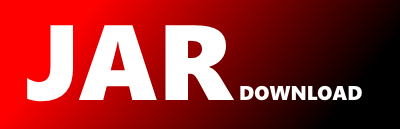
com.basho.riak.client.api.commands.kv.DeleteValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
The newest version!
/*
* Copyright 2013 Basho Technologies Inc
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basho.riak.client.api.commands.kv;
import com.basho.riak.client.api.AsIsRiakCommand;
import com.basho.riak.client.api.cap.Quorum;
import com.basho.riak.client.api.cap.VClock;
import com.basho.riak.client.core.RiakCluster;
import com.basho.riak.client.core.RiakFuture;
import com.basho.riak.client.core.operations.DeleteOperation;
import com.basho.riak.client.api.commands.RiakOption;
import com.basho.riak.client.core.query.Location;
import java.util.HashMap;
import java.util.Map;
/**
* Command used to delete a value from Riak.
*
*
* Deleting an object from Riak is a simple matter of supplying a {@link com.basho.riak.client.core.query.Location}
* and executing the operation.
*
*
* Note that this operation returns a {@code Void} type upon success. Any failure
* will be thrown as an exception when calling {@code DeleteValue} synchronously or
* when calling the future's get methods.
*
*
* {@code
* Namespace ns = new Namespace("my_type", "my_bucket");
* Location loc = new Location(ns, "my_key");
* DeleteValue dv = new DeleteValue.Builder(loc).build();
* client.execute(dv);}
*
*
* All operations can called async as well.
*
* {@code
* ...
* RiakFuture future = client.executeAsync(dv);
* ...
* future.await();
* if (future.isSuccess())
* {
* ...
* }}
*
*
* @author Dave Rusek
* @since 2.0
*/
public final class DeleteValue extends AsIsRiakCommand
{
private final Location location;
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy