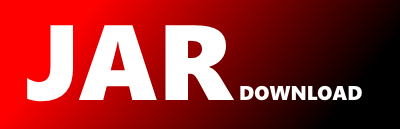
com.basho.riak.protobuf.RiakDtPB Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: riak_dt.proto
package com.basho.riak.protobuf;
public final class RiakDtPB {
private RiakDtPB() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface MapFieldOrBuilder extends
// @@protoc_insertion_point(interface_extends:MapField)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes name = 1;
*/
boolean hasName();
/**
* required bytes name = 1;
*/
com.google.protobuf.ByteString getName();
/**
* required .MapField.MapFieldType type = 2;
*/
boolean hasType();
/**
* required .MapField.MapFieldType type = 2;
*/
com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType getType();
}
/**
* Protobuf type {@code MapField}
*
*
* Field names in maps are composed of a binary identifier and a type.
* This is so that two clients can create fields with the same name
* but different types, and they converge independently.
*
*/
public static final class MapField extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:MapField)
MapFieldOrBuilder {
// Use MapField.newBuilder() to construct.
private MapField(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private MapField(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final MapField defaultInstance;
public static MapField getDefaultInstance() {
return defaultInstance;
}
public MapField getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MapField(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType value = com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
type_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapField_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapField_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.MapField.class, com.basho.riak.protobuf.RiakDtPB.MapField.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MapField parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MapField(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code MapField.MapFieldType}
*
*
* The types that can be stored in a map are limited to counters,
* sets, registers, flags, and maps.
*
*/
public enum MapFieldType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* COUNTER = 1;
*/
COUNTER(0, 1),
/**
* SET = 2;
*/
SET(1, 2),
/**
* REGISTER = 3;
*/
REGISTER(2, 3),
/**
* FLAG = 4;
*/
FLAG(3, 4),
/**
* MAP = 5;
*/
MAP(4, 5),
;
/**
* COUNTER = 1;
*/
public static final int COUNTER_VALUE = 1;
/**
* SET = 2;
*/
public static final int SET_VALUE = 2;
/**
* REGISTER = 3;
*/
public static final int REGISTER_VALUE = 3;
/**
* FLAG = 4;
*/
public static final int FLAG_VALUE = 4;
/**
* MAP = 5;
*/
public static final int MAP_VALUE = 5;
public final int getNumber() { return value; }
public static MapFieldType valueOf(int value) {
switch (value) {
case 1: return COUNTER;
case 2: return SET;
case 3: return REGISTER;
case 4: return FLAG;
case 5: return MAP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MapFieldType findValueByNumber(int number) {
return MapFieldType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.MapField.getDescriptor().getEnumTypes().get(0);
}
private static final MapFieldType[] VALUES = values();
public static MapFieldType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private MapFieldType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:MapField.MapFieldType)
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString name_;
/**
* required bytes name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes name = 1;
*/
public com.google.protobuf.ByteString getName() {
return name_;
}
public static final int TYPE_FIELD_NUMBER = 2;
private com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType type_;
/**
* required .MapField.MapFieldType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .MapField.MapFieldType type = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType getType() {
return type_;
}
private void initFields() {
name_ = com.google.protobuf.ByteString.EMPTY;
type_ = com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType.COUNTER;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, name_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, type_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, name_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapField parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.MapField prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code MapField}
*
*
* Field names in maps are composed of a binary identifier and a type.
* This is so that two clients can create fields with the same name
* but different types, and they converge independently.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:MapField)
com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapField_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapField_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.MapField.class, com.basho.riak.protobuf.RiakDtPB.MapField.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.MapField.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
type_ = com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType.COUNTER;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapField_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.MapField getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.MapField build() {
com.basho.riak.protobuf.RiakDtPB.MapField result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.MapField buildPartial() {
com.basho.riak.protobuf.RiakDtPB.MapField result = new com.basho.riak.protobuf.RiakDtPB.MapField(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.MapField) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.MapField)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.MapField other) {
if (other == com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasType()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.MapField parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.MapField) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes name = 1;
*/
public com.google.protobuf.ByteString getName() {
return name_;
}
/**
* required bytes name = 1;
*/
public Builder setName(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required bytes name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType type_ = com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType.COUNTER;
/**
* required .MapField.MapFieldType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .MapField.MapFieldType type = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType getType() {
return type_;
}
/**
* required .MapField.MapFieldType type = 2;
*/
public Builder setType(com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* required .MapField.MapFieldType type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = com.basho.riak.protobuf.RiakDtPB.MapField.MapFieldType.COUNTER;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:MapField)
}
static {
defaultInstance = new MapField(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MapField)
}
public interface MapEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:MapEntry)
com.google.protobuf.MessageOrBuilder {
/**
* required .MapField field = 1;
*/
boolean hasField();
/**
* required .MapField field = 1;
*/
com.basho.riak.protobuf.RiakDtPB.MapField getField();
/**
* required .MapField field = 1;
*/
com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getFieldOrBuilder();
/**
* optional sint64 counter_value = 2;
*/
boolean hasCounterValue();
/**
* optional sint64 counter_value = 2;
*/
long getCounterValue();
/**
* repeated bytes set_value = 3;
*/
java.util.List getSetValueList();
/**
* repeated bytes set_value = 3;
*/
int getSetValueCount();
/**
* repeated bytes set_value = 3;
*/
com.google.protobuf.ByteString getSetValue(int index);
/**
* optional bytes register_value = 4;
*/
boolean hasRegisterValue();
/**
* optional bytes register_value = 4;
*/
com.google.protobuf.ByteString getRegisterValue();
/**
* optional bool flag_value = 5;
*/
boolean hasFlagValue();
/**
* optional bool flag_value = 5;
*/
boolean getFlagValue();
/**
* repeated .MapEntry map_value = 6;
*/
java.util.List
getMapValueList();
/**
* repeated .MapEntry map_value = 6;
*/
com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index);
/**
* repeated .MapEntry map_value = 6;
*/
int getMapValueCount();
/**
* repeated .MapEntry map_value = 6;
*/
java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList();
/**
* repeated .MapEntry map_value = 6;
*/
com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index);
}
/**
* Protobuf type {@code MapEntry}
*
*
* An entry in a map is a pair of a field-name and value. The type
* defined in the field determines which value type is expected.
*
*/
public static final class MapEntry extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:MapEntry)
MapEntryOrBuilder {
// Use MapEntry.newBuilder() to construct.
private MapEntry(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private MapEntry(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final MapEntry defaultInstance;
public static MapEntry getDefaultInstance() {
return defaultInstance;
}
public MapEntry getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MapEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.basho.riak.protobuf.RiakDtPB.MapField.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = field_.toBuilder();
}
field_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapField.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(field_);
field_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
bitField0_ |= 0x00000002;
counterValue_ = input.readSInt64();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
setValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
setValue_.add(input.readBytes());
break;
}
case 34: {
bitField0_ |= 0x00000004;
registerValue_ = input.readBytes();
break;
}
case 40: {
bitField0_ |= 0x00000008;
flagValue_ = input.readBool();
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
mapValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
mapValue_.add(input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapEntry.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
setValue_ = java.util.Collections.unmodifiableList(setValue_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
mapValue_ = java.util.Collections.unmodifiableList(mapValue_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapEntry_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.MapEntry.class, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MapEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MapEntry(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int FIELD_FIELD_NUMBER = 1;
private com.basho.riak.protobuf.RiakDtPB.MapField field_;
/**
* required .MapField field = 1;
*/
public boolean hasField() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapField getField() {
return field_;
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getFieldOrBuilder() {
return field_;
}
public static final int COUNTER_VALUE_FIELD_NUMBER = 2;
private long counterValue_;
/**
* optional sint64 counter_value = 2;
*/
public boolean hasCounterValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional sint64 counter_value = 2;
*/
public long getCounterValue() {
return counterValue_;
}
public static final int SET_VALUE_FIELD_NUMBER = 3;
private java.util.List setValue_;
/**
* repeated bytes set_value = 3;
*/
public java.util.List
getSetValueList() {
return setValue_;
}
/**
* repeated bytes set_value = 3;
*/
public int getSetValueCount() {
return setValue_.size();
}
/**
* repeated bytes set_value = 3;
*/
public com.google.protobuf.ByteString getSetValue(int index) {
return setValue_.get(index);
}
public static final int REGISTER_VALUE_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString registerValue_;
/**
* optional bytes register_value = 4;
*/
public boolean hasRegisterValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes register_value = 4;
*/
public com.google.protobuf.ByteString getRegisterValue() {
return registerValue_;
}
public static final int FLAG_VALUE_FIELD_NUMBER = 5;
private boolean flagValue_;
/**
* optional bool flag_value = 5;
*/
public boolean hasFlagValue() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool flag_value = 5;
*/
public boolean getFlagValue() {
return flagValue_;
}
public static final int MAP_VALUE_FIELD_NUMBER = 6;
private java.util.List mapValue_;
/**
* repeated .MapEntry map_value = 6;
*/
public java.util.List getMapValueList() {
return mapValue_;
}
/**
* repeated .MapEntry map_value = 6;
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList() {
return mapValue_;
}
/**
* repeated .MapEntry map_value = 6;
*/
public int getMapValueCount() {
return mapValue_.size();
}
/**
* repeated .MapEntry map_value = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index) {
return mapValue_.get(index);
}
/**
* repeated .MapEntry map_value = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index) {
return mapValue_.get(index);
}
private void initFields() {
field_ = com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
counterValue_ = 0L;
setValue_ = java.util.Collections.emptyList();
registerValue_ = com.google.protobuf.ByteString.EMPTY;
flagValue_ = false;
mapValue_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasField()) {
memoizedIsInitialized = 0;
return false;
}
if (!getField().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getMapValueCount(); i++) {
if (!getMapValue(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, field_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeSInt64(2, counterValue_);
}
for (int i = 0; i < setValue_.size(); i++) {
output.writeBytes(3, setValue_.get(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(4, registerValue_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(5, flagValue_);
}
for (int i = 0; i < mapValue_.size(); i++) {
output.writeMessage(6, mapValue_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, field_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(2, counterValue_);
}
{
int dataSize = 0;
for (int i = 0; i < setValue_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(setValue_.get(i));
}
size += dataSize;
size += 1 * getSetValueList().size();
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, registerValue_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, flagValue_);
}
for (int i = 0; i < mapValue_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, mapValue_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.MapEntry prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code MapEntry}
*
*
* An entry in a map is a pair of a field-name and value. The type
* defined in the field determines which value type is expected.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:MapEntry)
com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapEntry_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.MapEntry.class, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.MapEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getFieldFieldBuilder();
getMapValueFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (fieldBuilder_ == null) {
field_ = com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
} else {
fieldBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
counterValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
setValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
registerValue_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
flagValue_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
if (mapValueBuilder_ == null) {
mapValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
mapValueBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapEntry_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.MapEntry getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.MapEntry.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.MapEntry build() {
com.basho.riak.protobuf.RiakDtPB.MapEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.MapEntry buildPartial() {
com.basho.riak.protobuf.RiakDtPB.MapEntry result = new com.basho.riak.protobuf.RiakDtPB.MapEntry(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (fieldBuilder_ == null) {
result.field_ = field_;
} else {
result.field_ = fieldBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.counterValue_ = counterValue_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
setValue_ = java.util.Collections.unmodifiableList(setValue_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.setValue_ = setValue_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.registerValue_ = registerValue_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.flagValue_ = flagValue_;
if (mapValueBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
mapValue_ = java.util.Collections.unmodifiableList(mapValue_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.mapValue_ = mapValue_;
} else {
result.mapValue_ = mapValueBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.MapEntry) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.MapEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.MapEntry other) {
if (other == com.basho.riak.protobuf.RiakDtPB.MapEntry.getDefaultInstance()) return this;
if (other.hasField()) {
mergeField(other.getField());
}
if (other.hasCounterValue()) {
setCounterValue(other.getCounterValue());
}
if (!other.setValue_.isEmpty()) {
if (setValue_.isEmpty()) {
setValue_ = other.setValue_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureSetValueIsMutable();
setValue_.addAll(other.setValue_);
}
onChanged();
}
if (other.hasRegisterValue()) {
setRegisterValue(other.getRegisterValue());
}
if (other.hasFlagValue()) {
setFlagValue(other.getFlagValue());
}
if (mapValueBuilder_ == null) {
if (!other.mapValue_.isEmpty()) {
if (mapValue_.isEmpty()) {
mapValue_ = other.mapValue_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureMapValueIsMutable();
mapValue_.addAll(other.mapValue_);
}
onChanged();
}
} else {
if (!other.mapValue_.isEmpty()) {
if (mapValueBuilder_.isEmpty()) {
mapValueBuilder_.dispose();
mapValueBuilder_ = null;
mapValue_ = other.mapValue_;
bitField0_ = (bitField0_ & ~0x00000020);
mapValueBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getMapValueFieldBuilder() : null;
} else {
mapValueBuilder_.addAllMessages(other.mapValue_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasField()) {
return false;
}
if (!getField().isInitialized()) {
return false;
}
for (int i = 0; i < getMapValueCount(); i++) {
if (!getMapValue(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.MapEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.MapEntry) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.basho.riak.protobuf.RiakDtPB.MapField field_ = com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder> fieldBuilder_;
/**
* required .MapField field = 1;
*/
public boolean hasField() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapField getField() {
if (fieldBuilder_ == null) {
return field_;
} else {
return fieldBuilder_.getMessage();
}
}
/**
* required .MapField field = 1;
*/
public Builder setField(com.basho.riak.protobuf.RiakDtPB.MapField value) {
if (fieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
field_ = value;
onChanged();
} else {
fieldBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .MapField field = 1;
*/
public Builder setField(
com.basho.riak.protobuf.RiakDtPB.MapField.Builder builderForValue) {
if (fieldBuilder_ == null) {
field_ = builderForValue.build();
onChanged();
} else {
fieldBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .MapField field = 1;
*/
public Builder mergeField(com.basho.riak.protobuf.RiakDtPB.MapField value) {
if (fieldBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
field_ != com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance()) {
field_ =
com.basho.riak.protobuf.RiakDtPB.MapField.newBuilder(field_).mergeFrom(value).buildPartial();
} else {
field_ = value;
}
onChanged();
} else {
fieldBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .MapField field = 1;
*/
public Builder clearField() {
if (fieldBuilder_ == null) {
field_ = com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
onChanged();
} else {
fieldBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapField.Builder getFieldBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getFieldFieldBuilder().getBuilder();
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getFieldOrBuilder() {
if (fieldBuilder_ != null) {
return fieldBuilder_.getMessageOrBuilder();
} else {
return field_;
}
}
/**
* required .MapField field = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>
getFieldFieldBuilder() {
if (fieldBuilder_ == null) {
fieldBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>(
getField(),
getParentForChildren(),
isClean());
field_ = null;
}
return fieldBuilder_;
}
private long counterValue_ ;
/**
* optional sint64 counter_value = 2;
*/
public boolean hasCounterValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional sint64 counter_value = 2;
*/
public long getCounterValue() {
return counterValue_;
}
/**
* optional sint64 counter_value = 2;
*/
public Builder setCounterValue(long value) {
bitField0_ |= 0x00000002;
counterValue_ = value;
onChanged();
return this;
}
/**
* optional sint64 counter_value = 2;
*/
public Builder clearCounterValue() {
bitField0_ = (bitField0_ & ~0x00000002);
counterValue_ = 0L;
onChanged();
return this;
}
private java.util.List setValue_ = java.util.Collections.emptyList();
private void ensureSetValueIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
setValue_ = new java.util.ArrayList(setValue_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated bytes set_value = 3;
*/
public java.util.List
getSetValueList() {
return java.util.Collections.unmodifiableList(setValue_);
}
/**
* repeated bytes set_value = 3;
*/
public int getSetValueCount() {
return setValue_.size();
}
/**
* repeated bytes set_value = 3;
*/
public com.google.protobuf.ByteString getSetValue(int index) {
return setValue_.get(index);
}
/**
* repeated bytes set_value = 3;
*/
public Builder setSetValue(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSetValueIsMutable();
setValue_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes set_value = 3;
*/
public Builder addSetValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSetValueIsMutable();
setValue_.add(value);
onChanged();
return this;
}
/**
* repeated bytes set_value = 3;
*/
public Builder addAllSetValue(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureSetValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, setValue_);
onChanged();
return this;
}
/**
* repeated bytes set_value = 3;
*/
public Builder clearSetValue() {
setValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
private com.google.protobuf.ByteString registerValue_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes register_value = 4;
*/
public boolean hasRegisterValue() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes register_value = 4;
*/
public com.google.protobuf.ByteString getRegisterValue() {
return registerValue_;
}
/**
* optional bytes register_value = 4;
*/
public Builder setRegisterValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
registerValue_ = value;
onChanged();
return this;
}
/**
* optional bytes register_value = 4;
*/
public Builder clearRegisterValue() {
bitField0_ = (bitField0_ & ~0x00000008);
registerValue_ = getDefaultInstance().getRegisterValue();
onChanged();
return this;
}
private boolean flagValue_ ;
/**
* optional bool flag_value = 5;
*/
public boolean hasFlagValue() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool flag_value = 5;
*/
public boolean getFlagValue() {
return flagValue_;
}
/**
* optional bool flag_value = 5;
*/
public Builder setFlagValue(boolean value) {
bitField0_ |= 0x00000010;
flagValue_ = value;
onChanged();
return this;
}
/**
* optional bool flag_value = 5;
*/
public Builder clearFlagValue() {
bitField0_ = (bitField0_ & ~0x00000010);
flagValue_ = false;
onChanged();
return this;
}
private java.util.List mapValue_ =
java.util.Collections.emptyList();
private void ensureMapValueIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
mapValue_ = new java.util.ArrayList(mapValue_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder> mapValueBuilder_;
/**
* repeated .MapEntry map_value = 6;
*/
public java.util.List getMapValueList() {
if (mapValueBuilder_ == null) {
return java.util.Collections.unmodifiableList(mapValue_);
} else {
return mapValueBuilder_.getMessageList();
}
}
/**
* repeated .MapEntry map_value = 6;
*/
public int getMapValueCount() {
if (mapValueBuilder_ == null) {
return mapValue_.size();
} else {
return mapValueBuilder_.getCount();
}
}
/**
* repeated .MapEntry map_value = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index) {
if (mapValueBuilder_ == null) {
return mapValue_.get(index);
} else {
return mapValueBuilder_.getMessage(index);
}
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder setMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.set(index, value);
onChanged();
} else {
mapValueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder setMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.set(index, builderForValue.build());
onChanged();
} else {
mapValueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder addMapValue(com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.add(value);
onChanged();
} else {
mapValueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder addMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.add(index, value);
onChanged();
} else {
mapValueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder addMapValue(
com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.add(builderForValue.build());
onChanged();
} else {
mapValueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder addMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.add(index, builderForValue.build());
onChanged();
} else {
mapValueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder addAllMapValue(
java.lang.Iterable extends com.basho.riak.protobuf.RiakDtPB.MapEntry> values) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, mapValue_);
onChanged();
} else {
mapValueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder clearMapValue() {
if (mapValueBuilder_ == null) {
mapValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
mapValueBuilder_.clear();
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public Builder removeMapValue(int index) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.remove(index);
onChanged();
} else {
mapValueBuilder_.remove(index);
}
return this;
}
/**
* repeated .MapEntry map_value = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder getMapValueBuilder(
int index) {
return getMapValueFieldBuilder().getBuilder(index);
}
/**
* repeated .MapEntry map_value = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index) {
if (mapValueBuilder_ == null) {
return mapValue_.get(index); } else {
return mapValueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .MapEntry map_value = 6;
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList() {
if (mapValueBuilder_ != null) {
return mapValueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(mapValue_);
}
}
/**
* repeated .MapEntry map_value = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder addMapValueBuilder() {
return getMapValueFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakDtPB.MapEntry.getDefaultInstance());
}
/**
* repeated .MapEntry map_value = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder addMapValueBuilder(
int index) {
return getMapValueFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakDtPB.MapEntry.getDefaultInstance());
}
/**
* repeated .MapEntry map_value = 6;
*/
public java.util.List
getMapValueBuilderList() {
return getMapValueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueFieldBuilder() {
if (mapValueBuilder_ == null) {
mapValueBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>(
mapValue_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
mapValue_ = null;
}
return mapValueBuilder_;
}
// @@protoc_insertion_point(builder_scope:MapEntry)
}
static {
defaultInstance = new MapEntry(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MapEntry)
}
public interface DtFetchReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:DtFetchReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes bucket = 1;
*
*
* The identifier: bucket, key and bucket-type
*
*/
boolean hasBucket();
/**
* required bytes bucket = 1;
*
*
* The identifier: bucket, key and bucket-type
*
*/
com.google.protobuf.ByteString getBucket();
/**
* required bytes key = 2;
*/
boolean hasKey();
/**
* required bytes key = 2;
*/
com.google.protobuf.ByteString getKey();
/**
* required bytes type = 3;
*/
boolean hasType();
/**
* required bytes type = 3;
*/
com.google.protobuf.ByteString getType();
/**
* optional uint32 r = 4;
*
*
* Request options
*
*/
boolean hasR();
/**
* optional uint32 r = 4;
*
*
* Request options
*
*/
int getR();
/**
* optional uint32 pr = 5;
*/
boolean hasPr();
/**
* optional uint32 pr = 5;
*/
int getPr();
/**
* optional bool basic_quorum = 6;
*/
boolean hasBasicQuorum();
/**
* optional bool basic_quorum = 6;
*/
boolean getBasicQuorum();
/**
* optional bool notfound_ok = 7;
*/
boolean hasNotfoundOk();
/**
* optional bool notfound_ok = 7;
*/
boolean getNotfoundOk();
/**
* optional uint32 timeout = 8;
*/
boolean hasTimeout();
/**
* optional uint32 timeout = 8;
*/
int getTimeout();
/**
* optional bool sloppy_quorum = 9;
*
*
* Experimental, may change/disappear
*
*/
boolean hasSloppyQuorum();
/**
* optional bool sloppy_quorum = 9;
*
*
* Experimental, may change/disappear
*
*/
boolean getSloppyQuorum();
/**
* optional uint32 n_val = 10;
*
*
* Experimental, may change/disappear
*
*/
boolean hasNVal();
/**
* optional uint32 n_val = 10;
*
*
* Experimental, may change/disappear
*
*/
int getNVal();
/**
* optional bool include_context = 11 [default = true];
*
*
* For read-only requests or context-free operations, you can set
* this to false to reduce the size of the response payload.
*
*/
boolean hasIncludeContext();
/**
* optional bool include_context = 11 [default = true];
*
*
* For read-only requests or context-free operations, you can set
* this to false to reduce the size of the response payload.
*
*/
boolean getIncludeContext();
}
/**
* Protobuf type {@code DtFetchReq}
*
*
* The equivalent of KV's "RpbGetReq", results in a DtFetchResp. The
* request-time options are limited to ones that are relevant to
* structured data-types.
*
*/
public static final class DtFetchReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:DtFetchReq)
DtFetchReqOrBuilder {
// Use DtFetchReq.newBuilder() to construct.
private DtFetchReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private DtFetchReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final DtFetchReq defaultInstance;
public static DtFetchReq getDefaultInstance() {
return defaultInstance;
}
public DtFetchReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DtFetchReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
bucket_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
key_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
type_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000008;
r_ = input.readUInt32();
break;
}
case 40: {
bitField0_ |= 0x00000010;
pr_ = input.readUInt32();
break;
}
case 48: {
bitField0_ |= 0x00000020;
basicQuorum_ = input.readBool();
break;
}
case 56: {
bitField0_ |= 0x00000040;
notfoundOk_ = input.readBool();
break;
}
case 64: {
bitField0_ |= 0x00000080;
timeout_ = input.readUInt32();
break;
}
case 72: {
bitField0_ |= 0x00000100;
sloppyQuorum_ = input.readBool();
break;
}
case 80: {
bitField0_ |= 0x00000200;
nVal_ = input.readUInt32();
break;
}
case 88: {
bitField0_ |= 0x00000400;
includeContext_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtFetchReq.class, com.basho.riak.protobuf.RiakDtPB.DtFetchReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public DtFetchReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DtFetchReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int BUCKET_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString bucket_;
/**
* required bytes bucket = 1;
*
*
* The identifier: bucket, key and bucket-type
*
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*
*
* The identifier: bucket, key and bucket-type
*
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
public static final int KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString key_;
/**
* required bytes key = 2;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes key = 2;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int TYPE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString type_;
/**
* required bytes type = 3;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bytes type = 3;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
public static final int R_FIELD_NUMBER = 4;
private int r_;
/**
* optional uint32 r = 4;
*
*
* Request options
*
*/
public boolean hasR() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 r = 4;
*
*
* Request options
*
*/
public int getR() {
return r_;
}
public static final int PR_FIELD_NUMBER = 5;
private int pr_;
/**
* optional uint32 pr = 5;
*/
public boolean hasPr() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint32 pr = 5;
*/
public int getPr() {
return pr_;
}
public static final int BASIC_QUORUM_FIELD_NUMBER = 6;
private boolean basicQuorum_;
/**
* optional bool basic_quorum = 6;
*/
public boolean hasBasicQuorum() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool basic_quorum = 6;
*/
public boolean getBasicQuorum() {
return basicQuorum_;
}
public static final int NOTFOUND_OK_FIELD_NUMBER = 7;
private boolean notfoundOk_;
/**
* optional bool notfound_ok = 7;
*/
public boolean hasNotfoundOk() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool notfound_ok = 7;
*/
public boolean getNotfoundOk() {
return notfoundOk_;
}
public static final int TIMEOUT_FIELD_NUMBER = 8;
private int timeout_;
/**
* optional uint32 timeout = 8;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 timeout = 8;
*/
public int getTimeout() {
return timeout_;
}
public static final int SLOPPY_QUORUM_FIELD_NUMBER = 9;
private boolean sloppyQuorum_;
/**
* optional bool sloppy_quorum = 9;
*
*
* Experimental, may change/disappear
*
*/
public boolean hasSloppyQuorum() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool sloppy_quorum = 9;
*
*
* Experimental, may change/disappear
*
*/
public boolean getSloppyQuorum() {
return sloppyQuorum_;
}
public static final int N_VAL_FIELD_NUMBER = 10;
private int nVal_;
/**
* optional uint32 n_val = 10;
*
*
* Experimental, may change/disappear
*
*/
public boolean hasNVal() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional uint32 n_val = 10;
*
*
* Experimental, may change/disappear
*
*/
public int getNVal() {
return nVal_;
}
public static final int INCLUDE_CONTEXT_FIELD_NUMBER = 11;
private boolean includeContext_;
/**
* optional bool include_context = 11 [default = true];
*
*
* For read-only requests or context-free operations, you can set
* this to false to reduce the size of the response payload.
*
*/
public boolean hasIncludeContext() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool include_context = 11 [default = true];
*
*
* For read-only requests or context-free operations, you can set
* this to false to reduce the size of the response payload.
*
*/
public boolean getIncludeContext() {
return includeContext_;
}
private void initFields() {
bucket_ = com.google.protobuf.ByteString.EMPTY;
key_ = com.google.protobuf.ByteString.EMPTY;
type_ = com.google.protobuf.ByteString.EMPTY;
r_ = 0;
pr_ = 0;
basicQuorum_ = false;
notfoundOk_ = false;
timeout_ = 0;
sloppyQuorum_ = false;
nVal_ = 0;
includeContext_ = true;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasBucket()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, key_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, type_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt32(4, r_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeUInt32(5, pr_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBool(6, basicQuorum_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBool(7, notfoundOk_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeUInt32(8, timeout_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(9, sloppyQuorum_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeUInt32(10, nVal_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBool(11, includeContext_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, key_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, type_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, r_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, pr_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, basicQuorum_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, notfoundOk_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(8, timeout_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, sloppyQuorum_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(10, nVal_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, includeContext_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.DtFetchReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code DtFetchReq}
*
*
* The equivalent of KV's "RpbGetReq", results in a DtFetchResp. The
* request-time options are limited to ones that are relevant to
* structured data-types.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:DtFetchReq)
com.basho.riak.protobuf.RiakDtPB.DtFetchReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtFetchReq.class, com.basho.riak.protobuf.RiakDtPB.DtFetchReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.DtFetchReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
bucket_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
key_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
type_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
r_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
pr_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
basicQuorum_ = false;
bitField0_ = (bitField0_ & ~0x00000020);
notfoundOk_ = false;
bitField0_ = (bitField0_ & ~0x00000040);
timeout_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
sloppyQuorum_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
nVal_ = 0;
bitField0_ = (bitField0_ & ~0x00000200);
includeContext_ = true;
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchReq_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.DtFetchReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.DtFetchReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.DtFetchReq build() {
com.basho.riak.protobuf.RiakDtPB.DtFetchReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.DtFetchReq buildPartial() {
com.basho.riak.protobuf.RiakDtPB.DtFetchReq result = new com.basho.riak.protobuf.RiakDtPB.DtFetchReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.bucket_ = bucket_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.r_ = r_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.pr_ = pr_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.basicQuorum_ = basicQuorum_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.notfoundOk_ = notfoundOk_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.timeout_ = timeout_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.sloppyQuorum_ = sloppyQuorum_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.nVal_ = nVal_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
result.includeContext_ = includeContext_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.DtFetchReq) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.DtFetchReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.DtFetchReq other) {
if (other == com.basho.riak.protobuf.RiakDtPB.DtFetchReq.getDefaultInstance()) return this;
if (other.hasBucket()) {
setBucket(other.getBucket());
}
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasR()) {
setR(other.getR());
}
if (other.hasPr()) {
setPr(other.getPr());
}
if (other.hasBasicQuorum()) {
setBasicQuorum(other.getBasicQuorum());
}
if (other.hasNotfoundOk()) {
setNotfoundOk(other.getNotfoundOk());
}
if (other.hasTimeout()) {
setTimeout(other.getTimeout());
}
if (other.hasSloppyQuorum()) {
setSloppyQuorum(other.getSloppyQuorum());
}
if (other.hasNVal()) {
setNVal(other.getNVal());
}
if (other.hasIncludeContext()) {
setIncludeContext(other.getIncludeContext());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasBucket()) {
return false;
}
if (!hasKey()) {
return false;
}
if (!hasType()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.DtFetchReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.DtFetchReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString bucket_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes bucket = 1;
*
*
* The identifier: bucket, key and bucket-type
*
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*
*
* The identifier: bucket, key and bucket-type
*
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
/**
* required bytes bucket = 1;
*
*
* The identifier: bucket, key and bucket-type
*
*/
public Builder setBucket(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
bucket_ = value;
onChanged();
return this;
}
/**
* required bytes bucket = 1;
*
*
* The identifier: bucket, key and bucket-type
*
*/
public Builder clearBucket() {
bitField0_ = (bitField0_ & ~0x00000001);
bucket_ = getDefaultInstance().getBucket();
onChanged();
return this;
}
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 2;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes key = 2;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* required bytes key = 2;
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
key_ = value;
onChanged();
return this;
}
/**
* required bytes key = 2;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000002);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString type_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes type = 3;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bytes type = 3;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
/**
* required bytes type = 3;
*/
public Builder setType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value;
onChanged();
return this;
}
/**
* required bytes type = 3;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000004);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
private int r_ ;
/**
* optional uint32 r = 4;
*
*
* Request options
*
*/
public boolean hasR() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 r = 4;
*
*
* Request options
*
*/
public int getR() {
return r_;
}
/**
* optional uint32 r = 4;
*
*
* Request options
*
*/
public Builder setR(int value) {
bitField0_ |= 0x00000008;
r_ = value;
onChanged();
return this;
}
/**
* optional uint32 r = 4;
*
*
* Request options
*
*/
public Builder clearR() {
bitField0_ = (bitField0_ & ~0x00000008);
r_ = 0;
onChanged();
return this;
}
private int pr_ ;
/**
* optional uint32 pr = 5;
*/
public boolean hasPr() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint32 pr = 5;
*/
public int getPr() {
return pr_;
}
/**
* optional uint32 pr = 5;
*/
public Builder setPr(int value) {
bitField0_ |= 0x00000010;
pr_ = value;
onChanged();
return this;
}
/**
* optional uint32 pr = 5;
*/
public Builder clearPr() {
bitField0_ = (bitField0_ & ~0x00000010);
pr_ = 0;
onChanged();
return this;
}
private boolean basicQuorum_ ;
/**
* optional bool basic_quorum = 6;
*/
public boolean hasBasicQuorum() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool basic_quorum = 6;
*/
public boolean getBasicQuorum() {
return basicQuorum_;
}
/**
* optional bool basic_quorum = 6;
*/
public Builder setBasicQuorum(boolean value) {
bitField0_ |= 0x00000020;
basicQuorum_ = value;
onChanged();
return this;
}
/**
* optional bool basic_quorum = 6;
*/
public Builder clearBasicQuorum() {
bitField0_ = (bitField0_ & ~0x00000020);
basicQuorum_ = false;
onChanged();
return this;
}
private boolean notfoundOk_ ;
/**
* optional bool notfound_ok = 7;
*/
public boolean hasNotfoundOk() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool notfound_ok = 7;
*/
public boolean getNotfoundOk() {
return notfoundOk_;
}
/**
* optional bool notfound_ok = 7;
*/
public Builder setNotfoundOk(boolean value) {
bitField0_ |= 0x00000040;
notfoundOk_ = value;
onChanged();
return this;
}
/**
* optional bool notfound_ok = 7;
*/
public Builder clearNotfoundOk() {
bitField0_ = (bitField0_ & ~0x00000040);
notfoundOk_ = false;
onChanged();
return this;
}
private int timeout_ ;
/**
* optional uint32 timeout = 8;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 timeout = 8;
*/
public int getTimeout() {
return timeout_;
}
/**
* optional uint32 timeout = 8;
*/
public Builder setTimeout(int value) {
bitField0_ |= 0x00000080;
timeout_ = value;
onChanged();
return this;
}
/**
* optional uint32 timeout = 8;
*/
public Builder clearTimeout() {
bitField0_ = (bitField0_ & ~0x00000080);
timeout_ = 0;
onChanged();
return this;
}
private boolean sloppyQuorum_ ;
/**
* optional bool sloppy_quorum = 9;
*
*
* Experimental, may change/disappear
*
*/
public boolean hasSloppyQuorum() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool sloppy_quorum = 9;
*
*
* Experimental, may change/disappear
*
*/
public boolean getSloppyQuorum() {
return sloppyQuorum_;
}
/**
* optional bool sloppy_quorum = 9;
*
*
* Experimental, may change/disappear
*
*/
public Builder setSloppyQuorum(boolean value) {
bitField0_ |= 0x00000100;
sloppyQuorum_ = value;
onChanged();
return this;
}
/**
* optional bool sloppy_quorum = 9;
*
*
* Experimental, may change/disappear
*
*/
public Builder clearSloppyQuorum() {
bitField0_ = (bitField0_ & ~0x00000100);
sloppyQuorum_ = false;
onChanged();
return this;
}
private int nVal_ ;
/**
* optional uint32 n_val = 10;
*
*
* Experimental, may change/disappear
*
*/
public boolean hasNVal() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional uint32 n_val = 10;
*
*
* Experimental, may change/disappear
*
*/
public int getNVal() {
return nVal_;
}
/**
* optional uint32 n_val = 10;
*
*
* Experimental, may change/disappear
*
*/
public Builder setNVal(int value) {
bitField0_ |= 0x00000200;
nVal_ = value;
onChanged();
return this;
}
/**
* optional uint32 n_val = 10;
*
*
* Experimental, may change/disappear
*
*/
public Builder clearNVal() {
bitField0_ = (bitField0_ & ~0x00000200);
nVal_ = 0;
onChanged();
return this;
}
private boolean includeContext_ = true;
/**
* optional bool include_context = 11 [default = true];
*
*
* For read-only requests or context-free operations, you can set
* this to false to reduce the size of the response payload.
*
*/
public boolean hasIncludeContext() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool include_context = 11 [default = true];
*
*
* For read-only requests or context-free operations, you can set
* this to false to reduce the size of the response payload.
*
*/
public boolean getIncludeContext() {
return includeContext_;
}
/**
* optional bool include_context = 11 [default = true];
*
*
* For read-only requests or context-free operations, you can set
* this to false to reduce the size of the response payload.
*
*/
public Builder setIncludeContext(boolean value) {
bitField0_ |= 0x00000400;
includeContext_ = value;
onChanged();
return this;
}
/**
* optional bool include_context = 11 [default = true];
*
*
* For read-only requests or context-free operations, you can set
* this to false to reduce the size of the response payload.
*
*/
public Builder clearIncludeContext() {
bitField0_ = (bitField0_ & ~0x00000400);
includeContext_ = true;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:DtFetchReq)
}
static {
defaultInstance = new DtFetchReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:DtFetchReq)
}
public interface DtValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:DtValue)
com.google.protobuf.MessageOrBuilder {
/**
* optional sint64 counter_value = 1;
*/
boolean hasCounterValue();
/**
* optional sint64 counter_value = 1;
*/
long getCounterValue();
/**
* repeated bytes set_value = 2;
*/
java.util.List getSetValueList();
/**
* repeated bytes set_value = 2;
*/
int getSetValueCount();
/**
* repeated bytes set_value = 2;
*/
com.google.protobuf.ByteString getSetValue(int index);
/**
* repeated .MapEntry map_value = 3;
*/
java.util.List
getMapValueList();
/**
* repeated .MapEntry map_value = 3;
*/
com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index);
/**
* repeated .MapEntry map_value = 3;
*/
int getMapValueCount();
/**
* repeated .MapEntry map_value = 3;
*/
java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList();
/**
* repeated .MapEntry map_value = 3;
*/
com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index);
/**
* optional uint64 hll_value = 4;
*
*
* We return an estimated cardinality of the Hyperloglog set
* on fetch.
*
*/
boolean hasHllValue();
/**
* optional uint64 hll_value = 4;
*
*
* We return an estimated cardinality of the Hyperloglog set
* on fetch.
*
*/
long getHllValue();
/**
* repeated bytes gset_value = 5;
*/
java.util.List getGsetValueList();
/**
* repeated bytes gset_value = 5;
*/
int getGsetValueCount();
/**
* repeated bytes gset_value = 5;
*/
com.google.protobuf.ByteString getGsetValue(int index);
}
/**
* Protobuf type {@code DtValue}
*
*
* The value of the fetched data type. If present in the response,
* then empty values (sets, maps) should be treated as such.
*
*/
public static final class DtValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:DtValue)
DtValueOrBuilder {
// Use DtValue.newBuilder() to construct.
private DtValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private DtValue(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final DtValue defaultInstance;
public static DtValue getDefaultInstance() {
return defaultInstance;
}
public DtValue getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DtValue(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
counterValue_ = input.readSInt64();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
setValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
setValue_.add(input.readBytes());
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
mapValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
mapValue_.add(input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapEntry.PARSER, extensionRegistry));
break;
}
case 32: {
bitField0_ |= 0x00000002;
hllValue_ = input.readUInt64();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
gsetValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
gsetValue_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
setValue_ = java.util.Collections.unmodifiableList(setValue_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
mapValue_ = java.util.Collections.unmodifiableList(mapValue_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
gsetValue_ = java.util.Collections.unmodifiableList(gsetValue_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtValue.class, com.basho.riak.protobuf.RiakDtPB.DtValue.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public DtValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DtValue(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int COUNTER_VALUE_FIELD_NUMBER = 1;
private long counterValue_;
/**
* optional sint64 counter_value = 1;
*/
public boolean hasCounterValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional sint64 counter_value = 1;
*/
public long getCounterValue() {
return counterValue_;
}
public static final int SET_VALUE_FIELD_NUMBER = 2;
private java.util.List setValue_;
/**
* repeated bytes set_value = 2;
*/
public java.util.List
getSetValueList() {
return setValue_;
}
/**
* repeated bytes set_value = 2;
*/
public int getSetValueCount() {
return setValue_.size();
}
/**
* repeated bytes set_value = 2;
*/
public com.google.protobuf.ByteString getSetValue(int index) {
return setValue_.get(index);
}
public static final int MAP_VALUE_FIELD_NUMBER = 3;
private java.util.List mapValue_;
/**
* repeated .MapEntry map_value = 3;
*/
public java.util.List getMapValueList() {
return mapValue_;
}
/**
* repeated .MapEntry map_value = 3;
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList() {
return mapValue_;
}
/**
* repeated .MapEntry map_value = 3;
*/
public int getMapValueCount() {
return mapValue_.size();
}
/**
* repeated .MapEntry map_value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index) {
return mapValue_.get(index);
}
/**
* repeated .MapEntry map_value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index) {
return mapValue_.get(index);
}
public static final int HLL_VALUE_FIELD_NUMBER = 4;
private long hllValue_;
/**
* optional uint64 hll_value = 4;
*
*
* We return an estimated cardinality of the Hyperloglog set
* on fetch.
*
*/
public boolean hasHllValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 hll_value = 4;
*
*
* We return an estimated cardinality of the Hyperloglog set
* on fetch.
*
*/
public long getHllValue() {
return hllValue_;
}
public static final int GSET_VALUE_FIELD_NUMBER = 5;
private java.util.List gsetValue_;
/**
* repeated bytes gset_value = 5;
*/
public java.util.List
getGsetValueList() {
return gsetValue_;
}
/**
* repeated bytes gset_value = 5;
*/
public int getGsetValueCount() {
return gsetValue_.size();
}
/**
* repeated bytes gset_value = 5;
*/
public com.google.protobuf.ByteString getGsetValue(int index) {
return gsetValue_.get(index);
}
private void initFields() {
counterValue_ = 0L;
setValue_ = java.util.Collections.emptyList();
mapValue_ = java.util.Collections.emptyList();
hllValue_ = 0L;
gsetValue_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getMapValueCount(); i++) {
if (!getMapValue(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeSInt64(1, counterValue_);
}
for (int i = 0; i < setValue_.size(); i++) {
output.writeBytes(2, setValue_.get(i));
}
for (int i = 0; i < mapValue_.size(); i++) {
output.writeMessage(3, mapValue_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt64(4, hllValue_);
}
for (int i = 0; i < gsetValue_.size(); i++) {
output.writeBytes(5, gsetValue_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, counterValue_);
}
{
int dataSize = 0;
for (int i = 0; i < setValue_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(setValue_.get(i));
}
size += dataSize;
size += 1 * getSetValueList().size();
}
for (int i = 0; i < mapValue_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, mapValue_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, hllValue_);
}
{
int dataSize = 0;
for (int i = 0; i < gsetValue_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(gsetValue_.get(i));
}
size += dataSize;
size += 1 * getGsetValueList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.DtValue prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code DtValue}
*
*
* The value of the fetched data type. If present in the response,
* then empty values (sets, maps) should be treated as such.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:DtValue)
com.basho.riak.protobuf.RiakDtPB.DtValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtValue_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtValue.class, com.basho.riak.protobuf.RiakDtPB.DtValue.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.DtValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getMapValueFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
counterValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
setValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
if (mapValueBuilder_ == null) {
mapValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
mapValueBuilder_.clear();
}
hllValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
gsetValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtValue_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.DtValue getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.DtValue.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.DtValue build() {
com.basho.riak.protobuf.RiakDtPB.DtValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.DtValue buildPartial() {
com.basho.riak.protobuf.RiakDtPB.DtValue result = new com.basho.riak.protobuf.RiakDtPB.DtValue(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.counterValue_ = counterValue_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
setValue_ = java.util.Collections.unmodifiableList(setValue_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.setValue_ = setValue_;
if (mapValueBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
mapValue_ = java.util.Collections.unmodifiableList(mapValue_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.mapValue_ = mapValue_;
} else {
result.mapValue_ = mapValueBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000002;
}
result.hllValue_ = hllValue_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
gsetValue_ = java.util.Collections.unmodifiableList(gsetValue_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.gsetValue_ = gsetValue_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.DtValue) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.DtValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.DtValue other) {
if (other == com.basho.riak.protobuf.RiakDtPB.DtValue.getDefaultInstance()) return this;
if (other.hasCounterValue()) {
setCounterValue(other.getCounterValue());
}
if (!other.setValue_.isEmpty()) {
if (setValue_.isEmpty()) {
setValue_ = other.setValue_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSetValueIsMutable();
setValue_.addAll(other.setValue_);
}
onChanged();
}
if (mapValueBuilder_ == null) {
if (!other.mapValue_.isEmpty()) {
if (mapValue_.isEmpty()) {
mapValue_ = other.mapValue_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureMapValueIsMutable();
mapValue_.addAll(other.mapValue_);
}
onChanged();
}
} else {
if (!other.mapValue_.isEmpty()) {
if (mapValueBuilder_.isEmpty()) {
mapValueBuilder_.dispose();
mapValueBuilder_ = null;
mapValue_ = other.mapValue_;
bitField0_ = (bitField0_ & ~0x00000004);
mapValueBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getMapValueFieldBuilder() : null;
} else {
mapValueBuilder_.addAllMessages(other.mapValue_);
}
}
}
if (other.hasHllValue()) {
setHllValue(other.getHllValue());
}
if (!other.gsetValue_.isEmpty()) {
if (gsetValue_.isEmpty()) {
gsetValue_ = other.gsetValue_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureGsetValueIsMutable();
gsetValue_.addAll(other.gsetValue_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getMapValueCount(); i++) {
if (!getMapValue(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.DtValue parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.DtValue) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long counterValue_ ;
/**
* optional sint64 counter_value = 1;
*/
public boolean hasCounterValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional sint64 counter_value = 1;
*/
public long getCounterValue() {
return counterValue_;
}
/**
* optional sint64 counter_value = 1;
*/
public Builder setCounterValue(long value) {
bitField0_ |= 0x00000001;
counterValue_ = value;
onChanged();
return this;
}
/**
* optional sint64 counter_value = 1;
*/
public Builder clearCounterValue() {
bitField0_ = (bitField0_ & ~0x00000001);
counterValue_ = 0L;
onChanged();
return this;
}
private java.util.List setValue_ = java.util.Collections.emptyList();
private void ensureSetValueIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
setValue_ = new java.util.ArrayList(setValue_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated bytes set_value = 2;
*/
public java.util.List
getSetValueList() {
return java.util.Collections.unmodifiableList(setValue_);
}
/**
* repeated bytes set_value = 2;
*/
public int getSetValueCount() {
return setValue_.size();
}
/**
* repeated bytes set_value = 2;
*/
public com.google.protobuf.ByteString getSetValue(int index) {
return setValue_.get(index);
}
/**
* repeated bytes set_value = 2;
*/
public Builder setSetValue(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSetValueIsMutable();
setValue_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes set_value = 2;
*/
public Builder addSetValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSetValueIsMutable();
setValue_.add(value);
onChanged();
return this;
}
/**
* repeated bytes set_value = 2;
*/
public Builder addAllSetValue(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureSetValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, setValue_);
onChanged();
return this;
}
/**
* repeated bytes set_value = 2;
*/
public Builder clearSetValue() {
setValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private java.util.List mapValue_ =
java.util.Collections.emptyList();
private void ensureMapValueIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
mapValue_ = new java.util.ArrayList(mapValue_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder> mapValueBuilder_;
/**
* repeated .MapEntry map_value = 3;
*/
public java.util.List getMapValueList() {
if (mapValueBuilder_ == null) {
return java.util.Collections.unmodifiableList(mapValue_);
} else {
return mapValueBuilder_.getMessageList();
}
}
/**
* repeated .MapEntry map_value = 3;
*/
public int getMapValueCount() {
if (mapValueBuilder_ == null) {
return mapValue_.size();
} else {
return mapValueBuilder_.getCount();
}
}
/**
* repeated .MapEntry map_value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index) {
if (mapValueBuilder_ == null) {
return mapValue_.get(index);
} else {
return mapValueBuilder_.getMessage(index);
}
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder setMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.set(index, value);
onChanged();
} else {
mapValueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder setMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.set(index, builderForValue.build());
onChanged();
} else {
mapValueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder addMapValue(com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.add(value);
onChanged();
} else {
mapValueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder addMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.add(index, value);
onChanged();
} else {
mapValueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder addMapValue(
com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.add(builderForValue.build());
onChanged();
} else {
mapValueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder addMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.add(index, builderForValue.build());
onChanged();
} else {
mapValueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder addAllMapValue(
java.lang.Iterable extends com.basho.riak.protobuf.RiakDtPB.MapEntry> values) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, mapValue_);
onChanged();
} else {
mapValueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder clearMapValue() {
if (mapValueBuilder_ == null) {
mapValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
mapValueBuilder_.clear();
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public Builder removeMapValue(int index) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.remove(index);
onChanged();
} else {
mapValueBuilder_.remove(index);
}
return this;
}
/**
* repeated .MapEntry map_value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder getMapValueBuilder(
int index) {
return getMapValueFieldBuilder().getBuilder(index);
}
/**
* repeated .MapEntry map_value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index) {
if (mapValueBuilder_ == null) {
return mapValue_.get(index); } else {
return mapValueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .MapEntry map_value = 3;
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList() {
if (mapValueBuilder_ != null) {
return mapValueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(mapValue_);
}
}
/**
* repeated .MapEntry map_value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder addMapValueBuilder() {
return getMapValueFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakDtPB.MapEntry.getDefaultInstance());
}
/**
* repeated .MapEntry map_value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder addMapValueBuilder(
int index) {
return getMapValueFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakDtPB.MapEntry.getDefaultInstance());
}
/**
* repeated .MapEntry map_value = 3;
*/
public java.util.List
getMapValueBuilderList() {
return getMapValueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueFieldBuilder() {
if (mapValueBuilder_ == null) {
mapValueBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>(
mapValue_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
mapValue_ = null;
}
return mapValueBuilder_;
}
private long hllValue_ ;
/**
* optional uint64 hll_value = 4;
*
*
* We return an estimated cardinality of the Hyperloglog set
* on fetch.
*
*/
public boolean hasHllValue() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint64 hll_value = 4;
*
*
* We return an estimated cardinality of the Hyperloglog set
* on fetch.
*
*/
public long getHllValue() {
return hllValue_;
}
/**
* optional uint64 hll_value = 4;
*
*
* We return an estimated cardinality of the Hyperloglog set
* on fetch.
*
*/
public Builder setHllValue(long value) {
bitField0_ |= 0x00000008;
hllValue_ = value;
onChanged();
return this;
}
/**
* optional uint64 hll_value = 4;
*
*
* We return an estimated cardinality of the Hyperloglog set
* on fetch.
*
*/
public Builder clearHllValue() {
bitField0_ = (bitField0_ & ~0x00000008);
hllValue_ = 0L;
onChanged();
return this;
}
private java.util.List gsetValue_ = java.util.Collections.emptyList();
private void ensureGsetValueIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
gsetValue_ = new java.util.ArrayList(gsetValue_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated bytes gset_value = 5;
*/
public java.util.List
getGsetValueList() {
return java.util.Collections.unmodifiableList(gsetValue_);
}
/**
* repeated bytes gset_value = 5;
*/
public int getGsetValueCount() {
return gsetValue_.size();
}
/**
* repeated bytes gset_value = 5;
*/
public com.google.protobuf.ByteString getGsetValue(int index) {
return gsetValue_.get(index);
}
/**
* repeated bytes gset_value = 5;
*/
public Builder setGsetValue(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureGsetValueIsMutable();
gsetValue_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes gset_value = 5;
*/
public Builder addGsetValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureGsetValueIsMutable();
gsetValue_.add(value);
onChanged();
return this;
}
/**
* repeated bytes gset_value = 5;
*/
public Builder addAllGsetValue(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureGsetValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, gsetValue_);
onChanged();
return this;
}
/**
* repeated bytes gset_value = 5;
*/
public Builder clearGsetValue() {
gsetValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:DtValue)
}
static {
defaultInstance = new DtValue(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:DtValue)
}
public interface DtFetchRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:DtFetchResp)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes context = 1;
*/
boolean hasContext();
/**
* optional bytes context = 1;
*/
com.google.protobuf.ByteString getContext();
/**
* required .DtFetchResp.DataType type = 2;
*/
boolean hasType();
/**
* required .DtFetchResp.DataType type = 2;
*/
com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType getType();
/**
* optional .DtValue value = 3;
*/
boolean hasValue();
/**
* optional .DtValue value = 3;
*/
com.basho.riak.protobuf.RiakDtPB.DtValue getValue();
/**
* optional .DtValue value = 3;
*/
com.basho.riak.protobuf.RiakDtPB.DtValueOrBuilder getValueOrBuilder();
}
/**
* Protobuf type {@code DtFetchResp}
*
*
* The response to a "Fetch" request. If the `include_context` option
* is specified, an opaque "context" value will be returned along with
* the user-friendly data. When sending an "Update" request, the
* client should send this context as well, similar to how one would
* send a vclock for KV updates. The `type` field indicates which
* value type to expect. When the `value` field is missing from the
* message, the client should interpret it as a "not found".
*
*/
public static final class DtFetchResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:DtFetchResp)
DtFetchRespOrBuilder {
// Use DtFetchResp.newBuilder() to construct.
private DtFetchResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private DtFetchResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final DtFetchResp defaultInstance;
public static DtFetchResp getDefaultInstance() {
return defaultInstance;
}
public DtFetchResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DtFetchResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
context_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType value = com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
type_ = value;
}
break;
}
case 26: {
com.basho.riak.protobuf.RiakDtPB.DtValue.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = value_.toBuilder();
}
value_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.DtValue.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(value_);
value_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtFetchResp.class, com.basho.riak.protobuf.RiakDtPB.DtFetchResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public DtFetchResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DtFetchResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code DtFetchResp.DataType}
*/
public enum DataType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* COUNTER = 1;
*/
COUNTER(0, 1),
/**
* SET = 2;
*/
SET(1, 2),
/**
* MAP = 3;
*/
MAP(2, 3),
/**
* HLL = 4;
*/
HLL(3, 4),
/**
* GSET = 5;
*/
GSET(4, 5),
;
/**
* COUNTER = 1;
*/
public static final int COUNTER_VALUE = 1;
/**
* SET = 2;
*/
public static final int SET_VALUE = 2;
/**
* MAP = 3;
*/
public static final int MAP_VALUE = 3;
/**
* HLL = 4;
*/
public static final int HLL_VALUE = 4;
/**
* GSET = 5;
*/
public static final int GSET_VALUE = 5;
public final int getNumber() { return value; }
public static DataType valueOf(int value) {
switch (value) {
case 1: return COUNTER;
case 2: return SET;
case 3: return MAP;
case 4: return HLL;
case 5: return GSET;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DataType findValueByNumber(int number) {
return DataType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.DtFetchResp.getDescriptor().getEnumTypes().get(0);
}
private static final DataType[] VALUES = values();
public static DataType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private DataType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:DtFetchResp.DataType)
}
private int bitField0_;
public static final int CONTEXT_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString context_;
/**
* optional bytes context = 1;
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes context = 1;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
public static final int TYPE_FIELD_NUMBER = 2;
private com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType type_;
/**
* required .DtFetchResp.DataType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .DtFetchResp.DataType type = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType getType() {
return type_;
}
public static final int VALUE_FIELD_NUMBER = 3;
private com.basho.riak.protobuf.RiakDtPB.DtValue value_;
/**
* optional .DtValue value = 3;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .DtValue value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.DtValue getValue() {
return value_;
}
/**
* optional .DtValue value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.DtValueOrBuilder getValueOrBuilder() {
return value_;
}
private void initFields() {
context_ = com.google.protobuf.ByteString.EMPTY;
type_ = com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType.COUNTER;
value_ = com.basho.riak.protobuf.RiakDtPB.DtValue.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (hasValue()) {
if (!getValue().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, context_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, type_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, value_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, context_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtFetchResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.DtFetchResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code DtFetchResp}
*
*
* The response to a "Fetch" request. If the `include_context` option
* is specified, an opaque "context" value will be returned along with
* the user-friendly data. When sending an "Update" request, the
* client should send this context as well, similar to how one would
* send a vclock for KV updates. The `type` field indicates which
* value type to expect. When the `value` field is missing from the
* message, the client should interpret it as a "not found".
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:DtFetchResp)
com.basho.riak.protobuf.RiakDtPB.DtFetchRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtFetchResp.class, com.basho.riak.protobuf.RiakDtPB.DtFetchResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.DtFetchResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
context_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
type_ = com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType.COUNTER;
bitField0_ = (bitField0_ & ~0x00000002);
if (valueBuilder_ == null) {
value_ = com.basho.riak.protobuf.RiakDtPB.DtValue.getDefaultInstance();
} else {
valueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtFetchResp_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.DtFetchResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.DtFetchResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.DtFetchResp build() {
com.basho.riak.protobuf.RiakDtPB.DtFetchResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.DtFetchResp buildPartial() {
com.basho.riak.protobuf.RiakDtPB.DtFetchResp result = new com.basho.riak.protobuf.RiakDtPB.DtFetchResp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.context_ = context_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (valueBuilder_ == null) {
result.value_ = value_;
} else {
result.value_ = valueBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.DtFetchResp) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.DtFetchResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.DtFetchResp other) {
if (other == com.basho.riak.protobuf.RiakDtPB.DtFetchResp.getDefaultInstance()) return this;
if (other.hasContext()) {
setContext(other.getContext());
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasType()) {
return false;
}
if (hasValue()) {
if (!getValue().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.DtFetchResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.DtFetchResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString context_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes context = 1;
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes context = 1;
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
/**
* optional bytes context = 1;
*/
public Builder setContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
context_ = value;
onChanged();
return this;
}
/**
* optional bytes context = 1;
*/
public Builder clearContext() {
bitField0_ = (bitField0_ & ~0x00000001);
context_ = getDefaultInstance().getContext();
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType type_ = com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType.COUNTER;
/**
* required .DtFetchResp.DataType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .DtFetchResp.DataType type = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType getType() {
return type_;
}
/**
* required .DtFetchResp.DataType type = 2;
*/
public Builder setType(com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* required .DtFetchResp.DataType type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = com.basho.riak.protobuf.RiakDtPB.DtFetchResp.DataType.COUNTER;
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakDtPB.DtValue value_ = com.basho.riak.protobuf.RiakDtPB.DtValue.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.DtValue, com.basho.riak.protobuf.RiakDtPB.DtValue.Builder, com.basho.riak.protobuf.RiakDtPB.DtValueOrBuilder> valueBuilder_;
/**
* optional .DtValue value = 3;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .DtValue value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.DtValue getValue() {
if (valueBuilder_ == null) {
return value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
* optional .DtValue value = 3;
*/
public Builder setValue(com.basho.riak.protobuf.RiakDtPB.DtValue value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
} else {
valueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .DtValue value = 3;
*/
public Builder setValue(
com.basho.riak.protobuf.RiakDtPB.DtValue.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
onChanged();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .DtValue value = 3;
*/
public Builder mergeValue(com.basho.riak.protobuf.RiakDtPB.DtValue value) {
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
value_ != com.basho.riak.protobuf.RiakDtPB.DtValue.getDefaultInstance()) {
value_ =
com.basho.riak.protobuf.RiakDtPB.DtValue.newBuilder(value_).mergeFrom(value).buildPartial();
} else {
value_ = value;
}
onChanged();
} else {
valueBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .DtValue value = 3;
*/
public Builder clearValue() {
if (valueBuilder_ == null) {
value_ = com.basho.riak.protobuf.RiakDtPB.DtValue.getDefaultInstance();
onChanged();
} else {
valueBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .DtValue value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.DtValue.Builder getValueBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
* optional .DtValue value = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.DtValueOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_;
}
}
/**
* optional .DtValue value = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.DtValue, com.basho.riak.protobuf.RiakDtPB.DtValue.Builder, com.basho.riak.protobuf.RiakDtPB.DtValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.DtValue, com.basho.riak.protobuf.RiakDtPB.DtValue.Builder, com.basho.riak.protobuf.RiakDtPB.DtValueOrBuilder>(
getValue(),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
// @@protoc_insertion_point(builder_scope:DtFetchResp)
}
static {
defaultInstance = new DtFetchResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:DtFetchResp)
}
public interface CounterOpOrBuilder extends
// @@protoc_insertion_point(interface_extends:CounterOp)
com.google.protobuf.MessageOrBuilder {
/**
* optional sint64 increment = 1;
*/
boolean hasIncrement();
/**
* optional sint64 increment = 1;
*/
long getIncrement();
}
/**
* Protobuf type {@code CounterOp}
*
*
* An operation to update a Counter, either on its own or inside a
* Map. The `increment` field can be positive or negative. When absent,
* the meaning is an increment by 1.
*
*/
public static final class CounterOp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:CounterOp)
CounterOpOrBuilder {
// Use CounterOp.newBuilder() to construct.
private CounterOp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private CounterOp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final CounterOp defaultInstance;
public static CounterOp getDefaultInstance() {
return defaultInstance;
}
public CounterOp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CounterOp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
increment_ = input.readSInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_CounterOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_CounterOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.CounterOp.class, com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CounterOp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CounterOp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int INCREMENT_FIELD_NUMBER = 1;
private long increment_;
/**
* optional sint64 increment = 1;
*/
public boolean hasIncrement() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional sint64 increment = 1;
*/
public long getIncrement() {
return increment_;
}
private void initFields() {
increment_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeSInt64(1, increment_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, increment_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.CounterOp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.CounterOp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code CounterOp}
*
*
* An operation to update a Counter, either on its own or inside a
* Map. The `increment` field can be positive or negative. When absent,
* the meaning is an increment by 1.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:CounterOp)
com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_CounterOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_CounterOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.CounterOp.class, com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.CounterOp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
increment_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_CounterOp_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.CounterOp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.CounterOp build() {
com.basho.riak.protobuf.RiakDtPB.CounterOp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.CounterOp buildPartial() {
com.basho.riak.protobuf.RiakDtPB.CounterOp result = new com.basho.riak.protobuf.RiakDtPB.CounterOp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.increment_ = increment_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.CounterOp) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.CounterOp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.CounterOp other) {
if (other == com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance()) return this;
if (other.hasIncrement()) {
setIncrement(other.getIncrement());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.CounterOp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.CounterOp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long increment_ ;
/**
* optional sint64 increment = 1;
*/
public boolean hasIncrement() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional sint64 increment = 1;
*/
public long getIncrement() {
return increment_;
}
/**
* optional sint64 increment = 1;
*/
public Builder setIncrement(long value) {
bitField0_ |= 0x00000001;
increment_ = value;
onChanged();
return this;
}
/**
* optional sint64 increment = 1;
*/
public Builder clearIncrement() {
bitField0_ = (bitField0_ & ~0x00000001);
increment_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:CounterOp)
}
static {
defaultInstance = new CounterOp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:CounterOp)
}
public interface SetOpOrBuilder extends
// @@protoc_insertion_point(interface_extends:SetOp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated bytes adds = 1;
*/
java.util.List getAddsList();
/**
* repeated bytes adds = 1;
*/
int getAddsCount();
/**
* repeated bytes adds = 1;
*/
com.google.protobuf.ByteString getAdds(int index);
/**
* repeated bytes removes = 2;
*/
java.util.List getRemovesList();
/**
* repeated bytes removes = 2;
*/
int getRemovesCount();
/**
* repeated bytes removes = 2;
*/
com.google.protobuf.ByteString getRemoves(int index);
}
/**
* Protobuf type {@code SetOp}
*
*
* An operation to update a Set, either on its own or inside a Map.
* Set members are opaque binary values, you can only add or remove
* them from a Set.
*
*/
public static final class SetOp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:SetOp)
SetOpOrBuilder {
// Use SetOp.newBuilder() to construct.
private SetOp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SetOp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SetOp defaultInstance;
public static SetOp getDefaultInstance() {
return defaultInstance;
}
public SetOp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SetOp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
adds_.add(input.readBytes());
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
removes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
removes_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = java.util.Collections.unmodifiableList(adds_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
removes_ = java.util.Collections.unmodifiableList(removes_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_SetOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_SetOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.SetOp.class, com.basho.riak.protobuf.RiakDtPB.SetOp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SetOp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SetOp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int ADDS_FIELD_NUMBER = 1;
private java.util.List adds_;
/**
* repeated bytes adds = 1;
*/
public java.util.List
getAddsList() {
return adds_;
}
/**
* repeated bytes adds = 1;
*/
public int getAddsCount() {
return adds_.size();
}
/**
* repeated bytes adds = 1;
*/
public com.google.protobuf.ByteString getAdds(int index) {
return adds_.get(index);
}
public static final int REMOVES_FIELD_NUMBER = 2;
private java.util.List removes_;
/**
* repeated bytes removes = 2;
*/
public java.util.List
getRemovesList() {
return removes_;
}
/**
* repeated bytes removes = 2;
*/
public int getRemovesCount() {
return removes_.size();
}
/**
* repeated bytes removes = 2;
*/
public com.google.protobuf.ByteString getRemoves(int index) {
return removes_.get(index);
}
private void initFields() {
adds_ = java.util.Collections.emptyList();
removes_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < adds_.size(); i++) {
output.writeBytes(1, adds_.get(i));
}
for (int i = 0; i < removes_.size(); i++) {
output.writeBytes(2, removes_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < adds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(adds_.get(i));
}
size += dataSize;
size += 1 * getAddsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < removes_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(removes_.get(i));
}
size += dataSize;
size += 1 * getRemovesList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.SetOp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.SetOp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code SetOp}
*
*
* An operation to update a Set, either on its own or inside a Map.
* Set members are opaque binary values, you can only add or remove
* them from a Set.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:SetOp)
com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_SetOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_SetOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.SetOp.class, com.basho.riak.protobuf.RiakDtPB.SetOp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.SetOp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
adds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
removes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_SetOp_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.SetOp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.SetOp build() {
com.basho.riak.protobuf.RiakDtPB.SetOp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.SetOp buildPartial() {
com.basho.riak.protobuf.RiakDtPB.SetOp result = new com.basho.riak.protobuf.RiakDtPB.SetOp(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = java.util.Collections.unmodifiableList(adds_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.adds_ = adds_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
removes_ = java.util.Collections.unmodifiableList(removes_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.removes_ = removes_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.SetOp) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.SetOp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.SetOp other) {
if (other == com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance()) return this;
if (!other.adds_.isEmpty()) {
if (adds_.isEmpty()) {
adds_ = other.adds_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAddsIsMutable();
adds_.addAll(other.adds_);
}
onChanged();
}
if (!other.removes_.isEmpty()) {
if (removes_.isEmpty()) {
removes_ = other.removes_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureRemovesIsMutable();
removes_.addAll(other.removes_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.SetOp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.SetOp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List adds_ = java.util.Collections.emptyList();
private void ensureAddsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = new java.util.ArrayList(adds_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes adds = 1;
*/
public java.util.List
getAddsList() {
return java.util.Collections.unmodifiableList(adds_);
}
/**
* repeated bytes adds = 1;
*/
public int getAddsCount() {
return adds_.size();
}
/**
* repeated bytes adds = 1;
*/
public com.google.protobuf.ByteString getAdds(int index) {
return adds_.get(index);
}
/**
* repeated bytes adds = 1;
*/
public Builder setAdds(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAddsIsMutable();
adds_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder addAdds(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAddsIsMutable();
adds_.add(value);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder addAllAdds(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureAddsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, adds_);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder clearAdds() {
adds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private java.util.List removes_ = java.util.Collections.emptyList();
private void ensureRemovesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
removes_ = new java.util.ArrayList(removes_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated bytes removes = 2;
*/
public java.util.List
getRemovesList() {
return java.util.Collections.unmodifiableList(removes_);
}
/**
* repeated bytes removes = 2;
*/
public int getRemovesCount() {
return removes_.size();
}
/**
* repeated bytes removes = 2;
*/
public com.google.protobuf.ByteString getRemoves(int index) {
return removes_.get(index);
}
/**
* repeated bytes removes = 2;
*/
public Builder setRemoves(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRemovesIsMutable();
removes_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes removes = 2;
*/
public Builder addRemoves(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRemovesIsMutable();
removes_.add(value);
onChanged();
return this;
}
/**
* repeated bytes removes = 2;
*/
public Builder addAllRemoves(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureRemovesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, removes_);
onChanged();
return this;
}
/**
* repeated bytes removes = 2;
*/
public Builder clearRemoves() {
removes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:SetOp)
}
static {
defaultInstance = new SetOp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:SetOp)
}
public interface GSetOpOrBuilder extends
// @@protoc_insertion_point(interface_extends:GSetOp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated bytes adds = 1;
*/
java.util.List getAddsList();
/**
* repeated bytes adds = 1;
*/
int getAddsCount();
/**
* repeated bytes adds = 1;
*/
com.google.protobuf.ByteString getAdds(int index);
}
/**
* Protobuf type {@code GSetOp}
*
*
* An operation to update a GSet, on its own.
* GSet members are opaque binary values, you can only add
* them to a Set.
*
*/
public static final class GSetOp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:GSetOp)
GSetOpOrBuilder {
// Use GSetOp.newBuilder() to construct.
private GSetOp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private GSetOp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final GSetOp defaultInstance;
public static GSetOp getDefaultInstance() {
return defaultInstance;
}
public GSetOp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GSetOp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
adds_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = java.util.Collections.unmodifiableList(adds_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_GSetOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_GSetOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.GSetOp.class, com.basho.riak.protobuf.RiakDtPB.GSetOp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public GSetOp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GSetOp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int ADDS_FIELD_NUMBER = 1;
private java.util.List adds_;
/**
* repeated bytes adds = 1;
*/
public java.util.List
getAddsList() {
return adds_;
}
/**
* repeated bytes adds = 1;
*/
public int getAddsCount() {
return adds_.size();
}
/**
* repeated bytes adds = 1;
*/
public com.google.protobuf.ByteString getAdds(int index) {
return adds_.get(index);
}
private void initFields() {
adds_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < adds_.size(); i++) {
output.writeBytes(1, adds_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < adds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(adds_.get(i));
}
size += dataSize;
size += 1 * getAddsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.GSetOp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.GSetOp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code GSetOp}
*
*
* An operation to update a GSet, on its own.
* GSet members are opaque binary values, you can only add
* them to a Set.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:GSetOp)
com.basho.riak.protobuf.RiakDtPB.GSetOpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_GSetOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_GSetOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.GSetOp.class, com.basho.riak.protobuf.RiakDtPB.GSetOp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.GSetOp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
adds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_GSetOp_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.GSetOp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.GSetOp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.GSetOp build() {
com.basho.riak.protobuf.RiakDtPB.GSetOp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.GSetOp buildPartial() {
com.basho.riak.protobuf.RiakDtPB.GSetOp result = new com.basho.riak.protobuf.RiakDtPB.GSetOp(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = java.util.Collections.unmodifiableList(adds_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.adds_ = adds_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.GSetOp) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.GSetOp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.GSetOp other) {
if (other == com.basho.riak.protobuf.RiakDtPB.GSetOp.getDefaultInstance()) return this;
if (!other.adds_.isEmpty()) {
if (adds_.isEmpty()) {
adds_ = other.adds_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAddsIsMutable();
adds_.addAll(other.adds_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.GSetOp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.GSetOp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List adds_ = java.util.Collections.emptyList();
private void ensureAddsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = new java.util.ArrayList(adds_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes adds = 1;
*/
public java.util.List
getAddsList() {
return java.util.Collections.unmodifiableList(adds_);
}
/**
* repeated bytes adds = 1;
*/
public int getAddsCount() {
return adds_.size();
}
/**
* repeated bytes adds = 1;
*/
public com.google.protobuf.ByteString getAdds(int index) {
return adds_.get(index);
}
/**
* repeated bytes adds = 1;
*/
public Builder setAdds(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAddsIsMutable();
adds_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder addAdds(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAddsIsMutable();
adds_.add(value);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder addAllAdds(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureAddsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, adds_);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder clearAdds() {
adds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:GSetOp)
}
static {
defaultInstance = new GSetOp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:GSetOp)
}
public interface HllOpOrBuilder extends
// @@protoc_insertion_point(interface_extends:HllOp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated bytes adds = 1;
*/
java.util.List getAddsList();
/**
* repeated bytes adds = 1;
*/
int getAddsCount();
/**
* repeated bytes adds = 1;
*/
com.google.protobuf.ByteString getAdds(int index);
}
/**
* Protobuf type {@code HllOp}
*
*
* An operation to update a Hyperloglog Set, a top-level DT.
* You can only add to a HllSet.
*
*/
public static final class HllOp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:HllOp)
HllOpOrBuilder {
// Use HllOp.newBuilder() to construct.
private HllOp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private HllOp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final HllOp defaultInstance;
public static HllOp getDefaultInstance() {
return defaultInstance;
}
public HllOp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HllOp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
adds_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = java.util.Collections.unmodifiableList(adds_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_HllOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_HllOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.HllOp.class, com.basho.riak.protobuf.RiakDtPB.HllOp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public HllOp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HllOp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int ADDS_FIELD_NUMBER = 1;
private java.util.List adds_;
/**
* repeated bytes adds = 1;
*/
public java.util.List
getAddsList() {
return adds_;
}
/**
* repeated bytes adds = 1;
*/
public int getAddsCount() {
return adds_.size();
}
/**
* repeated bytes adds = 1;
*/
public com.google.protobuf.ByteString getAdds(int index) {
return adds_.get(index);
}
private void initFields() {
adds_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < adds_.size(); i++) {
output.writeBytes(1, adds_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < adds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(adds_.get(i));
}
size += dataSize;
size += 1 * getAddsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.HllOp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.HllOp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code HllOp}
*
*
* An operation to update a Hyperloglog Set, a top-level DT.
* You can only add to a HllSet.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:HllOp)
com.basho.riak.protobuf.RiakDtPB.HllOpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_HllOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_HllOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.HllOp.class, com.basho.riak.protobuf.RiakDtPB.HllOp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.HllOp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
adds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_HllOp_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.HllOp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.HllOp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.HllOp build() {
com.basho.riak.protobuf.RiakDtPB.HllOp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.HllOp buildPartial() {
com.basho.riak.protobuf.RiakDtPB.HllOp result = new com.basho.riak.protobuf.RiakDtPB.HllOp(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = java.util.Collections.unmodifiableList(adds_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.adds_ = adds_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.HllOp) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.HllOp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.HllOp other) {
if (other == com.basho.riak.protobuf.RiakDtPB.HllOp.getDefaultInstance()) return this;
if (!other.adds_.isEmpty()) {
if (adds_.isEmpty()) {
adds_ = other.adds_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAddsIsMutable();
adds_.addAll(other.adds_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.HllOp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.HllOp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List adds_ = java.util.Collections.emptyList();
private void ensureAddsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
adds_ = new java.util.ArrayList(adds_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes adds = 1;
*/
public java.util.List
getAddsList() {
return java.util.Collections.unmodifiableList(adds_);
}
/**
* repeated bytes adds = 1;
*/
public int getAddsCount() {
return adds_.size();
}
/**
* repeated bytes adds = 1;
*/
public com.google.protobuf.ByteString getAdds(int index) {
return adds_.get(index);
}
/**
* repeated bytes adds = 1;
*/
public Builder setAdds(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAddsIsMutable();
adds_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder addAdds(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAddsIsMutable();
adds_.add(value);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder addAllAdds(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureAddsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, adds_);
onChanged();
return this;
}
/**
* repeated bytes adds = 1;
*/
public Builder clearAdds() {
adds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:HllOp)
}
static {
defaultInstance = new HllOp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:HllOp)
}
public interface MapUpdateOrBuilder extends
// @@protoc_insertion_point(interface_extends:MapUpdate)
com.google.protobuf.MessageOrBuilder {
/**
* required .MapField field = 1;
*/
boolean hasField();
/**
* required .MapField field = 1;
*/
com.basho.riak.protobuf.RiakDtPB.MapField getField();
/**
* required .MapField field = 1;
*/
com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getFieldOrBuilder();
/**
* optional .CounterOp counter_op = 2;
*/
boolean hasCounterOp();
/**
* optional .CounterOp counter_op = 2;
*/
com.basho.riak.protobuf.RiakDtPB.CounterOp getCounterOp();
/**
* optional .CounterOp counter_op = 2;
*/
com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder getCounterOpOrBuilder();
/**
* optional .SetOp set_op = 3;
*/
boolean hasSetOp();
/**
* optional .SetOp set_op = 3;
*/
com.basho.riak.protobuf.RiakDtPB.SetOp getSetOp();
/**
* optional .SetOp set_op = 3;
*/
com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder getSetOpOrBuilder();
/**
* optional bytes register_op = 4;
*
*
* There is only one operation on a register, which is to set its
* value, therefore the "operation" is the new value.
*
*/
boolean hasRegisterOp();
/**
* optional bytes register_op = 4;
*
*
* There is only one operation on a register, which is to set its
* value, therefore the "operation" is the new value.
*
*/
com.google.protobuf.ByteString getRegisterOp();
/**
* optional .MapUpdate.FlagOp flag_op = 5;
*/
boolean hasFlagOp();
/**
* optional .MapUpdate.FlagOp flag_op = 5;
*/
com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp getFlagOp();
/**
* optional .MapOp map_op = 6;
*/
boolean hasMapOp();
/**
* optional .MapOp map_op = 6;
*/
com.basho.riak.protobuf.RiakDtPB.MapOp getMapOp();
/**
* optional .MapOp map_op = 6;
*/
com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder getMapOpOrBuilder();
}
/**
* Protobuf type {@code MapUpdate}
*
*
* An operation to be applied to a value stored in a Map -- the
* contents of an UPDATE operation. The operation field that is
* present depends on the type of the field to which it is applied.
*
*/
public static final class MapUpdate extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:MapUpdate)
MapUpdateOrBuilder {
// Use MapUpdate.newBuilder() to construct.
private MapUpdate(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private MapUpdate(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final MapUpdate defaultInstance;
public static MapUpdate getDefaultInstance() {
return defaultInstance;
}
public MapUpdate getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MapUpdate(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.basho.riak.protobuf.RiakDtPB.MapField.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = field_.toBuilder();
}
field_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapField.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(field_);
field_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = counterOp_.toBuilder();
}
counterOp_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.CounterOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(counterOp_);
counterOp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.basho.riak.protobuf.RiakDtPB.SetOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = setOp_.toBuilder();
}
setOp_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.SetOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(setOp_);
setOp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
bitField0_ |= 0x00000008;
registerOp_ = input.readBytes();
break;
}
case 40: {
int rawValue = input.readEnum();
com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp value = com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(5, rawValue);
} else {
bitField0_ |= 0x00000010;
flagOp_ = value;
}
break;
}
case 50: {
com.basho.riak.protobuf.RiakDtPB.MapOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = mapOp_.toBuilder();
}
mapOp_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(mapOp_);
mapOp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapUpdate_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapUpdate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.MapUpdate.class, com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MapUpdate parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MapUpdate(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code MapUpdate.FlagOp}
*
*
* Flags only exist inside Maps and can only be enabled or
* disabled, and there are no arguments to the operations.
*
*/
public enum FlagOp
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ENABLE = 1;
*/
ENABLE(0, 1),
/**
* DISABLE = 2;
*/
DISABLE(1, 2),
;
/**
* ENABLE = 1;
*/
public static final int ENABLE_VALUE = 1;
/**
* DISABLE = 2;
*/
public static final int DISABLE_VALUE = 2;
public final int getNumber() { return value; }
public static FlagOp valueOf(int value) {
switch (value) {
case 1: return ENABLE;
case 2: return DISABLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public FlagOp findValueByNumber(int number) {
return FlagOp.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.MapUpdate.getDescriptor().getEnumTypes().get(0);
}
private static final FlagOp[] VALUES = values();
public static FlagOp valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private FlagOp(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:MapUpdate.FlagOp)
}
private int bitField0_;
public static final int FIELD_FIELD_NUMBER = 1;
private com.basho.riak.protobuf.RiakDtPB.MapField field_;
/**
* required .MapField field = 1;
*/
public boolean hasField() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapField getField() {
return field_;
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getFieldOrBuilder() {
return field_;
}
public static final int COUNTER_OP_FIELD_NUMBER = 2;
private com.basho.riak.protobuf.RiakDtPB.CounterOp counterOp_;
/**
* optional .CounterOp counter_op = 2;
*/
public boolean hasCounterOp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .CounterOp counter_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOp getCounterOp() {
return counterOp_;
}
/**
* optional .CounterOp counter_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder getCounterOpOrBuilder() {
return counterOp_;
}
public static final int SET_OP_FIELD_NUMBER = 3;
private com.basho.riak.protobuf.RiakDtPB.SetOp setOp_;
/**
* optional .SetOp set_op = 3;
*/
public boolean hasSetOp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .SetOp set_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOp getSetOp() {
return setOp_;
}
/**
* optional .SetOp set_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder getSetOpOrBuilder() {
return setOp_;
}
public static final int REGISTER_OP_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString registerOp_;
/**
* optional bytes register_op = 4;
*
*
* There is only one operation on a register, which is to set its
* value, therefore the "operation" is the new value.
*
*/
public boolean hasRegisterOp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes register_op = 4;
*
*
* There is only one operation on a register, which is to set its
* value, therefore the "operation" is the new value.
*
*/
public com.google.protobuf.ByteString getRegisterOp() {
return registerOp_;
}
public static final int FLAG_OP_FIELD_NUMBER = 5;
private com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp flagOp_;
/**
* optional .MapUpdate.FlagOp flag_op = 5;
*/
public boolean hasFlagOp() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .MapUpdate.FlagOp flag_op = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp getFlagOp() {
return flagOp_;
}
public static final int MAP_OP_FIELD_NUMBER = 6;
private com.basho.riak.protobuf.RiakDtPB.MapOp mapOp_;
/**
* optional .MapOp map_op = 6;
*/
public boolean hasMapOp() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .MapOp map_op = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOp getMapOp() {
return mapOp_;
}
/**
* optional .MapOp map_op = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder getMapOpOrBuilder() {
return mapOp_;
}
private void initFields() {
field_ = com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
counterOp_ = com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
setOp_ = com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
registerOp_ = com.google.protobuf.ByteString.EMPTY;
flagOp_ = com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp.ENABLE;
mapOp_ = com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasField()) {
memoizedIsInitialized = 0;
return false;
}
if (!getField().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasMapOp()) {
if (!getMapOp().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, field_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, counterOp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, setOp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, registerOp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeEnum(5, flagOp_.getNumber());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(6, mapOp_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, field_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, counterOp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, setOp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, registerOp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, flagOp_.getNumber());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, mapOp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapUpdate parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.MapUpdate prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code MapUpdate}
*
*
* An operation to be applied to a value stored in a Map -- the
* contents of an UPDATE operation. The operation field that is
* present depends on the type of the field to which it is applied.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:MapUpdate)
com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapUpdate_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapUpdate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.MapUpdate.class, com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.MapUpdate.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getFieldFieldBuilder();
getCounterOpFieldBuilder();
getSetOpFieldBuilder();
getMapOpFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (fieldBuilder_ == null) {
field_ = com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
} else {
fieldBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (counterOpBuilder_ == null) {
counterOp_ = com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
} else {
counterOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (setOpBuilder_ == null) {
setOp_ = com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
} else {
setOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
registerOp_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
flagOp_ = com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp.ENABLE;
bitField0_ = (bitField0_ & ~0x00000010);
if (mapOpBuilder_ == null) {
mapOp_ = com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
} else {
mapOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapUpdate_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.MapUpdate getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.MapUpdate.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.MapUpdate build() {
com.basho.riak.protobuf.RiakDtPB.MapUpdate result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.MapUpdate buildPartial() {
com.basho.riak.protobuf.RiakDtPB.MapUpdate result = new com.basho.riak.protobuf.RiakDtPB.MapUpdate(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (fieldBuilder_ == null) {
result.field_ = field_;
} else {
result.field_ = fieldBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (counterOpBuilder_ == null) {
result.counterOp_ = counterOp_;
} else {
result.counterOp_ = counterOpBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (setOpBuilder_ == null) {
result.setOp_ = setOp_;
} else {
result.setOp_ = setOpBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.registerOp_ = registerOp_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.flagOp_ = flagOp_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
if (mapOpBuilder_ == null) {
result.mapOp_ = mapOp_;
} else {
result.mapOp_ = mapOpBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.MapUpdate) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.MapUpdate)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.MapUpdate other) {
if (other == com.basho.riak.protobuf.RiakDtPB.MapUpdate.getDefaultInstance()) return this;
if (other.hasField()) {
mergeField(other.getField());
}
if (other.hasCounterOp()) {
mergeCounterOp(other.getCounterOp());
}
if (other.hasSetOp()) {
mergeSetOp(other.getSetOp());
}
if (other.hasRegisterOp()) {
setRegisterOp(other.getRegisterOp());
}
if (other.hasFlagOp()) {
setFlagOp(other.getFlagOp());
}
if (other.hasMapOp()) {
mergeMapOp(other.getMapOp());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasField()) {
return false;
}
if (!getField().isInitialized()) {
return false;
}
if (hasMapOp()) {
if (!getMapOp().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.MapUpdate parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.MapUpdate) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.basho.riak.protobuf.RiakDtPB.MapField field_ = com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder> fieldBuilder_;
/**
* required .MapField field = 1;
*/
public boolean hasField() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapField getField() {
if (fieldBuilder_ == null) {
return field_;
} else {
return fieldBuilder_.getMessage();
}
}
/**
* required .MapField field = 1;
*/
public Builder setField(com.basho.riak.protobuf.RiakDtPB.MapField value) {
if (fieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
field_ = value;
onChanged();
} else {
fieldBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .MapField field = 1;
*/
public Builder setField(
com.basho.riak.protobuf.RiakDtPB.MapField.Builder builderForValue) {
if (fieldBuilder_ == null) {
field_ = builderForValue.build();
onChanged();
} else {
fieldBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .MapField field = 1;
*/
public Builder mergeField(com.basho.riak.protobuf.RiakDtPB.MapField value) {
if (fieldBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
field_ != com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance()) {
field_ =
com.basho.riak.protobuf.RiakDtPB.MapField.newBuilder(field_).mergeFrom(value).buildPartial();
} else {
field_ = value;
}
onChanged();
} else {
fieldBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .MapField field = 1;
*/
public Builder clearField() {
if (fieldBuilder_ == null) {
field_ = com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance();
onChanged();
} else {
fieldBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapField.Builder getFieldBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getFieldFieldBuilder().getBuilder();
}
/**
* required .MapField field = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getFieldOrBuilder() {
if (fieldBuilder_ != null) {
return fieldBuilder_.getMessageOrBuilder();
} else {
return field_;
}
}
/**
* required .MapField field = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>
getFieldFieldBuilder() {
if (fieldBuilder_ == null) {
fieldBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>(
getField(),
getParentForChildren(),
isClean());
field_ = null;
}
return fieldBuilder_;
}
private com.basho.riak.protobuf.RiakDtPB.CounterOp counterOp_ = com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.CounterOp, com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder, com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder> counterOpBuilder_;
/**
* optional .CounterOp counter_op = 2;
*/
public boolean hasCounterOp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .CounterOp counter_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOp getCounterOp() {
if (counterOpBuilder_ == null) {
return counterOp_;
} else {
return counterOpBuilder_.getMessage();
}
}
/**
* optional .CounterOp counter_op = 2;
*/
public Builder setCounterOp(com.basho.riak.protobuf.RiakDtPB.CounterOp value) {
if (counterOpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
counterOp_ = value;
onChanged();
} else {
counterOpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .CounterOp counter_op = 2;
*/
public Builder setCounterOp(
com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder builderForValue) {
if (counterOpBuilder_ == null) {
counterOp_ = builderForValue.build();
onChanged();
} else {
counterOpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .CounterOp counter_op = 2;
*/
public Builder mergeCounterOp(com.basho.riak.protobuf.RiakDtPB.CounterOp value) {
if (counterOpBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
counterOp_ != com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance()) {
counterOp_ =
com.basho.riak.protobuf.RiakDtPB.CounterOp.newBuilder(counterOp_).mergeFrom(value).buildPartial();
} else {
counterOp_ = value;
}
onChanged();
} else {
counterOpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .CounterOp counter_op = 2;
*/
public Builder clearCounterOp() {
if (counterOpBuilder_ == null) {
counterOp_ = com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
onChanged();
} else {
counterOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .CounterOp counter_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder getCounterOpBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getCounterOpFieldBuilder().getBuilder();
}
/**
* optional .CounterOp counter_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder getCounterOpOrBuilder() {
if (counterOpBuilder_ != null) {
return counterOpBuilder_.getMessageOrBuilder();
} else {
return counterOp_;
}
}
/**
* optional .CounterOp counter_op = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.CounterOp, com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder, com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder>
getCounterOpFieldBuilder() {
if (counterOpBuilder_ == null) {
counterOpBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.CounterOp, com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder, com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder>(
getCounterOp(),
getParentForChildren(),
isClean());
counterOp_ = null;
}
return counterOpBuilder_;
}
private com.basho.riak.protobuf.RiakDtPB.SetOp setOp_ = com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.SetOp, com.basho.riak.protobuf.RiakDtPB.SetOp.Builder, com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder> setOpBuilder_;
/**
* optional .SetOp set_op = 3;
*/
public boolean hasSetOp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .SetOp set_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOp getSetOp() {
if (setOpBuilder_ == null) {
return setOp_;
} else {
return setOpBuilder_.getMessage();
}
}
/**
* optional .SetOp set_op = 3;
*/
public Builder setSetOp(com.basho.riak.protobuf.RiakDtPB.SetOp value) {
if (setOpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
setOp_ = value;
onChanged();
} else {
setOpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .SetOp set_op = 3;
*/
public Builder setSetOp(
com.basho.riak.protobuf.RiakDtPB.SetOp.Builder builderForValue) {
if (setOpBuilder_ == null) {
setOp_ = builderForValue.build();
onChanged();
} else {
setOpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .SetOp set_op = 3;
*/
public Builder mergeSetOp(com.basho.riak.protobuf.RiakDtPB.SetOp value) {
if (setOpBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
setOp_ != com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance()) {
setOp_ =
com.basho.riak.protobuf.RiakDtPB.SetOp.newBuilder(setOp_).mergeFrom(value).buildPartial();
} else {
setOp_ = value;
}
onChanged();
} else {
setOpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .SetOp set_op = 3;
*/
public Builder clearSetOp() {
if (setOpBuilder_ == null) {
setOp_ = com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
onChanged();
} else {
setOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .SetOp set_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOp.Builder getSetOpBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getSetOpFieldBuilder().getBuilder();
}
/**
* optional .SetOp set_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder getSetOpOrBuilder() {
if (setOpBuilder_ != null) {
return setOpBuilder_.getMessageOrBuilder();
} else {
return setOp_;
}
}
/**
* optional .SetOp set_op = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.SetOp, com.basho.riak.protobuf.RiakDtPB.SetOp.Builder, com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder>
getSetOpFieldBuilder() {
if (setOpBuilder_ == null) {
setOpBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.SetOp, com.basho.riak.protobuf.RiakDtPB.SetOp.Builder, com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder>(
getSetOp(),
getParentForChildren(),
isClean());
setOp_ = null;
}
return setOpBuilder_;
}
private com.google.protobuf.ByteString registerOp_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes register_op = 4;
*
*
* There is only one operation on a register, which is to set its
* value, therefore the "operation" is the new value.
*
*/
public boolean hasRegisterOp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes register_op = 4;
*
*
* There is only one operation on a register, which is to set its
* value, therefore the "operation" is the new value.
*
*/
public com.google.protobuf.ByteString getRegisterOp() {
return registerOp_;
}
/**
* optional bytes register_op = 4;
*
*
* There is only one operation on a register, which is to set its
* value, therefore the "operation" is the new value.
*
*/
public Builder setRegisterOp(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
registerOp_ = value;
onChanged();
return this;
}
/**
* optional bytes register_op = 4;
*
*
* There is only one operation on a register, which is to set its
* value, therefore the "operation" is the new value.
*
*/
public Builder clearRegisterOp() {
bitField0_ = (bitField0_ & ~0x00000008);
registerOp_ = getDefaultInstance().getRegisterOp();
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp flagOp_ = com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp.ENABLE;
/**
* optional .MapUpdate.FlagOp flag_op = 5;
*/
public boolean hasFlagOp() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .MapUpdate.FlagOp flag_op = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp getFlagOp() {
return flagOp_;
}
/**
* optional .MapUpdate.FlagOp flag_op = 5;
*/
public Builder setFlagOp(com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
flagOp_ = value;
onChanged();
return this;
}
/**
* optional .MapUpdate.FlagOp flag_op = 5;
*/
public Builder clearFlagOp() {
bitField0_ = (bitField0_ & ~0x00000010);
flagOp_ = com.basho.riak.protobuf.RiakDtPB.MapUpdate.FlagOp.ENABLE;
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakDtPB.MapOp mapOp_ = com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapOp, com.basho.riak.protobuf.RiakDtPB.MapOp.Builder, com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder> mapOpBuilder_;
/**
* optional .MapOp map_op = 6;
*/
public boolean hasMapOp() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .MapOp map_op = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOp getMapOp() {
if (mapOpBuilder_ == null) {
return mapOp_;
} else {
return mapOpBuilder_.getMessage();
}
}
/**
* optional .MapOp map_op = 6;
*/
public Builder setMapOp(com.basho.riak.protobuf.RiakDtPB.MapOp value) {
if (mapOpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
mapOp_ = value;
onChanged();
} else {
mapOpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .MapOp map_op = 6;
*/
public Builder setMapOp(
com.basho.riak.protobuf.RiakDtPB.MapOp.Builder builderForValue) {
if (mapOpBuilder_ == null) {
mapOp_ = builderForValue.build();
onChanged();
} else {
mapOpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .MapOp map_op = 6;
*/
public Builder mergeMapOp(com.basho.riak.protobuf.RiakDtPB.MapOp value) {
if (mapOpBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020) &&
mapOp_ != com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance()) {
mapOp_ =
com.basho.riak.protobuf.RiakDtPB.MapOp.newBuilder(mapOp_).mergeFrom(value).buildPartial();
} else {
mapOp_ = value;
}
onChanged();
} else {
mapOpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
* optional .MapOp map_op = 6;
*/
public Builder clearMapOp() {
if (mapOpBuilder_ == null) {
mapOp_ = com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
onChanged();
} else {
mapOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
* optional .MapOp map_op = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOp.Builder getMapOpBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getMapOpFieldBuilder().getBuilder();
}
/**
* optional .MapOp map_op = 6;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder getMapOpOrBuilder() {
if (mapOpBuilder_ != null) {
return mapOpBuilder_.getMessageOrBuilder();
} else {
return mapOp_;
}
}
/**
* optional .MapOp map_op = 6;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapOp, com.basho.riak.protobuf.RiakDtPB.MapOp.Builder, com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder>
getMapOpFieldBuilder() {
if (mapOpBuilder_ == null) {
mapOpBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapOp, com.basho.riak.protobuf.RiakDtPB.MapOp.Builder, com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder>(
getMapOp(),
getParentForChildren(),
isClean());
mapOp_ = null;
}
return mapOpBuilder_;
}
// @@protoc_insertion_point(builder_scope:MapUpdate)
}
static {
defaultInstance = new MapUpdate(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MapUpdate)
}
public interface MapOpOrBuilder extends
// @@protoc_insertion_point(interface_extends:MapOp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
java.util.List
getRemovesList();
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
com.basho.riak.protobuf.RiakDtPB.MapField getRemoves(int index);
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
int getRemovesCount();
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>
getRemovesOrBuilderList();
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getRemovesOrBuilder(
int index);
/**
* repeated .MapUpdate updates = 2;
*/
java.util.List
getUpdatesList();
/**
* repeated .MapUpdate updates = 2;
*/
com.basho.riak.protobuf.RiakDtPB.MapUpdate getUpdates(int index);
/**
* repeated .MapUpdate updates = 2;
*/
int getUpdatesCount();
/**
* repeated .MapUpdate updates = 2;
*/
java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder>
getUpdatesOrBuilderList();
/**
* repeated .MapUpdate updates = 2;
*/
com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder getUpdatesOrBuilder(
int index);
}
/**
* Protobuf type {@code MapOp}
*
*
* An operation to update a Map. All operations apply to individual
* fields in the Map.
*
*/
public static final class MapOp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:MapOp)
MapOpOrBuilder {
// Use MapOp.newBuilder() to construct.
private MapOp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private MapOp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final MapOp defaultInstance;
public static MapOp getDefaultInstance() {
return defaultInstance;
}
public MapOp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MapOp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
removes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
removes_.add(input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapField.PARSER, extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
updates_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
updates_.add(input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapUpdate.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
removes_ = java.util.Collections.unmodifiableList(removes_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
updates_ = java.util.Collections.unmodifiableList(updates_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.MapOp.class, com.basho.riak.protobuf.RiakDtPB.MapOp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public MapOp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MapOp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int REMOVES_FIELD_NUMBER = 1;
private java.util.List removes_;
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public java.util.List getRemovesList() {
return removes_;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>
getRemovesOrBuilderList() {
return removes_;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public int getRemovesCount() {
return removes_.size();
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public com.basho.riak.protobuf.RiakDtPB.MapField getRemoves(int index) {
return removes_.get(index);
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getRemovesOrBuilder(
int index) {
return removes_.get(index);
}
public static final int UPDATES_FIELD_NUMBER = 2;
private java.util.List updates_;
/**
* repeated .MapUpdate updates = 2;
*/
public java.util.List getUpdatesList() {
return updates_;
}
/**
* repeated .MapUpdate updates = 2;
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder>
getUpdatesOrBuilderList() {
return updates_;
}
/**
* repeated .MapUpdate updates = 2;
*/
public int getUpdatesCount() {
return updates_.size();
}
/**
* repeated .MapUpdate updates = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdate getUpdates(int index) {
return updates_.get(index);
}
/**
* repeated .MapUpdate updates = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder getUpdatesOrBuilder(
int index) {
return updates_.get(index);
}
private void initFields() {
removes_ = java.util.Collections.emptyList();
updates_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getRemovesCount(); i++) {
if (!getRemoves(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getUpdatesCount(); i++) {
if (!getUpdates(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < removes_.size(); i++) {
output.writeMessage(1, removes_.get(i));
}
for (int i = 0; i < updates_.size(); i++) {
output.writeMessage(2, updates_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < removes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, removes_.get(i));
}
for (int i = 0; i < updates_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, updates_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.MapOp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.MapOp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code MapOp}
*
*
* An operation to update a Map. All operations apply to individual
* fields in the Map.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:MapOp)
com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.MapOp.class, com.basho.riak.protobuf.RiakDtPB.MapOp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.MapOp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getRemovesFieldBuilder();
getUpdatesFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (removesBuilder_ == null) {
removes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
removesBuilder_.clear();
}
if (updatesBuilder_ == null) {
updates_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
updatesBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_MapOp_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.MapOp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.MapOp build() {
com.basho.riak.protobuf.RiakDtPB.MapOp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.MapOp buildPartial() {
com.basho.riak.protobuf.RiakDtPB.MapOp result = new com.basho.riak.protobuf.RiakDtPB.MapOp(this);
int from_bitField0_ = bitField0_;
if (removesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
removes_ = java.util.Collections.unmodifiableList(removes_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.removes_ = removes_;
} else {
result.removes_ = removesBuilder_.build();
}
if (updatesBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
updates_ = java.util.Collections.unmodifiableList(updates_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.updates_ = updates_;
} else {
result.updates_ = updatesBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.MapOp) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.MapOp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.MapOp other) {
if (other == com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance()) return this;
if (removesBuilder_ == null) {
if (!other.removes_.isEmpty()) {
if (removes_.isEmpty()) {
removes_ = other.removes_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRemovesIsMutable();
removes_.addAll(other.removes_);
}
onChanged();
}
} else {
if (!other.removes_.isEmpty()) {
if (removesBuilder_.isEmpty()) {
removesBuilder_.dispose();
removesBuilder_ = null;
removes_ = other.removes_;
bitField0_ = (bitField0_ & ~0x00000001);
removesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRemovesFieldBuilder() : null;
} else {
removesBuilder_.addAllMessages(other.removes_);
}
}
}
if (updatesBuilder_ == null) {
if (!other.updates_.isEmpty()) {
if (updates_.isEmpty()) {
updates_ = other.updates_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureUpdatesIsMutable();
updates_.addAll(other.updates_);
}
onChanged();
}
} else {
if (!other.updates_.isEmpty()) {
if (updatesBuilder_.isEmpty()) {
updatesBuilder_.dispose();
updatesBuilder_ = null;
updates_ = other.updates_;
bitField0_ = (bitField0_ & ~0x00000002);
updatesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getUpdatesFieldBuilder() : null;
} else {
updatesBuilder_.addAllMessages(other.updates_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getRemovesCount(); i++) {
if (!getRemoves(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getUpdatesCount(); i++) {
if (!getUpdates(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.MapOp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.MapOp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List removes_ =
java.util.Collections.emptyList();
private void ensureRemovesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
removes_ = new java.util.ArrayList(removes_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder> removesBuilder_;
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public java.util.List getRemovesList() {
if (removesBuilder_ == null) {
return java.util.Collections.unmodifiableList(removes_);
} else {
return removesBuilder_.getMessageList();
}
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public int getRemovesCount() {
if (removesBuilder_ == null) {
return removes_.size();
} else {
return removesBuilder_.getCount();
}
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public com.basho.riak.protobuf.RiakDtPB.MapField getRemoves(int index) {
if (removesBuilder_ == null) {
return removes_.get(index);
} else {
return removesBuilder_.getMessage(index);
}
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder setRemoves(
int index, com.basho.riak.protobuf.RiakDtPB.MapField value) {
if (removesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemovesIsMutable();
removes_.set(index, value);
onChanged();
} else {
removesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder setRemoves(
int index, com.basho.riak.protobuf.RiakDtPB.MapField.Builder builderForValue) {
if (removesBuilder_ == null) {
ensureRemovesIsMutable();
removes_.set(index, builderForValue.build());
onChanged();
} else {
removesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder addRemoves(com.basho.riak.protobuf.RiakDtPB.MapField value) {
if (removesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemovesIsMutable();
removes_.add(value);
onChanged();
} else {
removesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder addRemoves(
int index, com.basho.riak.protobuf.RiakDtPB.MapField value) {
if (removesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRemovesIsMutable();
removes_.add(index, value);
onChanged();
} else {
removesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder addRemoves(
com.basho.riak.protobuf.RiakDtPB.MapField.Builder builderForValue) {
if (removesBuilder_ == null) {
ensureRemovesIsMutable();
removes_.add(builderForValue.build());
onChanged();
} else {
removesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder addRemoves(
int index, com.basho.riak.protobuf.RiakDtPB.MapField.Builder builderForValue) {
if (removesBuilder_ == null) {
ensureRemovesIsMutable();
removes_.add(index, builderForValue.build());
onChanged();
} else {
removesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder addAllRemoves(
java.lang.Iterable extends com.basho.riak.protobuf.RiakDtPB.MapField> values) {
if (removesBuilder_ == null) {
ensureRemovesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, removes_);
onChanged();
} else {
removesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder clearRemoves() {
if (removesBuilder_ == null) {
removes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
removesBuilder_.clear();
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public Builder removeRemoves(int index) {
if (removesBuilder_ == null) {
ensureRemovesIsMutable();
removes_.remove(index);
onChanged();
} else {
removesBuilder_.remove(index);
}
return this;
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public com.basho.riak.protobuf.RiakDtPB.MapField.Builder getRemovesBuilder(
int index) {
return getRemovesFieldBuilder().getBuilder(index);
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder getRemovesOrBuilder(
int index) {
if (removesBuilder_ == null) {
return removes_.get(index); } else {
return removesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>
getRemovesOrBuilderList() {
if (removesBuilder_ != null) {
return removesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(removes_);
}
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public com.basho.riak.protobuf.RiakDtPB.MapField.Builder addRemovesBuilder() {
return getRemovesFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance());
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public com.basho.riak.protobuf.RiakDtPB.MapField.Builder addRemovesBuilder(
int index) {
return getRemovesFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakDtPB.MapField.getDefaultInstance());
}
/**
* repeated .MapField removes = 1;
*
*
* REMOVE removes a field and value from the Map.
* UPDATE applies type-specific
* operations to the values stored in the Map.
*
*/
public java.util.List
getRemovesBuilderList() {
return getRemovesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>
getRemovesFieldBuilder() {
if (removesBuilder_ == null) {
removesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapField, com.basho.riak.protobuf.RiakDtPB.MapField.Builder, com.basho.riak.protobuf.RiakDtPB.MapFieldOrBuilder>(
removes_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
removes_ = null;
}
return removesBuilder_;
}
private java.util.List updates_ =
java.util.Collections.emptyList();
private void ensureUpdatesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
updates_ = new java.util.ArrayList(updates_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapUpdate, com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder, com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder> updatesBuilder_;
/**
* repeated .MapUpdate updates = 2;
*/
public java.util.List getUpdatesList() {
if (updatesBuilder_ == null) {
return java.util.Collections.unmodifiableList(updates_);
} else {
return updatesBuilder_.getMessageList();
}
}
/**
* repeated .MapUpdate updates = 2;
*/
public int getUpdatesCount() {
if (updatesBuilder_ == null) {
return updates_.size();
} else {
return updatesBuilder_.getCount();
}
}
/**
* repeated .MapUpdate updates = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdate getUpdates(int index) {
if (updatesBuilder_ == null) {
return updates_.get(index);
} else {
return updatesBuilder_.getMessage(index);
}
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder setUpdates(
int index, com.basho.riak.protobuf.RiakDtPB.MapUpdate value) {
if (updatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUpdatesIsMutable();
updates_.set(index, value);
onChanged();
} else {
updatesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder setUpdates(
int index, com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder builderForValue) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.set(index, builderForValue.build());
onChanged();
} else {
updatesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder addUpdates(com.basho.riak.protobuf.RiakDtPB.MapUpdate value) {
if (updatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUpdatesIsMutable();
updates_.add(value);
onChanged();
} else {
updatesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder addUpdates(
int index, com.basho.riak.protobuf.RiakDtPB.MapUpdate value) {
if (updatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUpdatesIsMutable();
updates_.add(index, value);
onChanged();
} else {
updatesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder addUpdates(
com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder builderForValue) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.add(builderForValue.build());
onChanged();
} else {
updatesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder addUpdates(
int index, com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder builderForValue) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.add(index, builderForValue.build());
onChanged();
} else {
updatesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder addAllUpdates(
java.lang.Iterable extends com.basho.riak.protobuf.RiakDtPB.MapUpdate> values) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, updates_);
onChanged();
} else {
updatesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder clearUpdates() {
if (updatesBuilder_ == null) {
updates_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
updatesBuilder_.clear();
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public Builder removeUpdates(int index) {
if (updatesBuilder_ == null) {
ensureUpdatesIsMutable();
updates_.remove(index);
onChanged();
} else {
updatesBuilder_.remove(index);
}
return this;
}
/**
* repeated .MapUpdate updates = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder getUpdatesBuilder(
int index) {
return getUpdatesFieldBuilder().getBuilder(index);
}
/**
* repeated .MapUpdate updates = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder getUpdatesOrBuilder(
int index) {
if (updatesBuilder_ == null) {
return updates_.get(index); } else {
return updatesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .MapUpdate updates = 2;
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder>
getUpdatesOrBuilderList() {
if (updatesBuilder_ != null) {
return updatesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(updates_);
}
}
/**
* repeated .MapUpdate updates = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder addUpdatesBuilder() {
return getUpdatesFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakDtPB.MapUpdate.getDefaultInstance());
}
/**
* repeated .MapUpdate updates = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder addUpdatesBuilder(
int index) {
return getUpdatesFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakDtPB.MapUpdate.getDefaultInstance());
}
/**
* repeated .MapUpdate updates = 2;
*/
public java.util.List
getUpdatesBuilderList() {
return getUpdatesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapUpdate, com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder, com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder>
getUpdatesFieldBuilder() {
if (updatesBuilder_ == null) {
updatesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapUpdate, com.basho.riak.protobuf.RiakDtPB.MapUpdate.Builder, com.basho.riak.protobuf.RiakDtPB.MapUpdateOrBuilder>(
updates_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
updates_ = null;
}
return updatesBuilder_;
}
// @@protoc_insertion_point(builder_scope:MapOp)
}
static {
defaultInstance = new MapOp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:MapOp)
}
public interface DtOpOrBuilder extends
// @@protoc_insertion_point(interface_extends:DtOp)
com.google.protobuf.MessageOrBuilder {
/**
* optional .CounterOp counter_op = 1;
*/
boolean hasCounterOp();
/**
* optional .CounterOp counter_op = 1;
*/
com.basho.riak.protobuf.RiakDtPB.CounterOp getCounterOp();
/**
* optional .CounterOp counter_op = 1;
*/
com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder getCounterOpOrBuilder();
/**
* optional .SetOp set_op = 2;
*/
boolean hasSetOp();
/**
* optional .SetOp set_op = 2;
*/
com.basho.riak.protobuf.RiakDtPB.SetOp getSetOp();
/**
* optional .SetOp set_op = 2;
*/
com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder getSetOpOrBuilder();
/**
* optional .MapOp map_op = 3;
*/
boolean hasMapOp();
/**
* optional .MapOp map_op = 3;
*/
com.basho.riak.protobuf.RiakDtPB.MapOp getMapOp();
/**
* optional .MapOp map_op = 3;
*/
com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder getMapOpOrBuilder();
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
boolean hasHllOp();
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
com.basho.riak.protobuf.RiakDtPB.HllOp getHllOp();
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
com.basho.riak.protobuf.RiakDtPB.HllOpOrBuilder getHllOpOrBuilder();
/**
* optional .GSetOp gset_op = 5;
*/
boolean hasGsetOp();
/**
* optional .GSetOp gset_op = 5;
*/
com.basho.riak.protobuf.RiakDtPB.GSetOp getGsetOp();
/**
* optional .GSetOp gset_op = 5;
*/
com.basho.riak.protobuf.RiakDtPB.GSetOpOrBuilder getGsetOpOrBuilder();
}
/**
* Protobuf type {@code DtOp}
*
*
* A "union" type for update operations. The included operation
* depends on the datatype being updated.
*
*/
public static final class DtOp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:DtOp)
DtOpOrBuilder {
// Use DtOp.newBuilder() to construct.
private DtOp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private DtOp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final DtOp defaultInstance;
public static DtOp getDefaultInstance() {
return defaultInstance;
}
public DtOp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DtOp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = counterOp_.toBuilder();
}
counterOp_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.CounterOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(counterOp_);
counterOp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
com.basho.riak.protobuf.RiakDtPB.SetOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = setOp_.toBuilder();
}
setOp_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.SetOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(setOp_);
setOp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.basho.riak.protobuf.RiakDtPB.MapOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = mapOp_.toBuilder();
}
mapOp_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(mapOp_);
mapOp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 34: {
com.basho.riak.protobuf.RiakDtPB.HllOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = hllOp_.toBuilder();
}
hllOp_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.HllOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(hllOp_);
hllOp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 42: {
com.basho.riak.protobuf.RiakDtPB.GSetOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = gsetOp_.toBuilder();
}
gsetOp_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.GSetOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(gsetOp_);
gsetOp_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtOp.class, com.basho.riak.protobuf.RiakDtPB.DtOp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public DtOp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DtOp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int COUNTER_OP_FIELD_NUMBER = 1;
private com.basho.riak.protobuf.RiakDtPB.CounterOp counterOp_;
/**
* optional .CounterOp counter_op = 1;
*/
public boolean hasCounterOp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .CounterOp counter_op = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOp getCounterOp() {
return counterOp_;
}
/**
* optional .CounterOp counter_op = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder getCounterOpOrBuilder() {
return counterOp_;
}
public static final int SET_OP_FIELD_NUMBER = 2;
private com.basho.riak.protobuf.RiakDtPB.SetOp setOp_;
/**
* optional .SetOp set_op = 2;
*/
public boolean hasSetOp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .SetOp set_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOp getSetOp() {
return setOp_;
}
/**
* optional .SetOp set_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder getSetOpOrBuilder() {
return setOp_;
}
public static final int MAP_OP_FIELD_NUMBER = 3;
private com.basho.riak.protobuf.RiakDtPB.MapOp mapOp_;
/**
* optional .MapOp map_op = 3;
*/
public boolean hasMapOp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .MapOp map_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOp getMapOp() {
return mapOp_;
}
/**
* optional .MapOp map_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder getMapOpOrBuilder() {
return mapOp_;
}
public static final int HLL_OP_FIELD_NUMBER = 4;
private com.basho.riak.protobuf.RiakDtPB.HllOp hllOp_;
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public boolean hasHllOp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public com.basho.riak.protobuf.RiakDtPB.HllOp getHllOp() {
return hllOp_;
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public com.basho.riak.protobuf.RiakDtPB.HllOpOrBuilder getHllOpOrBuilder() {
return hllOp_;
}
public static final int GSET_OP_FIELD_NUMBER = 5;
private com.basho.riak.protobuf.RiakDtPB.GSetOp gsetOp_;
/**
* optional .GSetOp gset_op = 5;
*/
public boolean hasGsetOp() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .GSetOp gset_op = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.GSetOp getGsetOp() {
return gsetOp_;
}
/**
* optional .GSetOp gset_op = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.GSetOpOrBuilder getGsetOpOrBuilder() {
return gsetOp_;
}
private void initFields() {
counterOp_ = com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
setOp_ = com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
mapOp_ = com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
hllOp_ = com.basho.riak.protobuf.RiakDtPB.HllOp.getDefaultInstance();
gsetOp_ = com.basho.riak.protobuf.RiakDtPB.GSetOp.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasMapOp()) {
if (!getMapOp().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, counterOp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, setOp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, mapOp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, hllOp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, gsetOp_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, counterOp_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, setOp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, mapOp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, hllOp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, gsetOp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtOp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.DtOp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code DtOp}
*
*
* A "union" type for update operations. The included operation
* depends on the datatype being updated.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:DtOp)
com.basho.riak.protobuf.RiakDtPB.DtOpOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtOp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtOp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtOp.class, com.basho.riak.protobuf.RiakDtPB.DtOp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.DtOp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getCounterOpFieldBuilder();
getSetOpFieldBuilder();
getMapOpFieldBuilder();
getHllOpFieldBuilder();
getGsetOpFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (counterOpBuilder_ == null) {
counterOp_ = com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
} else {
counterOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (setOpBuilder_ == null) {
setOp_ = com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
} else {
setOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (mapOpBuilder_ == null) {
mapOp_ = com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
} else {
mapOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
if (hllOpBuilder_ == null) {
hllOp_ = com.basho.riak.protobuf.RiakDtPB.HllOp.getDefaultInstance();
} else {
hllOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (gsetOpBuilder_ == null) {
gsetOp_ = com.basho.riak.protobuf.RiakDtPB.GSetOp.getDefaultInstance();
} else {
gsetOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtOp_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.DtOp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.DtOp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.DtOp build() {
com.basho.riak.protobuf.RiakDtPB.DtOp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.DtOp buildPartial() {
com.basho.riak.protobuf.RiakDtPB.DtOp result = new com.basho.riak.protobuf.RiakDtPB.DtOp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (counterOpBuilder_ == null) {
result.counterOp_ = counterOp_;
} else {
result.counterOp_ = counterOpBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (setOpBuilder_ == null) {
result.setOp_ = setOp_;
} else {
result.setOp_ = setOpBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (mapOpBuilder_ == null) {
result.mapOp_ = mapOp_;
} else {
result.mapOp_ = mapOpBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (hllOpBuilder_ == null) {
result.hllOp_ = hllOp_;
} else {
result.hllOp_ = hllOpBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (gsetOpBuilder_ == null) {
result.gsetOp_ = gsetOp_;
} else {
result.gsetOp_ = gsetOpBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.DtOp) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.DtOp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.DtOp other) {
if (other == com.basho.riak.protobuf.RiakDtPB.DtOp.getDefaultInstance()) return this;
if (other.hasCounterOp()) {
mergeCounterOp(other.getCounterOp());
}
if (other.hasSetOp()) {
mergeSetOp(other.getSetOp());
}
if (other.hasMapOp()) {
mergeMapOp(other.getMapOp());
}
if (other.hasHllOp()) {
mergeHllOp(other.getHllOp());
}
if (other.hasGsetOp()) {
mergeGsetOp(other.getGsetOp());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (hasMapOp()) {
if (!getMapOp().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.DtOp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.DtOp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.basho.riak.protobuf.RiakDtPB.CounterOp counterOp_ = com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.CounterOp, com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder, com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder> counterOpBuilder_;
/**
* optional .CounterOp counter_op = 1;
*/
public boolean hasCounterOp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .CounterOp counter_op = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOp getCounterOp() {
if (counterOpBuilder_ == null) {
return counterOp_;
} else {
return counterOpBuilder_.getMessage();
}
}
/**
* optional .CounterOp counter_op = 1;
*/
public Builder setCounterOp(com.basho.riak.protobuf.RiakDtPB.CounterOp value) {
if (counterOpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
counterOp_ = value;
onChanged();
} else {
counterOpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .CounterOp counter_op = 1;
*/
public Builder setCounterOp(
com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder builderForValue) {
if (counterOpBuilder_ == null) {
counterOp_ = builderForValue.build();
onChanged();
} else {
counterOpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .CounterOp counter_op = 1;
*/
public Builder mergeCounterOp(com.basho.riak.protobuf.RiakDtPB.CounterOp value) {
if (counterOpBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
counterOp_ != com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance()) {
counterOp_ =
com.basho.riak.protobuf.RiakDtPB.CounterOp.newBuilder(counterOp_).mergeFrom(value).buildPartial();
} else {
counterOp_ = value;
}
onChanged();
} else {
counterOpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .CounterOp counter_op = 1;
*/
public Builder clearCounterOp() {
if (counterOpBuilder_ == null) {
counterOp_ = com.basho.riak.protobuf.RiakDtPB.CounterOp.getDefaultInstance();
onChanged();
} else {
counterOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .CounterOp counter_op = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder getCounterOpBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getCounterOpFieldBuilder().getBuilder();
}
/**
* optional .CounterOp counter_op = 1;
*/
public com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder getCounterOpOrBuilder() {
if (counterOpBuilder_ != null) {
return counterOpBuilder_.getMessageOrBuilder();
} else {
return counterOp_;
}
}
/**
* optional .CounterOp counter_op = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.CounterOp, com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder, com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder>
getCounterOpFieldBuilder() {
if (counterOpBuilder_ == null) {
counterOpBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.CounterOp, com.basho.riak.protobuf.RiakDtPB.CounterOp.Builder, com.basho.riak.protobuf.RiakDtPB.CounterOpOrBuilder>(
getCounterOp(),
getParentForChildren(),
isClean());
counterOp_ = null;
}
return counterOpBuilder_;
}
private com.basho.riak.protobuf.RiakDtPB.SetOp setOp_ = com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.SetOp, com.basho.riak.protobuf.RiakDtPB.SetOp.Builder, com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder> setOpBuilder_;
/**
* optional .SetOp set_op = 2;
*/
public boolean hasSetOp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .SetOp set_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOp getSetOp() {
if (setOpBuilder_ == null) {
return setOp_;
} else {
return setOpBuilder_.getMessage();
}
}
/**
* optional .SetOp set_op = 2;
*/
public Builder setSetOp(com.basho.riak.protobuf.RiakDtPB.SetOp value) {
if (setOpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
setOp_ = value;
onChanged();
} else {
setOpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .SetOp set_op = 2;
*/
public Builder setSetOp(
com.basho.riak.protobuf.RiakDtPB.SetOp.Builder builderForValue) {
if (setOpBuilder_ == null) {
setOp_ = builderForValue.build();
onChanged();
} else {
setOpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .SetOp set_op = 2;
*/
public Builder mergeSetOp(com.basho.riak.protobuf.RiakDtPB.SetOp value) {
if (setOpBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
setOp_ != com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance()) {
setOp_ =
com.basho.riak.protobuf.RiakDtPB.SetOp.newBuilder(setOp_).mergeFrom(value).buildPartial();
} else {
setOp_ = value;
}
onChanged();
} else {
setOpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .SetOp set_op = 2;
*/
public Builder clearSetOp() {
if (setOpBuilder_ == null) {
setOp_ = com.basho.riak.protobuf.RiakDtPB.SetOp.getDefaultInstance();
onChanged();
} else {
setOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .SetOp set_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOp.Builder getSetOpBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getSetOpFieldBuilder().getBuilder();
}
/**
* optional .SetOp set_op = 2;
*/
public com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder getSetOpOrBuilder() {
if (setOpBuilder_ != null) {
return setOpBuilder_.getMessageOrBuilder();
} else {
return setOp_;
}
}
/**
* optional .SetOp set_op = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.SetOp, com.basho.riak.protobuf.RiakDtPB.SetOp.Builder, com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder>
getSetOpFieldBuilder() {
if (setOpBuilder_ == null) {
setOpBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.SetOp, com.basho.riak.protobuf.RiakDtPB.SetOp.Builder, com.basho.riak.protobuf.RiakDtPB.SetOpOrBuilder>(
getSetOp(),
getParentForChildren(),
isClean());
setOp_ = null;
}
return setOpBuilder_;
}
private com.basho.riak.protobuf.RiakDtPB.MapOp mapOp_ = com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapOp, com.basho.riak.protobuf.RiakDtPB.MapOp.Builder, com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder> mapOpBuilder_;
/**
* optional .MapOp map_op = 3;
*/
public boolean hasMapOp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .MapOp map_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOp getMapOp() {
if (mapOpBuilder_ == null) {
return mapOp_;
} else {
return mapOpBuilder_.getMessage();
}
}
/**
* optional .MapOp map_op = 3;
*/
public Builder setMapOp(com.basho.riak.protobuf.RiakDtPB.MapOp value) {
if (mapOpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
mapOp_ = value;
onChanged();
} else {
mapOpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .MapOp map_op = 3;
*/
public Builder setMapOp(
com.basho.riak.protobuf.RiakDtPB.MapOp.Builder builderForValue) {
if (mapOpBuilder_ == null) {
mapOp_ = builderForValue.build();
onChanged();
} else {
mapOpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .MapOp map_op = 3;
*/
public Builder mergeMapOp(com.basho.riak.protobuf.RiakDtPB.MapOp value) {
if (mapOpBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
mapOp_ != com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance()) {
mapOp_ =
com.basho.riak.protobuf.RiakDtPB.MapOp.newBuilder(mapOp_).mergeFrom(value).buildPartial();
} else {
mapOp_ = value;
}
onChanged();
} else {
mapOpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .MapOp map_op = 3;
*/
public Builder clearMapOp() {
if (mapOpBuilder_ == null) {
mapOp_ = com.basho.riak.protobuf.RiakDtPB.MapOp.getDefaultInstance();
onChanged();
} else {
mapOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .MapOp map_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOp.Builder getMapOpBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getMapOpFieldBuilder().getBuilder();
}
/**
* optional .MapOp map_op = 3;
*/
public com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder getMapOpOrBuilder() {
if (mapOpBuilder_ != null) {
return mapOpBuilder_.getMessageOrBuilder();
} else {
return mapOp_;
}
}
/**
* optional .MapOp map_op = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapOp, com.basho.riak.protobuf.RiakDtPB.MapOp.Builder, com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder>
getMapOpFieldBuilder() {
if (mapOpBuilder_ == null) {
mapOpBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapOp, com.basho.riak.protobuf.RiakDtPB.MapOp.Builder, com.basho.riak.protobuf.RiakDtPB.MapOpOrBuilder>(
getMapOp(),
getParentForChildren(),
isClean());
mapOp_ = null;
}
return mapOpBuilder_;
}
private com.basho.riak.protobuf.RiakDtPB.HllOp hllOp_ = com.basho.riak.protobuf.RiakDtPB.HllOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.HllOp, com.basho.riak.protobuf.RiakDtPB.HllOp.Builder, com.basho.riak.protobuf.RiakDtPB.HllOpOrBuilder> hllOpBuilder_;
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public boolean hasHllOp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public com.basho.riak.protobuf.RiakDtPB.HllOp getHllOp() {
if (hllOpBuilder_ == null) {
return hllOp_;
} else {
return hllOpBuilder_.getMessage();
}
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public Builder setHllOp(com.basho.riak.protobuf.RiakDtPB.HllOp value) {
if (hllOpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
hllOp_ = value;
onChanged();
} else {
hllOpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public Builder setHllOp(
com.basho.riak.protobuf.RiakDtPB.HllOp.Builder builderForValue) {
if (hllOpBuilder_ == null) {
hllOp_ = builderForValue.build();
onChanged();
} else {
hllOpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public Builder mergeHllOp(com.basho.riak.protobuf.RiakDtPB.HllOp value) {
if (hllOpBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
hllOp_ != com.basho.riak.protobuf.RiakDtPB.HllOp.getDefaultInstance()) {
hllOp_ =
com.basho.riak.protobuf.RiakDtPB.HllOp.newBuilder(hllOp_).mergeFrom(value).buildPartial();
} else {
hllOp_ = value;
}
onChanged();
} else {
hllOpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public Builder clearHllOp() {
if (hllOpBuilder_ == null) {
hllOp_ = com.basho.riak.protobuf.RiakDtPB.HllOp.getDefaultInstance();
onChanged();
} else {
hllOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public com.basho.riak.protobuf.RiakDtPB.HllOp.Builder getHllOpBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getHllOpFieldBuilder().getBuilder();
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
public com.basho.riak.protobuf.RiakDtPB.HllOpOrBuilder getHllOpOrBuilder() {
if (hllOpBuilder_ != null) {
return hllOpBuilder_.getMessageOrBuilder();
} else {
return hllOp_;
}
}
/**
* optional .HllOp hll_op = 4;
*
*
* Adding values to a hyperloglog (set) is just like adding values
* to a set.
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.HllOp, com.basho.riak.protobuf.RiakDtPB.HllOp.Builder, com.basho.riak.protobuf.RiakDtPB.HllOpOrBuilder>
getHllOpFieldBuilder() {
if (hllOpBuilder_ == null) {
hllOpBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.HllOp, com.basho.riak.protobuf.RiakDtPB.HllOp.Builder, com.basho.riak.protobuf.RiakDtPB.HllOpOrBuilder>(
getHllOp(),
getParentForChildren(),
isClean());
hllOp_ = null;
}
return hllOpBuilder_;
}
private com.basho.riak.protobuf.RiakDtPB.GSetOp gsetOp_ = com.basho.riak.protobuf.RiakDtPB.GSetOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.GSetOp, com.basho.riak.protobuf.RiakDtPB.GSetOp.Builder, com.basho.riak.protobuf.RiakDtPB.GSetOpOrBuilder> gsetOpBuilder_;
/**
* optional .GSetOp gset_op = 5;
*/
public boolean hasGsetOp() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .GSetOp gset_op = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.GSetOp getGsetOp() {
if (gsetOpBuilder_ == null) {
return gsetOp_;
} else {
return gsetOpBuilder_.getMessage();
}
}
/**
* optional .GSetOp gset_op = 5;
*/
public Builder setGsetOp(com.basho.riak.protobuf.RiakDtPB.GSetOp value) {
if (gsetOpBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
gsetOp_ = value;
onChanged();
} else {
gsetOpBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .GSetOp gset_op = 5;
*/
public Builder setGsetOp(
com.basho.riak.protobuf.RiakDtPB.GSetOp.Builder builderForValue) {
if (gsetOpBuilder_ == null) {
gsetOp_ = builderForValue.build();
onChanged();
} else {
gsetOpBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .GSetOp gset_op = 5;
*/
public Builder mergeGsetOp(com.basho.riak.protobuf.RiakDtPB.GSetOp value) {
if (gsetOpBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
gsetOp_ != com.basho.riak.protobuf.RiakDtPB.GSetOp.getDefaultInstance()) {
gsetOp_ =
com.basho.riak.protobuf.RiakDtPB.GSetOp.newBuilder(gsetOp_).mergeFrom(value).buildPartial();
} else {
gsetOp_ = value;
}
onChanged();
} else {
gsetOpBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .GSetOp gset_op = 5;
*/
public Builder clearGsetOp() {
if (gsetOpBuilder_ == null) {
gsetOp_ = com.basho.riak.protobuf.RiakDtPB.GSetOp.getDefaultInstance();
onChanged();
} else {
gsetOpBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .GSetOp gset_op = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.GSetOp.Builder getGsetOpBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getGsetOpFieldBuilder().getBuilder();
}
/**
* optional .GSetOp gset_op = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.GSetOpOrBuilder getGsetOpOrBuilder() {
if (gsetOpBuilder_ != null) {
return gsetOpBuilder_.getMessageOrBuilder();
} else {
return gsetOp_;
}
}
/**
* optional .GSetOp gset_op = 5;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.GSetOp, com.basho.riak.protobuf.RiakDtPB.GSetOp.Builder, com.basho.riak.protobuf.RiakDtPB.GSetOpOrBuilder>
getGsetOpFieldBuilder() {
if (gsetOpBuilder_ == null) {
gsetOpBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.GSetOp, com.basho.riak.protobuf.RiakDtPB.GSetOp.Builder, com.basho.riak.protobuf.RiakDtPB.GSetOpOrBuilder>(
getGsetOp(),
getParentForChildren(),
isClean());
gsetOp_ = null;
}
return gsetOpBuilder_;
}
// @@protoc_insertion_point(builder_scope:DtOp)
}
static {
defaultInstance = new DtOp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:DtOp)
}
public interface DtUpdateReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:DtUpdateReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes bucket = 1;
*
*
* The identifier
*
*/
boolean hasBucket();
/**
* required bytes bucket = 1;
*
*
* The identifier
*
*/
com.google.protobuf.ByteString getBucket();
/**
* optional bytes key = 2;
*
*
* missing key results in server-assigned key, like KV
*
*/
boolean hasKey();
/**
* optional bytes key = 2;
*
*
* missing key results in server-assigned key, like KV
*
*/
com.google.protobuf.ByteString getKey();
/**
* required bytes type = 3;
*
*
* bucket type, not data-type (but the data-type is constrained per bucket-type)
*
*/
boolean hasType();
/**
* required bytes type = 3;
*
*
* bucket type, not data-type (but the data-type is constrained per bucket-type)
*
*/
com.google.protobuf.ByteString getType();
/**
* optional bytes context = 4;
*
*
* Opaque update-context
*
*/
boolean hasContext();
/**
* optional bytes context = 4;
*
*
* Opaque update-context
*
*/
com.google.protobuf.ByteString getContext();
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
boolean hasOp();
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
com.basho.riak.protobuf.RiakDtPB.DtOp getOp();
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
com.basho.riak.protobuf.RiakDtPB.DtOpOrBuilder getOpOrBuilder();
/**
* optional uint32 w = 6;
*
*
* Request options
*
*/
boolean hasW();
/**
* optional uint32 w = 6;
*
*
* Request options
*
*/
int getW();
/**
* optional uint32 dw = 7;
*/
boolean hasDw();
/**
* optional uint32 dw = 7;
*/
int getDw();
/**
* optional uint32 pw = 8;
*/
boolean hasPw();
/**
* optional uint32 pw = 8;
*/
int getPw();
/**
* optional bool return_body = 9 [default = false];
*/
boolean hasReturnBody();
/**
* optional bool return_body = 9 [default = false];
*/
boolean getReturnBody();
/**
* optional uint32 timeout = 10;
*/
boolean hasTimeout();
/**
* optional uint32 timeout = 10;
*/
int getTimeout();
/**
* optional bool sloppy_quorum = 11;
*
*
* Experimental, may change/disappear
*
*/
boolean hasSloppyQuorum();
/**
* optional bool sloppy_quorum = 11;
*
*
* Experimental, may change/disappear
*
*/
boolean getSloppyQuorum();
/**
* optional uint32 n_val = 12;
*
*
* Experimental, may change/disappear
*
*/
boolean hasNVal();
/**
* optional uint32 n_val = 12;
*
*
* Experimental, may change/disappear
*
*/
int getNVal();
/**
* optional bool include_context = 13 [default = true];
*
*
* When return_body is true, should the context be returned too?
*
*/
boolean hasIncludeContext();
/**
* optional bool include_context = 13 [default = true];
*
*
* When return_body is true, should the context be returned too?
*
*/
boolean getIncludeContext();
}
/**
* Protobuf type {@code DtUpdateReq}
*
*
* The equivalent of KV's "RpbPutReq", results in an empty response or
* "DtUpdateResp" if `return_body` is specified, or the key is
* assigned by the server. The request-time options are limited to
* ones that are relevant to structured data-types.
*
*/
public static final class DtUpdateReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:DtUpdateReq)
DtUpdateReqOrBuilder {
// Use DtUpdateReq.newBuilder() to construct.
private DtUpdateReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private DtUpdateReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final DtUpdateReq defaultInstance;
public static DtUpdateReq getDefaultInstance() {
return defaultInstance;
}
public DtUpdateReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DtUpdateReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
bucket_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
key_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
type_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
context_ = input.readBytes();
break;
}
case 42: {
com.basho.riak.protobuf.RiakDtPB.DtOp.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = op_.toBuilder();
}
op_ = input.readMessage(com.basho.riak.protobuf.RiakDtPB.DtOp.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(op_);
op_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 48: {
bitField0_ |= 0x00000020;
w_ = input.readUInt32();
break;
}
case 56: {
bitField0_ |= 0x00000040;
dw_ = input.readUInt32();
break;
}
case 64: {
bitField0_ |= 0x00000080;
pw_ = input.readUInt32();
break;
}
case 72: {
bitField0_ |= 0x00000100;
returnBody_ = input.readBool();
break;
}
case 80: {
bitField0_ |= 0x00000200;
timeout_ = input.readUInt32();
break;
}
case 88: {
bitField0_ |= 0x00000400;
sloppyQuorum_ = input.readBool();
break;
}
case 96: {
bitField0_ |= 0x00000800;
nVal_ = input.readUInt32();
break;
}
case 104: {
bitField0_ |= 0x00001000;
includeContext_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtUpdateReq.class, com.basho.riak.protobuf.RiakDtPB.DtUpdateReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public DtUpdateReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DtUpdateReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int BUCKET_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString bucket_;
/**
* required bytes bucket = 1;
*
*
* The identifier
*
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*
*
* The identifier
*
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
public static final int KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString key_;
/**
* optional bytes key = 2;
*
*
* missing key results in server-assigned key, like KV
*
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes key = 2;
*
*
* missing key results in server-assigned key, like KV
*
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int TYPE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString type_;
/**
* required bytes type = 3;
*
*
* bucket type, not data-type (but the data-type is constrained per bucket-type)
*
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bytes type = 3;
*
*
* bucket type, not data-type (but the data-type is constrained per bucket-type)
*
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
public static final int CONTEXT_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString context_;
/**
* optional bytes context = 4;
*
*
* Opaque update-context
*
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes context = 4;
*
*
* Opaque update-context
*
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
public static final int OP_FIELD_NUMBER = 5;
private com.basho.riak.protobuf.RiakDtPB.DtOp op_;
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public boolean hasOp() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public com.basho.riak.protobuf.RiakDtPB.DtOp getOp() {
return op_;
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public com.basho.riak.protobuf.RiakDtPB.DtOpOrBuilder getOpOrBuilder() {
return op_;
}
public static final int W_FIELD_NUMBER = 6;
private int w_;
/**
* optional uint32 w = 6;
*
*
* Request options
*
*/
public boolean hasW() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional uint32 w = 6;
*
*
* Request options
*
*/
public int getW() {
return w_;
}
public static final int DW_FIELD_NUMBER = 7;
private int dw_;
/**
* optional uint32 dw = 7;
*/
public boolean hasDw() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional uint32 dw = 7;
*/
public int getDw() {
return dw_;
}
public static final int PW_FIELD_NUMBER = 8;
private int pw_;
/**
* optional uint32 pw = 8;
*/
public boolean hasPw() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 pw = 8;
*/
public int getPw() {
return pw_;
}
public static final int RETURN_BODY_FIELD_NUMBER = 9;
private boolean returnBody_;
/**
* optional bool return_body = 9 [default = false];
*/
public boolean hasReturnBody() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool return_body = 9 [default = false];
*/
public boolean getReturnBody() {
return returnBody_;
}
public static final int TIMEOUT_FIELD_NUMBER = 10;
private int timeout_;
/**
* optional uint32 timeout = 10;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional uint32 timeout = 10;
*/
public int getTimeout() {
return timeout_;
}
public static final int SLOPPY_QUORUM_FIELD_NUMBER = 11;
private boolean sloppyQuorum_;
/**
* optional bool sloppy_quorum = 11;
*
*
* Experimental, may change/disappear
*
*/
public boolean hasSloppyQuorum() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool sloppy_quorum = 11;
*
*
* Experimental, may change/disappear
*
*/
public boolean getSloppyQuorum() {
return sloppyQuorum_;
}
public static final int N_VAL_FIELD_NUMBER = 12;
private int nVal_;
/**
* optional uint32 n_val = 12;
*
*
* Experimental, may change/disappear
*
*/
public boolean hasNVal() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional uint32 n_val = 12;
*
*
* Experimental, may change/disappear
*
*/
public int getNVal() {
return nVal_;
}
public static final int INCLUDE_CONTEXT_FIELD_NUMBER = 13;
private boolean includeContext_;
/**
* optional bool include_context = 13 [default = true];
*
*
* When return_body is true, should the context be returned too?
*
*/
public boolean hasIncludeContext() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool include_context = 13 [default = true];
*
*
* When return_body is true, should the context be returned too?
*
*/
public boolean getIncludeContext() {
return includeContext_;
}
private void initFields() {
bucket_ = com.google.protobuf.ByteString.EMPTY;
key_ = com.google.protobuf.ByteString.EMPTY;
type_ = com.google.protobuf.ByteString.EMPTY;
context_ = com.google.protobuf.ByteString.EMPTY;
op_ = com.basho.riak.protobuf.RiakDtPB.DtOp.getDefaultInstance();
w_ = 0;
dw_ = 0;
pw_ = 0;
returnBody_ = false;
timeout_ = 0;
sloppyQuorum_ = false;
nVal_ = 0;
includeContext_ = true;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasBucket()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasOp()) {
memoizedIsInitialized = 0;
return false;
}
if (!getOp().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, key_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, type_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, context_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, op_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeUInt32(6, w_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeUInt32(7, dw_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeUInt32(8, pw_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(9, returnBody_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeUInt32(10, timeout_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBool(11, sloppyQuorum_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeUInt32(12, nVal_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBool(13, includeContext_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, key_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, type_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, context_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, op_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(6, w_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(7, dw_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(8, pw_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, returnBody_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(10, timeout_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, sloppyQuorum_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(12, nVal_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, includeContext_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.DtUpdateReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code DtUpdateReq}
*
*
* The equivalent of KV's "RpbPutReq", results in an empty response or
* "DtUpdateResp" if `return_body` is specified, or the key is
* assigned by the server. The request-time options are limited to
* ones that are relevant to structured data-types.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:DtUpdateReq)
com.basho.riak.protobuf.RiakDtPB.DtUpdateReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtUpdateReq.class, com.basho.riak.protobuf.RiakDtPB.DtUpdateReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.DtUpdateReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getOpFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
bucket_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
key_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
type_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
context_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
if (opBuilder_ == null) {
op_ = com.basho.riak.protobuf.RiakDtPB.DtOp.getDefaultInstance();
} else {
opBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
w_ = 0;
bitField0_ = (bitField0_ & ~0x00000020);
dw_ = 0;
bitField0_ = (bitField0_ & ~0x00000040);
pw_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
returnBody_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
timeout_ = 0;
bitField0_ = (bitField0_ & ~0x00000200);
sloppyQuorum_ = false;
bitField0_ = (bitField0_ & ~0x00000400);
nVal_ = 0;
bitField0_ = (bitField0_ & ~0x00000800);
includeContext_ = true;
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateReq_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.DtUpdateReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.DtUpdateReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.DtUpdateReq build() {
com.basho.riak.protobuf.RiakDtPB.DtUpdateReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.DtUpdateReq buildPartial() {
com.basho.riak.protobuf.RiakDtPB.DtUpdateReq result = new com.basho.riak.protobuf.RiakDtPB.DtUpdateReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.bucket_ = bucket_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.context_ = context_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (opBuilder_ == null) {
result.op_ = op_;
} else {
result.op_ = opBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.w_ = w_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.dw_ = dw_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.pw_ = pw_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.returnBody_ = returnBody_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.timeout_ = timeout_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
result.sloppyQuorum_ = sloppyQuorum_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
result.nVal_ = nVal_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00001000;
}
result.includeContext_ = includeContext_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.DtUpdateReq) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.DtUpdateReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.DtUpdateReq other) {
if (other == com.basho.riak.protobuf.RiakDtPB.DtUpdateReq.getDefaultInstance()) return this;
if (other.hasBucket()) {
setBucket(other.getBucket());
}
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasContext()) {
setContext(other.getContext());
}
if (other.hasOp()) {
mergeOp(other.getOp());
}
if (other.hasW()) {
setW(other.getW());
}
if (other.hasDw()) {
setDw(other.getDw());
}
if (other.hasPw()) {
setPw(other.getPw());
}
if (other.hasReturnBody()) {
setReturnBody(other.getReturnBody());
}
if (other.hasTimeout()) {
setTimeout(other.getTimeout());
}
if (other.hasSloppyQuorum()) {
setSloppyQuorum(other.getSloppyQuorum());
}
if (other.hasNVal()) {
setNVal(other.getNVal());
}
if (other.hasIncludeContext()) {
setIncludeContext(other.getIncludeContext());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasBucket()) {
return false;
}
if (!hasType()) {
return false;
}
if (!hasOp()) {
return false;
}
if (!getOp().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.DtUpdateReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.DtUpdateReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString bucket_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes bucket = 1;
*
*
* The identifier
*
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*
*
* The identifier
*
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
/**
* required bytes bucket = 1;
*
*
* The identifier
*
*/
public Builder setBucket(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
bucket_ = value;
onChanged();
return this;
}
/**
* required bytes bucket = 1;
*
*
* The identifier
*
*/
public Builder clearBucket() {
bitField0_ = (bitField0_ & ~0x00000001);
bucket_ = getDefaultInstance().getBucket();
onChanged();
return this;
}
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes key = 2;
*
*
* missing key results in server-assigned key, like KV
*
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes key = 2;
*
*
* missing key results in server-assigned key, like KV
*
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* optional bytes key = 2;
*
*
* missing key results in server-assigned key, like KV
*
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
key_ = value;
onChanged();
return this;
}
/**
* optional bytes key = 2;
*
*
* missing key results in server-assigned key, like KV
*
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000002);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString type_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes type = 3;
*
*
* bucket type, not data-type (but the data-type is constrained per bucket-type)
*
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bytes type = 3;
*
*
* bucket type, not data-type (but the data-type is constrained per bucket-type)
*
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
/**
* required bytes type = 3;
*
*
* bucket type, not data-type (but the data-type is constrained per bucket-type)
*
*/
public Builder setType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value;
onChanged();
return this;
}
/**
* required bytes type = 3;
*
*
* bucket type, not data-type (but the data-type is constrained per bucket-type)
*
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000004);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
private com.google.protobuf.ByteString context_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes context = 4;
*
*
* Opaque update-context
*
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bytes context = 4;
*
*
* Opaque update-context
*
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
/**
* optional bytes context = 4;
*
*
* Opaque update-context
*
*/
public Builder setContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
context_ = value;
onChanged();
return this;
}
/**
* optional bytes context = 4;
*
*
* Opaque update-context
*
*/
public Builder clearContext() {
bitField0_ = (bitField0_ & ~0x00000008);
context_ = getDefaultInstance().getContext();
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakDtPB.DtOp op_ = com.basho.riak.protobuf.RiakDtPB.DtOp.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.DtOp, com.basho.riak.protobuf.RiakDtPB.DtOp.Builder, com.basho.riak.protobuf.RiakDtPB.DtOpOrBuilder> opBuilder_;
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public boolean hasOp() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public com.basho.riak.protobuf.RiakDtPB.DtOp getOp() {
if (opBuilder_ == null) {
return op_;
} else {
return opBuilder_.getMessage();
}
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public Builder setOp(com.basho.riak.protobuf.RiakDtPB.DtOp value) {
if (opBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
op_ = value;
onChanged();
} else {
opBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public Builder setOp(
com.basho.riak.protobuf.RiakDtPB.DtOp.Builder builderForValue) {
if (opBuilder_ == null) {
op_ = builderForValue.build();
onChanged();
} else {
opBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public Builder mergeOp(com.basho.riak.protobuf.RiakDtPB.DtOp value) {
if (opBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
op_ != com.basho.riak.protobuf.RiakDtPB.DtOp.getDefaultInstance()) {
op_ =
com.basho.riak.protobuf.RiakDtPB.DtOp.newBuilder(op_).mergeFrom(value).buildPartial();
} else {
op_ = value;
}
onChanged();
} else {
opBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public Builder clearOp() {
if (opBuilder_ == null) {
op_ = com.basho.riak.protobuf.RiakDtPB.DtOp.getDefaultInstance();
onChanged();
} else {
opBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public com.basho.riak.protobuf.RiakDtPB.DtOp.Builder getOpBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getOpFieldBuilder().getBuilder();
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
public com.basho.riak.protobuf.RiakDtPB.DtOpOrBuilder getOpOrBuilder() {
if (opBuilder_ != null) {
return opBuilder_.getMessageOrBuilder();
} else {
return op_;
}
}
/**
* required .DtOp op = 5;
*
*
* The operations
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.DtOp, com.basho.riak.protobuf.RiakDtPB.DtOp.Builder, com.basho.riak.protobuf.RiakDtPB.DtOpOrBuilder>
getOpFieldBuilder() {
if (opBuilder_ == null) {
opBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.DtOp, com.basho.riak.protobuf.RiakDtPB.DtOp.Builder, com.basho.riak.protobuf.RiakDtPB.DtOpOrBuilder>(
getOp(),
getParentForChildren(),
isClean());
op_ = null;
}
return opBuilder_;
}
private int w_ ;
/**
* optional uint32 w = 6;
*
*
* Request options
*
*/
public boolean hasW() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional uint32 w = 6;
*
*
* Request options
*
*/
public int getW() {
return w_;
}
/**
* optional uint32 w = 6;
*
*
* Request options
*
*/
public Builder setW(int value) {
bitField0_ |= 0x00000020;
w_ = value;
onChanged();
return this;
}
/**
* optional uint32 w = 6;
*
*
* Request options
*
*/
public Builder clearW() {
bitField0_ = (bitField0_ & ~0x00000020);
w_ = 0;
onChanged();
return this;
}
private int dw_ ;
/**
* optional uint32 dw = 7;
*/
public boolean hasDw() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional uint32 dw = 7;
*/
public int getDw() {
return dw_;
}
/**
* optional uint32 dw = 7;
*/
public Builder setDw(int value) {
bitField0_ |= 0x00000040;
dw_ = value;
onChanged();
return this;
}
/**
* optional uint32 dw = 7;
*/
public Builder clearDw() {
bitField0_ = (bitField0_ & ~0x00000040);
dw_ = 0;
onChanged();
return this;
}
private int pw_ ;
/**
* optional uint32 pw = 8;
*/
public boolean hasPw() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 pw = 8;
*/
public int getPw() {
return pw_;
}
/**
* optional uint32 pw = 8;
*/
public Builder setPw(int value) {
bitField0_ |= 0x00000080;
pw_ = value;
onChanged();
return this;
}
/**
* optional uint32 pw = 8;
*/
public Builder clearPw() {
bitField0_ = (bitField0_ & ~0x00000080);
pw_ = 0;
onChanged();
return this;
}
private boolean returnBody_ ;
/**
* optional bool return_body = 9 [default = false];
*/
public boolean hasReturnBody() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool return_body = 9 [default = false];
*/
public boolean getReturnBody() {
return returnBody_;
}
/**
* optional bool return_body = 9 [default = false];
*/
public Builder setReturnBody(boolean value) {
bitField0_ |= 0x00000100;
returnBody_ = value;
onChanged();
return this;
}
/**
* optional bool return_body = 9 [default = false];
*/
public Builder clearReturnBody() {
bitField0_ = (bitField0_ & ~0x00000100);
returnBody_ = false;
onChanged();
return this;
}
private int timeout_ ;
/**
* optional uint32 timeout = 10;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional uint32 timeout = 10;
*/
public int getTimeout() {
return timeout_;
}
/**
* optional uint32 timeout = 10;
*/
public Builder setTimeout(int value) {
bitField0_ |= 0x00000200;
timeout_ = value;
onChanged();
return this;
}
/**
* optional uint32 timeout = 10;
*/
public Builder clearTimeout() {
bitField0_ = (bitField0_ & ~0x00000200);
timeout_ = 0;
onChanged();
return this;
}
private boolean sloppyQuorum_ ;
/**
* optional bool sloppy_quorum = 11;
*
*
* Experimental, may change/disappear
*
*/
public boolean hasSloppyQuorum() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool sloppy_quorum = 11;
*
*
* Experimental, may change/disappear
*
*/
public boolean getSloppyQuorum() {
return sloppyQuorum_;
}
/**
* optional bool sloppy_quorum = 11;
*
*
* Experimental, may change/disappear
*
*/
public Builder setSloppyQuorum(boolean value) {
bitField0_ |= 0x00000400;
sloppyQuorum_ = value;
onChanged();
return this;
}
/**
* optional bool sloppy_quorum = 11;
*
*
* Experimental, may change/disappear
*
*/
public Builder clearSloppyQuorum() {
bitField0_ = (bitField0_ & ~0x00000400);
sloppyQuorum_ = false;
onChanged();
return this;
}
private int nVal_ ;
/**
* optional uint32 n_val = 12;
*
*
* Experimental, may change/disappear
*
*/
public boolean hasNVal() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional uint32 n_val = 12;
*
*
* Experimental, may change/disappear
*
*/
public int getNVal() {
return nVal_;
}
/**
* optional uint32 n_val = 12;
*
*
* Experimental, may change/disappear
*
*/
public Builder setNVal(int value) {
bitField0_ |= 0x00000800;
nVal_ = value;
onChanged();
return this;
}
/**
* optional uint32 n_val = 12;
*
*
* Experimental, may change/disappear
*
*/
public Builder clearNVal() {
bitField0_ = (bitField0_ & ~0x00000800);
nVal_ = 0;
onChanged();
return this;
}
private boolean includeContext_ = true;
/**
* optional bool include_context = 13 [default = true];
*
*
* When return_body is true, should the context be returned too?
*
*/
public boolean hasIncludeContext() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool include_context = 13 [default = true];
*
*
* When return_body is true, should the context be returned too?
*
*/
public boolean getIncludeContext() {
return includeContext_;
}
/**
* optional bool include_context = 13 [default = true];
*
*
* When return_body is true, should the context be returned too?
*
*/
public Builder setIncludeContext(boolean value) {
bitField0_ |= 0x00001000;
includeContext_ = value;
onChanged();
return this;
}
/**
* optional bool include_context = 13 [default = true];
*
*
* When return_body is true, should the context be returned too?
*
*/
public Builder clearIncludeContext() {
bitField0_ = (bitField0_ & ~0x00001000);
includeContext_ = true;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:DtUpdateReq)
}
static {
defaultInstance = new DtUpdateReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:DtUpdateReq)
}
public interface DtUpdateRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:DtUpdateResp)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes key = 1;
*
*
* The key, if assigned by the server
*
*/
boolean hasKey();
/**
* optional bytes key = 1;
*
*
* The key, if assigned by the server
*
*/
com.google.protobuf.ByteString getKey();
/**
* optional bytes context = 2;
*
*
* The opaque update context and value, if return_body was set.
*
*/
boolean hasContext();
/**
* optional bytes context = 2;
*
*
* The opaque update context and value, if return_body was set.
*
*/
com.google.protobuf.ByteString getContext();
/**
* optional sint64 counter_value = 3;
*/
boolean hasCounterValue();
/**
* optional sint64 counter_value = 3;
*/
long getCounterValue();
/**
* repeated bytes set_value = 4;
*/
java.util.List getSetValueList();
/**
* repeated bytes set_value = 4;
*/
int getSetValueCount();
/**
* repeated bytes set_value = 4;
*/
com.google.protobuf.ByteString getSetValue(int index);
/**
* repeated .MapEntry map_value = 5;
*/
java.util.List
getMapValueList();
/**
* repeated .MapEntry map_value = 5;
*/
com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index);
/**
* repeated .MapEntry map_value = 5;
*/
int getMapValueCount();
/**
* repeated .MapEntry map_value = 5;
*/
java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList();
/**
* repeated .MapEntry map_value = 5;
*/
com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index);
/**
* optional uint64 hll_value = 6;
*/
boolean hasHllValue();
/**
* optional uint64 hll_value = 6;
*/
long getHllValue();
/**
* repeated bytes gset_value = 7;
*/
java.util.List getGsetValueList();
/**
* repeated bytes gset_value = 7;
*/
int getGsetValueCount();
/**
* repeated bytes gset_value = 7;
*/
com.google.protobuf.ByteString getGsetValue(int index);
}
/**
* Protobuf type {@code DtUpdateResp}
*
*
* The equivalent of KV's "RpbPutResp", contains the assigned key if
* it was assigned by the server, and the resulting value and context
* if return_body was set.
*
*/
public static final class DtUpdateResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:DtUpdateResp)
DtUpdateRespOrBuilder {
// Use DtUpdateResp.newBuilder() to construct.
private DtUpdateResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private DtUpdateResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final DtUpdateResp defaultInstance;
public static DtUpdateResp getDefaultInstance() {
return defaultInstance;
}
public DtUpdateResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DtUpdateResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
key_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
context_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
counterValue_ = input.readSInt64();
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
setValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
setValue_.add(input.readBytes());
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
mapValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
mapValue_.add(input.readMessage(com.basho.riak.protobuf.RiakDtPB.MapEntry.PARSER, extensionRegistry));
break;
}
case 48: {
bitField0_ |= 0x00000008;
hllValue_ = input.readUInt64();
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
gsetValue_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
gsetValue_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
setValue_ = java.util.Collections.unmodifiableList(setValue_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
mapValue_ = java.util.Collections.unmodifiableList(mapValue_);
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
gsetValue_ = java.util.Collections.unmodifiableList(gsetValue_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtUpdateResp.class, com.basho.riak.protobuf.RiakDtPB.DtUpdateResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public DtUpdateResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DtUpdateResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString key_;
/**
* optional bytes key = 1;
*
*
* The key, if assigned by the server
*
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes key = 1;
*
*
* The key, if assigned by the server
*
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int CONTEXT_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString context_;
/**
* optional bytes context = 2;
*
*
* The opaque update context and value, if return_body was set.
*
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes context = 2;
*
*
* The opaque update context and value, if return_body was set.
*
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
public static final int COUNTER_VALUE_FIELD_NUMBER = 3;
private long counterValue_;
/**
* optional sint64 counter_value = 3;
*/
public boolean hasCounterValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional sint64 counter_value = 3;
*/
public long getCounterValue() {
return counterValue_;
}
public static final int SET_VALUE_FIELD_NUMBER = 4;
private java.util.List setValue_;
/**
* repeated bytes set_value = 4;
*/
public java.util.List
getSetValueList() {
return setValue_;
}
/**
* repeated bytes set_value = 4;
*/
public int getSetValueCount() {
return setValue_.size();
}
/**
* repeated bytes set_value = 4;
*/
public com.google.protobuf.ByteString getSetValue(int index) {
return setValue_.get(index);
}
public static final int MAP_VALUE_FIELD_NUMBER = 5;
private java.util.List mapValue_;
/**
* repeated .MapEntry map_value = 5;
*/
public java.util.List getMapValueList() {
return mapValue_;
}
/**
* repeated .MapEntry map_value = 5;
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList() {
return mapValue_;
}
/**
* repeated .MapEntry map_value = 5;
*/
public int getMapValueCount() {
return mapValue_.size();
}
/**
* repeated .MapEntry map_value = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index) {
return mapValue_.get(index);
}
/**
* repeated .MapEntry map_value = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index) {
return mapValue_.get(index);
}
public static final int HLL_VALUE_FIELD_NUMBER = 6;
private long hllValue_;
/**
* optional uint64 hll_value = 6;
*/
public boolean hasHllValue() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint64 hll_value = 6;
*/
public long getHllValue() {
return hllValue_;
}
public static final int GSET_VALUE_FIELD_NUMBER = 7;
private java.util.List gsetValue_;
/**
* repeated bytes gset_value = 7;
*/
public java.util.List
getGsetValueList() {
return gsetValue_;
}
/**
* repeated bytes gset_value = 7;
*/
public int getGsetValueCount() {
return gsetValue_.size();
}
/**
* repeated bytes gset_value = 7;
*/
public com.google.protobuf.ByteString getGsetValue(int index) {
return gsetValue_.get(index);
}
private void initFields() {
key_ = com.google.protobuf.ByteString.EMPTY;
context_ = com.google.protobuf.ByteString.EMPTY;
counterValue_ = 0L;
setValue_ = java.util.Collections.emptyList();
mapValue_ = java.util.Collections.emptyList();
hllValue_ = 0L;
gsetValue_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getMapValueCount(); i++) {
if (!getMapValue(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, context_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeSInt64(3, counterValue_);
}
for (int i = 0; i < setValue_.size(); i++) {
output.writeBytes(4, setValue_.get(i));
}
for (int i = 0; i < mapValue_.size(); i++) {
output.writeMessage(5, mapValue_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt64(6, hllValue_);
}
for (int i = 0; i < gsetValue_.size(); i++) {
output.writeBytes(7, gsetValue_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, context_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(3, counterValue_);
}
{
int dataSize = 0;
for (int i = 0; i < setValue_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(setValue_.get(i));
}
size += dataSize;
size += 1 * getSetValueList().size();
}
for (int i = 0; i < mapValue_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, mapValue_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, hllValue_);
}
{
int dataSize = 0;
for (int i = 0; i < gsetValue_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(gsetValue_.get(i));
}
size += dataSize;
size += 1 * getGsetValueList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakDtPB.DtUpdateResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code DtUpdateResp}
*
*
* The equivalent of KV's "RpbPutResp", contains the assigned key if
* it was assigned by the server, and the resulting value and context
* if return_body was set.
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:DtUpdateResp)
com.basho.riak.protobuf.RiakDtPB.DtUpdateRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakDtPB.DtUpdateResp.class, com.basho.riak.protobuf.RiakDtPB.DtUpdateResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakDtPB.DtUpdateResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getMapValueFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
key_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
context_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
counterValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
setValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
if (mapValueBuilder_ == null) {
mapValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
mapValueBuilder_.clear();
}
hllValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
gsetValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakDtPB.internal_static_DtUpdateResp_descriptor;
}
public com.basho.riak.protobuf.RiakDtPB.DtUpdateResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakDtPB.DtUpdateResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakDtPB.DtUpdateResp build() {
com.basho.riak.protobuf.RiakDtPB.DtUpdateResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakDtPB.DtUpdateResp buildPartial() {
com.basho.riak.protobuf.RiakDtPB.DtUpdateResp result = new com.basho.riak.protobuf.RiakDtPB.DtUpdateResp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.context_ = context_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.counterValue_ = counterValue_;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
setValue_ = java.util.Collections.unmodifiableList(setValue_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.setValue_ = setValue_;
if (mapValueBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
mapValue_ = java.util.Collections.unmodifiableList(mapValue_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.mapValue_ = mapValue_;
} else {
result.mapValue_ = mapValueBuilder_.build();
}
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000008;
}
result.hllValue_ = hllValue_;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
gsetValue_ = java.util.Collections.unmodifiableList(gsetValue_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.gsetValue_ = gsetValue_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakDtPB.DtUpdateResp) {
return mergeFrom((com.basho.riak.protobuf.RiakDtPB.DtUpdateResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakDtPB.DtUpdateResp other) {
if (other == com.basho.riak.protobuf.RiakDtPB.DtUpdateResp.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasContext()) {
setContext(other.getContext());
}
if (other.hasCounterValue()) {
setCounterValue(other.getCounterValue());
}
if (!other.setValue_.isEmpty()) {
if (setValue_.isEmpty()) {
setValue_ = other.setValue_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureSetValueIsMutable();
setValue_.addAll(other.setValue_);
}
onChanged();
}
if (mapValueBuilder_ == null) {
if (!other.mapValue_.isEmpty()) {
if (mapValue_.isEmpty()) {
mapValue_ = other.mapValue_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureMapValueIsMutable();
mapValue_.addAll(other.mapValue_);
}
onChanged();
}
} else {
if (!other.mapValue_.isEmpty()) {
if (mapValueBuilder_.isEmpty()) {
mapValueBuilder_.dispose();
mapValueBuilder_ = null;
mapValue_ = other.mapValue_;
bitField0_ = (bitField0_ & ~0x00000010);
mapValueBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getMapValueFieldBuilder() : null;
} else {
mapValueBuilder_.addAllMessages(other.mapValue_);
}
}
}
if (other.hasHllValue()) {
setHllValue(other.getHllValue());
}
if (!other.gsetValue_.isEmpty()) {
if (gsetValue_.isEmpty()) {
gsetValue_ = other.gsetValue_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureGsetValueIsMutable();
gsetValue_.addAll(other.gsetValue_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getMapValueCount(); i++) {
if (!getMapValue(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakDtPB.DtUpdateResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakDtPB.DtUpdateResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes key = 1;
*
*
* The key, if assigned by the server
*
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes key = 1;
*
*
* The key, if assigned by the server
*
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* optional bytes key = 1;
*
*
* The key, if assigned by the server
*
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* optional bytes key = 1;
*
*
* The key, if assigned by the server
*
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString context_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes context = 2;
*
*
* The opaque update context and value, if return_body was set.
*
*/
public boolean hasContext() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes context = 2;
*
*
* The opaque update context and value, if return_body was set.
*
*/
public com.google.protobuf.ByteString getContext() {
return context_;
}
/**
* optional bytes context = 2;
*
*
* The opaque update context and value, if return_body was set.
*
*/
public Builder setContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
context_ = value;
onChanged();
return this;
}
/**
* optional bytes context = 2;
*
*
* The opaque update context and value, if return_body was set.
*
*/
public Builder clearContext() {
bitField0_ = (bitField0_ & ~0x00000002);
context_ = getDefaultInstance().getContext();
onChanged();
return this;
}
private long counterValue_ ;
/**
* optional sint64 counter_value = 3;
*/
public boolean hasCounterValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional sint64 counter_value = 3;
*/
public long getCounterValue() {
return counterValue_;
}
/**
* optional sint64 counter_value = 3;
*/
public Builder setCounterValue(long value) {
bitField0_ |= 0x00000004;
counterValue_ = value;
onChanged();
return this;
}
/**
* optional sint64 counter_value = 3;
*/
public Builder clearCounterValue() {
bitField0_ = (bitField0_ & ~0x00000004);
counterValue_ = 0L;
onChanged();
return this;
}
private java.util.List setValue_ = java.util.Collections.emptyList();
private void ensureSetValueIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
setValue_ = new java.util.ArrayList(setValue_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated bytes set_value = 4;
*/
public java.util.List
getSetValueList() {
return java.util.Collections.unmodifiableList(setValue_);
}
/**
* repeated bytes set_value = 4;
*/
public int getSetValueCount() {
return setValue_.size();
}
/**
* repeated bytes set_value = 4;
*/
public com.google.protobuf.ByteString getSetValue(int index) {
return setValue_.get(index);
}
/**
* repeated bytes set_value = 4;
*/
public Builder setSetValue(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSetValueIsMutable();
setValue_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes set_value = 4;
*/
public Builder addSetValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSetValueIsMutable();
setValue_.add(value);
onChanged();
return this;
}
/**
* repeated bytes set_value = 4;
*/
public Builder addAllSetValue(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureSetValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, setValue_);
onChanged();
return this;
}
/**
* repeated bytes set_value = 4;
*/
public Builder clearSetValue() {
setValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
private java.util.List mapValue_ =
java.util.Collections.emptyList();
private void ensureMapValueIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
mapValue_ = new java.util.ArrayList(mapValue_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder> mapValueBuilder_;
/**
* repeated .MapEntry map_value = 5;
*/
public java.util.List getMapValueList() {
if (mapValueBuilder_ == null) {
return java.util.Collections.unmodifiableList(mapValue_);
} else {
return mapValueBuilder_.getMessageList();
}
}
/**
* repeated .MapEntry map_value = 5;
*/
public int getMapValueCount() {
if (mapValueBuilder_ == null) {
return mapValue_.size();
} else {
return mapValueBuilder_.getCount();
}
}
/**
* repeated .MapEntry map_value = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry getMapValue(int index) {
if (mapValueBuilder_ == null) {
return mapValue_.get(index);
} else {
return mapValueBuilder_.getMessage(index);
}
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder setMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.set(index, value);
onChanged();
} else {
mapValueBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder setMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.set(index, builderForValue.build());
onChanged();
} else {
mapValueBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder addMapValue(com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.add(value);
onChanged();
} else {
mapValueBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder addMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry value) {
if (mapValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMapValueIsMutable();
mapValue_.add(index, value);
onChanged();
} else {
mapValueBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder addMapValue(
com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.add(builderForValue.build());
onChanged();
} else {
mapValueBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder addMapValue(
int index, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder builderForValue) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.add(index, builderForValue.build());
onChanged();
} else {
mapValueBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder addAllMapValue(
java.lang.Iterable extends com.basho.riak.protobuf.RiakDtPB.MapEntry> values) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, mapValue_);
onChanged();
} else {
mapValueBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder clearMapValue() {
if (mapValueBuilder_ == null) {
mapValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
mapValueBuilder_.clear();
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public Builder removeMapValue(int index) {
if (mapValueBuilder_ == null) {
ensureMapValueIsMutable();
mapValue_.remove(index);
onChanged();
} else {
mapValueBuilder_.remove(index);
}
return this;
}
/**
* repeated .MapEntry map_value = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder getMapValueBuilder(
int index) {
return getMapValueFieldBuilder().getBuilder(index);
}
/**
* repeated .MapEntry map_value = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder getMapValueOrBuilder(
int index) {
if (mapValueBuilder_ == null) {
return mapValue_.get(index); } else {
return mapValueBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .MapEntry map_value = 5;
*/
public java.util.List extends com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueOrBuilderList() {
if (mapValueBuilder_ != null) {
return mapValueBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(mapValue_);
}
}
/**
* repeated .MapEntry map_value = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder addMapValueBuilder() {
return getMapValueFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakDtPB.MapEntry.getDefaultInstance());
}
/**
* repeated .MapEntry map_value = 5;
*/
public com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder addMapValueBuilder(
int index) {
return getMapValueFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakDtPB.MapEntry.getDefaultInstance());
}
/**
* repeated .MapEntry map_value = 5;
*/
public java.util.List
getMapValueBuilderList() {
return getMapValueFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>
getMapValueFieldBuilder() {
if (mapValueBuilder_ == null) {
mapValueBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakDtPB.MapEntry, com.basho.riak.protobuf.RiakDtPB.MapEntry.Builder, com.basho.riak.protobuf.RiakDtPB.MapEntryOrBuilder>(
mapValue_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
mapValue_ = null;
}
return mapValueBuilder_;
}
private long hllValue_ ;
/**
* optional uint64 hll_value = 6;
*/
public boolean hasHllValue() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional uint64 hll_value = 6;
*/
public long getHllValue() {
return hllValue_;
}
/**
* optional uint64 hll_value = 6;
*/
public Builder setHllValue(long value) {
bitField0_ |= 0x00000020;
hllValue_ = value;
onChanged();
return this;
}
/**
* optional uint64 hll_value = 6;
*/
public Builder clearHllValue() {
bitField0_ = (bitField0_ & ~0x00000020);
hllValue_ = 0L;
onChanged();
return this;
}
private java.util.List gsetValue_ = java.util.Collections.emptyList();
private void ensureGsetValueIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
gsetValue_ = new java.util.ArrayList(gsetValue_);
bitField0_ |= 0x00000040;
}
}
/**
* repeated bytes gset_value = 7;
*/
public java.util.List
getGsetValueList() {
return java.util.Collections.unmodifiableList(gsetValue_);
}
/**
* repeated bytes gset_value = 7;
*/
public int getGsetValueCount() {
return gsetValue_.size();
}
/**
* repeated bytes gset_value = 7;
*/
public com.google.protobuf.ByteString getGsetValue(int index) {
return gsetValue_.get(index);
}
/**
* repeated bytes gset_value = 7;
*/
public Builder setGsetValue(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureGsetValueIsMutable();
gsetValue_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes gset_value = 7;
*/
public Builder addGsetValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureGsetValueIsMutable();
gsetValue_.add(value);
onChanged();
return this;
}
/**
* repeated bytes gset_value = 7;
*/
public Builder addAllGsetValue(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureGsetValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, gsetValue_);
onChanged();
return this;
}
/**
* repeated bytes gset_value = 7;
*/
public Builder clearGsetValue() {
gsetValue_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:DtUpdateResp)
}
static {
defaultInstance = new DtUpdateResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:DtUpdateResp)
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_MapField_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_MapField_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_MapEntry_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_MapEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_DtFetchReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_DtFetchReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_DtValue_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_DtValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_DtFetchResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_DtFetchResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_CounterOp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_CounterOp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_SetOp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_SetOp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_GSetOp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_GSetOp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_HllOp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_HllOp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_MapUpdate_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_MapUpdate_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_MapOp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_MapOp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_DtOp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_DtOp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_DtUpdateReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_DtUpdateReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_DtUpdateResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_DtUpdateResp_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\rriak_dt.proto\"\205\001\n\010MapField\022\014\n\004name\030\001 \002" +
"(\014\022$\n\004type\030\002 \002(\0162\026.MapField.MapFieldType" +
"\"E\n\014MapFieldType\022\013\n\007COUNTER\020\001\022\007\n\003SET\020\002\022\014" +
"\n\010REGISTER\020\003\022\010\n\004FLAG\020\004\022\007\n\003MAP\020\005\"\230\001\n\010MapE" +
"ntry\022\030\n\005field\030\001 \002(\0132\t.MapField\022\025\n\rcounte" +
"r_value\030\002 \001(\022\022\021\n\tset_value\030\003 \003(\014\022\026\n\016regi" +
"ster_value\030\004 \001(\014\022\022\n\nflag_value\030\005 \001(\010\022\034\n\t" +
"map_value\030\006 \003(\0132\t.MapEntry\"\317\001\n\nDtFetchRe" +
"q\022\016\n\006bucket\030\001 \002(\014\022\013\n\003key\030\002 \002(\014\022\014\n\004type\030\003" +
" \002(\014\022\t\n\001r\030\004 \001(\r\022\n\n\002pr\030\005 \001(\r\022\024\n\014basic_quo",
"rum\030\006 \001(\010\022\023\n\013notfound_ok\030\007 \001(\010\022\017\n\007timeou" +
"t\030\010 \001(\r\022\025\n\rsloppy_quorum\030\t \001(\010\022\r\n\005n_val\030" +
"\n \001(\r\022\035\n\017include_context\030\013 \001(\010:\004true\"x\n\007" +
"DtValue\022\025\n\rcounter_value\030\001 \001(\022\022\021\n\tset_va" +
"lue\030\002 \003(\014\022\034\n\tmap_value\030\003 \003(\0132\t.MapEntry\022" +
"\021\n\thll_value\030\004 \001(\004\022\022\n\ngset_value\030\005 \003(\014\"\232" +
"\001\n\013DtFetchResp\022\017\n\007context\030\001 \001(\014\022#\n\004type\030" +
"\002 \002(\0162\025.DtFetchResp.DataType\022\027\n\005value\030\003 " +
"\001(\0132\010.DtValue\"<\n\010DataType\022\013\n\007COUNTER\020\001\022\007" +
"\n\003SET\020\002\022\007\n\003MAP\020\003\022\007\n\003HLL\020\004\022\010\n\004GSET\020\005\"\036\n\tC",
"ounterOp\022\021\n\tincrement\030\001 \001(\022\"&\n\005SetOp\022\014\n\004" +
"adds\030\001 \003(\014\022\017\n\007removes\030\002 \003(\014\"\026\n\006GSetOp\022\014\n" +
"\004adds\030\001 \003(\014\"\025\n\005HllOp\022\014\n\004adds\030\001 \003(\014\"\321\001\n\tM" +
"apUpdate\022\030\n\005field\030\001 \002(\0132\t.MapField\022\036\n\nco" +
"unter_op\030\002 \001(\0132\n.CounterOp\022\026\n\006set_op\030\003 \001" +
"(\0132\006.SetOp\022\023\n\013register_op\030\004 \001(\014\022\"\n\007flag_" +
"op\030\005 \001(\0162\021.MapUpdate.FlagOp\022\026\n\006map_op\030\006 " +
"\001(\0132\006.MapOp\"!\n\006FlagOp\022\n\n\006ENABLE\020\001\022\013\n\007DIS" +
"ABLE\020\002\"@\n\005MapOp\022\032\n\007removes\030\001 \003(\0132\t.MapFi" +
"eld\022\033\n\007updates\030\002 \003(\0132\n.MapUpdate\"\210\001\n\004DtO",
"p\022\036\n\ncounter_op\030\001 \001(\0132\n.CounterOp\022\026\n\006set" +
"_op\030\002 \001(\0132\006.SetOp\022\026\n\006map_op\030\003 \001(\0132\006.MapO" +
"p\022\026\n\006hll_op\030\004 \001(\0132\006.HllOp\022\030\n\007gset_op\030\005 \001" +
"(\0132\007.GSetOp\"\361\001\n\013DtUpdateReq\022\016\n\006bucket\030\001 " +
"\002(\014\022\013\n\003key\030\002 \001(\014\022\014\n\004type\030\003 \002(\014\022\017\n\007contex" +
"t\030\004 \001(\014\022\021\n\002op\030\005 \002(\0132\005.DtOp\022\t\n\001w\030\006 \001(\r\022\n\n" +
"\002dw\030\007 \001(\r\022\n\n\002pw\030\010 \001(\r\022\032\n\013return_body\030\t \001" +
"(\010:\005false\022\017\n\007timeout\030\n \001(\r\022\025\n\rsloppy_quo" +
"rum\030\013 \001(\010\022\r\n\005n_val\030\014 \001(\r\022\035\n\017include_cont" +
"ext\030\r \001(\010:\004true\"\233\001\n\014DtUpdateResp\022\013\n\003key\030",
"\001 \001(\014\022\017\n\007context\030\002 \001(\014\022\025\n\rcounter_value\030" +
"\003 \001(\022\022\021\n\tset_value\030\004 \003(\014\022\034\n\tmap_value\030\005 " +
"\003(\0132\t.MapEntry\022\021\n\thll_value\030\006 \001(\004\022\022\n\ngse" +
"t_value\030\007 \003(\014B#\n\027com.basho.riak.protobuf" +
"B\010RiakDtPB"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_MapField_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_MapField_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_MapField_descriptor,
new java.lang.String[] { "Name", "Type", });
internal_static_MapEntry_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_MapEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_MapEntry_descriptor,
new java.lang.String[] { "Field", "CounterValue", "SetValue", "RegisterValue", "FlagValue", "MapValue", });
internal_static_DtFetchReq_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_DtFetchReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_DtFetchReq_descriptor,
new java.lang.String[] { "Bucket", "Key", "Type", "R", "Pr", "BasicQuorum", "NotfoundOk", "Timeout", "SloppyQuorum", "NVal", "IncludeContext", });
internal_static_DtValue_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_DtValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_DtValue_descriptor,
new java.lang.String[] { "CounterValue", "SetValue", "MapValue", "HllValue", "GsetValue", });
internal_static_DtFetchResp_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_DtFetchResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_DtFetchResp_descriptor,
new java.lang.String[] { "Context", "Type", "Value", });
internal_static_CounterOp_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_CounterOp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_CounterOp_descriptor,
new java.lang.String[] { "Increment", });
internal_static_SetOp_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_SetOp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_SetOp_descriptor,
new java.lang.String[] { "Adds", "Removes", });
internal_static_GSetOp_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_GSetOp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_GSetOp_descriptor,
new java.lang.String[] { "Adds", });
internal_static_HllOp_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_HllOp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_HllOp_descriptor,
new java.lang.String[] { "Adds", });
internal_static_MapUpdate_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_MapUpdate_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_MapUpdate_descriptor,
new java.lang.String[] { "Field", "CounterOp", "SetOp", "RegisterOp", "FlagOp", "MapOp", });
internal_static_MapOp_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_MapOp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_MapOp_descriptor,
new java.lang.String[] { "Removes", "Updates", });
internal_static_DtOp_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_DtOp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_DtOp_descriptor,
new java.lang.String[] { "CounterOp", "SetOp", "MapOp", "HllOp", "GsetOp", });
internal_static_DtUpdateReq_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_DtUpdateReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_DtUpdateReq_descriptor,
new java.lang.String[] { "Bucket", "Key", "Type", "Context", "Op", "W", "Dw", "Pw", "ReturnBody", "Timeout", "SloppyQuorum", "NVal", "IncludeContext", });
internal_static_DtUpdateResp_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_DtUpdateResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_DtUpdateResp_descriptor,
new java.lang.String[] { "Key", "Context", "CounterValue", "SetValue", "MapValue", "HllValue", "GsetValue", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy