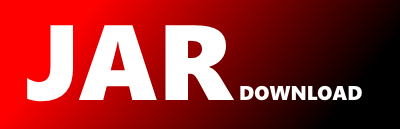
com.basho.riak.protobuf.RiakPB Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: riak.proto
package com.basho.riak.protobuf;
public final class RiakPB {
private RiakPB() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface RpbErrorRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbErrorResp)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes errmsg = 1;
*/
boolean hasErrmsg();
/**
* required bytes errmsg = 1;
*/
com.google.protobuf.ByteString getErrmsg();
/**
* required uint32 errcode = 2;
*/
boolean hasErrcode();
/**
* required uint32 errcode = 2;
*/
int getErrcode();
}
/**
* Protobuf type {@code RpbErrorResp}
*
*
* Error response - may be generated for any Req
*
*/
public static final class RpbErrorResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbErrorResp)
RpbErrorRespOrBuilder {
// Use RpbErrorResp.newBuilder() to construct.
private RpbErrorResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbErrorResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbErrorResp defaultInstance;
public static RpbErrorResp getDefaultInstance() {
return defaultInstance;
}
public RpbErrorResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbErrorResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
errmsg_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
errcode_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbErrorResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbErrorResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbErrorResp.class, com.basho.riak.protobuf.RiakPB.RpbErrorResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbErrorResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbErrorResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int ERRMSG_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString errmsg_;
/**
* required bytes errmsg = 1;
*/
public boolean hasErrmsg() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes errmsg = 1;
*/
public com.google.protobuf.ByteString getErrmsg() {
return errmsg_;
}
public static final int ERRCODE_FIELD_NUMBER = 2;
private int errcode_;
/**
* required uint32 errcode = 2;
*/
public boolean hasErrcode() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint32 errcode = 2;
*/
public int getErrcode() {
return errcode_;
}
private void initFields() {
errmsg_ = com.google.protobuf.ByteString.EMPTY;
errcode_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasErrmsg()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasErrcode()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, errmsg_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(2, errcode_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, errmsg_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, errcode_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbErrorResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbErrorResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbErrorResp}
*
*
* Error response - may be generated for any Req
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbErrorResp)
com.basho.riak.protobuf.RiakPB.RpbErrorRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbErrorResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbErrorResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbErrorResp.class, com.basho.riak.protobuf.RiakPB.RpbErrorResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbErrorResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
errmsg_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
errcode_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbErrorResp_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbErrorResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbErrorResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbErrorResp build() {
com.basho.riak.protobuf.RiakPB.RpbErrorResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbErrorResp buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbErrorResp result = new com.basho.riak.protobuf.RiakPB.RpbErrorResp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.errmsg_ = errmsg_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.errcode_ = errcode_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbErrorResp) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbErrorResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbErrorResp other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbErrorResp.getDefaultInstance()) return this;
if (other.hasErrmsg()) {
setErrmsg(other.getErrmsg());
}
if (other.hasErrcode()) {
setErrcode(other.getErrcode());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasErrmsg()) {
return false;
}
if (!hasErrcode()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbErrorResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbErrorResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString errmsg_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes errmsg = 1;
*/
public boolean hasErrmsg() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes errmsg = 1;
*/
public com.google.protobuf.ByteString getErrmsg() {
return errmsg_;
}
/**
* required bytes errmsg = 1;
*/
public Builder setErrmsg(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
errmsg_ = value;
onChanged();
return this;
}
/**
* required bytes errmsg = 1;
*/
public Builder clearErrmsg() {
bitField0_ = (bitField0_ & ~0x00000001);
errmsg_ = getDefaultInstance().getErrmsg();
onChanged();
return this;
}
private int errcode_ ;
/**
* required uint32 errcode = 2;
*/
public boolean hasErrcode() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint32 errcode = 2;
*/
public int getErrcode() {
return errcode_;
}
/**
* required uint32 errcode = 2;
*/
public Builder setErrcode(int value) {
bitField0_ |= 0x00000002;
errcode_ = value;
onChanged();
return this;
}
/**
* required uint32 errcode = 2;
*/
public Builder clearErrcode() {
bitField0_ = (bitField0_ & ~0x00000002);
errcode_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbErrorResp)
}
static {
defaultInstance = new RpbErrorResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbErrorResp)
}
public interface RpbGetServerInfoRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbGetServerInfoResp)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes node = 1;
*/
boolean hasNode();
/**
* optional bytes node = 1;
*/
com.google.protobuf.ByteString getNode();
/**
* optional bytes server_version = 2;
*/
boolean hasServerVersion();
/**
* optional bytes server_version = 2;
*/
com.google.protobuf.ByteString getServerVersion();
}
/**
* Protobuf type {@code RpbGetServerInfoResp}
*
*
* Get server info request - no message defined, just send RpbGetServerInfoReq message code
*
*/
public static final class RpbGetServerInfoResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbGetServerInfoResp)
RpbGetServerInfoRespOrBuilder {
// Use RpbGetServerInfoResp.newBuilder() to construct.
private RpbGetServerInfoResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbGetServerInfoResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbGetServerInfoResp defaultInstance;
public static RpbGetServerInfoResp getDefaultInstance() {
return defaultInstance;
}
public RpbGetServerInfoResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbGetServerInfoResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
node_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
serverVersion_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetServerInfoResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetServerInfoResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp.class, com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbGetServerInfoResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbGetServerInfoResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int NODE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString node_;
/**
* optional bytes node = 1;
*/
public boolean hasNode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes node = 1;
*/
public com.google.protobuf.ByteString getNode() {
return node_;
}
public static final int SERVER_VERSION_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString serverVersion_;
/**
* optional bytes server_version = 2;
*/
public boolean hasServerVersion() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes server_version = 2;
*/
public com.google.protobuf.ByteString getServerVersion() {
return serverVersion_;
}
private void initFields() {
node_ = com.google.protobuf.ByteString.EMPTY;
serverVersion_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, node_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, serverVersion_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, node_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, serverVersion_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbGetServerInfoResp}
*
*
* Get server info request - no message defined, just send RpbGetServerInfoReq message code
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbGetServerInfoResp)
com.basho.riak.protobuf.RiakPB.RpbGetServerInfoRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetServerInfoResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetServerInfoResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp.class, com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
node_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
serverVersion_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetServerInfoResp_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp build() {
com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp result = new com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.node_ = node_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.serverVersion_ = serverVersion_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp.getDefaultInstance()) return this;
if (other.hasNode()) {
setNode(other.getNode());
}
if (other.hasServerVersion()) {
setServerVersion(other.getServerVersion());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbGetServerInfoResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString node_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes node = 1;
*/
public boolean hasNode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes node = 1;
*/
public com.google.protobuf.ByteString getNode() {
return node_;
}
/**
* optional bytes node = 1;
*/
public Builder setNode(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
node_ = value;
onChanged();
return this;
}
/**
* optional bytes node = 1;
*/
public Builder clearNode() {
bitField0_ = (bitField0_ & ~0x00000001);
node_ = getDefaultInstance().getNode();
onChanged();
return this;
}
private com.google.protobuf.ByteString serverVersion_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes server_version = 2;
*/
public boolean hasServerVersion() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes server_version = 2;
*/
public com.google.protobuf.ByteString getServerVersion() {
return serverVersion_;
}
/**
* optional bytes server_version = 2;
*/
public Builder setServerVersion(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
serverVersion_ = value;
onChanged();
return this;
}
/**
* optional bytes server_version = 2;
*/
public Builder clearServerVersion() {
bitField0_ = (bitField0_ & ~0x00000002);
serverVersion_ = getDefaultInstance().getServerVersion();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbGetServerInfoResp)
}
static {
defaultInstance = new RpbGetServerInfoResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbGetServerInfoResp)
}
public interface RpbPairOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbPair)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes key = 1;
*/
boolean hasKey();
/**
* required bytes key = 1;
*/
com.google.protobuf.ByteString getKey();
/**
* optional bytes value = 2;
*/
boolean hasValue();
/**
* optional bytes value = 2;
*/
com.google.protobuf.ByteString getValue();
}
/**
* Protobuf type {@code RpbPair}
*
*
* Key/value pair - used for user metadata, indexes, search doc fields
*
*/
public static final class RpbPair extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbPair)
RpbPairOrBuilder {
// Use RpbPair.newBuilder() to construct.
private RpbPair(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbPair(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbPair defaultInstance;
public static RpbPair getDefaultInstance() {
return defaultInstance;
}
public RpbPair getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbPair(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
key_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbPair_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbPair_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbPair.class, com.basho.riak.protobuf.RiakPB.RpbPair.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbPair parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbPair(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString key_;
/**
* required bytes key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes key = 1;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int VALUE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString value_;
/**
* optional bytes value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes value = 2;
*/
public com.google.protobuf.ByteString getValue() {
return value_;
}
private void initFields() {
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, value_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbPair parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbPair prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbPair}
*
*
* Key/value pair - used for user metadata, indexes, search doc fields
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbPair)
com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbPair_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbPair_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbPair.class, com.basho.riak.protobuf.RiakPB.RpbPair.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbPair.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
key_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
value_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbPair_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbPair getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbPair.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbPair build() {
com.basho.riak.protobuf.RiakPB.RpbPair result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbPair buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbPair result = new com.basho.riak.protobuf.RiakPB.RpbPair(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbPair) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbPair)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbPair other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbPair.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbPair parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbPair) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes key = 1;
*/
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* required bytes key = 1;
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* required bytes key = 1;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes value = 2;
*/
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* optional bytes value = 2;
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
/**
* optional bytes value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbPair)
}
static {
defaultInstance = new RpbPair(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbPair)
}
public interface RpbGetBucketReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbGetBucketReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes bucket = 1;
*/
boolean hasBucket();
/**
* required bytes bucket = 1;
*/
com.google.protobuf.ByteString getBucket();
/**
* optional bytes type = 2;
*/
boolean hasType();
/**
* optional bytes type = 2;
*/
com.google.protobuf.ByteString getType();
}
/**
* Protobuf type {@code RpbGetBucketReq}
*
*
* Get bucket properties request
*
*/
public static final class RpbGetBucketReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbGetBucketReq)
RpbGetBucketReqOrBuilder {
// Use RpbGetBucketReq.newBuilder() to construct.
private RpbGetBucketReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbGetBucketReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbGetBucketReq defaultInstance;
public static RpbGetBucketReq getDefaultInstance() {
return defaultInstance;
}
public RpbGetBucketReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbGetBucketReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
bucket_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
type_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbGetBucketReq.class, com.basho.riak.protobuf.RiakPB.RpbGetBucketReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbGetBucketReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbGetBucketReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int BUCKET_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString bucket_;
/**
* required bytes bucket = 1;
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
public static final int TYPE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString type_;
/**
* optional bytes type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes type = 2;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
private void initFields() {
bucket_ = com.google.protobuf.ByteString.EMPTY;
type_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasBucket()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, type_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, type_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbGetBucketReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbGetBucketReq}
*
*
* Get bucket properties request
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbGetBucketReq)
com.basho.riak.protobuf.RiakPB.RpbGetBucketReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbGetBucketReq.class, com.basho.riak.protobuf.RiakPB.RpbGetBucketReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbGetBucketReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
bucket_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
type_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketReq_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbGetBucketReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketReq build() {
com.basho.riak.protobuf.RiakPB.RpbGetBucketReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketReq buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbGetBucketReq result = new com.basho.riak.protobuf.RiakPB.RpbGetBucketReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.bucket_ = bucket_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbGetBucketReq) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbGetBucketReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbGetBucketReq other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbGetBucketReq.getDefaultInstance()) return this;
if (other.hasBucket()) {
setBucket(other.getBucket());
}
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasBucket()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbGetBucketReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbGetBucketReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString bucket_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes bucket = 1;
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
/**
* required bytes bucket = 1;
*/
public Builder setBucket(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
bucket_ = value;
onChanged();
return this;
}
/**
* required bytes bucket = 1;
*/
public Builder clearBucket() {
bitField0_ = (bitField0_ & ~0x00000001);
bucket_ = getDefaultInstance().getBucket();
onChanged();
return this;
}
private com.google.protobuf.ByteString type_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes type = 2;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
/**
* optional bytes type = 2;
*/
public Builder setType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* optional bytes type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbGetBucketReq)
}
static {
defaultInstance = new RpbGetBucketReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbGetBucketReq)
}
public interface RpbGetBucketRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbGetBucketResp)
com.google.protobuf.MessageOrBuilder {
/**
* required .RpbBucketProps props = 1;
*/
boolean hasProps();
/**
* required .RpbBucketProps props = 1;
*/
com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps();
/**
* required .RpbBucketProps props = 1;
*/
com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder();
}
/**
* Protobuf type {@code RpbGetBucketResp}
*
*
* Get bucket properties response
*
*/
public static final class RpbGetBucketResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbGetBucketResp)
RpbGetBucketRespOrBuilder {
// Use RpbGetBucketResp.newBuilder() to construct.
private RpbGetBucketResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbGetBucketResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbGetBucketResp defaultInstance;
public static RpbGetBucketResp getDefaultInstance() {
return defaultInstance;
}
public RpbGetBucketResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbGetBucketResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = props_.toBuilder();
}
props_ = input.readMessage(com.basho.riak.protobuf.RiakPB.RpbBucketProps.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(props_);
props_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbGetBucketResp.class, com.basho.riak.protobuf.RiakPB.RpbGetBucketResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbGetBucketResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbGetBucketResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int PROPS_FIELD_NUMBER = 1;
private com.basho.riak.protobuf.RiakPB.RpbBucketProps props_;
/**
* required .RpbBucketProps props = 1;
*/
public boolean hasProps() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RpbBucketProps props = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps() {
return props_;
}
/**
* required .RpbBucketProps props = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder() {
return props_;
}
private void initFields() {
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasProps()) {
memoizedIsInitialized = 0;
return false;
}
if (!getProps().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, props_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, props_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbGetBucketResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbGetBucketResp}
*
*
* Get bucket properties response
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbGetBucketResp)
com.basho.riak.protobuf.RiakPB.RpbGetBucketRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbGetBucketResp.class, com.basho.riak.protobuf.RiakPB.RpbGetBucketResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbGetBucketResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getPropsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (propsBuilder_ == null) {
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
} else {
propsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketResp_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbGetBucketResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketResp build() {
com.basho.riak.protobuf.RiakPB.RpbGetBucketResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketResp buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbGetBucketResp result = new com.basho.riak.protobuf.RiakPB.RpbGetBucketResp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (propsBuilder_ == null) {
result.props_ = props_;
} else {
result.props_ = propsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbGetBucketResp) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbGetBucketResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbGetBucketResp other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbGetBucketResp.getDefaultInstance()) return this;
if (other.hasProps()) {
mergeProps(other.getProps());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasProps()) {
return false;
}
if (!getProps().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbGetBucketResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbGetBucketResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.basho.riak.protobuf.RiakPB.RpbBucketProps props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder> propsBuilder_;
/**
* required .RpbBucketProps props = 1;
*/
public boolean hasProps() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .RpbBucketProps props = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps() {
if (propsBuilder_ == null) {
return props_;
} else {
return propsBuilder_.getMessage();
}
}
/**
* required .RpbBucketProps props = 1;
*/
public Builder setProps(com.basho.riak.protobuf.RiakPB.RpbBucketProps value) {
if (propsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
props_ = value;
onChanged();
} else {
propsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RpbBucketProps props = 1;
*/
public Builder setProps(
com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder builderForValue) {
if (propsBuilder_ == null) {
props_ = builderForValue.build();
onChanged();
} else {
propsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RpbBucketProps props = 1;
*/
public Builder mergeProps(com.basho.riak.protobuf.RiakPB.RpbBucketProps value) {
if (propsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
props_ != com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance()) {
props_ =
com.basho.riak.protobuf.RiakPB.RpbBucketProps.newBuilder(props_).mergeFrom(value).buildPartial();
} else {
props_ = value;
}
onChanged();
} else {
propsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* required .RpbBucketProps props = 1;
*/
public Builder clearProps() {
if (propsBuilder_ == null) {
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
onChanged();
} else {
propsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* required .RpbBucketProps props = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder getPropsBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getPropsFieldBuilder().getBuilder();
}
/**
* required .RpbBucketProps props = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder() {
if (propsBuilder_ != null) {
return propsBuilder_.getMessageOrBuilder();
} else {
return props_;
}
}
/**
* required .RpbBucketProps props = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder>
getPropsFieldBuilder() {
if (propsBuilder_ == null) {
propsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder>(
getProps(),
getParentForChildren(),
isClean());
props_ = null;
}
return propsBuilder_;
}
// @@protoc_insertion_point(builder_scope:RpbGetBucketResp)
}
static {
defaultInstance = new RpbGetBucketResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbGetBucketResp)
}
public interface RpbSetBucketReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbSetBucketReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes bucket = 1;
*/
boolean hasBucket();
/**
* required bytes bucket = 1;
*/
com.google.protobuf.ByteString getBucket();
/**
* required .RpbBucketProps props = 2;
*/
boolean hasProps();
/**
* required .RpbBucketProps props = 2;
*/
com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps();
/**
* required .RpbBucketProps props = 2;
*/
com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder();
/**
* optional bytes type = 3;
*/
boolean hasType();
/**
* optional bytes type = 3;
*/
com.google.protobuf.ByteString getType();
}
/**
* Protobuf type {@code RpbSetBucketReq}
*
*
* Set bucket properties request
*
*/
public static final class RpbSetBucketReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbSetBucketReq)
RpbSetBucketReqOrBuilder {
// Use RpbSetBucketReq.newBuilder() to construct.
private RpbSetBucketReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbSetBucketReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbSetBucketReq defaultInstance;
public static RpbSetBucketReq getDefaultInstance() {
return defaultInstance;
}
public RpbSetBucketReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbSetBucketReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
bucket_ = input.readBytes();
break;
}
case 18: {
com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = props_.toBuilder();
}
props_ = input.readMessage(com.basho.riak.protobuf.RiakPB.RpbBucketProps.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(props_);
props_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
bitField0_ |= 0x00000004;
type_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbSetBucketReq.class, com.basho.riak.protobuf.RiakPB.RpbSetBucketReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbSetBucketReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbSetBucketReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int BUCKET_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString bucket_;
/**
* required bytes bucket = 1;
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
public static final int PROPS_FIELD_NUMBER = 2;
private com.basho.riak.protobuf.RiakPB.RpbBucketProps props_;
/**
* required .RpbBucketProps props = 2;
*/
public boolean hasProps() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps() {
return props_;
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder() {
return props_;
}
public static final int TYPE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString type_;
/**
* optional bytes type = 3;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes type = 3;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
private void initFields() {
bucket_ = com.google.protobuf.ByteString.EMPTY;
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
type_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasBucket()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasProps()) {
memoizedIsInitialized = 0;
return false;
}
if (!getProps().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, props_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, type_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, props_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, type_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbSetBucketReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbSetBucketReq}
*
*
* Set bucket properties request
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbSetBucketReq)
com.basho.riak.protobuf.RiakPB.RpbSetBucketReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbSetBucketReq.class, com.basho.riak.protobuf.RiakPB.RpbSetBucketReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbSetBucketReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getPropsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
bucket_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (propsBuilder_ == null) {
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
} else {
propsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
type_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketReq_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbSetBucketReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbSetBucketReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbSetBucketReq build() {
com.basho.riak.protobuf.RiakPB.RpbSetBucketReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbSetBucketReq buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbSetBucketReq result = new com.basho.riak.protobuf.RiakPB.RpbSetBucketReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.bucket_ = bucket_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (propsBuilder_ == null) {
result.props_ = props_;
} else {
result.props_ = propsBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbSetBucketReq) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbSetBucketReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbSetBucketReq other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbSetBucketReq.getDefaultInstance()) return this;
if (other.hasBucket()) {
setBucket(other.getBucket());
}
if (other.hasProps()) {
mergeProps(other.getProps());
}
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasBucket()) {
return false;
}
if (!hasProps()) {
return false;
}
if (!getProps().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbSetBucketReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbSetBucketReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString bucket_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes bucket = 1;
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
/**
* required bytes bucket = 1;
*/
public Builder setBucket(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
bucket_ = value;
onChanged();
return this;
}
/**
* required bytes bucket = 1;
*/
public Builder clearBucket() {
bitField0_ = (bitField0_ & ~0x00000001);
bucket_ = getDefaultInstance().getBucket();
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakPB.RpbBucketProps props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder> propsBuilder_;
/**
* required .RpbBucketProps props = 2;
*/
public boolean hasProps() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps() {
if (propsBuilder_ == null) {
return props_;
} else {
return propsBuilder_.getMessage();
}
}
/**
* required .RpbBucketProps props = 2;
*/
public Builder setProps(com.basho.riak.protobuf.RiakPB.RpbBucketProps value) {
if (propsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
props_ = value;
onChanged();
} else {
propsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .RpbBucketProps props = 2;
*/
public Builder setProps(
com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder builderForValue) {
if (propsBuilder_ == null) {
props_ = builderForValue.build();
onChanged();
} else {
propsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .RpbBucketProps props = 2;
*/
public Builder mergeProps(com.basho.riak.protobuf.RiakPB.RpbBucketProps value) {
if (propsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
props_ != com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance()) {
props_ =
com.basho.riak.protobuf.RiakPB.RpbBucketProps.newBuilder(props_).mergeFrom(value).buildPartial();
} else {
props_ = value;
}
onChanged();
} else {
propsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .RpbBucketProps props = 2;
*/
public Builder clearProps() {
if (propsBuilder_ == null) {
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
onChanged();
} else {
propsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder getPropsBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getPropsFieldBuilder().getBuilder();
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder() {
if (propsBuilder_ != null) {
return propsBuilder_.getMessageOrBuilder();
} else {
return props_;
}
}
/**
* required .RpbBucketProps props = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder>
getPropsFieldBuilder() {
if (propsBuilder_ == null) {
propsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder>(
getProps(),
getParentForChildren(),
isClean());
props_ = null;
}
return propsBuilder_;
}
private com.google.protobuf.ByteString type_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes type = 3;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes type = 3;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
/**
* optional bytes type = 3;
*/
public Builder setType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value;
onChanged();
return this;
}
/**
* optional bytes type = 3;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000004);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbSetBucketReq)
}
static {
defaultInstance = new RpbSetBucketReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbSetBucketReq)
}
public interface RpbResetBucketReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbResetBucketReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes bucket = 1;
*/
boolean hasBucket();
/**
* required bytes bucket = 1;
*/
com.google.protobuf.ByteString getBucket();
/**
* optional bytes type = 2;
*/
boolean hasType();
/**
* optional bytes type = 2;
*/
com.google.protobuf.ByteString getType();
}
/**
* Protobuf type {@code RpbResetBucketReq}
*
*
* Reset bucket properties request
*
*/
public static final class RpbResetBucketReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbResetBucketReq)
RpbResetBucketReqOrBuilder {
// Use RpbResetBucketReq.newBuilder() to construct.
private RpbResetBucketReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbResetBucketReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbResetBucketReq defaultInstance;
public static RpbResetBucketReq getDefaultInstance() {
return defaultInstance;
}
public RpbResetBucketReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbResetBucketReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
bucket_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
type_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbResetBucketReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbResetBucketReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbResetBucketReq.class, com.basho.riak.protobuf.RiakPB.RpbResetBucketReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbResetBucketReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbResetBucketReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int BUCKET_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString bucket_;
/**
* required bytes bucket = 1;
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
public static final int TYPE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString type_;
/**
* optional bytes type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes type = 2;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
private void initFields() {
bucket_ = com.google.protobuf.ByteString.EMPTY;
type_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasBucket()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, type_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, bucket_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, type_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbResetBucketReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbResetBucketReq}
*
*
* Reset bucket properties request
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbResetBucketReq)
com.basho.riak.protobuf.RiakPB.RpbResetBucketReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbResetBucketReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbResetBucketReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbResetBucketReq.class, com.basho.riak.protobuf.RiakPB.RpbResetBucketReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbResetBucketReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
bucket_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
type_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbResetBucketReq_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbResetBucketReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbResetBucketReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbResetBucketReq build() {
com.basho.riak.protobuf.RiakPB.RpbResetBucketReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbResetBucketReq buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbResetBucketReq result = new com.basho.riak.protobuf.RiakPB.RpbResetBucketReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.bucket_ = bucket_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbResetBucketReq) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbResetBucketReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbResetBucketReq other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbResetBucketReq.getDefaultInstance()) return this;
if (other.hasBucket()) {
setBucket(other.getBucket());
}
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasBucket()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbResetBucketReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbResetBucketReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString bucket_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes bucket = 1;
*/
public boolean hasBucket() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes bucket = 1;
*/
public com.google.protobuf.ByteString getBucket() {
return bucket_;
}
/**
* required bytes bucket = 1;
*/
public Builder setBucket(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
bucket_ = value;
onChanged();
return this;
}
/**
* required bytes bucket = 1;
*/
public Builder clearBucket() {
bitField0_ = (bitField0_ & ~0x00000001);
bucket_ = getDefaultInstance().getBucket();
onChanged();
return this;
}
private com.google.protobuf.ByteString type_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes type = 2;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
/**
* optional bytes type = 2;
*/
public Builder setType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* optional bytes type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbResetBucketReq)
}
static {
defaultInstance = new RpbResetBucketReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbResetBucketReq)
}
public interface RpbGetBucketTypeReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbGetBucketTypeReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes type = 1;
*/
boolean hasType();
/**
* required bytes type = 1;
*/
com.google.protobuf.ByteString getType();
}
/**
* Protobuf type {@code RpbGetBucketTypeReq}
*
*
* Get bucket properties request
*
*/
public static final class RpbGetBucketTypeReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbGetBucketTypeReq)
RpbGetBucketTypeReqOrBuilder {
// Use RpbGetBucketTypeReq.newBuilder() to construct.
private RpbGetBucketTypeReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbGetBucketTypeReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbGetBucketTypeReq defaultInstance;
public static RpbGetBucketTypeReq getDefaultInstance() {
return defaultInstance;
}
public RpbGetBucketTypeReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbGetBucketTypeReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
type_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketTypeReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketTypeReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq.class, com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbGetBucketTypeReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbGetBucketTypeReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString type_;
/**
* required bytes type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes type = 1;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
private void initFields() {
type_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, type_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, type_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbGetBucketTypeReq}
*
*
* Get bucket properties request
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbGetBucketTypeReq)
com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketTypeReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketTypeReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq.class, com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
type_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbGetBucketTypeReq_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq build() {
com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq result = new com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasType()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbGetBucketTypeReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString type_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes type = 1;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
/**
* required bytes type = 1;
*/
public Builder setType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value;
onChanged();
return this;
}
/**
* required bytes type = 1;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbGetBucketTypeReq)
}
static {
defaultInstance = new RpbGetBucketTypeReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbGetBucketTypeReq)
}
public interface RpbSetBucketTypeReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbSetBucketTypeReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes type = 1;
*/
boolean hasType();
/**
* required bytes type = 1;
*/
com.google.protobuf.ByteString getType();
/**
* required .RpbBucketProps props = 2;
*/
boolean hasProps();
/**
* required .RpbBucketProps props = 2;
*/
com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps();
/**
* required .RpbBucketProps props = 2;
*/
com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder();
}
/**
* Protobuf type {@code RpbSetBucketTypeReq}
*
*
* Set bucket properties request
*
*/
public static final class RpbSetBucketTypeReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbSetBucketTypeReq)
RpbSetBucketTypeReqOrBuilder {
// Use RpbSetBucketTypeReq.newBuilder() to construct.
private RpbSetBucketTypeReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbSetBucketTypeReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbSetBucketTypeReq defaultInstance;
public static RpbSetBucketTypeReq getDefaultInstance() {
return defaultInstance;
}
public RpbSetBucketTypeReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbSetBucketTypeReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
type_ = input.readBytes();
break;
}
case 18: {
com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = props_.toBuilder();
}
props_ = input.readMessage(com.basho.riak.protobuf.RiakPB.RpbBucketProps.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(props_);
props_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketTypeReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketTypeReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq.class, com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbSetBucketTypeReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbSetBucketTypeReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString type_;
/**
* required bytes type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes type = 1;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
public static final int PROPS_FIELD_NUMBER = 2;
private com.basho.riak.protobuf.RiakPB.RpbBucketProps props_;
/**
* required .RpbBucketProps props = 2;
*/
public boolean hasProps() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps() {
return props_;
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder() {
return props_;
}
private void initFields() {
type_ = com.google.protobuf.ByteString.EMPTY;
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasProps()) {
memoizedIsInitialized = 0;
return false;
}
if (!getProps().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, type_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, props_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, type_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, props_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbSetBucketTypeReq}
*
*
* Set bucket properties request
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbSetBucketTypeReq)
com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketTypeReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketTypeReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq.class, com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getPropsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
type_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (propsBuilder_ == null) {
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
} else {
propsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbSetBucketTypeReq_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq build() {
com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq result = new com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (propsBuilder_ == null) {
result.props_ = props_;
} else {
result.props_ = propsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasProps()) {
mergeProps(other.getProps());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasType()) {
return false;
}
if (!hasProps()) {
return false;
}
if (!getProps().isInitialized()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbSetBucketTypeReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString type_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes type = 1;
*/
public com.google.protobuf.ByteString getType() {
return type_;
}
/**
* required bytes type = 1;
*/
public Builder setType(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value;
onChanged();
return this;
}
/**
* required bytes type = 1;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakPB.RpbBucketProps props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder> propsBuilder_;
/**
* required .RpbBucketProps props = 2;
*/
public boolean hasProps() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps getProps() {
if (propsBuilder_ == null) {
return props_;
} else {
return propsBuilder_.getMessage();
}
}
/**
* required .RpbBucketProps props = 2;
*/
public Builder setProps(com.basho.riak.protobuf.RiakPB.RpbBucketProps value) {
if (propsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
props_ = value;
onChanged();
} else {
propsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .RpbBucketProps props = 2;
*/
public Builder setProps(
com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder builderForValue) {
if (propsBuilder_ == null) {
props_ = builderForValue.build();
onChanged();
} else {
propsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .RpbBucketProps props = 2;
*/
public Builder mergeProps(com.basho.riak.protobuf.RiakPB.RpbBucketProps value) {
if (propsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
props_ != com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance()) {
props_ =
com.basho.riak.protobuf.RiakPB.RpbBucketProps.newBuilder(props_).mergeFrom(value).buildPartial();
} else {
props_ = value;
}
onChanged();
} else {
propsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* required .RpbBucketProps props = 2;
*/
public Builder clearProps() {
if (propsBuilder_ == null) {
props_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
onChanged();
} else {
propsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder getPropsBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getPropsFieldBuilder().getBuilder();
}
/**
* required .RpbBucketProps props = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder getPropsOrBuilder() {
if (propsBuilder_ != null) {
return propsBuilder_.getMessageOrBuilder();
} else {
return props_;
}
}
/**
* required .RpbBucketProps props = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder>
getPropsFieldBuilder() {
if (propsBuilder_ == null) {
propsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbBucketProps, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder, com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder>(
getProps(),
getParentForChildren(),
isClean());
props_ = null;
}
return propsBuilder_;
}
// @@protoc_insertion_point(builder_scope:RpbSetBucketTypeReq)
}
static {
defaultInstance = new RpbSetBucketTypeReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbSetBucketTypeReq)
}
public interface RpbModFunOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbModFun)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes module = 1;
*/
boolean hasModule();
/**
* required bytes module = 1;
*/
com.google.protobuf.ByteString getModule();
/**
* required bytes function = 2;
*/
boolean hasFunction();
/**
* required bytes function = 2;
*/
com.google.protobuf.ByteString getFunction();
}
/**
* Protobuf type {@code RpbModFun}
*
*
* Module-Function pairs for commit hooks and other bucket properties
* that take functions
*
*/
public static final class RpbModFun extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbModFun)
RpbModFunOrBuilder {
// Use RpbModFun.newBuilder() to construct.
private RpbModFun(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbModFun(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbModFun defaultInstance;
public static RpbModFun getDefaultInstance() {
return defaultInstance;
}
public RpbModFun getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbModFun(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
module_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
function_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbModFun_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbModFun_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbModFun.class, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbModFun parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbModFun(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int MODULE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString module_;
/**
* required bytes module = 1;
*/
public boolean hasModule() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes module = 1;
*/
public com.google.protobuf.ByteString getModule() {
return module_;
}
public static final int FUNCTION_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString function_;
/**
* required bytes function = 2;
*/
public boolean hasFunction() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes function = 2;
*/
public com.google.protobuf.ByteString getFunction() {
return function_;
}
private void initFields() {
module_ = com.google.protobuf.ByteString.EMPTY;
function_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasModule()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasFunction()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, module_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, function_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, module_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, function_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbModFun parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbModFun prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbModFun}
*
*
* Module-Function pairs for commit hooks and other bucket properties
* that take functions
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbModFun)
com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbModFun_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbModFun_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbModFun.class, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbModFun.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
module_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
function_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbModFun_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbModFun getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbModFun build() {
com.basho.riak.protobuf.RiakPB.RpbModFun result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbModFun buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbModFun result = new com.basho.riak.protobuf.RiakPB.RpbModFun(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.module_ = module_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.function_ = function_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbModFun) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbModFun)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbModFun other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance()) return this;
if (other.hasModule()) {
setModule(other.getModule());
}
if (other.hasFunction()) {
setFunction(other.getFunction());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasModule()) {
return false;
}
if (!hasFunction()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbModFun parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbModFun) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString module_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes module = 1;
*/
public boolean hasModule() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes module = 1;
*/
public com.google.protobuf.ByteString getModule() {
return module_;
}
/**
* required bytes module = 1;
*/
public Builder setModule(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
module_ = value;
onChanged();
return this;
}
/**
* required bytes module = 1;
*/
public Builder clearModule() {
bitField0_ = (bitField0_ & ~0x00000001);
module_ = getDefaultInstance().getModule();
onChanged();
return this;
}
private com.google.protobuf.ByteString function_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes function = 2;
*/
public boolean hasFunction() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes function = 2;
*/
public com.google.protobuf.ByteString getFunction() {
return function_;
}
/**
* required bytes function = 2;
*/
public Builder setFunction(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
function_ = value;
onChanged();
return this;
}
/**
* required bytes function = 2;
*/
public Builder clearFunction() {
bitField0_ = (bitField0_ & ~0x00000002);
function_ = getDefaultInstance().getFunction();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbModFun)
}
static {
defaultInstance = new RpbModFun(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbModFun)
}
public interface RpbCommitHookOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbCommitHook)
com.google.protobuf.MessageOrBuilder {
/**
* optional .RpbModFun modfun = 1;
*/
boolean hasModfun();
/**
* optional .RpbModFun modfun = 1;
*/
com.basho.riak.protobuf.RiakPB.RpbModFun getModfun();
/**
* optional .RpbModFun modfun = 1;
*/
com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getModfunOrBuilder();
/**
* optional bytes name = 2;
*/
boolean hasName();
/**
* optional bytes name = 2;
*/
com.google.protobuf.ByteString getName();
}
/**
* Protobuf type {@code RpbCommitHook}
*
*
* A commit hook, which may either be a modfun or a JavaScript named
* function
*
*/
public static final class RpbCommitHook extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbCommitHook)
RpbCommitHookOrBuilder {
// Use RpbCommitHook.newBuilder() to construct.
private RpbCommitHook(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbCommitHook(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbCommitHook defaultInstance;
public static RpbCommitHook getDefaultInstance() {
return defaultInstance;
}
public RpbCommitHook getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbCommitHook(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.basho.riak.protobuf.RiakPB.RpbModFun.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = modfun_.toBuilder();
}
modfun_ = input.readMessage(com.basho.riak.protobuf.RiakPB.RpbModFun.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(modfun_);
modfun_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
bitField0_ |= 0x00000002;
name_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbCommitHook_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbCommitHook_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbCommitHook.class, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbCommitHook parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbCommitHook(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int MODFUN_FIELD_NUMBER = 1;
private com.basho.riak.protobuf.RiakPB.RpbModFun modfun_;
/**
* optional .RpbModFun modfun = 1;
*/
public boolean hasModfun() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .RpbModFun modfun = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun getModfun() {
return modfun_;
}
/**
* optional .RpbModFun modfun = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getModfunOrBuilder() {
return modfun_;
}
public static final int NAME_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString name_;
/**
* optional bytes name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes name = 2;
*/
public com.google.protobuf.ByteString getName() {
return name_;
}
private void initFields() {
modfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
name_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasModfun()) {
if (!getModfun().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, modfun_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, name_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, modfun_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, name_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbCommitHook parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbCommitHook prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbCommitHook}
*
*
* A commit hook, which may either be a modfun or a JavaScript named
* function
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbCommitHook)
com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbCommitHook_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbCommitHook_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbCommitHook.class, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbCommitHook.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getModfunFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (modfunBuilder_ == null) {
modfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
} else {
modfunBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
name_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbCommitHook_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbCommitHook getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbCommitHook.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbCommitHook build() {
com.basho.riak.protobuf.RiakPB.RpbCommitHook result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbCommitHook buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbCommitHook result = new com.basho.riak.protobuf.RiakPB.RpbCommitHook(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (modfunBuilder_ == null) {
result.modfun_ = modfun_;
} else {
result.modfun_ = modfunBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbCommitHook) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbCommitHook)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbCommitHook other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbCommitHook.getDefaultInstance()) return this;
if (other.hasModfun()) {
mergeModfun(other.getModfun());
}
if (other.hasName()) {
setName(other.getName());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (hasModfun()) {
if (!getModfun().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbCommitHook parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbCommitHook) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.basho.riak.protobuf.RiakPB.RpbModFun modfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder> modfunBuilder_;
/**
* optional .RpbModFun modfun = 1;
*/
public boolean hasModfun() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .RpbModFun modfun = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun getModfun() {
if (modfunBuilder_ == null) {
return modfun_;
} else {
return modfunBuilder_.getMessage();
}
}
/**
* optional .RpbModFun modfun = 1;
*/
public Builder setModfun(com.basho.riak.protobuf.RiakPB.RpbModFun value) {
if (modfunBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
modfun_ = value;
onChanged();
} else {
modfunBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .RpbModFun modfun = 1;
*/
public Builder setModfun(
com.basho.riak.protobuf.RiakPB.RpbModFun.Builder builderForValue) {
if (modfunBuilder_ == null) {
modfun_ = builderForValue.build();
onChanged();
} else {
modfunBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .RpbModFun modfun = 1;
*/
public Builder mergeModfun(com.basho.riak.protobuf.RiakPB.RpbModFun value) {
if (modfunBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
modfun_ != com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance()) {
modfun_ =
com.basho.riak.protobuf.RiakPB.RpbModFun.newBuilder(modfun_).mergeFrom(value).buildPartial();
} else {
modfun_ = value;
}
onChanged();
} else {
modfunBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .RpbModFun modfun = 1;
*/
public Builder clearModfun() {
if (modfunBuilder_ == null) {
modfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
onChanged();
} else {
modfunBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .RpbModFun modfun = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun.Builder getModfunBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getModfunFieldBuilder().getBuilder();
}
/**
* optional .RpbModFun modfun = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getModfunOrBuilder() {
if (modfunBuilder_ != null) {
return modfunBuilder_.getMessageOrBuilder();
} else {
return modfun_;
}
}
/**
* optional .RpbModFun modfun = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder>
getModfunFieldBuilder() {
if (modfunBuilder_ == null) {
modfunBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder>(
getModfun(),
getParentForChildren(),
isClean());
modfun_ = null;
}
return modfunBuilder_;
}
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes name = 2;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes name = 2;
*/
public com.google.protobuf.ByteString getName() {
return name_;
}
/**
* optional bytes name = 2;
*/
public Builder setName(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
* optional bytes name = 2;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbCommitHook)
}
static {
defaultInstance = new RpbCommitHook(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbCommitHook)
}
public interface RpbBucketPropsOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbBucketProps)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint32 n_val = 1;
*
*
* Declared in riak_core_app
*
*/
boolean hasNVal();
/**
* optional uint32 n_val = 1;
*
*
* Declared in riak_core_app
*
*/
int getNVal();
/**
* optional bool allow_mult = 2;
*/
boolean hasAllowMult();
/**
* optional bool allow_mult = 2;
*/
boolean getAllowMult();
/**
* optional bool last_write_wins = 3;
*/
boolean hasLastWriteWins();
/**
* optional bool last_write_wins = 3;
*/
boolean getLastWriteWins();
/**
* repeated .RpbCommitHook precommit = 4;
*/
java.util.List
getPrecommitList();
/**
* repeated .RpbCommitHook precommit = 4;
*/
com.basho.riak.protobuf.RiakPB.RpbCommitHook getPrecommit(int index);
/**
* repeated .RpbCommitHook precommit = 4;
*/
int getPrecommitCount();
/**
* repeated .RpbCommitHook precommit = 4;
*/
java.util.List extends com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>
getPrecommitOrBuilderList();
/**
* repeated .RpbCommitHook precommit = 4;
*/
com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder getPrecommitOrBuilder(
int index);
/**
* optional bool has_precommit = 5 [default = false];
*/
boolean hasHasPrecommit();
/**
* optional bool has_precommit = 5 [default = false];
*/
boolean getHasPrecommit();
/**
* repeated .RpbCommitHook postcommit = 6;
*/
java.util.List
getPostcommitList();
/**
* repeated .RpbCommitHook postcommit = 6;
*/
com.basho.riak.protobuf.RiakPB.RpbCommitHook getPostcommit(int index);
/**
* repeated .RpbCommitHook postcommit = 6;
*/
int getPostcommitCount();
/**
* repeated .RpbCommitHook postcommit = 6;
*/
java.util.List extends com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>
getPostcommitOrBuilderList();
/**
* repeated .RpbCommitHook postcommit = 6;
*/
com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder getPostcommitOrBuilder(
int index);
/**
* optional bool has_postcommit = 7 [default = false];
*/
boolean hasHasPostcommit();
/**
* optional bool has_postcommit = 7 [default = false];
*/
boolean getHasPostcommit();
/**
* optional .RpbModFun chash_keyfun = 8;
*/
boolean hasChashKeyfun();
/**
* optional .RpbModFun chash_keyfun = 8;
*/
com.basho.riak.protobuf.RiakPB.RpbModFun getChashKeyfun();
/**
* optional .RpbModFun chash_keyfun = 8;
*/
com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getChashKeyfunOrBuilder();
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
boolean hasLinkfun();
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
com.basho.riak.protobuf.RiakPB.RpbModFun getLinkfun();
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getLinkfunOrBuilder();
/**
* optional uint32 old_vclock = 10;
*/
boolean hasOldVclock();
/**
* optional uint32 old_vclock = 10;
*/
int getOldVclock();
/**
* optional uint32 young_vclock = 11;
*/
boolean hasYoungVclock();
/**
* optional uint32 young_vclock = 11;
*/
int getYoungVclock();
/**
* optional uint32 big_vclock = 12;
*/
boolean hasBigVclock();
/**
* optional uint32 big_vclock = 12;
*/
int getBigVclock();
/**
* optional uint32 small_vclock = 13;
*/
boolean hasSmallVclock();
/**
* optional uint32 small_vclock = 13;
*/
int getSmallVclock();
/**
* optional uint32 pr = 14;
*/
boolean hasPr();
/**
* optional uint32 pr = 14;
*/
int getPr();
/**
* optional uint32 r = 15;
*/
boolean hasR();
/**
* optional uint32 r = 15;
*/
int getR();
/**
* optional uint32 w = 16;
*/
boolean hasW();
/**
* optional uint32 w = 16;
*/
int getW();
/**
* optional uint32 pw = 17;
*/
boolean hasPw();
/**
* optional uint32 pw = 17;
*/
int getPw();
/**
* optional uint32 dw = 18;
*/
boolean hasDw();
/**
* optional uint32 dw = 18;
*/
int getDw();
/**
* optional uint32 rw = 19;
*/
boolean hasRw();
/**
* optional uint32 rw = 19;
*/
int getRw();
/**
* optional bool basic_quorum = 20;
*/
boolean hasBasicQuorum();
/**
* optional bool basic_quorum = 20;
*/
boolean getBasicQuorum();
/**
* optional bool notfound_ok = 21;
*/
boolean hasNotfoundOk();
/**
* optional bool notfound_ok = 21;
*/
boolean getNotfoundOk();
/**
* optional bytes backend = 22;
*
*
* Used by riak_kv_multi_backend
*
*/
boolean hasBackend();
/**
* optional bytes backend = 22;
*
*
* Used by riak_kv_multi_backend
*
*/
com.google.protobuf.ByteString getBackend();
/**
* optional bool search = 23;
*
*
* Used by riak_search bucket fixup
*
*/
boolean hasSearch();
/**
* optional bool search = 23;
*
*
* Used by riak_search bucket fixup
*
*/
boolean getSearch();
/**
* optional .RpbBucketProps.RpbReplMode repl = 24;
*/
boolean hasRepl();
/**
* optional .RpbBucketProps.RpbReplMode repl = 24;
*/
com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode getRepl();
/**
* optional bytes search_index = 25;
*
*
* Search index
*
*/
boolean hasSearchIndex();
/**
* optional bytes search_index = 25;
*
*
* Search index
*
*/
com.google.protobuf.ByteString getSearchIndex();
/**
* optional bytes datatype = 26;
*
*
* KV Datatypes
*
*/
boolean hasDatatype();
/**
* optional bytes datatype = 26;
*
*
* KV Datatypes
*
*/
com.google.protobuf.ByteString getDatatype();
/**
* optional bool consistent = 27;
*
*
* KV strong consistency
*
*/
boolean hasConsistent();
/**
* optional bool consistent = 27;
*
*
* KV strong consistency
*
*/
boolean getConsistent();
/**
* optional bool write_once = 28;
*
*
* KV fast path
*
*/
boolean hasWriteOnce();
/**
* optional bool write_once = 28;
*
*
* KV fast path
*
*/
boolean getWriteOnce();
/**
* optional uint32 hll_precision = 29;
*
*
* Hyperlolog DT Precision
*
*/
boolean hasHllPrecision();
/**
* optional uint32 hll_precision = 29;
*
*
* Hyperlolog DT Precision
*
*/
int getHllPrecision();
}
/**
* Protobuf type {@code RpbBucketProps}
*
*
* Bucket properties
*
*/
public static final class RpbBucketProps extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbBucketProps)
RpbBucketPropsOrBuilder {
// Use RpbBucketProps.newBuilder() to construct.
private RpbBucketProps(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbBucketProps(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbBucketProps defaultInstance;
public static RpbBucketProps getDefaultInstance() {
return defaultInstance;
}
public RpbBucketProps getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbBucketProps(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
nVal_ = input.readUInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
allowMult_ = input.readBool();
break;
}
case 24: {
bitField0_ |= 0x00000004;
lastWriteWins_ = input.readBool();
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
precommit_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
precommit_.add(input.readMessage(com.basho.riak.protobuf.RiakPB.RpbCommitHook.PARSER, extensionRegistry));
break;
}
case 40: {
bitField0_ |= 0x00000008;
hasPrecommit_ = input.readBool();
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
postcommit_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
postcommit_.add(input.readMessage(com.basho.riak.protobuf.RiakPB.RpbCommitHook.PARSER, extensionRegistry));
break;
}
case 56: {
bitField0_ |= 0x00000010;
hasPostcommit_ = input.readBool();
break;
}
case 66: {
com.basho.riak.protobuf.RiakPB.RpbModFun.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = chashKeyfun_.toBuilder();
}
chashKeyfun_ = input.readMessage(com.basho.riak.protobuf.RiakPB.RpbModFun.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(chashKeyfun_);
chashKeyfun_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 74: {
com.basho.riak.protobuf.RiakPB.RpbModFun.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = linkfun_.toBuilder();
}
linkfun_ = input.readMessage(com.basho.riak.protobuf.RiakPB.RpbModFun.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(linkfun_);
linkfun_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 80: {
bitField0_ |= 0x00000080;
oldVclock_ = input.readUInt32();
break;
}
case 88: {
bitField0_ |= 0x00000100;
youngVclock_ = input.readUInt32();
break;
}
case 96: {
bitField0_ |= 0x00000200;
bigVclock_ = input.readUInt32();
break;
}
case 104: {
bitField0_ |= 0x00000400;
smallVclock_ = input.readUInt32();
break;
}
case 112: {
bitField0_ |= 0x00000800;
pr_ = input.readUInt32();
break;
}
case 120: {
bitField0_ |= 0x00001000;
r_ = input.readUInt32();
break;
}
case 128: {
bitField0_ |= 0x00002000;
w_ = input.readUInt32();
break;
}
case 136: {
bitField0_ |= 0x00004000;
pw_ = input.readUInt32();
break;
}
case 144: {
bitField0_ |= 0x00008000;
dw_ = input.readUInt32();
break;
}
case 152: {
bitField0_ |= 0x00010000;
rw_ = input.readUInt32();
break;
}
case 160: {
bitField0_ |= 0x00020000;
basicQuorum_ = input.readBool();
break;
}
case 168: {
bitField0_ |= 0x00040000;
notfoundOk_ = input.readBool();
break;
}
case 178: {
bitField0_ |= 0x00080000;
backend_ = input.readBytes();
break;
}
case 184: {
bitField0_ |= 0x00100000;
search_ = input.readBool();
break;
}
case 192: {
int rawValue = input.readEnum();
com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode value = com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(24, rawValue);
} else {
bitField0_ |= 0x00200000;
repl_ = value;
}
break;
}
case 202: {
bitField0_ |= 0x00400000;
searchIndex_ = input.readBytes();
break;
}
case 210: {
bitField0_ |= 0x00800000;
datatype_ = input.readBytes();
break;
}
case 216: {
bitField0_ |= 0x01000000;
consistent_ = input.readBool();
break;
}
case 224: {
bitField0_ |= 0x02000000;
writeOnce_ = input.readBool();
break;
}
case 232: {
bitField0_ |= 0x04000000;
hllPrecision_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
precommit_ = java.util.Collections.unmodifiableList(precommit_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
postcommit_ = java.util.Collections.unmodifiableList(postcommit_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbBucketProps_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbBucketProps_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbBucketProps.class, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbBucketProps parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbBucketProps(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code RpbBucketProps.RpbReplMode}
*
*
* Used by riak_repl bucket fixup
*
*/
public enum RpbReplMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* FALSE = 0;
*/
FALSE(0, 0),
/**
* REALTIME = 1;
*/
REALTIME(1, 1),
/**
* FULLSYNC = 2;
*/
FULLSYNC(2, 2),
/**
* TRUE = 3;
*/
TRUE(3, 3),
;
/**
* FALSE = 0;
*/
public static final int FALSE_VALUE = 0;
/**
* REALTIME = 1;
*/
public static final int REALTIME_VALUE = 1;
/**
* FULLSYNC = 2;
*/
public static final int FULLSYNC_VALUE = 2;
/**
* TRUE = 3;
*/
public static final int TRUE_VALUE = 3;
public final int getNumber() { return value; }
public static RpbReplMode valueOf(int value) {
switch (value) {
case 0: return FALSE;
case 1: return REALTIME;
case 2: return FULLSYNC;
case 3: return TRUE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RpbReplMode findValueByNumber(int number) {
return RpbReplMode.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDescriptor().getEnumTypes().get(0);
}
private static final RpbReplMode[] VALUES = values();
public static RpbReplMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private RpbReplMode(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:RpbBucketProps.RpbReplMode)
}
private int bitField0_;
public static final int N_VAL_FIELD_NUMBER = 1;
private int nVal_;
/**
* optional uint32 n_val = 1;
*
*
* Declared in riak_core_app
*
*/
public boolean hasNVal() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 n_val = 1;
*
*
* Declared in riak_core_app
*
*/
public int getNVal() {
return nVal_;
}
public static final int ALLOW_MULT_FIELD_NUMBER = 2;
private boolean allowMult_;
/**
* optional bool allow_mult = 2;
*/
public boolean hasAllowMult() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool allow_mult = 2;
*/
public boolean getAllowMult() {
return allowMult_;
}
public static final int LAST_WRITE_WINS_FIELD_NUMBER = 3;
private boolean lastWriteWins_;
/**
* optional bool last_write_wins = 3;
*/
public boolean hasLastWriteWins() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool last_write_wins = 3;
*/
public boolean getLastWriteWins() {
return lastWriteWins_;
}
public static final int PRECOMMIT_FIELD_NUMBER = 4;
private java.util.List precommit_;
/**
* repeated .RpbCommitHook precommit = 4;
*/
public java.util.List getPrecommitList() {
return precommit_;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public java.util.List extends com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>
getPrecommitOrBuilderList() {
return precommit_;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public int getPrecommitCount() {
return precommit_.size();
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook getPrecommit(int index) {
return precommit_.get(index);
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder getPrecommitOrBuilder(
int index) {
return precommit_.get(index);
}
public static final int HAS_PRECOMMIT_FIELD_NUMBER = 5;
private boolean hasPrecommit_;
/**
* optional bool has_precommit = 5 [default = false];
*/
public boolean hasHasPrecommit() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool has_precommit = 5 [default = false];
*/
public boolean getHasPrecommit() {
return hasPrecommit_;
}
public static final int POSTCOMMIT_FIELD_NUMBER = 6;
private java.util.List postcommit_;
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public java.util.List getPostcommitList() {
return postcommit_;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public java.util.List extends com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>
getPostcommitOrBuilderList() {
return postcommit_;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public int getPostcommitCount() {
return postcommit_.size();
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook getPostcommit(int index) {
return postcommit_.get(index);
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder getPostcommitOrBuilder(
int index) {
return postcommit_.get(index);
}
public static final int HAS_POSTCOMMIT_FIELD_NUMBER = 7;
private boolean hasPostcommit_;
/**
* optional bool has_postcommit = 7 [default = false];
*/
public boolean hasHasPostcommit() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool has_postcommit = 7 [default = false];
*/
public boolean getHasPostcommit() {
return hasPostcommit_;
}
public static final int CHASH_KEYFUN_FIELD_NUMBER = 8;
private com.basho.riak.protobuf.RiakPB.RpbModFun chashKeyfun_;
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public boolean hasChashKeyfun() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun getChashKeyfun() {
return chashKeyfun_;
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getChashKeyfunOrBuilder() {
return chashKeyfun_;
}
public static final int LINKFUN_FIELD_NUMBER = 9;
private com.basho.riak.protobuf.RiakPB.RpbModFun linkfun_;
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public boolean hasLinkfun() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun getLinkfun() {
return linkfun_;
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getLinkfunOrBuilder() {
return linkfun_;
}
public static final int OLD_VCLOCK_FIELD_NUMBER = 10;
private int oldVclock_;
/**
* optional uint32 old_vclock = 10;
*/
public boolean hasOldVclock() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 old_vclock = 10;
*/
public int getOldVclock() {
return oldVclock_;
}
public static final int YOUNG_VCLOCK_FIELD_NUMBER = 11;
private int youngVclock_;
/**
* optional uint32 young_vclock = 11;
*/
public boolean hasYoungVclock() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional uint32 young_vclock = 11;
*/
public int getYoungVclock() {
return youngVclock_;
}
public static final int BIG_VCLOCK_FIELD_NUMBER = 12;
private int bigVclock_;
/**
* optional uint32 big_vclock = 12;
*/
public boolean hasBigVclock() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional uint32 big_vclock = 12;
*/
public int getBigVclock() {
return bigVclock_;
}
public static final int SMALL_VCLOCK_FIELD_NUMBER = 13;
private int smallVclock_;
/**
* optional uint32 small_vclock = 13;
*/
public boolean hasSmallVclock() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional uint32 small_vclock = 13;
*/
public int getSmallVclock() {
return smallVclock_;
}
public static final int PR_FIELD_NUMBER = 14;
private int pr_;
/**
* optional uint32 pr = 14;
*/
public boolean hasPr() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional uint32 pr = 14;
*/
public int getPr() {
return pr_;
}
public static final int R_FIELD_NUMBER = 15;
private int r_;
/**
* optional uint32 r = 15;
*/
public boolean hasR() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional uint32 r = 15;
*/
public int getR() {
return r_;
}
public static final int W_FIELD_NUMBER = 16;
private int w_;
/**
* optional uint32 w = 16;
*/
public boolean hasW() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional uint32 w = 16;
*/
public int getW() {
return w_;
}
public static final int PW_FIELD_NUMBER = 17;
private int pw_;
/**
* optional uint32 pw = 17;
*/
public boolean hasPw() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional uint32 pw = 17;
*/
public int getPw() {
return pw_;
}
public static final int DW_FIELD_NUMBER = 18;
private int dw_;
/**
* optional uint32 dw = 18;
*/
public boolean hasDw() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional uint32 dw = 18;
*/
public int getDw() {
return dw_;
}
public static final int RW_FIELD_NUMBER = 19;
private int rw_;
/**
* optional uint32 rw = 19;
*/
public boolean hasRw() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional uint32 rw = 19;
*/
public int getRw() {
return rw_;
}
public static final int BASIC_QUORUM_FIELD_NUMBER = 20;
private boolean basicQuorum_;
/**
* optional bool basic_quorum = 20;
*/
public boolean hasBasicQuorum() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional bool basic_quorum = 20;
*/
public boolean getBasicQuorum() {
return basicQuorum_;
}
public static final int NOTFOUND_OK_FIELD_NUMBER = 21;
private boolean notfoundOk_;
/**
* optional bool notfound_ok = 21;
*/
public boolean hasNotfoundOk() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional bool notfound_ok = 21;
*/
public boolean getNotfoundOk() {
return notfoundOk_;
}
public static final int BACKEND_FIELD_NUMBER = 22;
private com.google.protobuf.ByteString backend_;
/**
* optional bytes backend = 22;
*
*
* Used by riak_kv_multi_backend
*
*/
public boolean hasBackend() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional bytes backend = 22;
*
*
* Used by riak_kv_multi_backend
*
*/
public com.google.protobuf.ByteString getBackend() {
return backend_;
}
public static final int SEARCH_FIELD_NUMBER = 23;
private boolean search_;
/**
* optional bool search = 23;
*
*
* Used by riak_search bucket fixup
*
*/
public boolean hasSearch() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional bool search = 23;
*
*
* Used by riak_search bucket fixup
*
*/
public boolean getSearch() {
return search_;
}
public static final int REPL_FIELD_NUMBER = 24;
private com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode repl_;
/**
* optional .RpbBucketProps.RpbReplMode repl = 24;
*/
public boolean hasRepl() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional .RpbBucketProps.RpbReplMode repl = 24;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode getRepl() {
return repl_;
}
public static final int SEARCH_INDEX_FIELD_NUMBER = 25;
private com.google.protobuf.ByteString searchIndex_;
/**
* optional bytes search_index = 25;
*
*
* Search index
*
*/
public boolean hasSearchIndex() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional bytes search_index = 25;
*
*
* Search index
*
*/
public com.google.protobuf.ByteString getSearchIndex() {
return searchIndex_;
}
public static final int DATATYPE_FIELD_NUMBER = 26;
private com.google.protobuf.ByteString datatype_;
/**
* optional bytes datatype = 26;
*
*
* KV Datatypes
*
*/
public boolean hasDatatype() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional bytes datatype = 26;
*
*
* KV Datatypes
*
*/
public com.google.protobuf.ByteString getDatatype() {
return datatype_;
}
public static final int CONSISTENT_FIELD_NUMBER = 27;
private boolean consistent_;
/**
* optional bool consistent = 27;
*
*
* KV strong consistency
*
*/
public boolean hasConsistent() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional bool consistent = 27;
*
*
* KV strong consistency
*
*/
public boolean getConsistent() {
return consistent_;
}
public static final int WRITE_ONCE_FIELD_NUMBER = 28;
private boolean writeOnce_;
/**
* optional bool write_once = 28;
*
*
* KV fast path
*
*/
public boolean hasWriteOnce() {
return ((bitField0_ & 0x02000000) == 0x02000000);
}
/**
* optional bool write_once = 28;
*
*
* KV fast path
*
*/
public boolean getWriteOnce() {
return writeOnce_;
}
public static final int HLL_PRECISION_FIELD_NUMBER = 29;
private int hllPrecision_;
/**
* optional uint32 hll_precision = 29;
*
*
* Hyperlolog DT Precision
*
*/
public boolean hasHllPrecision() {
return ((bitField0_ & 0x04000000) == 0x04000000);
}
/**
* optional uint32 hll_precision = 29;
*
*
* Hyperlolog DT Precision
*
*/
public int getHllPrecision() {
return hllPrecision_;
}
private void initFields() {
nVal_ = 0;
allowMult_ = false;
lastWriteWins_ = false;
precommit_ = java.util.Collections.emptyList();
hasPrecommit_ = false;
postcommit_ = java.util.Collections.emptyList();
hasPostcommit_ = false;
chashKeyfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
linkfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
oldVclock_ = 0;
youngVclock_ = 0;
bigVclock_ = 0;
smallVclock_ = 0;
pr_ = 0;
r_ = 0;
w_ = 0;
pw_ = 0;
dw_ = 0;
rw_ = 0;
basicQuorum_ = false;
notfoundOk_ = false;
backend_ = com.google.protobuf.ByteString.EMPTY;
search_ = false;
repl_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode.FALSE;
searchIndex_ = com.google.protobuf.ByteString.EMPTY;
datatype_ = com.google.protobuf.ByteString.EMPTY;
consistent_ = false;
writeOnce_ = false;
hllPrecision_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getPrecommitCount(); i++) {
if (!getPrecommit(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getPostcommitCount(); i++) {
if (!getPostcommit(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasChashKeyfun()) {
if (!getChashKeyfun().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasLinkfun()) {
if (!getLinkfun().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, nVal_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(2, allowMult_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBool(3, lastWriteWins_);
}
for (int i = 0; i < precommit_.size(); i++) {
output.writeMessage(4, precommit_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(5, hasPrecommit_);
}
for (int i = 0; i < postcommit_.size(); i++) {
output.writeMessage(6, postcommit_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(7, hasPostcommit_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(8, chashKeyfun_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(9, linkfun_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeUInt32(10, oldVclock_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeUInt32(11, youngVclock_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeUInt32(12, bigVclock_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeUInt32(13, smallVclock_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeUInt32(14, pr_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeUInt32(15, r_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeUInt32(16, w_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeUInt32(17, pw_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeUInt32(18, dw_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeUInt32(19, rw_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
output.writeBool(20, basicQuorum_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
output.writeBool(21, notfoundOk_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
output.writeBytes(22, backend_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
output.writeBool(23, search_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
output.writeEnum(24, repl_.getNumber());
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
output.writeBytes(25, searchIndex_);
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
output.writeBytes(26, datatype_);
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
output.writeBool(27, consistent_);
}
if (((bitField0_ & 0x02000000) == 0x02000000)) {
output.writeBool(28, writeOnce_);
}
if (((bitField0_ & 0x04000000) == 0x04000000)) {
output.writeUInt32(29, hllPrecision_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, nVal_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, allowMult_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, lastWriteWins_);
}
for (int i = 0; i < precommit_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, precommit_.get(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, hasPrecommit_);
}
for (int i = 0; i < postcommit_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, postcommit_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, hasPostcommit_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, chashKeyfun_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, linkfun_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(10, oldVclock_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(11, youngVclock_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(12, bigVclock_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(13, smallVclock_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(14, pr_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(15, r_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(16, w_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(17, pw_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(18, dw_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(19, rw_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(20, basicQuorum_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(21, notfoundOk_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(22, backend_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(23, search_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(24, repl_.getNumber());
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(25, searchIndex_);
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(26, datatype_);
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(27, consistent_);
}
if (((bitField0_ & 0x02000000) == 0x02000000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(28, writeOnce_);
}
if (((bitField0_ & 0x04000000) == 0x04000000)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(29, hllPrecision_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbBucketProps parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbBucketProps prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbBucketProps}
*
*
* Bucket properties
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbBucketProps)
com.basho.riak.protobuf.RiakPB.RpbBucketPropsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbBucketProps_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbBucketProps_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbBucketProps.class, com.basho.riak.protobuf.RiakPB.RpbBucketProps.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbBucketProps.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getPrecommitFieldBuilder();
getPostcommitFieldBuilder();
getChashKeyfunFieldBuilder();
getLinkfunFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
nVal_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
allowMult_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
lastWriteWins_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
if (precommitBuilder_ == null) {
precommit_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
precommitBuilder_.clear();
}
hasPrecommit_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
if (postcommitBuilder_ == null) {
postcommit_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
postcommitBuilder_.clear();
}
hasPostcommit_ = false;
bitField0_ = (bitField0_ & ~0x00000040);
if (chashKeyfunBuilder_ == null) {
chashKeyfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
} else {
chashKeyfunBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
if (linkfunBuilder_ == null) {
linkfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
} else {
linkfunBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
oldVclock_ = 0;
bitField0_ = (bitField0_ & ~0x00000200);
youngVclock_ = 0;
bitField0_ = (bitField0_ & ~0x00000400);
bigVclock_ = 0;
bitField0_ = (bitField0_ & ~0x00000800);
smallVclock_ = 0;
bitField0_ = (bitField0_ & ~0x00001000);
pr_ = 0;
bitField0_ = (bitField0_ & ~0x00002000);
r_ = 0;
bitField0_ = (bitField0_ & ~0x00004000);
w_ = 0;
bitField0_ = (bitField0_ & ~0x00008000);
pw_ = 0;
bitField0_ = (bitField0_ & ~0x00010000);
dw_ = 0;
bitField0_ = (bitField0_ & ~0x00020000);
rw_ = 0;
bitField0_ = (bitField0_ & ~0x00040000);
basicQuorum_ = false;
bitField0_ = (bitField0_ & ~0x00080000);
notfoundOk_ = false;
bitField0_ = (bitField0_ & ~0x00100000);
backend_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00200000);
search_ = false;
bitField0_ = (bitField0_ & ~0x00400000);
repl_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode.FALSE;
bitField0_ = (bitField0_ & ~0x00800000);
searchIndex_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x01000000);
datatype_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x02000000);
consistent_ = false;
bitField0_ = (bitField0_ & ~0x04000000);
writeOnce_ = false;
bitField0_ = (bitField0_ & ~0x08000000);
hllPrecision_ = 0;
bitField0_ = (bitField0_ & ~0x10000000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbBucketProps_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbBucketProps getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbBucketProps build() {
com.basho.riak.protobuf.RiakPB.RpbBucketProps result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbBucketProps buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbBucketProps result = new com.basho.riak.protobuf.RiakPB.RpbBucketProps(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.nVal_ = nVal_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.allowMult_ = allowMult_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.lastWriteWins_ = lastWriteWins_;
if (precommitBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
precommit_ = java.util.Collections.unmodifiableList(precommit_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.precommit_ = precommit_;
} else {
result.precommit_ = precommitBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.hasPrecommit_ = hasPrecommit_;
if (postcommitBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
postcommit_ = java.util.Collections.unmodifiableList(postcommit_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.postcommit_ = postcommit_;
} else {
result.postcommit_ = postcommitBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000010;
}
result.hasPostcommit_ = hasPostcommit_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000020;
}
if (chashKeyfunBuilder_ == null) {
result.chashKeyfun_ = chashKeyfun_;
} else {
result.chashKeyfun_ = chashKeyfunBuilder_.build();
}
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000040;
}
if (linkfunBuilder_ == null) {
result.linkfun_ = linkfun_;
} else {
result.linkfun_ = linkfunBuilder_.build();
}
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000080;
}
result.oldVclock_ = oldVclock_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000100;
}
result.youngVclock_ = youngVclock_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000200;
}
result.bigVclock_ = bigVclock_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000400;
}
result.smallVclock_ = smallVclock_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00000800;
}
result.pr_ = pr_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00001000;
}
result.r_ = r_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00002000;
}
result.w_ = w_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00004000;
}
result.pw_ = pw_;
if (((from_bitField0_ & 0x00020000) == 0x00020000)) {
to_bitField0_ |= 0x00008000;
}
result.dw_ = dw_;
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00010000;
}
result.rw_ = rw_;
if (((from_bitField0_ & 0x00080000) == 0x00080000)) {
to_bitField0_ |= 0x00020000;
}
result.basicQuorum_ = basicQuorum_;
if (((from_bitField0_ & 0x00100000) == 0x00100000)) {
to_bitField0_ |= 0x00040000;
}
result.notfoundOk_ = notfoundOk_;
if (((from_bitField0_ & 0x00200000) == 0x00200000)) {
to_bitField0_ |= 0x00080000;
}
result.backend_ = backend_;
if (((from_bitField0_ & 0x00400000) == 0x00400000)) {
to_bitField0_ |= 0x00100000;
}
result.search_ = search_;
if (((from_bitField0_ & 0x00800000) == 0x00800000)) {
to_bitField0_ |= 0x00200000;
}
result.repl_ = repl_;
if (((from_bitField0_ & 0x01000000) == 0x01000000)) {
to_bitField0_ |= 0x00400000;
}
result.searchIndex_ = searchIndex_;
if (((from_bitField0_ & 0x02000000) == 0x02000000)) {
to_bitField0_ |= 0x00800000;
}
result.datatype_ = datatype_;
if (((from_bitField0_ & 0x04000000) == 0x04000000)) {
to_bitField0_ |= 0x01000000;
}
result.consistent_ = consistent_;
if (((from_bitField0_ & 0x08000000) == 0x08000000)) {
to_bitField0_ |= 0x02000000;
}
result.writeOnce_ = writeOnce_;
if (((from_bitField0_ & 0x10000000) == 0x10000000)) {
to_bitField0_ |= 0x04000000;
}
result.hllPrecision_ = hllPrecision_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbBucketProps) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbBucketProps)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbBucketProps other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbBucketProps.getDefaultInstance()) return this;
if (other.hasNVal()) {
setNVal(other.getNVal());
}
if (other.hasAllowMult()) {
setAllowMult(other.getAllowMult());
}
if (other.hasLastWriteWins()) {
setLastWriteWins(other.getLastWriteWins());
}
if (precommitBuilder_ == null) {
if (!other.precommit_.isEmpty()) {
if (precommit_.isEmpty()) {
precommit_ = other.precommit_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensurePrecommitIsMutable();
precommit_.addAll(other.precommit_);
}
onChanged();
}
} else {
if (!other.precommit_.isEmpty()) {
if (precommitBuilder_.isEmpty()) {
precommitBuilder_.dispose();
precommitBuilder_ = null;
precommit_ = other.precommit_;
bitField0_ = (bitField0_ & ~0x00000008);
precommitBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPrecommitFieldBuilder() : null;
} else {
precommitBuilder_.addAllMessages(other.precommit_);
}
}
}
if (other.hasHasPrecommit()) {
setHasPrecommit(other.getHasPrecommit());
}
if (postcommitBuilder_ == null) {
if (!other.postcommit_.isEmpty()) {
if (postcommit_.isEmpty()) {
postcommit_ = other.postcommit_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensurePostcommitIsMutable();
postcommit_.addAll(other.postcommit_);
}
onChanged();
}
} else {
if (!other.postcommit_.isEmpty()) {
if (postcommitBuilder_.isEmpty()) {
postcommitBuilder_.dispose();
postcommitBuilder_ = null;
postcommit_ = other.postcommit_;
bitField0_ = (bitField0_ & ~0x00000020);
postcommitBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPostcommitFieldBuilder() : null;
} else {
postcommitBuilder_.addAllMessages(other.postcommit_);
}
}
}
if (other.hasHasPostcommit()) {
setHasPostcommit(other.getHasPostcommit());
}
if (other.hasChashKeyfun()) {
mergeChashKeyfun(other.getChashKeyfun());
}
if (other.hasLinkfun()) {
mergeLinkfun(other.getLinkfun());
}
if (other.hasOldVclock()) {
setOldVclock(other.getOldVclock());
}
if (other.hasYoungVclock()) {
setYoungVclock(other.getYoungVclock());
}
if (other.hasBigVclock()) {
setBigVclock(other.getBigVclock());
}
if (other.hasSmallVclock()) {
setSmallVclock(other.getSmallVclock());
}
if (other.hasPr()) {
setPr(other.getPr());
}
if (other.hasR()) {
setR(other.getR());
}
if (other.hasW()) {
setW(other.getW());
}
if (other.hasPw()) {
setPw(other.getPw());
}
if (other.hasDw()) {
setDw(other.getDw());
}
if (other.hasRw()) {
setRw(other.getRw());
}
if (other.hasBasicQuorum()) {
setBasicQuorum(other.getBasicQuorum());
}
if (other.hasNotfoundOk()) {
setNotfoundOk(other.getNotfoundOk());
}
if (other.hasBackend()) {
setBackend(other.getBackend());
}
if (other.hasSearch()) {
setSearch(other.getSearch());
}
if (other.hasRepl()) {
setRepl(other.getRepl());
}
if (other.hasSearchIndex()) {
setSearchIndex(other.getSearchIndex());
}
if (other.hasDatatype()) {
setDatatype(other.getDatatype());
}
if (other.hasConsistent()) {
setConsistent(other.getConsistent());
}
if (other.hasWriteOnce()) {
setWriteOnce(other.getWriteOnce());
}
if (other.hasHllPrecision()) {
setHllPrecision(other.getHllPrecision());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getPrecommitCount(); i++) {
if (!getPrecommit(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getPostcommitCount(); i++) {
if (!getPostcommit(i).isInitialized()) {
return false;
}
}
if (hasChashKeyfun()) {
if (!getChashKeyfun().isInitialized()) {
return false;
}
}
if (hasLinkfun()) {
if (!getLinkfun().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbBucketProps parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbBucketProps) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int nVal_ ;
/**
* optional uint32 n_val = 1;
*
*
* Declared in riak_core_app
*
*/
public boolean hasNVal() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 n_val = 1;
*
*
* Declared in riak_core_app
*
*/
public int getNVal() {
return nVal_;
}
/**
* optional uint32 n_val = 1;
*
*
* Declared in riak_core_app
*
*/
public Builder setNVal(int value) {
bitField0_ |= 0x00000001;
nVal_ = value;
onChanged();
return this;
}
/**
* optional uint32 n_val = 1;
*
*
* Declared in riak_core_app
*
*/
public Builder clearNVal() {
bitField0_ = (bitField0_ & ~0x00000001);
nVal_ = 0;
onChanged();
return this;
}
private boolean allowMult_ ;
/**
* optional bool allow_mult = 2;
*/
public boolean hasAllowMult() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool allow_mult = 2;
*/
public boolean getAllowMult() {
return allowMult_;
}
/**
* optional bool allow_mult = 2;
*/
public Builder setAllowMult(boolean value) {
bitField0_ |= 0x00000002;
allowMult_ = value;
onChanged();
return this;
}
/**
* optional bool allow_mult = 2;
*/
public Builder clearAllowMult() {
bitField0_ = (bitField0_ & ~0x00000002);
allowMult_ = false;
onChanged();
return this;
}
private boolean lastWriteWins_ ;
/**
* optional bool last_write_wins = 3;
*/
public boolean hasLastWriteWins() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool last_write_wins = 3;
*/
public boolean getLastWriteWins() {
return lastWriteWins_;
}
/**
* optional bool last_write_wins = 3;
*/
public Builder setLastWriteWins(boolean value) {
bitField0_ |= 0x00000004;
lastWriteWins_ = value;
onChanged();
return this;
}
/**
* optional bool last_write_wins = 3;
*/
public Builder clearLastWriteWins() {
bitField0_ = (bitField0_ & ~0x00000004);
lastWriteWins_ = false;
onChanged();
return this;
}
private java.util.List precommit_ =
java.util.Collections.emptyList();
private void ensurePrecommitIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
precommit_ = new java.util.ArrayList(precommit_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbCommitHook, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder, com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder> precommitBuilder_;
/**
* repeated .RpbCommitHook precommit = 4;
*/
public java.util.List getPrecommitList() {
if (precommitBuilder_ == null) {
return java.util.Collections.unmodifiableList(precommit_);
} else {
return precommitBuilder_.getMessageList();
}
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public int getPrecommitCount() {
if (precommitBuilder_ == null) {
return precommit_.size();
} else {
return precommitBuilder_.getCount();
}
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook getPrecommit(int index) {
if (precommitBuilder_ == null) {
return precommit_.get(index);
} else {
return precommitBuilder_.getMessage(index);
}
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder setPrecommit(
int index, com.basho.riak.protobuf.RiakPB.RpbCommitHook value) {
if (precommitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePrecommitIsMutable();
precommit_.set(index, value);
onChanged();
} else {
precommitBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder setPrecommit(
int index, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder builderForValue) {
if (precommitBuilder_ == null) {
ensurePrecommitIsMutable();
precommit_.set(index, builderForValue.build());
onChanged();
} else {
precommitBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder addPrecommit(com.basho.riak.protobuf.RiakPB.RpbCommitHook value) {
if (precommitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePrecommitIsMutable();
precommit_.add(value);
onChanged();
} else {
precommitBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder addPrecommit(
int index, com.basho.riak.protobuf.RiakPB.RpbCommitHook value) {
if (precommitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePrecommitIsMutable();
precommit_.add(index, value);
onChanged();
} else {
precommitBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder addPrecommit(
com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder builderForValue) {
if (precommitBuilder_ == null) {
ensurePrecommitIsMutable();
precommit_.add(builderForValue.build());
onChanged();
} else {
precommitBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder addPrecommit(
int index, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder builderForValue) {
if (precommitBuilder_ == null) {
ensurePrecommitIsMutable();
precommit_.add(index, builderForValue.build());
onChanged();
} else {
precommitBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder addAllPrecommit(
java.lang.Iterable extends com.basho.riak.protobuf.RiakPB.RpbCommitHook> values) {
if (precommitBuilder_ == null) {
ensurePrecommitIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, precommit_);
onChanged();
} else {
precommitBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder clearPrecommit() {
if (precommitBuilder_ == null) {
precommit_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
precommitBuilder_.clear();
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public Builder removePrecommit(int index) {
if (precommitBuilder_ == null) {
ensurePrecommitIsMutable();
precommit_.remove(index);
onChanged();
} else {
precommitBuilder_.remove(index);
}
return this;
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder getPrecommitBuilder(
int index) {
return getPrecommitFieldBuilder().getBuilder(index);
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder getPrecommitOrBuilder(
int index) {
if (precommitBuilder_ == null) {
return precommit_.get(index); } else {
return precommitBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public java.util.List extends com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>
getPrecommitOrBuilderList() {
if (precommitBuilder_ != null) {
return precommitBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(precommit_);
}
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder addPrecommitBuilder() {
return getPrecommitFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakPB.RpbCommitHook.getDefaultInstance());
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder addPrecommitBuilder(
int index) {
return getPrecommitFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakPB.RpbCommitHook.getDefaultInstance());
}
/**
* repeated .RpbCommitHook precommit = 4;
*/
public java.util.List
getPrecommitBuilderList() {
return getPrecommitFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbCommitHook, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder, com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>
getPrecommitFieldBuilder() {
if (precommitBuilder_ == null) {
precommitBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbCommitHook, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder, com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>(
precommit_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
precommit_ = null;
}
return precommitBuilder_;
}
private boolean hasPrecommit_ ;
/**
* optional bool has_precommit = 5 [default = false];
*/
public boolean hasHasPrecommit() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool has_precommit = 5 [default = false];
*/
public boolean getHasPrecommit() {
return hasPrecommit_;
}
/**
* optional bool has_precommit = 5 [default = false];
*/
public Builder setHasPrecommit(boolean value) {
bitField0_ |= 0x00000010;
hasPrecommit_ = value;
onChanged();
return this;
}
/**
* optional bool has_precommit = 5 [default = false];
*/
public Builder clearHasPrecommit() {
bitField0_ = (bitField0_ & ~0x00000010);
hasPrecommit_ = false;
onChanged();
return this;
}
private java.util.List postcommit_ =
java.util.Collections.emptyList();
private void ensurePostcommitIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
postcommit_ = new java.util.ArrayList(postcommit_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbCommitHook, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder, com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder> postcommitBuilder_;
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public java.util.List getPostcommitList() {
if (postcommitBuilder_ == null) {
return java.util.Collections.unmodifiableList(postcommit_);
} else {
return postcommitBuilder_.getMessageList();
}
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public int getPostcommitCount() {
if (postcommitBuilder_ == null) {
return postcommit_.size();
} else {
return postcommitBuilder_.getCount();
}
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook getPostcommit(int index) {
if (postcommitBuilder_ == null) {
return postcommit_.get(index);
} else {
return postcommitBuilder_.getMessage(index);
}
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder setPostcommit(
int index, com.basho.riak.protobuf.RiakPB.RpbCommitHook value) {
if (postcommitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePostcommitIsMutable();
postcommit_.set(index, value);
onChanged();
} else {
postcommitBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder setPostcommit(
int index, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder builderForValue) {
if (postcommitBuilder_ == null) {
ensurePostcommitIsMutable();
postcommit_.set(index, builderForValue.build());
onChanged();
} else {
postcommitBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder addPostcommit(com.basho.riak.protobuf.RiakPB.RpbCommitHook value) {
if (postcommitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePostcommitIsMutable();
postcommit_.add(value);
onChanged();
} else {
postcommitBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder addPostcommit(
int index, com.basho.riak.protobuf.RiakPB.RpbCommitHook value) {
if (postcommitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePostcommitIsMutable();
postcommit_.add(index, value);
onChanged();
} else {
postcommitBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder addPostcommit(
com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder builderForValue) {
if (postcommitBuilder_ == null) {
ensurePostcommitIsMutable();
postcommit_.add(builderForValue.build());
onChanged();
} else {
postcommitBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder addPostcommit(
int index, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder builderForValue) {
if (postcommitBuilder_ == null) {
ensurePostcommitIsMutable();
postcommit_.add(index, builderForValue.build());
onChanged();
} else {
postcommitBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder addAllPostcommit(
java.lang.Iterable extends com.basho.riak.protobuf.RiakPB.RpbCommitHook> values) {
if (postcommitBuilder_ == null) {
ensurePostcommitIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, postcommit_);
onChanged();
} else {
postcommitBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder clearPostcommit() {
if (postcommitBuilder_ == null) {
postcommit_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
postcommitBuilder_.clear();
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public Builder removePostcommit(int index) {
if (postcommitBuilder_ == null) {
ensurePostcommitIsMutable();
postcommit_.remove(index);
onChanged();
} else {
postcommitBuilder_.remove(index);
}
return this;
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder getPostcommitBuilder(
int index) {
return getPostcommitFieldBuilder().getBuilder(index);
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder getPostcommitOrBuilder(
int index) {
if (postcommitBuilder_ == null) {
return postcommit_.get(index); } else {
return postcommitBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public java.util.List extends com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>
getPostcommitOrBuilderList() {
if (postcommitBuilder_ != null) {
return postcommitBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(postcommit_);
}
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder addPostcommitBuilder() {
return getPostcommitFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakPB.RpbCommitHook.getDefaultInstance());
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder addPostcommitBuilder(
int index) {
return getPostcommitFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakPB.RpbCommitHook.getDefaultInstance());
}
/**
* repeated .RpbCommitHook postcommit = 6;
*/
public java.util.List
getPostcommitBuilderList() {
return getPostcommitFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbCommitHook, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder, com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>
getPostcommitFieldBuilder() {
if (postcommitBuilder_ == null) {
postcommitBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbCommitHook, com.basho.riak.protobuf.RiakPB.RpbCommitHook.Builder, com.basho.riak.protobuf.RiakPB.RpbCommitHookOrBuilder>(
postcommit_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
postcommit_ = null;
}
return postcommitBuilder_;
}
private boolean hasPostcommit_ ;
/**
* optional bool has_postcommit = 7 [default = false];
*/
public boolean hasHasPostcommit() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool has_postcommit = 7 [default = false];
*/
public boolean getHasPostcommit() {
return hasPostcommit_;
}
/**
* optional bool has_postcommit = 7 [default = false];
*/
public Builder setHasPostcommit(boolean value) {
bitField0_ |= 0x00000040;
hasPostcommit_ = value;
onChanged();
return this;
}
/**
* optional bool has_postcommit = 7 [default = false];
*/
public Builder clearHasPostcommit() {
bitField0_ = (bitField0_ & ~0x00000040);
hasPostcommit_ = false;
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakPB.RpbModFun chashKeyfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder> chashKeyfunBuilder_;
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public boolean hasChashKeyfun() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun getChashKeyfun() {
if (chashKeyfunBuilder_ == null) {
return chashKeyfun_;
} else {
return chashKeyfunBuilder_.getMessage();
}
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public Builder setChashKeyfun(com.basho.riak.protobuf.RiakPB.RpbModFun value) {
if (chashKeyfunBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
chashKeyfun_ = value;
onChanged();
} else {
chashKeyfunBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public Builder setChashKeyfun(
com.basho.riak.protobuf.RiakPB.RpbModFun.Builder builderForValue) {
if (chashKeyfunBuilder_ == null) {
chashKeyfun_ = builderForValue.build();
onChanged();
} else {
chashKeyfunBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public Builder mergeChashKeyfun(com.basho.riak.protobuf.RiakPB.RpbModFun value) {
if (chashKeyfunBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080) &&
chashKeyfun_ != com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance()) {
chashKeyfun_ =
com.basho.riak.protobuf.RiakPB.RpbModFun.newBuilder(chashKeyfun_).mergeFrom(value).buildPartial();
} else {
chashKeyfun_ = value;
}
onChanged();
} else {
chashKeyfunBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public Builder clearChashKeyfun() {
if (chashKeyfunBuilder_ == null) {
chashKeyfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
onChanged();
} else {
chashKeyfunBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun.Builder getChashKeyfunBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getChashKeyfunFieldBuilder().getBuilder();
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
public com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getChashKeyfunOrBuilder() {
if (chashKeyfunBuilder_ != null) {
return chashKeyfunBuilder_.getMessageOrBuilder();
} else {
return chashKeyfun_;
}
}
/**
* optional .RpbModFun chash_keyfun = 8;
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder>
getChashKeyfunFieldBuilder() {
if (chashKeyfunBuilder_ == null) {
chashKeyfunBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder>(
getChashKeyfun(),
getParentForChildren(),
isClean());
chashKeyfun_ = null;
}
return chashKeyfunBuilder_;
}
private com.basho.riak.protobuf.RiakPB.RpbModFun linkfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder> linkfunBuilder_;
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public boolean hasLinkfun() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun getLinkfun() {
if (linkfunBuilder_ == null) {
return linkfun_;
} else {
return linkfunBuilder_.getMessage();
}
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public Builder setLinkfun(com.basho.riak.protobuf.RiakPB.RpbModFun value) {
if (linkfunBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
linkfun_ = value;
onChanged();
} else {
linkfunBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public Builder setLinkfun(
com.basho.riak.protobuf.RiakPB.RpbModFun.Builder builderForValue) {
if (linkfunBuilder_ == null) {
linkfun_ = builderForValue.build();
onChanged();
} else {
linkfunBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public Builder mergeLinkfun(com.basho.riak.protobuf.RiakPB.RpbModFun value) {
if (linkfunBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100) &&
linkfun_ != com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance()) {
linkfun_ =
com.basho.riak.protobuf.RiakPB.RpbModFun.newBuilder(linkfun_).mergeFrom(value).buildPartial();
} else {
linkfun_ = value;
}
onChanged();
} else {
linkfunBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public Builder clearLinkfun() {
if (linkfunBuilder_ == null) {
linkfun_ = com.basho.riak.protobuf.RiakPB.RpbModFun.getDefaultInstance();
onChanged();
} else {
linkfunBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public com.basho.riak.protobuf.RiakPB.RpbModFun.Builder getLinkfunBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getLinkfunFieldBuilder().getBuilder();
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
public com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder getLinkfunOrBuilder() {
if (linkfunBuilder_ != null) {
return linkfunBuilder_.getMessageOrBuilder();
} else {
return linkfun_;
}
}
/**
* optional .RpbModFun linkfun = 9;
*
*
* Declared in riak_kv_app
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder>
getLinkfunFieldBuilder() {
if (linkfunBuilder_ == null) {
linkfunBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbModFun, com.basho.riak.protobuf.RiakPB.RpbModFun.Builder, com.basho.riak.protobuf.RiakPB.RpbModFunOrBuilder>(
getLinkfun(),
getParentForChildren(),
isClean());
linkfun_ = null;
}
return linkfunBuilder_;
}
private int oldVclock_ ;
/**
* optional uint32 old_vclock = 10;
*/
public boolean hasOldVclock() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional uint32 old_vclock = 10;
*/
public int getOldVclock() {
return oldVclock_;
}
/**
* optional uint32 old_vclock = 10;
*/
public Builder setOldVclock(int value) {
bitField0_ |= 0x00000200;
oldVclock_ = value;
onChanged();
return this;
}
/**
* optional uint32 old_vclock = 10;
*/
public Builder clearOldVclock() {
bitField0_ = (bitField0_ & ~0x00000200);
oldVclock_ = 0;
onChanged();
return this;
}
private int youngVclock_ ;
/**
* optional uint32 young_vclock = 11;
*/
public boolean hasYoungVclock() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional uint32 young_vclock = 11;
*/
public int getYoungVclock() {
return youngVclock_;
}
/**
* optional uint32 young_vclock = 11;
*/
public Builder setYoungVclock(int value) {
bitField0_ |= 0x00000400;
youngVclock_ = value;
onChanged();
return this;
}
/**
* optional uint32 young_vclock = 11;
*/
public Builder clearYoungVclock() {
bitField0_ = (bitField0_ & ~0x00000400);
youngVclock_ = 0;
onChanged();
return this;
}
private int bigVclock_ ;
/**
* optional uint32 big_vclock = 12;
*/
public boolean hasBigVclock() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional uint32 big_vclock = 12;
*/
public int getBigVclock() {
return bigVclock_;
}
/**
* optional uint32 big_vclock = 12;
*/
public Builder setBigVclock(int value) {
bitField0_ |= 0x00000800;
bigVclock_ = value;
onChanged();
return this;
}
/**
* optional uint32 big_vclock = 12;
*/
public Builder clearBigVclock() {
bitField0_ = (bitField0_ & ~0x00000800);
bigVclock_ = 0;
onChanged();
return this;
}
private int smallVclock_ ;
/**
* optional uint32 small_vclock = 13;
*/
public boolean hasSmallVclock() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional uint32 small_vclock = 13;
*/
public int getSmallVclock() {
return smallVclock_;
}
/**
* optional uint32 small_vclock = 13;
*/
public Builder setSmallVclock(int value) {
bitField0_ |= 0x00001000;
smallVclock_ = value;
onChanged();
return this;
}
/**
* optional uint32 small_vclock = 13;
*/
public Builder clearSmallVclock() {
bitField0_ = (bitField0_ & ~0x00001000);
smallVclock_ = 0;
onChanged();
return this;
}
private int pr_ ;
/**
* optional uint32 pr = 14;
*/
public boolean hasPr() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional uint32 pr = 14;
*/
public int getPr() {
return pr_;
}
/**
* optional uint32 pr = 14;
*/
public Builder setPr(int value) {
bitField0_ |= 0x00002000;
pr_ = value;
onChanged();
return this;
}
/**
* optional uint32 pr = 14;
*/
public Builder clearPr() {
bitField0_ = (bitField0_ & ~0x00002000);
pr_ = 0;
onChanged();
return this;
}
private int r_ ;
/**
* optional uint32 r = 15;
*/
public boolean hasR() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional uint32 r = 15;
*/
public int getR() {
return r_;
}
/**
* optional uint32 r = 15;
*/
public Builder setR(int value) {
bitField0_ |= 0x00004000;
r_ = value;
onChanged();
return this;
}
/**
* optional uint32 r = 15;
*/
public Builder clearR() {
bitField0_ = (bitField0_ & ~0x00004000);
r_ = 0;
onChanged();
return this;
}
private int w_ ;
/**
* optional uint32 w = 16;
*/
public boolean hasW() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional uint32 w = 16;
*/
public int getW() {
return w_;
}
/**
* optional uint32 w = 16;
*/
public Builder setW(int value) {
bitField0_ |= 0x00008000;
w_ = value;
onChanged();
return this;
}
/**
* optional uint32 w = 16;
*/
public Builder clearW() {
bitField0_ = (bitField0_ & ~0x00008000);
w_ = 0;
onChanged();
return this;
}
private int pw_ ;
/**
* optional uint32 pw = 17;
*/
public boolean hasPw() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional uint32 pw = 17;
*/
public int getPw() {
return pw_;
}
/**
* optional uint32 pw = 17;
*/
public Builder setPw(int value) {
bitField0_ |= 0x00010000;
pw_ = value;
onChanged();
return this;
}
/**
* optional uint32 pw = 17;
*/
public Builder clearPw() {
bitField0_ = (bitField0_ & ~0x00010000);
pw_ = 0;
onChanged();
return this;
}
private int dw_ ;
/**
* optional uint32 dw = 18;
*/
public boolean hasDw() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional uint32 dw = 18;
*/
public int getDw() {
return dw_;
}
/**
* optional uint32 dw = 18;
*/
public Builder setDw(int value) {
bitField0_ |= 0x00020000;
dw_ = value;
onChanged();
return this;
}
/**
* optional uint32 dw = 18;
*/
public Builder clearDw() {
bitField0_ = (bitField0_ & ~0x00020000);
dw_ = 0;
onChanged();
return this;
}
private int rw_ ;
/**
* optional uint32 rw = 19;
*/
public boolean hasRw() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional uint32 rw = 19;
*/
public int getRw() {
return rw_;
}
/**
* optional uint32 rw = 19;
*/
public Builder setRw(int value) {
bitField0_ |= 0x00040000;
rw_ = value;
onChanged();
return this;
}
/**
* optional uint32 rw = 19;
*/
public Builder clearRw() {
bitField0_ = (bitField0_ & ~0x00040000);
rw_ = 0;
onChanged();
return this;
}
private boolean basicQuorum_ ;
/**
* optional bool basic_quorum = 20;
*/
public boolean hasBasicQuorum() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional bool basic_quorum = 20;
*/
public boolean getBasicQuorum() {
return basicQuorum_;
}
/**
* optional bool basic_quorum = 20;
*/
public Builder setBasicQuorum(boolean value) {
bitField0_ |= 0x00080000;
basicQuorum_ = value;
onChanged();
return this;
}
/**
* optional bool basic_quorum = 20;
*/
public Builder clearBasicQuorum() {
bitField0_ = (bitField0_ & ~0x00080000);
basicQuorum_ = false;
onChanged();
return this;
}
private boolean notfoundOk_ ;
/**
* optional bool notfound_ok = 21;
*/
public boolean hasNotfoundOk() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional bool notfound_ok = 21;
*/
public boolean getNotfoundOk() {
return notfoundOk_;
}
/**
* optional bool notfound_ok = 21;
*/
public Builder setNotfoundOk(boolean value) {
bitField0_ |= 0x00100000;
notfoundOk_ = value;
onChanged();
return this;
}
/**
* optional bool notfound_ok = 21;
*/
public Builder clearNotfoundOk() {
bitField0_ = (bitField0_ & ~0x00100000);
notfoundOk_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString backend_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes backend = 22;
*
*
* Used by riak_kv_multi_backend
*
*/
public boolean hasBackend() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional bytes backend = 22;
*
*
* Used by riak_kv_multi_backend
*
*/
public com.google.protobuf.ByteString getBackend() {
return backend_;
}
/**
* optional bytes backend = 22;
*
*
* Used by riak_kv_multi_backend
*
*/
public Builder setBackend(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00200000;
backend_ = value;
onChanged();
return this;
}
/**
* optional bytes backend = 22;
*
*
* Used by riak_kv_multi_backend
*
*/
public Builder clearBackend() {
bitField0_ = (bitField0_ & ~0x00200000);
backend_ = getDefaultInstance().getBackend();
onChanged();
return this;
}
private boolean search_ ;
/**
* optional bool search = 23;
*
*
* Used by riak_search bucket fixup
*
*/
public boolean hasSearch() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional bool search = 23;
*
*
* Used by riak_search bucket fixup
*
*/
public boolean getSearch() {
return search_;
}
/**
* optional bool search = 23;
*
*
* Used by riak_search bucket fixup
*
*/
public Builder setSearch(boolean value) {
bitField0_ |= 0x00400000;
search_ = value;
onChanged();
return this;
}
/**
* optional bool search = 23;
*
*
* Used by riak_search bucket fixup
*
*/
public Builder clearSearch() {
bitField0_ = (bitField0_ & ~0x00400000);
search_ = false;
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode repl_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode.FALSE;
/**
* optional .RpbBucketProps.RpbReplMode repl = 24;
*/
public boolean hasRepl() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional .RpbBucketProps.RpbReplMode repl = 24;
*/
public com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode getRepl() {
return repl_;
}
/**
* optional .RpbBucketProps.RpbReplMode repl = 24;
*/
public Builder setRepl(com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00800000;
repl_ = value;
onChanged();
return this;
}
/**
* optional .RpbBucketProps.RpbReplMode repl = 24;
*/
public Builder clearRepl() {
bitField0_ = (bitField0_ & ~0x00800000);
repl_ = com.basho.riak.protobuf.RiakPB.RpbBucketProps.RpbReplMode.FALSE;
onChanged();
return this;
}
private com.google.protobuf.ByteString searchIndex_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes search_index = 25;
*
*
* Search index
*
*/
public boolean hasSearchIndex() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional bytes search_index = 25;
*
*
* Search index
*
*/
public com.google.protobuf.ByteString getSearchIndex() {
return searchIndex_;
}
/**
* optional bytes search_index = 25;
*
*
* Search index
*
*/
public Builder setSearchIndex(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x01000000;
searchIndex_ = value;
onChanged();
return this;
}
/**
* optional bytes search_index = 25;
*
*
* Search index
*
*/
public Builder clearSearchIndex() {
bitField0_ = (bitField0_ & ~0x01000000);
searchIndex_ = getDefaultInstance().getSearchIndex();
onChanged();
return this;
}
private com.google.protobuf.ByteString datatype_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes datatype = 26;
*
*
* KV Datatypes
*
*/
public boolean hasDatatype() {
return ((bitField0_ & 0x02000000) == 0x02000000);
}
/**
* optional bytes datatype = 26;
*
*
* KV Datatypes
*
*/
public com.google.protobuf.ByteString getDatatype() {
return datatype_;
}
/**
* optional bytes datatype = 26;
*
*
* KV Datatypes
*
*/
public Builder setDatatype(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x02000000;
datatype_ = value;
onChanged();
return this;
}
/**
* optional bytes datatype = 26;
*
*
* KV Datatypes
*
*/
public Builder clearDatatype() {
bitField0_ = (bitField0_ & ~0x02000000);
datatype_ = getDefaultInstance().getDatatype();
onChanged();
return this;
}
private boolean consistent_ ;
/**
* optional bool consistent = 27;
*
*
* KV strong consistency
*
*/
public boolean hasConsistent() {
return ((bitField0_ & 0x04000000) == 0x04000000);
}
/**
* optional bool consistent = 27;
*
*
* KV strong consistency
*
*/
public boolean getConsistent() {
return consistent_;
}
/**
* optional bool consistent = 27;
*
*
* KV strong consistency
*
*/
public Builder setConsistent(boolean value) {
bitField0_ |= 0x04000000;
consistent_ = value;
onChanged();
return this;
}
/**
* optional bool consistent = 27;
*
*
* KV strong consistency
*
*/
public Builder clearConsistent() {
bitField0_ = (bitField0_ & ~0x04000000);
consistent_ = false;
onChanged();
return this;
}
private boolean writeOnce_ ;
/**
* optional bool write_once = 28;
*
*
* KV fast path
*
*/
public boolean hasWriteOnce() {
return ((bitField0_ & 0x08000000) == 0x08000000);
}
/**
* optional bool write_once = 28;
*
*
* KV fast path
*
*/
public boolean getWriteOnce() {
return writeOnce_;
}
/**
* optional bool write_once = 28;
*
*
* KV fast path
*
*/
public Builder setWriteOnce(boolean value) {
bitField0_ |= 0x08000000;
writeOnce_ = value;
onChanged();
return this;
}
/**
* optional bool write_once = 28;
*
*
* KV fast path
*
*/
public Builder clearWriteOnce() {
bitField0_ = (bitField0_ & ~0x08000000);
writeOnce_ = false;
onChanged();
return this;
}
private int hllPrecision_ ;
/**
* optional uint32 hll_precision = 29;
*
*
* Hyperlolog DT Precision
*
*/
public boolean hasHllPrecision() {
return ((bitField0_ & 0x10000000) == 0x10000000);
}
/**
* optional uint32 hll_precision = 29;
*
*
* Hyperlolog DT Precision
*
*/
public int getHllPrecision() {
return hllPrecision_;
}
/**
* optional uint32 hll_precision = 29;
*
*
* Hyperlolog DT Precision
*
*/
public Builder setHllPrecision(int value) {
bitField0_ |= 0x10000000;
hllPrecision_ = value;
onChanged();
return this;
}
/**
* optional uint32 hll_precision = 29;
*
*
* Hyperlolog DT Precision
*
*/
public Builder clearHllPrecision() {
bitField0_ = (bitField0_ & ~0x10000000);
hllPrecision_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbBucketProps)
}
static {
defaultInstance = new RpbBucketProps(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbBucketProps)
}
public interface RpbAuthReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbAuthReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes user = 1;
*/
boolean hasUser();
/**
* required bytes user = 1;
*/
com.google.protobuf.ByteString getUser();
/**
* required bytes password = 2;
*/
boolean hasPassword();
/**
* required bytes password = 2;
*/
com.google.protobuf.ByteString getPassword();
}
/**
* Protobuf type {@code RpbAuthReq}
*
*
* Authentication request
*
*/
public static final class RpbAuthReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbAuthReq)
RpbAuthReqOrBuilder {
// Use RpbAuthReq.newBuilder() to construct.
private RpbAuthReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbAuthReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbAuthReq defaultInstance;
public static RpbAuthReq getDefaultInstance() {
return defaultInstance;
}
public RpbAuthReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbAuthReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
user_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
password_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbAuthReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbAuthReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbAuthReq.class, com.basho.riak.protobuf.RiakPB.RpbAuthReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbAuthReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbAuthReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int USER_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString user_;
/**
* required bytes user = 1;
*/
public boolean hasUser() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes user = 1;
*/
public com.google.protobuf.ByteString getUser() {
return user_;
}
public static final int PASSWORD_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString password_;
/**
* required bytes password = 2;
*/
public boolean hasPassword() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes password = 2;
*/
public com.google.protobuf.ByteString getPassword() {
return password_;
}
private void initFields() {
user_ = com.google.protobuf.ByteString.EMPTY;
password_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasUser()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPassword()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, user_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, password_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, user_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, password_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakPB.RpbAuthReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakPB.RpbAuthReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbAuthReq}
*
*
* Authentication request
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbAuthReq)
com.basho.riak.protobuf.RiakPB.RpbAuthReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbAuthReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbAuthReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakPB.RpbAuthReq.class, com.basho.riak.protobuf.RiakPB.RpbAuthReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakPB.RpbAuthReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
user_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
password_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakPB.internal_static_RpbAuthReq_descriptor;
}
public com.basho.riak.protobuf.RiakPB.RpbAuthReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakPB.RpbAuthReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakPB.RpbAuthReq build() {
com.basho.riak.protobuf.RiakPB.RpbAuthReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakPB.RpbAuthReq buildPartial() {
com.basho.riak.protobuf.RiakPB.RpbAuthReq result = new com.basho.riak.protobuf.RiakPB.RpbAuthReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.user_ = user_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.password_ = password_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakPB.RpbAuthReq) {
return mergeFrom((com.basho.riak.protobuf.RiakPB.RpbAuthReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakPB.RpbAuthReq other) {
if (other == com.basho.riak.protobuf.RiakPB.RpbAuthReq.getDefaultInstance()) return this;
if (other.hasUser()) {
setUser(other.getUser());
}
if (other.hasPassword()) {
setPassword(other.getPassword());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasUser()) {
return false;
}
if (!hasPassword()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakPB.RpbAuthReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakPB.RpbAuthReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString user_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes user = 1;
*/
public boolean hasUser() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes user = 1;
*/
public com.google.protobuf.ByteString getUser() {
return user_;
}
/**
* required bytes user = 1;
*/
public Builder setUser(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
user_ = value;
onChanged();
return this;
}
/**
* required bytes user = 1;
*/
public Builder clearUser() {
bitField0_ = (bitField0_ & ~0x00000001);
user_ = getDefaultInstance().getUser();
onChanged();
return this;
}
private com.google.protobuf.ByteString password_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes password = 2;
*/
public boolean hasPassword() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes password = 2;
*/
public com.google.protobuf.ByteString getPassword() {
return password_;
}
/**
* required bytes password = 2;
*/
public Builder setPassword(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
password_ = value;
onChanged();
return this;
}
/**
* required bytes password = 2;
*/
public Builder clearPassword() {
bitField0_ = (bitField0_ & ~0x00000002);
password_ = getDefaultInstance().getPassword();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbAuthReq)
}
static {
defaultInstance = new RpbAuthReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbAuthReq)
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbErrorResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbErrorResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbGetServerInfoResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbGetServerInfoResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbPair_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbPair_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbGetBucketReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbGetBucketReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbGetBucketResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbGetBucketResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbSetBucketReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbSetBucketReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbResetBucketReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbResetBucketReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbGetBucketTypeReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbGetBucketTypeReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbSetBucketTypeReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbSetBucketTypeReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbModFun_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbModFun_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbCommitHook_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbCommitHook_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbBucketProps_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbBucketProps_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbAuthReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbAuthReq_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\nriak.proto\"/\n\014RpbErrorResp\022\016\n\006errmsg\030\001" +
" \002(\014\022\017\n\007errcode\030\002 \002(\r\"<\n\024RpbGetServerInf" +
"oResp\022\014\n\004node\030\001 \001(\014\022\026\n\016server_version\030\002 " +
"\001(\014\"%\n\007RpbPair\022\013\n\003key\030\001 \002(\014\022\r\n\005value\030\002 \001" +
"(\014\"/\n\017RpbGetBucketReq\022\016\n\006bucket\030\001 \002(\014\022\014\n" +
"\004type\030\002 \001(\014\"2\n\020RpbGetBucketResp\022\036\n\005props" +
"\030\001 \002(\0132\017.RpbBucketProps\"O\n\017RpbSetBucketR" +
"eq\022\016\n\006bucket\030\001 \002(\014\022\036\n\005props\030\002 \002(\0132\017.RpbB" +
"ucketProps\022\014\n\004type\030\003 \001(\014\"1\n\021RpbResetBuck" +
"etReq\022\016\n\006bucket\030\001 \002(\014\022\014\n\004type\030\002 \001(\014\"#\n\023R",
"pbGetBucketTypeReq\022\014\n\004type\030\001 \002(\014\"C\n\023RpbS" +
"etBucketTypeReq\022\014\n\004type\030\001 \002(\014\022\036\n\005props\030\002" +
" \002(\0132\017.RpbBucketProps\"-\n\tRpbModFun\022\016\n\006mo" +
"dule\030\001 \002(\014\022\020\n\010function\030\002 \002(\014\"9\n\rRpbCommi" +
"tHook\022\032\n\006modfun\030\001 \001(\0132\n.RpbModFun\022\014\n\004nam" +
"e\030\002 \001(\014\"\307\005\n\016RpbBucketProps\022\r\n\005n_val\030\001 \001(" +
"\r\022\022\n\nallow_mult\030\002 \001(\010\022\027\n\017last_write_wins" +
"\030\003 \001(\010\022!\n\tprecommit\030\004 \003(\0132\016.RpbCommitHoo" +
"k\022\034\n\rhas_precommit\030\005 \001(\010:\005false\022\"\n\npostc" +
"ommit\030\006 \003(\0132\016.RpbCommitHook\022\035\n\016has_postc",
"ommit\030\007 \001(\010:\005false\022 \n\014chash_keyfun\030\010 \001(\013" +
"2\n.RpbModFun\022\033\n\007linkfun\030\t \001(\0132\n.RpbModFu" +
"n\022\022\n\nold_vclock\030\n \001(\r\022\024\n\014young_vclock\030\013 " +
"\001(\r\022\022\n\nbig_vclock\030\014 \001(\r\022\024\n\014small_vclock\030" +
"\r \001(\r\022\n\n\002pr\030\016 \001(\r\022\t\n\001r\030\017 \001(\r\022\t\n\001w\030\020 \001(\r\022" +
"\n\n\002pw\030\021 \001(\r\022\n\n\002dw\030\022 \001(\r\022\n\n\002rw\030\023 \001(\r\022\024\n\014b" +
"asic_quorum\030\024 \001(\010\022\023\n\013notfound_ok\030\025 \001(\010\022\017" +
"\n\007backend\030\026 \001(\014\022\016\n\006search\030\027 \001(\010\022)\n\004repl\030" +
"\030 \001(\0162\033.RpbBucketProps.RpbReplMode\022\024\n\014se" +
"arch_index\030\031 \001(\014\022\020\n\010datatype\030\032 \001(\014\022\022\n\nco",
"nsistent\030\033 \001(\010\022\022\n\nwrite_once\030\034 \001(\010\022\025\n\rhl" +
"l_precision\030\035 \001(\r\">\n\013RpbReplMode\022\t\n\005FALS" +
"E\020\000\022\014\n\010REALTIME\020\001\022\014\n\010FULLSYNC\020\002\022\010\n\004TRUE\020" +
"\003\",\n\nRpbAuthReq\022\014\n\004user\030\001 \002(\014\022\020\n\010passwor" +
"d\030\002 \002(\014B!\n\027com.basho.riak.protobufB\006Riak" +
"PB"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_RpbErrorResp_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_RpbErrorResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbErrorResp_descriptor,
new java.lang.String[] { "Errmsg", "Errcode", });
internal_static_RpbGetServerInfoResp_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_RpbGetServerInfoResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbGetServerInfoResp_descriptor,
new java.lang.String[] { "Node", "ServerVersion", });
internal_static_RpbPair_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_RpbPair_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbPair_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_RpbGetBucketReq_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_RpbGetBucketReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbGetBucketReq_descriptor,
new java.lang.String[] { "Bucket", "Type", });
internal_static_RpbGetBucketResp_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_RpbGetBucketResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbGetBucketResp_descriptor,
new java.lang.String[] { "Props", });
internal_static_RpbSetBucketReq_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_RpbSetBucketReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbSetBucketReq_descriptor,
new java.lang.String[] { "Bucket", "Props", "Type", });
internal_static_RpbResetBucketReq_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_RpbResetBucketReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbResetBucketReq_descriptor,
new java.lang.String[] { "Bucket", "Type", });
internal_static_RpbGetBucketTypeReq_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_RpbGetBucketTypeReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbGetBucketTypeReq_descriptor,
new java.lang.String[] { "Type", });
internal_static_RpbSetBucketTypeReq_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_RpbSetBucketTypeReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbSetBucketTypeReq_descriptor,
new java.lang.String[] { "Type", "Props", });
internal_static_RpbModFun_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_RpbModFun_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbModFun_descriptor,
new java.lang.String[] { "Module", "Function", });
internal_static_RpbCommitHook_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_RpbCommitHook_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbCommitHook_descriptor,
new java.lang.String[] { "Modfun", "Name", });
internal_static_RpbBucketProps_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_RpbBucketProps_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbBucketProps_descriptor,
new java.lang.String[] { "NVal", "AllowMult", "LastWriteWins", "Precommit", "HasPrecommit", "Postcommit", "HasPostcommit", "ChashKeyfun", "Linkfun", "OldVclock", "YoungVclock", "BigVclock", "SmallVclock", "Pr", "R", "W", "Pw", "Dw", "Rw", "BasicQuorum", "NotfoundOk", "Backend", "Search", "Repl", "SearchIndex", "Datatype", "Consistent", "WriteOnce", "HllPrecision", });
internal_static_RpbAuthReq_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_RpbAuthReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbAuthReq_descriptor,
new java.lang.String[] { "User", "Password", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy