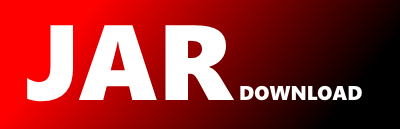
com.basho.riak.client.api.commands.indexes.KeyIndexQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
The newest version!
package com.basho.riak.client.api.commands.indexes;
import com.basho.riak.client.core.operations.SecondaryIndexQueryOperation;
import com.basho.riak.client.core.query.Namespace;
import com.basho.riak.client.core.query.indexes.IndexNames;
import com.basho.riak.client.core.util.BinaryValue;
import com.basho.riak.client.core.util.DefaultCharset;
/**
* Performs a 2i query across the special $key index, for a known bucket & range of keys,
* and returns the keys within that range in that bucket.
*
*
* A KeyIndexQuery is used when you want to fetch a range of keys for a bucket. A namespace and a key range is needed.
*
*
* {@code
* Namespace ns = new Namespace("my_type", "my_bucket");
* KeyIndexQuery q = new KeyIndexQuery.Builder(ns, "foo10", "foo19").build();
* RawIndexquery.Response resp = client.execute(q);}
*
*
* You can also stream the results back before the operation is fully complete.
* This reduces the time between executing the operation and seeing a result,
* and reduces overall memory usage if the iterator is consumed quickly enough.
* The result iterable can only be iterated once though.
* If the thread is interrupted while the iterator is polling for more results,
* a {@link RuntimeException} will be thrown.
*
* {@code
* Namespace ns = new Namespace("my_type", "my_bucket");
* KeyIndexQuery q = new KeyIndexQuery.Builder(ns, "foo10", "foo19").build();
* RiakFuture streamingFuture =
* client.executeAsyncStreaming(q, 200);
* RawIndexQuery.StreamingResponse streamingResponse = streamingFuture.get();
*
* for (BinIndexQuery.Response.Entry e : streamingResponse)
* {
* System.out.println(e.getRiakObjectLocation().getKeyAsString());
* }
* // Wait for the command to fully finish.
* streamingFuture.await();
* // The StreamingResponse will also contain the continuation, if the operation returned one.
* streamingResponse.getContinuation(); }
*
*
* @author Alex Moore
* @since 2.0.7
*/
public class KeyIndexQuery extends RawIndexQuery
{
private KeyIndexQuery(Init builder)
{
super(builder);
}
public static class Builder extends Init
{
public Builder(Namespace namespace, String start, String end)
{
this(namespace,
BinaryValue.unsafeCreate(start.getBytes(DefaultCharset.get())),
BinaryValue.unsafeCreate(end.getBytes(DefaultCharset.get())));
}
public Builder(Namespace namespace, BinaryValue start, BinaryValue end)
{
super(namespace, IndexNames.KEY, start, end);
}
@Override
protected Builder self()
{
return this;
}
public KeyIndexQuery build()
{
return new KeyIndexQuery(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy