.riak.client.api.commands.kv.FetchValue.Response}
* to resolve a set a of siblings to a single object.
*
*
* @author Brian Roach
* @since 2.0
*/
public enum ConflictResolverFactory
{
INSTANCE;
private final Map> resolverInstances = new ConcurrentHashMap<>();
/**
* Returns the instance of the ConflictResolverFactory.
* @return The ConflictResolverFactory
*/
public static ConflictResolverFactory getInstance()
{
return INSTANCE;
}
public ConflictResolver getConflictResolver(Class clazz)
{
if (clazz == null)
{
throw new IllegalArgumentException("clazz cannot be null");
}
return getConflictResolver(clazz, null);
}
public ConflictResolver getConflictResolver(TypeReference typeReference)
{
if (typeReference == null)
{
throw new IllegalArgumentException("typeReference cannot be null");
}
return getConflictResolver(null, typeReference);
}
/**
* Return the ConflictResolver for the given class.
*
* If no ConflictResolver is registered for the provided class, an instance of the
* {@link com.basho.riak.client.cap.DefaultResolver} is returned.
*
* @param The type being resolved
* @param clazz the class of the type being resolved
* @return The conflict resolver for the type.
* @throws UnresolvedConflictException
*/
@SuppressWarnings("unchecked")
private ConflictResolver getConflictResolver(Type type, TypeReference typeReference)
{
type = type != null ? type : typeReference.getType();
ConflictResolver resolver = (ConflictResolver) resolverInstances.get(type);
if (resolver == null)
{
// Cache this?
resolver = (ConflictResolver) new DefaultResolver();
}
return resolver;
}
/**
* Register a ConflictResolver.
*
* The instance provided will be used to resolve siblings for the given type.
*
*
* @param The type being resolved
* @param clazz the class of the type being resolved
* @param resolver an instance of a class implementing ConflictResolver.
*/
public void registerConflictResolver(Class clazz, ConflictResolver resolver)
{
resolverInstances.put(clazz, resolver);
}
public void registerConflictResolver(TypeReference typeReference, ConflictResolver resolver)
{
resolverInstances.put(typeReference.getType(), resolver);
}
/**
* Unregister a ConflictResolver.
* @param The type being Resolved
* @param clazz the class of the type being resolved.
*/
public void unregisterConflictResolver(Class clazz)
{
resolverInstances.remove(clazz);
}
public void unregisterConflictResolver(TypeReference typeReference)
{
resolverInstances.remove(typeReference.getType());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy