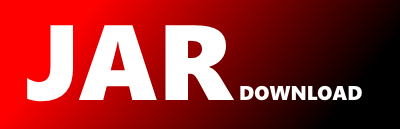
com.basho.riak.protobuf.RiakSearchPB Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: riak_search.proto
package com.basho.riak.protobuf;
public final class RiakSearchPB {
private RiakSearchPB() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface RpbSearchDocOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbSearchDoc)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .RpbPair fields = 1;
*/
java.util.List
getFieldsList();
/**
* repeated .RpbPair fields = 1;
*/
com.basho.riak.protobuf.RiakPB.RpbPair getFields(int index);
/**
* repeated .RpbPair fields = 1;
*/
int getFieldsCount();
/**
* repeated .RpbPair fields = 1;
*/
java.util.List extends com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>
getFieldsOrBuilderList();
/**
* repeated .RpbPair fields = 1;
*/
com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder getFieldsOrBuilder(
int index);
}
/**
* Protobuf type {@code RpbSearchDoc}
*/
public static final class RpbSearchDoc extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbSearchDoc)
RpbSearchDocOrBuilder {
// Use RpbSearchDoc.newBuilder() to construct.
private RpbSearchDoc(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbSearchDoc(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbSearchDoc defaultInstance;
public static RpbSearchDoc getDefaultInstance() {
return defaultInstance;
}
public RpbSearchDoc getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbSearchDoc(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
fields_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
fields_.add(input.readMessage(com.basho.riak.protobuf.RiakPB.RpbPair.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchDoc_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchDoc_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.class, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbSearchDoc parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbSearchDoc(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int FIELDS_FIELD_NUMBER = 1;
private java.util.List fields_;
/**
* repeated .RpbPair fields = 1;
*/
public java.util.List getFieldsList() {
return fields_;
}
/**
* repeated .RpbPair fields = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>
getFieldsOrBuilderList() {
return fields_;
}
/**
* repeated .RpbPair fields = 1;
*/
public int getFieldsCount() {
return fields_.size();
}
/**
* repeated .RpbPair fields = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair getFields(int index) {
return fields_.get(index);
}
/**
* repeated .RpbPair fields = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder getFieldsOrBuilder(
int index) {
return fields_.get(index);
}
private void initFields() {
fields_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getFieldsCount(); i++) {
if (!getFields(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < fields_.size(); i++) {
output.writeMessage(1, fields_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < fields_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, fields_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbSearchDoc}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbSearchDoc)
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchDoc_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchDoc_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.class, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getFieldsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
fieldsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchDoc_descriptor;
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc build() {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc buildPartial() {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc result = new com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc(this);
int from_bitField0_ = bitField0_;
if (fieldsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.fields_ = fields_;
} else {
result.fields_ = fieldsBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc) {
return mergeFrom((com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc other) {
if (other == com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.getDefaultInstance()) return this;
if (fieldsBuilder_ == null) {
if (!other.fields_.isEmpty()) {
if (fields_.isEmpty()) {
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFieldsIsMutable();
fields_.addAll(other.fields_);
}
onChanged();
}
} else {
if (!other.fields_.isEmpty()) {
if (fieldsBuilder_.isEmpty()) {
fieldsBuilder_.dispose();
fieldsBuilder_ = null;
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
fieldsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getFieldsFieldBuilder() : null;
} else {
fieldsBuilder_.addAllMessages(other.fields_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getFieldsCount(); i++) {
if (!getFields(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List fields_ =
java.util.Collections.emptyList();
private void ensureFieldsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
fields_ = new java.util.ArrayList(fields_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbPair, com.basho.riak.protobuf.RiakPB.RpbPair.Builder, com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder> fieldsBuilder_;
/**
* repeated .RpbPair fields = 1;
*/
public java.util.List getFieldsList() {
if (fieldsBuilder_ == null) {
return java.util.Collections.unmodifiableList(fields_);
} else {
return fieldsBuilder_.getMessageList();
}
}
/**
* repeated .RpbPair fields = 1;
*/
public int getFieldsCount() {
if (fieldsBuilder_ == null) {
return fields_.size();
} else {
return fieldsBuilder_.getCount();
}
}
/**
* repeated .RpbPair fields = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair getFields(int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index);
} else {
return fieldsBuilder_.getMessage(index);
}
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder setFields(
int index, com.basho.riak.protobuf.RiakPB.RpbPair value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.set(index, value);
onChanged();
} else {
fieldsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder setFields(
int index, com.basho.riak.protobuf.RiakPB.RpbPair.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.set(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder addFields(com.basho.riak.protobuf.RiakPB.RpbPair value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(value);
onChanged();
} else {
fieldsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder addFields(
int index, com.basho.riak.protobuf.RiakPB.RpbPair value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(index, value);
onChanged();
} else {
fieldsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder addFields(
com.basho.riak.protobuf.RiakPB.RpbPair.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder addFields(
int index, com.basho.riak.protobuf.RiakPB.RpbPair.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder addAllFields(
java.lang.Iterable extends com.basho.riak.protobuf.RiakPB.RpbPair> values) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fields_);
onChanged();
} else {
fieldsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder clearFields() {
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
fieldsBuilder_.clear();
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public Builder removeFields(int index) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.remove(index);
onChanged();
} else {
fieldsBuilder_.remove(index);
}
return this;
}
/**
* repeated .RpbPair fields = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair.Builder getFieldsBuilder(
int index) {
return getFieldsFieldBuilder().getBuilder(index);
}
/**
* repeated .RpbPair fields = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder getFieldsOrBuilder(
int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index); } else {
return fieldsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .RpbPair fields = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>
getFieldsOrBuilderList() {
if (fieldsBuilder_ != null) {
return fieldsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fields_);
}
}
/**
* repeated .RpbPair fields = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair.Builder addFieldsBuilder() {
return getFieldsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakPB.RpbPair.getDefaultInstance());
}
/**
* repeated .RpbPair fields = 1;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair.Builder addFieldsBuilder(
int index) {
return getFieldsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakPB.RpbPair.getDefaultInstance());
}
/**
* repeated .RpbPair fields = 1;
*/
public java.util.List
getFieldsBuilderList() {
return getFieldsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbPair, com.basho.riak.protobuf.RiakPB.RpbPair.Builder, com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>
getFieldsFieldBuilder() {
if (fieldsBuilder_ == null) {
fieldsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbPair, com.basho.riak.protobuf.RiakPB.RpbPair.Builder, com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>(
fields_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
fields_ = null;
}
return fieldsBuilder_;
}
// @@protoc_insertion_point(builder_scope:RpbSearchDoc)
}
static {
defaultInstance = new RpbSearchDoc(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbSearchDoc)
}
public interface RpbSearchQueryReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbSearchQueryReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes q = 1;
*
*
* Query string
*
*/
boolean hasQ();
/**
* required bytes q = 1;
*
*
* Query string
*
*/
com.google.protobuf.ByteString getQ();
/**
* required bytes index = 2;
*
*
* Index
*
*/
boolean hasIndex();
/**
* required bytes index = 2;
*
*
* Index
*
*/
com.google.protobuf.ByteString getIndex();
/**
* optional uint32 rows = 3;
*
*
* Limit rows
*
*/
boolean hasRows();
/**
* optional uint32 rows = 3;
*
*
* Limit rows
*
*/
int getRows();
/**
* optional uint32 start = 4;
*
*
* Starting offset
*
*/
boolean hasStart();
/**
* optional uint32 start = 4;
*
*
* Starting offset
*
*/
int getStart();
/**
* optional bytes sort = 5;
*
*
* Sort order
*
*/
boolean hasSort();
/**
* optional bytes sort = 5;
*
*
* Sort order
*
*/
com.google.protobuf.ByteString getSort();
/**
* optional bytes filter = 6;
*
*
* Inline fields filtering query
*
*/
boolean hasFilter();
/**
* optional bytes filter = 6;
*
*
* Inline fields filtering query
*
*/
com.google.protobuf.ByteString getFilter();
/**
* optional bytes df = 7;
*
*
* Default field
*
*/
boolean hasDf();
/**
* optional bytes df = 7;
*
*
* Default field
*
*/
com.google.protobuf.ByteString getDf();
/**
* optional bytes op = 8;
*
*
* Default op
*
*/
boolean hasOp();
/**
* optional bytes op = 8;
*
*
* Default op
*
*/
com.google.protobuf.ByteString getOp();
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
java.util.List getFlList();
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
int getFlCount();
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
com.google.protobuf.ByteString getFl(int index);
/**
* optional bytes presort = 10;
*
*
* Presort (key / score)
*
*/
boolean hasPresort();
/**
* optional bytes presort = 10;
*
*
* Presort (key / score)
*
*/
com.google.protobuf.ByteString getPresort();
}
/**
* Protobuf type {@code RpbSearchQueryReq}
*/
public static final class RpbSearchQueryReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbSearchQueryReq)
RpbSearchQueryReqOrBuilder {
// Use RpbSearchQueryReq.newBuilder() to construct.
private RpbSearchQueryReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbSearchQueryReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbSearchQueryReq defaultInstance;
public static RpbSearchQueryReq getDefaultInstance() {
return defaultInstance;
}
public RpbSearchQueryReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbSearchQueryReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
q_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
index_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
rows_ = input.readUInt32();
break;
}
case 32: {
bitField0_ |= 0x00000008;
start_ = input.readUInt32();
break;
}
case 42: {
bitField0_ |= 0x00000010;
sort_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000020;
filter_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000040;
df_ = input.readBytes();
break;
}
case 66: {
bitField0_ |= 0x00000080;
op_ = input.readBytes();
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
fl_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000100;
}
fl_.add(input.readBytes());
break;
}
case 82: {
bitField0_ |= 0x00000100;
presort_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
fl_ = java.util.Collections.unmodifiableList(fl_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq.class, com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbSearchQueryReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbSearchQueryReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int Q_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString q_;
/**
* required bytes q = 1;
*
*
* Query string
*
*/
public boolean hasQ() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes q = 1;
*
*
* Query string
*
*/
public com.google.protobuf.ByteString getQ() {
return q_;
}
public static final int INDEX_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString index_;
/**
* required bytes index = 2;
*
*
* Index
*
*/
public boolean hasIndex() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes index = 2;
*
*
* Index
*
*/
public com.google.protobuf.ByteString getIndex() {
return index_;
}
public static final int ROWS_FIELD_NUMBER = 3;
private int rows_;
/**
* optional uint32 rows = 3;
*
*
* Limit rows
*
*/
public boolean hasRows() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 rows = 3;
*
*
* Limit rows
*
*/
public int getRows() {
return rows_;
}
public static final int START_FIELD_NUMBER = 4;
private int start_;
/**
* optional uint32 start = 4;
*
*
* Starting offset
*
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 start = 4;
*
*
* Starting offset
*
*/
public int getStart() {
return start_;
}
public static final int SORT_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString sort_;
/**
* optional bytes sort = 5;
*
*
* Sort order
*
*/
public boolean hasSort() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bytes sort = 5;
*
*
* Sort order
*
*/
public com.google.protobuf.ByteString getSort() {
return sort_;
}
public static final int FILTER_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString filter_;
/**
* optional bytes filter = 6;
*
*
* Inline fields filtering query
*
*/
public boolean hasFilter() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bytes filter = 6;
*
*
* Inline fields filtering query
*
*/
public com.google.protobuf.ByteString getFilter() {
return filter_;
}
public static final int DF_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString df_;
/**
* optional bytes df = 7;
*
*
* Default field
*
*/
public boolean hasDf() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bytes df = 7;
*
*
* Default field
*
*/
public com.google.protobuf.ByteString getDf() {
return df_;
}
public static final int OP_FIELD_NUMBER = 8;
private com.google.protobuf.ByteString op_;
/**
* optional bytes op = 8;
*
*
* Default op
*
*/
public boolean hasOp() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bytes op = 8;
*
*
* Default op
*
*/
public com.google.protobuf.ByteString getOp() {
return op_;
}
public static final int FL_FIELD_NUMBER = 9;
private java.util.List fl_;
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public java.util.List
getFlList() {
return fl_;
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public int getFlCount() {
return fl_.size();
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public com.google.protobuf.ByteString getFl(int index) {
return fl_.get(index);
}
public static final int PRESORT_FIELD_NUMBER = 10;
private com.google.protobuf.ByteString presort_;
/**
* optional bytes presort = 10;
*
*
* Presort (key / score)
*
*/
public boolean hasPresort() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bytes presort = 10;
*
*
* Presort (key / score)
*
*/
public com.google.protobuf.ByteString getPresort() {
return presort_;
}
private void initFields() {
q_ = com.google.protobuf.ByteString.EMPTY;
index_ = com.google.protobuf.ByteString.EMPTY;
rows_ = 0;
start_ = 0;
sort_ = com.google.protobuf.ByteString.EMPTY;
filter_ = com.google.protobuf.ByteString.EMPTY;
df_ = com.google.protobuf.ByteString.EMPTY;
op_ = com.google.protobuf.ByteString.EMPTY;
fl_ = java.util.Collections.emptyList();
presort_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasQ()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasIndex()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, q_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, index_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt32(3, rows_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt32(4, start_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, sort_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, filter_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(7, df_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(8, op_);
}
for (int i = 0; i < fl_.size(); i++) {
output.writeBytes(9, fl_.get(i));
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBytes(10, presort_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, q_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, index_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, rows_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, start_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, sort_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, filter_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, df_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, op_);
}
{
int dataSize = 0;
for (int i = 0; i < fl_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(fl_.get(i));
}
size += dataSize;
size += 1 * getFlList().size();
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, presort_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbSearchQueryReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbSearchQueryReq)
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq.class, com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
q_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
index_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
rows_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
start_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
sort_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
filter_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
df_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
op_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
fl_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
presort_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryReq_descriptor;
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq build() {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq buildPartial() {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq result = new com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.q_ = q_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.index_ = index_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.rows_ = rows_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.start_ = start_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.sort_ = sort_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.filter_ = filter_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.df_ = df_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.op_ = op_;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
fl_ = java.util.Collections.unmodifiableList(fl_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.fl_ = fl_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000100;
}
result.presort_ = presort_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq) {
return mergeFrom((com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq other) {
if (other == com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq.getDefaultInstance()) return this;
if (other.hasQ()) {
setQ(other.getQ());
}
if (other.hasIndex()) {
setIndex(other.getIndex());
}
if (other.hasRows()) {
setRows(other.getRows());
}
if (other.hasStart()) {
setStart(other.getStart());
}
if (other.hasSort()) {
setSort(other.getSort());
}
if (other.hasFilter()) {
setFilter(other.getFilter());
}
if (other.hasDf()) {
setDf(other.getDf());
}
if (other.hasOp()) {
setOp(other.getOp());
}
if (!other.fl_.isEmpty()) {
if (fl_.isEmpty()) {
fl_ = other.fl_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureFlIsMutable();
fl_.addAll(other.fl_);
}
onChanged();
}
if (other.hasPresort()) {
setPresort(other.getPresort());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasQ()) {
return false;
}
if (!hasIndex()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString q_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes q = 1;
*
*
* Query string
*
*/
public boolean hasQ() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes q = 1;
*
*
* Query string
*
*/
public com.google.protobuf.ByteString getQ() {
return q_;
}
/**
* required bytes q = 1;
*
*
* Query string
*
*/
public Builder setQ(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
q_ = value;
onChanged();
return this;
}
/**
* required bytes q = 1;
*
*
* Query string
*
*/
public Builder clearQ() {
bitField0_ = (bitField0_ & ~0x00000001);
q_ = getDefaultInstance().getQ();
onChanged();
return this;
}
private com.google.protobuf.ByteString index_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes index = 2;
*
*
* Index
*
*/
public boolean hasIndex() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes index = 2;
*
*
* Index
*
*/
public com.google.protobuf.ByteString getIndex() {
return index_;
}
/**
* required bytes index = 2;
*
*
* Index
*
*/
public Builder setIndex(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
index_ = value;
onChanged();
return this;
}
/**
* required bytes index = 2;
*
*
* Index
*
*/
public Builder clearIndex() {
bitField0_ = (bitField0_ & ~0x00000002);
index_ = getDefaultInstance().getIndex();
onChanged();
return this;
}
private int rows_ ;
/**
* optional uint32 rows = 3;
*
*
* Limit rows
*
*/
public boolean hasRows() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 rows = 3;
*
*
* Limit rows
*
*/
public int getRows() {
return rows_;
}
/**
* optional uint32 rows = 3;
*
*
* Limit rows
*
*/
public Builder setRows(int value) {
bitField0_ |= 0x00000004;
rows_ = value;
onChanged();
return this;
}
/**
* optional uint32 rows = 3;
*
*
* Limit rows
*
*/
public Builder clearRows() {
bitField0_ = (bitField0_ & ~0x00000004);
rows_ = 0;
onChanged();
return this;
}
private int start_ ;
/**
* optional uint32 start = 4;
*
*
* Starting offset
*
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 start = 4;
*
*
* Starting offset
*
*/
public int getStart() {
return start_;
}
/**
* optional uint32 start = 4;
*
*
* Starting offset
*
*/
public Builder setStart(int value) {
bitField0_ |= 0x00000008;
start_ = value;
onChanged();
return this;
}
/**
* optional uint32 start = 4;
*
*
* Starting offset
*
*/
public Builder clearStart() {
bitField0_ = (bitField0_ & ~0x00000008);
start_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString sort_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes sort = 5;
*
*
* Sort order
*
*/
public boolean hasSort() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bytes sort = 5;
*
*
* Sort order
*
*/
public com.google.protobuf.ByteString getSort() {
return sort_;
}
/**
* optional bytes sort = 5;
*
*
* Sort order
*
*/
public Builder setSort(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
sort_ = value;
onChanged();
return this;
}
/**
* optional bytes sort = 5;
*
*
* Sort order
*
*/
public Builder clearSort() {
bitField0_ = (bitField0_ & ~0x00000010);
sort_ = getDefaultInstance().getSort();
onChanged();
return this;
}
private com.google.protobuf.ByteString filter_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes filter = 6;
*
*
* Inline fields filtering query
*
*/
public boolean hasFilter() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bytes filter = 6;
*
*
* Inline fields filtering query
*
*/
public com.google.protobuf.ByteString getFilter() {
return filter_;
}
/**
* optional bytes filter = 6;
*
*
* Inline fields filtering query
*
*/
public Builder setFilter(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
filter_ = value;
onChanged();
return this;
}
/**
* optional bytes filter = 6;
*
*
* Inline fields filtering query
*
*/
public Builder clearFilter() {
bitField0_ = (bitField0_ & ~0x00000020);
filter_ = getDefaultInstance().getFilter();
onChanged();
return this;
}
private com.google.protobuf.ByteString df_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes df = 7;
*
*
* Default field
*
*/
public boolean hasDf() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bytes df = 7;
*
*
* Default field
*
*/
public com.google.protobuf.ByteString getDf() {
return df_;
}
/**
* optional bytes df = 7;
*
*
* Default field
*
*/
public Builder setDf(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
df_ = value;
onChanged();
return this;
}
/**
* optional bytes df = 7;
*
*
* Default field
*
*/
public Builder clearDf() {
bitField0_ = (bitField0_ & ~0x00000040);
df_ = getDefaultInstance().getDf();
onChanged();
return this;
}
private com.google.protobuf.ByteString op_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes op = 8;
*
*
* Default op
*
*/
public boolean hasOp() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bytes op = 8;
*
*
* Default op
*
*/
public com.google.protobuf.ByteString getOp() {
return op_;
}
/**
* optional bytes op = 8;
*
*
* Default op
*
*/
public Builder setOp(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
op_ = value;
onChanged();
return this;
}
/**
* optional bytes op = 8;
*
*
* Default op
*
*/
public Builder clearOp() {
bitField0_ = (bitField0_ & ~0x00000080);
op_ = getDefaultInstance().getOp();
onChanged();
return this;
}
private java.util.List fl_ = java.util.Collections.emptyList();
private void ensureFlIsMutable() {
if (!((bitField0_ & 0x00000100) == 0x00000100)) {
fl_ = new java.util.ArrayList(fl_);
bitField0_ |= 0x00000100;
}
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public java.util.List
getFlList() {
return java.util.Collections.unmodifiableList(fl_);
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public int getFlCount() {
return fl_.size();
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public com.google.protobuf.ByteString getFl(int index) {
return fl_.get(index);
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public Builder setFl(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureFlIsMutable();
fl_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public Builder addFl(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureFlIsMutable();
fl_.add(value);
onChanged();
return this;
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public Builder addAllFl(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureFlIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fl_);
onChanged();
return this;
}
/**
* repeated bytes fl = 9;
*
*
* Return fields limit (for ids only, generally)
*
*/
public Builder clearFl() {
fl_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
private com.google.protobuf.ByteString presort_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes presort = 10;
*
*
* Presort (key / score)
*
*/
public boolean hasPresort() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bytes presort = 10;
*
*
* Presort (key / score)
*
*/
public com.google.protobuf.ByteString getPresort() {
return presort_;
}
/**
* optional bytes presort = 10;
*
*
* Presort (key / score)
*
*/
public Builder setPresort(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
presort_ = value;
onChanged();
return this;
}
/**
* optional bytes presort = 10;
*
*
* Presort (key / score)
*
*/
public Builder clearPresort() {
bitField0_ = (bitField0_ & ~0x00000200);
presort_ = getDefaultInstance().getPresort();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbSearchQueryReq)
}
static {
defaultInstance = new RpbSearchQueryReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbSearchQueryReq)
}
public interface RpbSearchQueryRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:RpbSearchQueryResp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
java.util.List
getDocsList();
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc getDocs(int index);
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
int getDocsCount();
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
java.util.List extends com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder>
getDocsOrBuilderList();
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder getDocsOrBuilder(
int index);
/**
* optional float max_score = 2;
*
*
* Maximum score
*
*/
boolean hasMaxScore();
/**
* optional float max_score = 2;
*
*
* Maximum score
*
*/
float getMaxScore();
/**
* optional uint32 num_found = 3;
*
*
* Number of results
*
*/
boolean hasNumFound();
/**
* optional uint32 num_found = 3;
*
*
* Number of results
*
*/
int getNumFound();
}
/**
* Protobuf type {@code RpbSearchQueryResp}
*/
public static final class RpbSearchQueryResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:RpbSearchQueryResp)
RpbSearchQueryRespOrBuilder {
// Use RpbSearchQueryResp.newBuilder() to construct.
private RpbSearchQueryResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RpbSearchQueryResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RpbSearchQueryResp defaultInstance;
public static RpbSearchQueryResp getDefaultInstance() {
return defaultInstance;
}
public RpbSearchQueryResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RpbSearchQueryResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
docs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
docs_.add(input.readMessage(com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.PARSER, extensionRegistry));
break;
}
case 21: {
bitField0_ |= 0x00000001;
maxScore_ = input.readFloat();
break;
}
case 24: {
bitField0_ |= 0x00000002;
numFound_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
docs_ = java.util.Collections.unmodifiableList(docs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp.class, com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RpbSearchQueryResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RpbSearchQueryResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int DOCS_FIELD_NUMBER = 1;
private java.util.List docs_;
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public java.util.List getDocsList() {
return docs_;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder>
getDocsOrBuilderList() {
return docs_;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public int getDocsCount() {
return docs_.size();
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc getDocs(int index) {
return docs_.get(index);
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder getDocsOrBuilder(
int index) {
return docs_.get(index);
}
public static final int MAX_SCORE_FIELD_NUMBER = 2;
private float maxScore_;
/**
* optional float max_score = 2;
*
*
* Maximum score
*
*/
public boolean hasMaxScore() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional float max_score = 2;
*
*
* Maximum score
*
*/
public float getMaxScore() {
return maxScore_;
}
public static final int NUM_FOUND_FIELD_NUMBER = 3;
private int numFound_;
/**
* optional uint32 num_found = 3;
*
*
* Number of results
*
*/
public boolean hasNumFound() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint32 num_found = 3;
*
*
* Number of results
*
*/
public int getNumFound() {
return numFound_;
}
private void initFields() {
docs_ = java.util.Collections.emptyList();
maxScore_ = 0F;
numFound_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getDocsCount(); i++) {
if (!getDocs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < docs_.size(); i++) {
output.writeMessage(1, docs_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeFloat(2, maxScore_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(3, numFound_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < docs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, docs_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(2, maxScore_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, numFound_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code RpbSearchQueryResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:RpbSearchQueryResp)
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp.class, com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getDocsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (docsBuilder_ == null) {
docs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
docsBuilder_.clear();
}
maxScore_ = 0F;
bitField0_ = (bitField0_ & ~0x00000002);
numFound_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakSearchPB.internal_static_RpbSearchQueryResp_descriptor;
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp build() {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp buildPartial() {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp result = new com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (docsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
docs_ = java.util.Collections.unmodifiableList(docs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.docs_ = docs_;
} else {
result.docs_ = docsBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.maxScore_ = maxScore_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.numFound_ = numFound_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp) {
return mergeFrom((com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp other) {
if (other == com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp.getDefaultInstance()) return this;
if (docsBuilder_ == null) {
if (!other.docs_.isEmpty()) {
if (docs_.isEmpty()) {
docs_ = other.docs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDocsIsMutable();
docs_.addAll(other.docs_);
}
onChanged();
}
} else {
if (!other.docs_.isEmpty()) {
if (docsBuilder_.isEmpty()) {
docsBuilder_.dispose();
docsBuilder_ = null;
docs_ = other.docs_;
bitField0_ = (bitField0_ & ~0x00000001);
docsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getDocsFieldBuilder() : null;
} else {
docsBuilder_.addAllMessages(other.docs_);
}
}
}
if (other.hasMaxScore()) {
setMaxScore(other.getMaxScore());
}
if (other.hasNumFound()) {
setNumFound(other.getNumFound());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getDocsCount(); i++) {
if (!getDocs(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakSearchPB.RpbSearchQueryResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List docs_ =
java.util.Collections.emptyList();
private void ensureDocsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
docs_ = new java.util.ArrayList(docs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder> docsBuilder_;
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public java.util.List getDocsList() {
if (docsBuilder_ == null) {
return java.util.Collections.unmodifiableList(docs_);
} else {
return docsBuilder_.getMessageList();
}
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public int getDocsCount() {
if (docsBuilder_ == null) {
return docs_.size();
} else {
return docsBuilder_.getCount();
}
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc getDocs(int index) {
if (docsBuilder_ == null) {
return docs_.get(index);
} else {
return docsBuilder_.getMessage(index);
}
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder setDocs(
int index, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc value) {
if (docsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDocsIsMutable();
docs_.set(index, value);
onChanged();
} else {
docsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder setDocs(
int index, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder builderForValue) {
if (docsBuilder_ == null) {
ensureDocsIsMutable();
docs_.set(index, builderForValue.build());
onChanged();
} else {
docsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder addDocs(com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc value) {
if (docsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDocsIsMutable();
docs_.add(value);
onChanged();
} else {
docsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder addDocs(
int index, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc value) {
if (docsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDocsIsMutable();
docs_.add(index, value);
onChanged();
} else {
docsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder addDocs(
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder builderForValue) {
if (docsBuilder_ == null) {
ensureDocsIsMutable();
docs_.add(builderForValue.build());
onChanged();
} else {
docsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder addDocs(
int index, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder builderForValue) {
if (docsBuilder_ == null) {
ensureDocsIsMutable();
docs_.add(index, builderForValue.build());
onChanged();
} else {
docsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder addAllDocs(
java.lang.Iterable extends com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc> values) {
if (docsBuilder_ == null) {
ensureDocsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, docs_);
onChanged();
} else {
docsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder clearDocs() {
if (docsBuilder_ == null) {
docs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
docsBuilder_.clear();
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public Builder removeDocs(int index) {
if (docsBuilder_ == null) {
ensureDocsIsMutable();
docs_.remove(index);
onChanged();
} else {
docsBuilder_.remove(index);
}
return this;
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder getDocsBuilder(
int index) {
return getDocsFieldBuilder().getBuilder(index);
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder getDocsOrBuilder(
int index) {
if (docsBuilder_ == null) {
return docs_.get(index); } else {
return docsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder>
getDocsOrBuilderList() {
if (docsBuilder_ != null) {
return docsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(docs_);
}
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder addDocsBuilder() {
return getDocsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.getDefaultInstance());
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder addDocsBuilder(
int index) {
return getDocsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.getDefaultInstance());
}
/**
* repeated .RpbSearchDoc docs = 1;
*
*
* Result documents
*
*/
public java.util.List
getDocsBuilderList() {
return getDocsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder>
getDocsFieldBuilder() {
if (docsBuilder_ == null) {
docsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDoc.Builder, com.basho.riak.protobuf.RiakSearchPB.RpbSearchDocOrBuilder>(
docs_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
docs_ = null;
}
return docsBuilder_;
}
private float maxScore_ ;
/**
* optional float max_score = 2;
*
*
* Maximum score
*
*/
public boolean hasMaxScore() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional float max_score = 2;
*
*
* Maximum score
*
*/
public float getMaxScore() {
return maxScore_;
}
/**
* optional float max_score = 2;
*
*
* Maximum score
*
*/
public Builder setMaxScore(float value) {
bitField0_ |= 0x00000002;
maxScore_ = value;
onChanged();
return this;
}
/**
* optional float max_score = 2;
*
*
* Maximum score
*
*/
public Builder clearMaxScore() {
bitField0_ = (bitField0_ & ~0x00000002);
maxScore_ = 0F;
onChanged();
return this;
}
private int numFound_ ;
/**
* optional uint32 num_found = 3;
*
*
* Number of results
*
*/
public boolean hasNumFound() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 num_found = 3;
*
*
* Number of results
*
*/
public int getNumFound() {
return numFound_;
}
/**
* optional uint32 num_found = 3;
*
*
* Number of results
*
*/
public Builder setNumFound(int value) {
bitField0_ |= 0x00000004;
numFound_ = value;
onChanged();
return this;
}
/**
* optional uint32 num_found = 3;
*
*
* Number of results
*
*/
public Builder clearNumFound() {
bitField0_ = (bitField0_ & ~0x00000004);
numFound_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:RpbSearchQueryResp)
}
static {
defaultInstance = new RpbSearchQueryResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:RpbSearchQueryResp)
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbSearchDoc_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbSearchDoc_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbSearchQueryReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbSearchQueryReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_RpbSearchQueryResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_RpbSearchQueryResp_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\021riak_search.proto\032\nriak.proto\"(\n\014RpbSe" +
"archDoc\022\030\n\006fields\030\001 \003(\0132\010.RpbPair\"\235\001\n\021Rp" +
"bSearchQueryReq\022\t\n\001q\030\001 \002(\014\022\r\n\005index\030\002 \002(" +
"\014\022\014\n\004rows\030\003 \001(\r\022\r\n\005start\030\004 \001(\r\022\014\n\004sort\030\005" +
" \001(\014\022\016\n\006filter\030\006 \001(\014\022\n\n\002df\030\007 \001(\014\022\n\n\002op\030\010" +
" \001(\014\022\n\n\002fl\030\t \003(\014\022\017\n\007presort\030\n \001(\014\"W\n\022Rpb" +
"SearchQueryResp\022\033\n\004docs\030\001 \003(\0132\r.RpbSearc" +
"hDoc\022\021\n\tmax_score\030\002 \001(\002\022\021\n\tnum_found\030\003 \001" +
"(\rB\'\n\027com.basho.riak.protobufB\014RiakSearc" +
"hPB"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.basho.riak.protobuf.RiakPB.getDescriptor(),
}, assigner);
internal_static_RpbSearchDoc_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_RpbSearchDoc_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbSearchDoc_descriptor,
new java.lang.String[] { "Fields", });
internal_static_RpbSearchQueryReq_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_RpbSearchQueryReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbSearchQueryReq_descriptor,
new java.lang.String[] { "Q", "Index", "Rows", "Start", "Sort", "Filter", "Df", "Op", "Fl", "Presort", });
internal_static_RpbSearchQueryResp_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_RpbSearchQueryResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_RpbSearchQueryResp_descriptor,
new java.lang.String[] { "Docs", "MaxScore", "NumFound", });
com.basho.riak.protobuf.RiakPB.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy