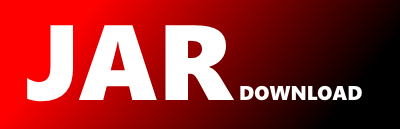
com.basho.riak.client.api.commands.indexes.IntIndexQuery Maven / Gradle / Ivy
Show all versions of riak-client Show documentation
/*
* Copyright 2014 Basho Technologies Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basho.riak.client.api.commands.indexes;
import com.basho.riak.client.core.StreamingRiakFuture;
import com.basho.riak.client.core.operations.SecondaryIndexQueryOperation;
import com.basho.riak.client.core.query.Namespace;
import com.basho.riak.client.core.util.BinaryValue;
/**
* Performs a 2i query where the 2i index keys are numeric.
*
*
* A IntIndexQuery is used when you are using integers for your 2i keys. The
* parameters are provided as long values.
*
*
* {@code
* Namespace ns = new Namespace("my_type", "my_bucket");
* long key = 1234L;
* IntIndexQuery q = new IntIndexQuery.Builder(ns, "my_index", key).build();
* IntIndexQuery.Response resp = client.execute(q);}
*
*
* You can also stream the results back before the operation is fully complete.
* This reduces the time between executing the operation and seeing a result,
* and reduces overall memory usage if the iterator is consumed quickly enough.
* The result iterable can only be iterated once though.
* If the thread is interrupted while the iterator is polling for more results,
* a {@link RuntimeException} will be thrown.
*
* {@code
* Namespace ns = new Namespace("my_type", "my_bucket");
* long key = 1234L;
* IntIndexQuery q = new IntIndexQuery.Builder(ns, "my_index", key).build();
* RiakFuture streamingFuture =
* client.executeAsyncStreaming(q, 200);
* IntIndexQuery.StreamingResponse streamingResponse = streamingFuture.get();
*
* for (IntIndexQuery.Response.Entry e : streamingResponse)
* {
* System.out.println(e.getRiakObjectLocation().getKey().toString());
* }
* // Wait for the command to fully finish.
* streamingFuture.await();
* // The StreamingResponse will also contain the continuation, if the operation returned one.
* streamingResponse.getContinuation(); }
*
*
* @author Brian Roach
* @author Alex Moore
* @author Sergey Galkin
* @since 2.0
*/
public class IntIndexQuery extends SecondaryIndexQuery
{
private final IndexConverter converter;
@Override
protected IndexConverter getConverter()
{
return converter;
}
protected IntIndexQuery(Init builder)
{
super(builder, Response::new, Response::new);
this.converter = new IndexConverter()
{
@Override
public Long convert(BinaryValue input)
{
// Riak. US_ASCII string instead of integer
return Long.valueOf(input.toStringUtf8());
}
@Override
public BinaryValue convert(Long input)
{
if (input == null)
{
return null;
}
// Riak. US_ASCII string instead of integer
return BinaryValue.createFromUtf8(String.valueOf(input));
}
};
}
protected static abstract class Init> extends SecondaryIndexQuery.Init
{
public Init(Namespace namespace, String indexName, S start, S end)
{
super(namespace, indexName + Type._INT, start, end);
}
public Init(Namespace namespace, String indexName, byte[] coverContext)
{
super(namespace, indexName + Type._INT, coverContext);
}
public Init(Namespace namespace, String indexName, S match)
{
super(namespace, indexName + Type._INT, match);
}
@Override
public T withRegexTermFilter(String filter)
{
throw new IllegalArgumentException("Cannot use term filter with _int query");
}
}
/**
* Builder used to construct a IntIndexQuery.
*/
public static class Builder extends Init
{
/**
* Construct a Builder for a IntIndexQuery with a cover context.
*
* Note that your index name should not include the Riak {@literal _int} or
* {@literal _bin} extension.
*
* @param namespace The namespace in Riak to query.
* @param indexName The name of the index in Riak.
* @param coverContext cover context.
*/
public Builder(Namespace namespace, String indexName, byte[] coverContext)
{
super(namespace, indexName, coverContext);
}
/**
* Construct a Builder for a IntIndexQuery with a range.
*
* Note that your index name should not include the Riak {@literal _int} or
* {@literal _bin} extension.
*
* @param namespace The namespace in Riak to query.
* @param indexName The name of the index in Riak.
* @param start the start of the 2i range.
* @param end the end of the 2i range.
*/
public Builder(Namespace namespace, String indexName, Long start, Long end)
{
super(namespace, indexName, start, end);
}
/**
* Construct a Builder for a IntIndexQuery with a single 2i key.
*
* Note that your index name should not include the Riak {@literal _int} or
* {@literal _bin} extension.
*
* @param namespace The namespace in Riak to query.
* @param indexName The name of the index in Riak.
* @param match the 2i key.
*/
public Builder(Namespace namespace, String indexName, Long match)
{
super(namespace, indexName, match);
}
@Override
protected Builder self()
{
return this;
}
/**
* Construct the query.
* @return a new IntIndexQuery
*/
public IntIndexQuery build()
{
return new IntIndexQuery(this);
}
}
public static class Response extends SecondaryIndexQuery.Response>
{
Response(Namespace queryLocation, IndexConverter converter, int timeout, StreamingRiakFuture coreFuture)
{
super(queryLocation, converter, timeout, coreFuture);
}
protected Response(Namespace queryLocation, SecondaryIndexQueryOperation.Response coreResponse, IndexConverter converter)
{
super(queryLocation, coreResponse, converter);
}
}
}