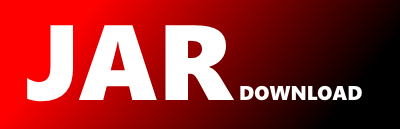
com.basho.riak.client.api.convert.ConverterFactory Maven / Gradle / Ivy
Show all versions of riak-client Show documentation
/*
* Copyright 2014 Basho Technologies Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basho.riak.client.api.convert;
import com.basho.riak.client.core.query.RiakObject;
import com.fasterxml.jackson.core.type.TypeReference;
import java.lang.reflect.Type;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Holds instances of converters to be used for serialization / deserialization
* of objects stored and fetched from Riak.
*
* When storing and retrieving your own domain objects to/from Riak, they
* need to be serialized / deserialized. By default the {@link JSONConverter}
* is provided. This uses the Jackson JSON library to translate your object to/from
* JSON. In many cases you will never need to create or register your own
* converter with the ConverterFactory.
*
*
* In the case you do need custom conversion, you would extend {@link Converter}
* and then register it with the ConverterFactory for your classes.
*
*
* @author Brian Roach
* @since 2.0
*/
public enum ConverterFactory
{
INSTANCE;
private final Map> converterInstances =
new ConcurrentHashMap>()
{{
put(new TypeReference() {}.getType(), new PassThroughConverter());
put(RiakObject.class, new PassThroughConverter());
put (new TypeReference() {}.getType(), new StringConverter());
put(String.class, new StringConverter());
}};
/**
* Get the instance of the ConverterFactory.
* @return the ConverterFactory
*/
public static ConverterFactory getInstance()
{
return INSTANCE;
}
/**
* Returns a Converter instance for the supplied class.
*
* If no converter is registered, the default {@link JSONConverter} is returned.
*
* @param The type for the converter
* @param type The type used to look up the converter
* @return an instance of the converter for this class
*/
public Converter getConverter(Type type)
{
return getConverter(type, null);
}
/**
* Returns a Converter instance for the supplied class.
*
* If no converter is registered, the default {@link JSONConverter} is returned.
*
* @param The type for the converter
* @param typeReference the TypeReference for the class being converted.
* @return an instance of the converter for this class
*/
public Converter getConverter(TypeReference typeReference)
{
return getConverter(null, typeReference);
}
@SuppressWarnings("unchecked")
private Converter getConverter(Type type, TypeReference typeReference)
{
type = type != null ? type : typeReference.getType();
Converter converter;
converter = (Converter) converterInstances.get(type);
if (converter == null)
{
if (typeReference != null)
{
// Should we cache this?
converter = new JSONConverter<>(typeReference);
}
else
{
converter = new JSONConverter<>(type);
}
}
return converter;
}
/**
* Register a converter for the supplied class.
*
* This instance be re-used for every conversion.
*
* @param The type being converted
* @param clazz the class for this converter.
* @param converter an instance of Converter
*/
public void registerConverterForClass(Class clazz, Converter converter)
{
converterInstances.put((Type)clazz, converter);
}
/**
* Register a converter for the supplied class.
*
* This instance be re-used for every conversion.
*
* @param The type being converted
* @param typeReference the TypeReference for the class being converted.
* @param converter an instance of Converter
*/
public void registerConverterForClass(TypeReference typeReference, Converter converter)
{
Type t = typeReference.getType();
converterInstances.put(t, converter);
}
/**
* Unregister a converter.
* @param The type
* @param clazz the class being converted
*/
public void unregisterConverterForClass(Class clazz)
{
converterInstances.remove((Type)clazz);
}
/**
* Unregister a converter.
* @param The type
* @param typeReference the TypeReference for the class being converted.
*/
public void unregisterConverterForClass(TypeReference typeReference)
{
Type t = typeReference.getType();
converterInstances.remove(t);
}
}