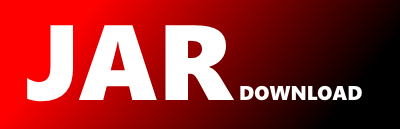
com.basho.riak.protobuf.RiakTsPB Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of riak-client Show documentation
Show all versions of riak-client Show documentation
HttpClient-based client for Riak
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: riak_ts.proto
package com.basho.riak.protobuf;
public final class RiakTsPB {
private RiakTsPB() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
/**
* Protobuf enum {@code TsColumnType}
*/
public enum TsColumnType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* VARCHAR = 0;
*/
VARCHAR(0, 0),
/**
* SINT64 = 1;
*/
SINT64(1, 1),
/**
* DOUBLE = 2;
*/
DOUBLE(2, 2),
/**
* TIMESTAMP = 3;
*/
TIMESTAMP(3, 3),
/**
* BOOLEAN = 4;
*/
BOOLEAN(4, 4),
/**
* BLOB = 5;
*/
BLOB(5, 5),
;
/**
* VARCHAR = 0;
*/
public static final int VARCHAR_VALUE = 0;
/**
* SINT64 = 1;
*/
public static final int SINT64_VALUE = 1;
/**
* DOUBLE = 2;
*/
public static final int DOUBLE_VALUE = 2;
/**
* TIMESTAMP = 3;
*/
public static final int TIMESTAMP_VALUE = 3;
/**
* BOOLEAN = 4;
*/
public static final int BOOLEAN_VALUE = 4;
/**
* BLOB = 5;
*/
public static final int BLOB_VALUE = 5;
public final int getNumber() { return value; }
public static TsColumnType valueOf(int value) {
switch (value) {
case 0: return VARCHAR;
case 1: return SINT64;
case 2: return DOUBLE;
case 3: return TIMESTAMP;
case 4: return BOOLEAN;
case 5: return BLOB;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TsColumnType findValueByNumber(int number) {
return TsColumnType.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.getDescriptor().getEnumTypes().get(0);
}
private static final TsColumnType[] VALUES = values();
public static TsColumnType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private TsColumnType(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:TsColumnType)
}
public interface TsQueryReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsQueryReq)
com.google.protobuf.MessageOrBuilder {
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
boolean hasQuery();
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
com.basho.riak.protobuf.RiakTsPB.TsInterpolation getQuery();
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder getQueryOrBuilder();
/**
* optional bool stream = 2 [default = false];
*/
boolean hasStream();
/**
* optional bool stream = 2 [default = false];
*/
boolean getStream();
/**
* optional bytes cover_context = 3;
*
*
* chopped up coverage plan per-req
*
*/
boolean hasCoverContext();
/**
* optional bytes cover_context = 3;
*
*
* chopped up coverage plan per-req
*
*/
com.google.protobuf.ByteString getCoverContext();
}
/**
* Protobuf type {@code TsQueryReq}
*
*
* Dispatch a query to Riak
*
*/
public static final class TsQueryReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsQueryReq)
TsQueryReqOrBuilder {
// Use TsQueryReq.newBuilder() to construct.
private TsQueryReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsQueryReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsQueryReq defaultInstance;
public static TsQueryReq getDefaultInstance() {
return defaultInstance;
}
public TsQueryReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsQueryReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = query_.toBuilder();
}
query_ = input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsInterpolation.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(query_);
query_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 16: {
bitField0_ |= 0x00000002;
stream_ = input.readBool();
break;
}
case 26: {
bitField0_ |= 0x00000004;
coverContext_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsQueryReq.class, com.basho.riak.protobuf.RiakTsPB.TsQueryReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsQueryReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsQueryReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int QUERY_FIELD_NUMBER = 1;
private com.basho.riak.protobuf.RiakTsPB.TsInterpolation query_;
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public boolean hasQuery() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation getQuery() {
return query_;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder getQueryOrBuilder() {
return query_;
}
public static final int STREAM_FIELD_NUMBER = 2;
private boolean stream_;
/**
* optional bool stream = 2 [default = false];
*/
public boolean hasStream() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool stream = 2 [default = false];
*/
public boolean getStream() {
return stream_;
}
public static final int COVER_CONTEXT_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString coverContext_;
/**
* optional bytes cover_context = 3;
*
*
* chopped up coverage plan per-req
*
*/
public boolean hasCoverContext() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes cover_context = 3;
*
*
* chopped up coverage plan per-req
*
*/
public com.google.protobuf.ByteString getCoverContext() {
return coverContext_;
}
private void initFields() {
query_ = com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
stream_ = false;
coverContext_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasQuery()) {
if (!getQuery().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, query_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(2, stream_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, coverContext_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, query_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, stream_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, coverContext_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsQueryReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsQueryReq}
*
*
* Dispatch a query to Riak
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsQueryReq)
com.basho.riak.protobuf.RiakTsPB.TsQueryReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsQueryReq.class, com.basho.riak.protobuf.RiakTsPB.TsQueryReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsQueryReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getQueryFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (queryBuilder_ == null) {
query_ = com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
} else {
queryBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
stream_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
coverContext_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryReq_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsQueryReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsQueryReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsQueryReq build() {
com.basho.riak.protobuf.RiakTsPB.TsQueryReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsQueryReq buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsQueryReq result = new com.basho.riak.protobuf.RiakTsPB.TsQueryReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (queryBuilder_ == null) {
result.query_ = query_;
} else {
result.query_ = queryBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.stream_ = stream_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.coverContext_ = coverContext_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsQueryReq) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsQueryReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsQueryReq other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsQueryReq.getDefaultInstance()) return this;
if (other.hasQuery()) {
mergeQuery(other.getQuery());
}
if (other.hasStream()) {
setStream(other.getStream());
}
if (other.hasCoverContext()) {
setCoverContext(other.getCoverContext());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (hasQuery()) {
if (!getQuery().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsQueryReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsQueryReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.basho.riak.protobuf.RiakTsPB.TsInterpolation query_ = com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsInterpolation, com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder, com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder> queryBuilder_;
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public boolean hasQuery() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation getQuery() {
if (queryBuilder_ == null) {
return query_;
} else {
return queryBuilder_.getMessage();
}
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public Builder setQuery(com.basho.riak.protobuf.RiakTsPB.TsInterpolation value) {
if (queryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
query_ = value;
onChanged();
} else {
queryBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public Builder setQuery(
com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder builderForValue) {
if (queryBuilder_ == null) {
query_ = builderForValue.build();
onChanged();
} else {
queryBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public Builder mergeQuery(com.basho.riak.protobuf.RiakTsPB.TsInterpolation value) {
if (queryBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
query_ != com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance()) {
query_ =
com.basho.riak.protobuf.RiakTsPB.TsInterpolation.newBuilder(query_).mergeFrom(value).buildPartial();
} else {
query_ = value;
}
onChanged();
} else {
queryBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public Builder clearQuery() {
if (queryBuilder_ == null) {
query_ = com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
onChanged();
} else {
queryBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder getQueryBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getQueryFieldBuilder().getBuilder();
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder getQueryOrBuilder() {
if (queryBuilder_ != null) {
return queryBuilder_.getMessageOrBuilder();
} else {
return query_;
}
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsInterpolation, com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder, com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder>
getQueryFieldBuilder() {
if (queryBuilder_ == null) {
queryBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsInterpolation, com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder, com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder>(
getQuery(),
getParentForChildren(),
isClean());
query_ = null;
}
return queryBuilder_;
}
private boolean stream_ ;
/**
* optional bool stream = 2 [default = false];
*/
public boolean hasStream() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool stream = 2 [default = false];
*/
public boolean getStream() {
return stream_;
}
/**
* optional bool stream = 2 [default = false];
*/
public Builder setStream(boolean value) {
bitField0_ |= 0x00000002;
stream_ = value;
onChanged();
return this;
}
/**
* optional bool stream = 2 [default = false];
*/
public Builder clearStream() {
bitField0_ = (bitField0_ & ~0x00000002);
stream_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString coverContext_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes cover_context = 3;
*
*
* chopped up coverage plan per-req
*
*/
public boolean hasCoverContext() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes cover_context = 3;
*
*
* chopped up coverage plan per-req
*
*/
public com.google.protobuf.ByteString getCoverContext() {
return coverContext_;
}
/**
* optional bytes cover_context = 3;
*
*
* chopped up coverage plan per-req
*
*/
public Builder setCoverContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
coverContext_ = value;
onChanged();
return this;
}
/**
* optional bytes cover_context = 3;
*
*
* chopped up coverage plan per-req
*
*/
public Builder clearCoverContext() {
bitField0_ = (bitField0_ & ~0x00000004);
coverContext_ = getDefaultInstance().getCoverContext();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsQueryReq)
}
static {
defaultInstance = new TsQueryReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsQueryReq)
}
public interface TsQueryRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsQueryResp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .TsColumnDescription columns = 1;
*/
java.util.List
getColumnsList();
/**
* repeated .TsColumnDescription columns = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index);
/**
* repeated .TsColumnDescription columns = 1;
*/
int getColumnsCount();
/**
* repeated .TsColumnDescription columns = 1;
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList();
/**
* repeated .TsColumnDescription columns = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index);
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
java.util.List
getRowsList();
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index);
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
int getRowsCount();
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList();
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index);
/**
* optional bool done = 3 [default = true];
*/
boolean hasDone();
/**
* optional bool done = 3 [default = true];
*/
boolean getDone();
}
/**
* Protobuf type {@code TsQueryResp}
*/
public static final class TsQueryResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsQueryResp)
TsQueryRespOrBuilder {
// Use TsQueryResp.newBuilder() to construct.
private TsQueryResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsQueryResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsQueryResp defaultInstance;
public static TsQueryResp getDefaultInstance() {
return defaultInstance;
}
public TsQueryResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsQueryResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
columns_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.PARSER, extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
rows_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
rows_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsRow.PARSER, extensionRegistry));
break;
}
case 24: {
bitField0_ |= 0x00000001;
done_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
rows_ = java.util.Collections.unmodifiableList(rows_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsQueryResp.class, com.basho.riak.protobuf.RiakTsPB.TsQueryResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsQueryResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsQueryResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int COLUMNS_FIELD_NUMBER = 1;
private java.util.List columns_;
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List getColumnsList() {
return columns_;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public int getColumnsCount() {
return columns_.size();
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index) {
return columns_.get(index);
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
public static final int ROWS_FIELD_NUMBER = 2;
private java.util.List rows_;
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public java.util.List getRowsList() {
return rows_;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList() {
return rows_;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public int getRowsCount() {
return rows_.size();
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index) {
return rows_.get(index);
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index) {
return rows_.get(index);
}
public static final int DONE_FIELD_NUMBER = 3;
private boolean done_;
/**
* optional bool done = 3 [default = true];
*/
public boolean hasDone() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bool done = 3 [default = true];
*/
public boolean getDone() {
return done_;
}
private void initFields() {
columns_ = java.util.Collections.emptyList();
rows_ = java.util.Collections.emptyList();
done_ = true;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(1, columns_.get(i));
}
for (int i = 0; i < rows_.size(); i++) {
output.writeMessage(2, rows_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBool(3, done_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, columns_.get(i));
}
for (int i = 0; i < rows_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, rows_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, done_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsQueryResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsQueryResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsQueryResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsQueryResp)
com.basho.riak.protobuf.RiakTsPB.TsQueryRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsQueryResp.class, com.basho.riak.protobuf.RiakTsPB.TsQueryResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsQueryResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
getRowsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
columnsBuilder_.clear();
}
if (rowsBuilder_ == null) {
rows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
rowsBuilder_.clear();
}
done_ = true;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsQueryResp_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsQueryResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsQueryResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsQueryResp build() {
com.basho.riak.protobuf.RiakTsPB.TsQueryResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsQueryResp buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsQueryResp result = new com.basho.riak.protobuf.RiakTsPB.TsQueryResp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
if (rowsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
rows_ = java.util.Collections.unmodifiableList(rows_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.rows_ = rows_;
} else {
result.rows_ = rowsBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000001;
}
result.done_ = done_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsQueryResp) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsQueryResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsQueryResp other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsQueryResp.getDefaultInstance()) return this;
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
columnsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
if (rowsBuilder_ == null) {
if (!other.rows_.isEmpty()) {
if (rows_.isEmpty()) {
rows_ = other.rows_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureRowsIsMutable();
rows_.addAll(other.rows_);
}
onChanged();
}
} else {
if (!other.rows_.isEmpty()) {
if (rowsBuilder_.isEmpty()) {
rowsBuilder_.dispose();
rowsBuilder_ = null;
rows_ = other.rows_;
bitField0_ = (bitField0_ & ~0x00000002);
rowsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRowsFieldBuilder() : null;
} else {
rowsBuilder_.addAllMessages(other.rows_);
}
}
}
if (other.hasDone()) {
setDone(other.getDone());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsQueryResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsQueryResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder> columnsBuilder_;
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder setColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder setColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addColumns(com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addColumns(
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addAllColumns(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescription> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.getDefaultInstance());
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.getDefaultInstance());
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>(
columns_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
private java.util.List rows_ =
java.util.Collections.emptyList();
private void ensureRowsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
rows_ = new java.util.ArrayList(rows_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder> rowsBuilder_;
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public java.util.List getRowsList() {
if (rowsBuilder_ == null) {
return java.util.Collections.unmodifiableList(rows_);
} else {
return rowsBuilder_.getMessageList();
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public int getRowsCount() {
if (rowsBuilder_ == null) {
return rows_.size();
} else {
return rowsBuilder_.getCount();
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index) {
if (rowsBuilder_ == null) {
return rows_.get(index);
} else {
return rowsBuilder_.getMessage(index);
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder setRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.set(index, value);
onChanged();
} else {
rowsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder setRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.set(index, builderForValue.build());
onChanged();
} else {
rowsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder addRows(com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.add(value);
onChanged();
} else {
rowsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder addRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.add(index, value);
onChanged();
} else {
rowsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder addRows(
com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.add(builderForValue.build());
onChanged();
} else {
rowsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder addRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.add(index, builderForValue.build());
onChanged();
} else {
rowsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder addAllRows(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsRow> values) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rows_);
onChanged();
} else {
rowsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder clearRows() {
if (rowsBuilder_ == null) {
rows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
rowsBuilder_.clear();
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public Builder removeRows(int index) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.remove(index);
onChanged();
} else {
rowsBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder getRowsBuilder(
int index) {
return getRowsFieldBuilder().getBuilder(index);
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index) {
if (rowsBuilder_ == null) {
return rows_.get(index); } else {
return rowsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList() {
if (rowsBuilder_ != null) {
return rowsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rows_);
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder addRowsBuilder() {
return getRowsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance());
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder addRowsBuilder(
int index) {
return getRowsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance());
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 to n rows
*
*/
public java.util.List
getRowsBuilderList() {
return getRowsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsFieldBuilder() {
if (rowsBuilder_ == null) {
rowsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>(
rows_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
rows_ = null;
}
return rowsBuilder_;
}
private boolean done_ = true;
/**
* optional bool done = 3 [default = true];
*/
public boolean hasDone() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool done = 3 [default = true];
*/
public boolean getDone() {
return done_;
}
/**
* optional bool done = 3 [default = true];
*/
public Builder setDone(boolean value) {
bitField0_ |= 0x00000004;
done_ = value;
onChanged();
return this;
}
/**
* optional bool done = 3 [default = true];
*/
public Builder clearDone() {
bitField0_ = (bitField0_ & ~0x00000004);
done_ = true;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsQueryResp)
}
static {
defaultInstance = new TsQueryResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsQueryResp)
}
public interface TsGetReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsGetReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes table = 1;
*/
boolean hasTable();
/**
* required bytes table = 1;
*/
com.google.protobuf.ByteString getTable();
/**
* repeated .TsCell key = 2;
*/
java.util.List
getKeyList();
/**
* repeated .TsCell key = 2;
*/
com.basho.riak.protobuf.RiakTsPB.TsCell getKey(int index);
/**
* repeated .TsCell key = 2;
*/
int getKeyCount();
/**
* repeated .TsCell key = 2;
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getKeyOrBuilderList();
/**
* repeated .TsCell key = 2;
*/
com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getKeyOrBuilder(
int index);
/**
* optional uint32 timeout = 3;
*/
boolean hasTimeout();
/**
* optional uint32 timeout = 3;
*/
int getTimeout();
}
/**
* Protobuf type {@code TsGetReq}
*/
public static final class TsGetReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsGetReq)
TsGetReqOrBuilder {
// Use TsGetReq.newBuilder() to construct.
private TsGetReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsGetReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsGetReq defaultInstance;
public static TsGetReq getDefaultInstance() {
return defaultInstance;
}
public TsGetReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsGetReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
table_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
key_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
key_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsCell.PARSER, extensionRegistry));
break;
}
case 24: {
bitField0_ |= 0x00000002;
timeout_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
key_ = java.util.Collections.unmodifiableList(key_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsGetReq.class, com.basho.riak.protobuf.RiakTsPB.TsGetReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsGetReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsGetReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int TABLE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString table_;
/**
* required bytes table = 1;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes table = 1;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
public static final int KEY_FIELD_NUMBER = 2;
private java.util.List key_;
/**
* repeated .TsCell key = 2;
*/
public java.util.List getKeyList() {
return key_;
}
/**
* repeated .TsCell key = 2;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getKeyOrBuilderList() {
return key_;
}
/**
* repeated .TsCell key = 2;
*/
public int getKeyCount() {
return key_.size();
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell getKey(int index) {
return key_.get(index);
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getKeyOrBuilder(
int index) {
return key_.get(index);
}
public static final int TIMEOUT_FIELD_NUMBER = 3;
private int timeout_;
/**
* optional uint32 timeout = 3;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint32 timeout = 3;
*/
public int getTimeout() {
return timeout_;
}
private void initFields() {
table_ = com.google.protobuf.ByteString.EMPTY;
key_ = java.util.Collections.emptyList();
timeout_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTable()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, table_);
}
for (int i = 0; i < key_.size(); i++) {
output.writeMessage(2, key_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(3, timeout_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, table_);
}
for (int i = 0; i < key_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, key_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, timeout_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsGetReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsGetReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsGetReq)
com.basho.riak.protobuf.RiakTsPB.TsGetReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsGetReq.class, com.basho.riak.protobuf.RiakTsPB.TsGetReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsGetReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getKeyFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
table_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (keyBuilder_ == null) {
key_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
keyBuilder_.clear();
}
timeout_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetReq_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsGetReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsGetReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsGetReq build() {
com.basho.riak.protobuf.RiakTsPB.TsGetReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsGetReq buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsGetReq result = new com.basho.riak.protobuf.RiakTsPB.TsGetReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.table_ = table_;
if (keyBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
key_ = java.util.Collections.unmodifiableList(key_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.timeout_ = timeout_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsGetReq) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsGetReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsGetReq other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsGetReq.getDefaultInstance()) return this;
if (other.hasTable()) {
setTable(other.getTable());
}
if (keyBuilder_ == null) {
if (!other.key_.isEmpty()) {
if (key_.isEmpty()) {
key_ = other.key_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureKeyIsMutable();
key_.addAll(other.key_);
}
onChanged();
}
} else {
if (!other.key_.isEmpty()) {
if (keyBuilder_.isEmpty()) {
keyBuilder_.dispose();
keyBuilder_ = null;
key_ = other.key_;
bitField0_ = (bitField0_ & ~0x00000002);
keyBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getKeyFieldBuilder() : null;
} else {
keyBuilder_.addAllMessages(other.key_);
}
}
}
if (other.hasTimeout()) {
setTimeout(other.getTimeout());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTable()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsGetReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsGetReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString table_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes table = 1;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes table = 1;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
/**
* required bytes table = 1;
*/
public Builder setTable(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
table_ = value;
onChanged();
return this;
}
/**
* required bytes table = 1;
*/
public Builder clearTable() {
bitField0_ = (bitField0_ & ~0x00000001);
table_ = getDefaultInstance().getTable();
onChanged();
return this;
}
private java.util.List key_ =
java.util.Collections.emptyList();
private void ensureKeyIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
key_ = new java.util.ArrayList(key_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder> keyBuilder_;
/**
* repeated .TsCell key = 2;
*/
public java.util.List getKeyList() {
if (keyBuilder_ == null) {
return java.util.Collections.unmodifiableList(key_);
} else {
return keyBuilder_.getMessageList();
}
}
/**
* repeated .TsCell key = 2;
*/
public int getKeyCount() {
if (keyBuilder_ == null) {
return key_.size();
} else {
return keyBuilder_.getCount();
}
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell getKey(int index) {
if (keyBuilder_ == null) {
return key_.get(index);
} else {
return keyBuilder_.getMessage(index);
}
}
/**
* repeated .TsCell key = 2;
*/
public Builder setKey(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.set(index, value);
onChanged();
} else {
keyBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder setKey(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.set(index, builderForValue.build());
onChanged();
} else {
keyBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addKey(com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.add(value);
onChanged();
} else {
keyBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addKey(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.add(index, value);
onChanged();
} else {
keyBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addKey(
com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.add(builderForValue.build());
onChanged();
} else {
keyBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addKey(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.add(index, builderForValue.build());
onChanged();
} else {
keyBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addAllKey(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsCell> values) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, key_);
onChanged();
} else {
keyBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
keyBuilder_.clear();
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder removeKey(int index) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.remove(index);
onChanged();
} else {
keyBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder getKeyBuilder(
int index) {
return getKeyFieldBuilder().getBuilder(index);
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getKeyOrBuilder(
int index) {
if (keyBuilder_ == null) {
return key_.get(index); } else {
return keyBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsCell key = 2;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getKeyOrBuilderList() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(key_);
}
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder addKeyBuilder() {
return getKeyFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsCell.getDefaultInstance());
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder addKeyBuilder(
int index) {
return getKeyFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsCell.getDefaultInstance());
}
/**
* repeated .TsCell key = 2;
*/
public java.util.List
getKeyBuilderList() {
return getKeyFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>(
key_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private int timeout_ ;
/**
* optional uint32 timeout = 3;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 timeout = 3;
*/
public int getTimeout() {
return timeout_;
}
/**
* optional uint32 timeout = 3;
*/
public Builder setTimeout(int value) {
bitField0_ |= 0x00000004;
timeout_ = value;
onChanged();
return this;
}
/**
* optional uint32 timeout = 3;
*/
public Builder clearTimeout() {
bitField0_ = (bitField0_ & ~0x00000004);
timeout_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsGetReq)
}
static {
defaultInstance = new TsGetReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsGetReq)
}
public interface TsGetRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsGetResp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .TsColumnDescription columns = 1;
*/
java.util.List
getColumnsList();
/**
* repeated .TsColumnDescription columns = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index);
/**
* repeated .TsColumnDescription columns = 1;
*/
int getColumnsCount();
/**
* repeated .TsColumnDescription columns = 1;
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList();
/**
* repeated .TsColumnDescription columns = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index);
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
java.util.List
getRowsList();
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index);
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
int getRowsCount();
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList();
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index);
}
/**
* Protobuf type {@code TsGetResp}
*/
public static final class TsGetResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsGetResp)
TsGetRespOrBuilder {
// Use TsGetResp.newBuilder() to construct.
private TsGetResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsGetResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsGetResp defaultInstance;
public static TsGetResp getDefaultInstance() {
return defaultInstance;
}
public TsGetResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsGetResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
columns_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.PARSER, extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
rows_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
rows_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsRow.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
rows_ = java.util.Collections.unmodifiableList(rows_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsGetResp.class, com.basho.riak.protobuf.RiakTsPB.TsGetResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsGetResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsGetResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int COLUMNS_FIELD_NUMBER = 1;
private java.util.List columns_;
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List getColumnsList() {
return columns_;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public int getColumnsCount() {
return columns_.size();
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index) {
return columns_.get(index);
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
public static final int ROWS_FIELD_NUMBER = 2;
private java.util.List rows_;
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public java.util.List getRowsList() {
return rows_;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList() {
return rows_;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public int getRowsCount() {
return rows_.size();
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index) {
return rows_.get(index);
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index) {
return rows_.get(index);
}
private void initFields() {
columns_ = java.util.Collections.emptyList();
rows_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(1, columns_.get(i));
}
for (int i = 0; i < rows_.size(); i++) {
output.writeMessage(2, rows_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, columns_.get(i));
}
for (int i = 0; i < rows_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, rows_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsGetResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsGetResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsGetResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsGetResp)
com.basho.riak.protobuf.RiakTsPB.TsGetRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsGetResp.class, com.basho.riak.protobuf.RiakTsPB.TsGetResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsGetResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
getRowsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
columnsBuilder_.clear();
}
if (rowsBuilder_ == null) {
rows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
rowsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsGetResp_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsGetResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsGetResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsGetResp build() {
com.basho.riak.protobuf.RiakTsPB.TsGetResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsGetResp buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsGetResp result = new com.basho.riak.protobuf.RiakTsPB.TsGetResp(this);
int from_bitField0_ = bitField0_;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
if (rowsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
rows_ = java.util.Collections.unmodifiableList(rows_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.rows_ = rows_;
} else {
result.rows_ = rowsBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsGetResp) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsGetResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsGetResp other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsGetResp.getDefaultInstance()) return this;
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
columnsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
if (rowsBuilder_ == null) {
if (!other.rows_.isEmpty()) {
if (rows_.isEmpty()) {
rows_ = other.rows_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureRowsIsMutable();
rows_.addAll(other.rows_);
}
onChanged();
}
} else {
if (!other.rows_.isEmpty()) {
if (rowsBuilder_.isEmpty()) {
rowsBuilder_.dispose();
rowsBuilder_ = null;
rows_ = other.rows_;
bitField0_ = (bitField0_ & ~0x00000002);
rowsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRowsFieldBuilder() : null;
} else {
rowsBuilder_.addAllMessages(other.rows_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsGetResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsGetResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder> columnsBuilder_;
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder setColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder setColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addColumns(com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addColumns(
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder addAllColumns(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescription> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.getDefaultInstance());
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.getDefaultInstance());
}
/**
* repeated .TsColumnDescription columns = 1;
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>(
columns_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
private java.util.List rows_ =
java.util.Collections.emptyList();
private void ensureRowsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
rows_ = new java.util.ArrayList(rows_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder> rowsBuilder_;
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public java.util.List getRowsList() {
if (rowsBuilder_ == null) {
return java.util.Collections.unmodifiableList(rows_);
} else {
return rowsBuilder_.getMessageList();
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public int getRowsCount() {
if (rowsBuilder_ == null) {
return rows_.size();
} else {
return rowsBuilder_.getCount();
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index) {
if (rowsBuilder_ == null) {
return rows_.get(index);
} else {
return rowsBuilder_.getMessage(index);
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder setRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.set(index, value);
onChanged();
} else {
rowsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder setRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.set(index, builderForValue.build());
onChanged();
} else {
rowsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder addRows(com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.add(value);
onChanged();
} else {
rowsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder addRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.add(index, value);
onChanged();
} else {
rowsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder addRows(
com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.add(builderForValue.build());
onChanged();
} else {
rowsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder addRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.add(index, builderForValue.build());
onChanged();
} else {
rowsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder addAllRows(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsRow> values) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rows_);
onChanged();
} else {
rowsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder clearRows() {
if (rowsBuilder_ == null) {
rows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
rowsBuilder_.clear();
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public Builder removeRows(int index) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.remove(index);
onChanged();
} else {
rowsBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder getRowsBuilder(
int index) {
return getRowsFieldBuilder().getBuilder(index);
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index) {
if (rowsBuilder_ == null) {
return rows_.get(index); } else {
return rowsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList() {
if (rowsBuilder_ != null) {
return rowsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rows_);
}
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder addRowsBuilder() {
return getRowsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance());
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder addRowsBuilder(
int index) {
return getRowsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance());
}
/**
* repeated .TsRow rows = 2;
*
*
* 0 or 1 rows
*
*/
public java.util.List
getRowsBuilderList() {
return getRowsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsFieldBuilder() {
if (rowsBuilder_ == null) {
rowsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>(
rows_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
rows_ = null;
}
return rowsBuilder_;
}
// @@protoc_insertion_point(builder_scope:TsGetResp)
}
static {
defaultInstance = new TsGetResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsGetResp)
}
public interface TsPutReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsPutReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes table = 1;
*/
boolean hasTable();
/**
* required bytes table = 1;
*/
com.google.protobuf.ByteString getTable();
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
java.util.List
getColumnsList();
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index);
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
int getColumnsCount();
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList();
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index);
/**
* repeated .TsRow rows = 3;
*/
java.util.List
getRowsList();
/**
* repeated .TsRow rows = 3;
*/
com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index);
/**
* repeated .TsRow rows = 3;
*/
int getRowsCount();
/**
* repeated .TsRow rows = 3;
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList();
/**
* repeated .TsRow rows = 3;
*/
com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index);
}
/**
* Protobuf type {@code TsPutReq}
*/
public static final class TsPutReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsPutReq)
TsPutReqOrBuilder {
// Use TsPutReq.newBuilder() to construct.
private TsPutReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsPutReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsPutReq defaultInstance;
public static TsPutReq getDefaultInstance() {
return defaultInstance;
}
public TsPutReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsPutReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
table_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
columns_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.PARSER, extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
rows_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
rows_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsRow.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
rows_ = java.util.Collections.unmodifiableList(rows_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsPutReq.class, com.basho.riak.protobuf.RiakTsPB.TsPutReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsPutReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsPutReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int TABLE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString table_;
/**
* required bytes table = 1;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes table = 1;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
public static final int COLUMNS_FIELD_NUMBER = 2;
private java.util.List columns_;
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public java.util.List getColumnsList() {
return columns_;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public int getColumnsCount() {
return columns_.size();
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index) {
return columns_.get(index);
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
public static final int ROWS_FIELD_NUMBER = 3;
private java.util.List rows_;
/**
* repeated .TsRow rows = 3;
*/
public java.util.List getRowsList() {
return rows_;
}
/**
* repeated .TsRow rows = 3;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList() {
return rows_;
}
/**
* repeated .TsRow rows = 3;
*/
public int getRowsCount() {
return rows_.size();
}
/**
* repeated .TsRow rows = 3;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index) {
return rows_.get(index);
}
/**
* repeated .TsRow rows = 3;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index) {
return rows_.get(index);
}
private void initFields() {
table_ = com.google.protobuf.ByteString.EMPTY;
columns_ = java.util.Collections.emptyList();
rows_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTable()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, table_);
}
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(2, columns_.get(i));
}
for (int i = 0; i < rows_.size(); i++) {
output.writeMessage(3, rows_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, table_);
}
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, columns_.get(i));
}
for (int i = 0; i < rows_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, rows_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsPutReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsPutReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsPutReq)
com.basho.riak.protobuf.RiakTsPB.TsPutReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsPutReq.class, com.basho.riak.protobuf.RiakTsPB.TsPutReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsPutReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
getRowsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
table_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
columnsBuilder_.clear();
}
if (rowsBuilder_ == null) {
rows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
rowsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutReq_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsPutReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsPutReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsPutReq build() {
com.basho.riak.protobuf.RiakTsPB.TsPutReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsPutReq buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsPutReq result = new com.basho.riak.protobuf.RiakTsPB.TsPutReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.table_ = table_;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
if (rowsBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
rows_ = java.util.Collections.unmodifiableList(rows_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.rows_ = rows_;
} else {
result.rows_ = rowsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsPutReq) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsPutReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsPutReq other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsPutReq.getDefaultInstance()) return this;
if (other.hasTable()) {
setTable(other.getTable());
}
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000002);
columnsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
if (rowsBuilder_ == null) {
if (!other.rows_.isEmpty()) {
if (rows_.isEmpty()) {
rows_ = other.rows_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureRowsIsMutable();
rows_.addAll(other.rows_);
}
onChanged();
}
} else {
if (!other.rows_.isEmpty()) {
if (rowsBuilder_.isEmpty()) {
rowsBuilder_.dispose();
rowsBuilder_ = null;
rows_ = other.rows_;
bitField0_ = (bitField0_ & ~0x00000004);
rowsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRowsFieldBuilder() : null;
} else {
rowsBuilder_.addAllMessages(other.rows_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTable()) {
return false;
}
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsPutReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsPutReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString table_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes table = 1;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes table = 1;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
/**
* required bytes table = 1;
*/
public Builder setTable(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
table_ = value;
onChanged();
return this;
}
/**
* required bytes table = 1;
*/
public Builder clearTable() {
bitField0_ = (bitField0_ & ~0x00000001);
table_ = getDefaultInstance().getTable();
onChanged();
return this;
}
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder> columnsBuilder_;
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder setColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder setColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder addColumns(com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder addColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder addColumns(
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder addColumns(
int index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder addAllColumns(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescription> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.getDefaultInstance());
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.getDefaultInstance());
}
/**
* repeated .TsColumnDescription columns = 2;
*
*
* optional: omitting it should use table order
*
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder, com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder>(
columns_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
private java.util.List rows_ =
java.util.Collections.emptyList();
private void ensureRowsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
rows_ = new java.util.ArrayList(rows_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder> rowsBuilder_;
/**
* repeated .TsRow rows = 3;
*/
public java.util.List getRowsList() {
if (rowsBuilder_ == null) {
return java.util.Collections.unmodifiableList(rows_);
} else {
return rowsBuilder_.getMessageList();
}
}
/**
* repeated .TsRow rows = 3;
*/
public int getRowsCount() {
if (rowsBuilder_ == null) {
return rows_.size();
} else {
return rowsBuilder_.getCount();
}
}
/**
* repeated .TsRow rows = 3;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow getRows(int index) {
if (rowsBuilder_ == null) {
return rows_.get(index);
} else {
return rowsBuilder_.getMessage(index);
}
}
/**
* repeated .TsRow rows = 3;
*/
public Builder setRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.set(index, value);
onChanged();
} else {
rowsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public Builder setRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.set(index, builderForValue.build());
onChanged();
} else {
rowsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public Builder addRows(com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.add(value);
onChanged();
} else {
rowsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public Builder addRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (rowsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRowsIsMutable();
rows_.add(index, value);
onChanged();
} else {
rowsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public Builder addRows(
com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.add(builderForValue.build());
onChanged();
} else {
rowsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public Builder addRows(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.add(index, builderForValue.build());
onChanged();
} else {
rowsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public Builder addAllRows(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsRow> values) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rows_);
onChanged();
} else {
rowsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public Builder clearRows() {
if (rowsBuilder_ == null) {
rows_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
rowsBuilder_.clear();
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public Builder removeRows(int index) {
if (rowsBuilder_ == null) {
ensureRowsIsMutable();
rows_.remove(index);
onChanged();
} else {
rowsBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsRow rows = 3;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder getRowsBuilder(
int index) {
return getRowsFieldBuilder().getBuilder(index);
}
/**
* repeated .TsRow rows = 3;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getRowsOrBuilder(
int index) {
if (rowsBuilder_ == null) {
return rows_.get(index); } else {
return rowsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsRow rows = 3;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsOrBuilderList() {
if (rowsBuilder_ != null) {
return rowsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rows_);
}
}
/**
* repeated .TsRow rows = 3;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder addRowsBuilder() {
return getRowsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance());
}
/**
* repeated .TsRow rows = 3;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder addRowsBuilder(
int index) {
return getRowsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance());
}
/**
* repeated .TsRow rows = 3;
*/
public java.util.List
getRowsBuilderList() {
return getRowsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getRowsFieldBuilder() {
if (rowsBuilder_ == null) {
rowsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>(
rows_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
rows_ = null;
}
return rowsBuilder_;
}
// @@protoc_insertion_point(builder_scope:TsPutReq)
}
static {
defaultInstance = new TsPutReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsPutReq)
}
public interface TsPutRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsPutResp)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code TsPutResp}
*/
public static final class TsPutResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsPutResp)
TsPutRespOrBuilder {
// Use TsPutResp.newBuilder() to construct.
private TsPutResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsPutResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsPutResp defaultInstance;
public static TsPutResp getDefaultInstance() {
return defaultInstance;
}
public TsPutResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsPutResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsPutResp.class, com.basho.riak.protobuf.RiakTsPB.TsPutResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsPutResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsPutResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private void initFields() {
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsPutResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsPutResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsPutResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsPutResp)
com.basho.riak.protobuf.RiakTsPB.TsPutRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsPutResp.class, com.basho.riak.protobuf.RiakTsPB.TsPutResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsPutResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsPutResp_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsPutResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsPutResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsPutResp build() {
com.basho.riak.protobuf.RiakTsPB.TsPutResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsPutResp buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsPutResp result = new com.basho.riak.protobuf.RiakTsPB.TsPutResp(this);
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsPutResp) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsPutResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsPutResp other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsPutResp.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsPutResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsPutResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
// @@protoc_insertion_point(builder_scope:TsPutResp)
}
static {
defaultInstance = new TsPutResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsPutResp)
}
public interface TsDelReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsDelReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes table = 1;
*/
boolean hasTable();
/**
* required bytes table = 1;
*/
com.google.protobuf.ByteString getTable();
/**
* repeated .TsCell key = 2;
*/
java.util.List
getKeyList();
/**
* repeated .TsCell key = 2;
*/
com.basho.riak.protobuf.RiakTsPB.TsCell getKey(int index);
/**
* repeated .TsCell key = 2;
*/
int getKeyCount();
/**
* repeated .TsCell key = 2;
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getKeyOrBuilderList();
/**
* repeated .TsCell key = 2;
*/
com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getKeyOrBuilder(
int index);
/**
* optional bytes vclock = 3;
*/
boolean hasVclock();
/**
* optional bytes vclock = 3;
*/
com.google.protobuf.ByteString getVclock();
/**
* optional uint32 timeout = 4;
*/
boolean hasTimeout();
/**
* optional uint32 timeout = 4;
*/
int getTimeout();
}
/**
* Protobuf type {@code TsDelReq}
*/
public static final class TsDelReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsDelReq)
TsDelReqOrBuilder {
// Use TsDelReq.newBuilder() to construct.
private TsDelReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsDelReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsDelReq defaultInstance;
public static TsDelReq getDefaultInstance() {
return defaultInstance;
}
public TsDelReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsDelReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
table_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
key_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
key_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsCell.PARSER, extensionRegistry));
break;
}
case 26: {
bitField0_ |= 0x00000002;
vclock_ = input.readBytes();
break;
}
case 32: {
bitField0_ |= 0x00000004;
timeout_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
key_ = java.util.Collections.unmodifiableList(key_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsDelReq.class, com.basho.riak.protobuf.RiakTsPB.TsDelReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsDelReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsDelReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int TABLE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString table_;
/**
* required bytes table = 1;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes table = 1;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
public static final int KEY_FIELD_NUMBER = 2;
private java.util.List key_;
/**
* repeated .TsCell key = 2;
*/
public java.util.List getKeyList() {
return key_;
}
/**
* repeated .TsCell key = 2;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getKeyOrBuilderList() {
return key_;
}
/**
* repeated .TsCell key = 2;
*/
public int getKeyCount() {
return key_.size();
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell getKey(int index) {
return key_.get(index);
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getKeyOrBuilder(
int index) {
return key_.get(index);
}
public static final int VCLOCK_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString vclock_;
/**
* optional bytes vclock = 3;
*/
public boolean hasVclock() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bytes vclock = 3;
*/
public com.google.protobuf.ByteString getVclock() {
return vclock_;
}
public static final int TIMEOUT_FIELD_NUMBER = 4;
private int timeout_;
/**
* optional uint32 timeout = 4;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 timeout = 4;
*/
public int getTimeout() {
return timeout_;
}
private void initFields() {
table_ = com.google.protobuf.ByteString.EMPTY;
key_ = java.util.Collections.emptyList();
vclock_ = com.google.protobuf.ByteString.EMPTY;
timeout_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTable()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, table_);
}
for (int i = 0; i < key_.size(); i++) {
output.writeMessage(2, key_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(3, vclock_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt32(4, timeout_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, table_);
}
for (int i = 0; i < key_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, key_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, vclock_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, timeout_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsDelReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsDelReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsDelReq)
com.basho.riak.protobuf.RiakTsPB.TsDelReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsDelReq.class, com.basho.riak.protobuf.RiakTsPB.TsDelReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsDelReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getKeyFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
table_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (keyBuilder_ == null) {
key_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
keyBuilder_.clear();
}
vclock_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
timeout_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelReq_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsDelReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsDelReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsDelReq build() {
com.basho.riak.protobuf.RiakTsPB.TsDelReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsDelReq buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsDelReq result = new com.basho.riak.protobuf.RiakTsPB.TsDelReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.table_ = table_;
if (keyBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
key_ = java.util.Collections.unmodifiableList(key_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.vclock_ = vclock_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.timeout_ = timeout_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsDelReq) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsDelReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsDelReq other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsDelReq.getDefaultInstance()) return this;
if (other.hasTable()) {
setTable(other.getTable());
}
if (keyBuilder_ == null) {
if (!other.key_.isEmpty()) {
if (key_.isEmpty()) {
key_ = other.key_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureKeyIsMutable();
key_.addAll(other.key_);
}
onChanged();
}
} else {
if (!other.key_.isEmpty()) {
if (keyBuilder_.isEmpty()) {
keyBuilder_.dispose();
keyBuilder_ = null;
key_ = other.key_;
bitField0_ = (bitField0_ & ~0x00000002);
keyBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getKeyFieldBuilder() : null;
} else {
keyBuilder_.addAllMessages(other.key_);
}
}
}
if (other.hasVclock()) {
setVclock(other.getVclock());
}
if (other.hasTimeout()) {
setTimeout(other.getTimeout());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTable()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsDelReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsDelReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString table_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes table = 1;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes table = 1;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
/**
* required bytes table = 1;
*/
public Builder setTable(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
table_ = value;
onChanged();
return this;
}
/**
* required bytes table = 1;
*/
public Builder clearTable() {
bitField0_ = (bitField0_ & ~0x00000001);
table_ = getDefaultInstance().getTable();
onChanged();
return this;
}
private java.util.List key_ =
java.util.Collections.emptyList();
private void ensureKeyIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
key_ = new java.util.ArrayList(key_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder> keyBuilder_;
/**
* repeated .TsCell key = 2;
*/
public java.util.List getKeyList() {
if (keyBuilder_ == null) {
return java.util.Collections.unmodifiableList(key_);
} else {
return keyBuilder_.getMessageList();
}
}
/**
* repeated .TsCell key = 2;
*/
public int getKeyCount() {
if (keyBuilder_ == null) {
return key_.size();
} else {
return keyBuilder_.getCount();
}
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell getKey(int index) {
if (keyBuilder_ == null) {
return key_.get(index);
} else {
return keyBuilder_.getMessage(index);
}
}
/**
* repeated .TsCell key = 2;
*/
public Builder setKey(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.set(index, value);
onChanged();
} else {
keyBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder setKey(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.set(index, builderForValue.build());
onChanged();
} else {
keyBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addKey(com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.add(value);
onChanged();
} else {
keyBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addKey(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyIsMutable();
key_.add(index, value);
onChanged();
} else {
keyBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addKey(
com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.add(builderForValue.build());
onChanged();
} else {
keyBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addKey(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.add(index, builderForValue.build());
onChanged();
} else {
keyBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder addAllKey(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsCell> values) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, key_);
onChanged();
} else {
keyBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
keyBuilder_.clear();
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public Builder removeKey(int index) {
if (keyBuilder_ == null) {
ensureKeyIsMutable();
key_.remove(index);
onChanged();
} else {
keyBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder getKeyBuilder(
int index) {
return getKeyFieldBuilder().getBuilder(index);
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getKeyOrBuilder(
int index) {
if (keyBuilder_ == null) {
return key_.get(index); } else {
return keyBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsCell key = 2;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getKeyOrBuilderList() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(key_);
}
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder addKeyBuilder() {
return getKeyFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsCell.getDefaultInstance());
}
/**
* repeated .TsCell key = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder addKeyBuilder(
int index) {
return getKeyFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsCell.getDefaultInstance());
}
/**
* repeated .TsCell key = 2;
*/
public java.util.List
getKeyBuilderList() {
return getKeyFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>(
key_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
private com.google.protobuf.ByteString vclock_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes vclock = 3;
*/
public boolean hasVclock() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes vclock = 3;
*/
public com.google.protobuf.ByteString getVclock() {
return vclock_;
}
/**
* optional bytes vclock = 3;
*/
public Builder setVclock(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
vclock_ = value;
onChanged();
return this;
}
/**
* optional bytes vclock = 3;
*/
public Builder clearVclock() {
bitField0_ = (bitField0_ & ~0x00000004);
vclock_ = getDefaultInstance().getVclock();
onChanged();
return this;
}
private int timeout_ ;
/**
* optional uint32 timeout = 4;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 timeout = 4;
*/
public int getTimeout() {
return timeout_;
}
/**
* optional uint32 timeout = 4;
*/
public Builder setTimeout(int value) {
bitField0_ |= 0x00000008;
timeout_ = value;
onChanged();
return this;
}
/**
* optional uint32 timeout = 4;
*/
public Builder clearTimeout() {
bitField0_ = (bitField0_ & ~0x00000008);
timeout_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsDelReq)
}
static {
defaultInstance = new TsDelReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsDelReq)
}
public interface TsDelRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsDelResp)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code TsDelResp}
*/
public static final class TsDelResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsDelResp)
TsDelRespOrBuilder {
// Use TsDelResp.newBuilder() to construct.
private TsDelResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsDelResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsDelResp defaultInstance;
public static TsDelResp getDefaultInstance() {
return defaultInstance;
}
public TsDelResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsDelResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsDelResp.class, com.basho.riak.protobuf.RiakTsPB.TsDelResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsDelResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsDelResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private void initFields() {
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsDelResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsDelResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsDelResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsDelResp)
com.basho.riak.protobuf.RiakTsPB.TsDelRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsDelResp.class, com.basho.riak.protobuf.RiakTsPB.TsDelResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsDelResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsDelResp_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsDelResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsDelResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsDelResp build() {
com.basho.riak.protobuf.RiakTsPB.TsDelResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsDelResp buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsDelResp result = new com.basho.riak.protobuf.RiakTsPB.TsDelResp(this);
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsDelResp) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsDelResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsDelResp other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsDelResp.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsDelResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsDelResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
// @@protoc_insertion_point(builder_scope:TsDelResp)
}
static {
defaultInstance = new TsDelResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsDelResp)
}
public interface TsInterpolationOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsInterpolation)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes base = 1;
*/
boolean hasBase();
/**
* required bytes base = 1;
*/
com.google.protobuf.ByteString getBase();
/**
* repeated .RpbPair interpolations = 2;
*/
java.util.List
getInterpolationsList();
/**
* repeated .RpbPair interpolations = 2;
*/
com.basho.riak.protobuf.RiakPB.RpbPair getInterpolations(int index);
/**
* repeated .RpbPair interpolations = 2;
*/
int getInterpolationsCount();
/**
* repeated .RpbPair interpolations = 2;
*/
java.util.List extends com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>
getInterpolationsOrBuilderList();
/**
* repeated .RpbPair interpolations = 2;
*/
com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder getInterpolationsOrBuilder(
int index);
}
/**
* Protobuf type {@code TsInterpolation}
*/
public static final class TsInterpolation extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsInterpolation)
TsInterpolationOrBuilder {
// Use TsInterpolation.newBuilder() to construct.
private TsInterpolation(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsInterpolation(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsInterpolation defaultInstance;
public static TsInterpolation getDefaultInstance() {
return defaultInstance;
}
public TsInterpolation getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsInterpolation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
base_ = input.readBytes();
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
interpolations_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
interpolations_.add(input.readMessage(com.basho.riak.protobuf.RiakPB.RpbPair.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
interpolations_ = java.util.Collections.unmodifiableList(interpolations_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsInterpolation_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsInterpolation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsInterpolation.class, com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsInterpolation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsInterpolation(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int BASE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString base_;
/**
* required bytes base = 1;
*/
public boolean hasBase() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes base = 1;
*/
public com.google.protobuf.ByteString getBase() {
return base_;
}
public static final int INTERPOLATIONS_FIELD_NUMBER = 2;
private java.util.List interpolations_;
/**
* repeated .RpbPair interpolations = 2;
*/
public java.util.List getInterpolationsList() {
return interpolations_;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public java.util.List extends com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>
getInterpolationsOrBuilderList() {
return interpolations_;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public int getInterpolationsCount() {
return interpolations_.size();
}
/**
* repeated .RpbPair interpolations = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair getInterpolations(int index) {
return interpolations_.get(index);
}
/**
* repeated .RpbPair interpolations = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder getInterpolationsOrBuilder(
int index) {
return interpolations_.get(index);
}
private void initFields() {
base_ = com.google.protobuf.ByteString.EMPTY;
interpolations_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasBase()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getInterpolationsCount(); i++) {
if (!getInterpolations(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, base_);
}
for (int i = 0; i < interpolations_.size(); i++) {
output.writeMessage(2, interpolations_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, base_);
}
for (int i = 0; i < interpolations_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, interpolations_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsInterpolation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsInterpolation prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsInterpolation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsInterpolation)
com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsInterpolation_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsInterpolation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsInterpolation.class, com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsInterpolation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getInterpolationsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
base_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (interpolationsBuilder_ == null) {
interpolations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
interpolationsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsInterpolation_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation build() {
com.basho.riak.protobuf.RiakTsPB.TsInterpolation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsInterpolation result = new com.basho.riak.protobuf.RiakTsPB.TsInterpolation(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.base_ = base_;
if (interpolationsBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
interpolations_ = java.util.Collections.unmodifiableList(interpolations_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.interpolations_ = interpolations_;
} else {
result.interpolations_ = interpolationsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsInterpolation) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsInterpolation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsInterpolation other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance()) return this;
if (other.hasBase()) {
setBase(other.getBase());
}
if (interpolationsBuilder_ == null) {
if (!other.interpolations_.isEmpty()) {
if (interpolations_.isEmpty()) {
interpolations_ = other.interpolations_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureInterpolationsIsMutable();
interpolations_.addAll(other.interpolations_);
}
onChanged();
}
} else {
if (!other.interpolations_.isEmpty()) {
if (interpolationsBuilder_.isEmpty()) {
interpolationsBuilder_.dispose();
interpolationsBuilder_ = null;
interpolations_ = other.interpolations_;
bitField0_ = (bitField0_ & ~0x00000002);
interpolationsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getInterpolationsFieldBuilder() : null;
} else {
interpolationsBuilder_.addAllMessages(other.interpolations_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasBase()) {
return false;
}
for (int i = 0; i < getInterpolationsCount(); i++) {
if (!getInterpolations(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsInterpolation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsInterpolation) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString base_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes base = 1;
*/
public boolean hasBase() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes base = 1;
*/
public com.google.protobuf.ByteString getBase() {
return base_;
}
/**
* required bytes base = 1;
*/
public Builder setBase(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
base_ = value;
onChanged();
return this;
}
/**
* required bytes base = 1;
*/
public Builder clearBase() {
bitField0_ = (bitField0_ & ~0x00000001);
base_ = getDefaultInstance().getBase();
onChanged();
return this;
}
private java.util.List interpolations_ =
java.util.Collections.emptyList();
private void ensureInterpolationsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
interpolations_ = new java.util.ArrayList(interpolations_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbPair, com.basho.riak.protobuf.RiakPB.RpbPair.Builder, com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder> interpolationsBuilder_;
/**
* repeated .RpbPair interpolations = 2;
*/
public java.util.List getInterpolationsList() {
if (interpolationsBuilder_ == null) {
return java.util.Collections.unmodifiableList(interpolations_);
} else {
return interpolationsBuilder_.getMessageList();
}
}
/**
* repeated .RpbPair interpolations = 2;
*/
public int getInterpolationsCount() {
if (interpolationsBuilder_ == null) {
return interpolations_.size();
} else {
return interpolationsBuilder_.getCount();
}
}
/**
* repeated .RpbPair interpolations = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair getInterpolations(int index) {
if (interpolationsBuilder_ == null) {
return interpolations_.get(index);
} else {
return interpolationsBuilder_.getMessage(index);
}
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder setInterpolations(
int index, com.basho.riak.protobuf.RiakPB.RpbPair value) {
if (interpolationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInterpolationsIsMutable();
interpolations_.set(index, value);
onChanged();
} else {
interpolationsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder setInterpolations(
int index, com.basho.riak.protobuf.RiakPB.RpbPair.Builder builderForValue) {
if (interpolationsBuilder_ == null) {
ensureInterpolationsIsMutable();
interpolations_.set(index, builderForValue.build());
onChanged();
} else {
interpolationsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder addInterpolations(com.basho.riak.protobuf.RiakPB.RpbPair value) {
if (interpolationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInterpolationsIsMutable();
interpolations_.add(value);
onChanged();
} else {
interpolationsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder addInterpolations(
int index, com.basho.riak.protobuf.RiakPB.RpbPair value) {
if (interpolationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInterpolationsIsMutable();
interpolations_.add(index, value);
onChanged();
} else {
interpolationsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder addInterpolations(
com.basho.riak.protobuf.RiakPB.RpbPair.Builder builderForValue) {
if (interpolationsBuilder_ == null) {
ensureInterpolationsIsMutable();
interpolations_.add(builderForValue.build());
onChanged();
} else {
interpolationsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder addInterpolations(
int index, com.basho.riak.protobuf.RiakPB.RpbPair.Builder builderForValue) {
if (interpolationsBuilder_ == null) {
ensureInterpolationsIsMutable();
interpolations_.add(index, builderForValue.build());
onChanged();
} else {
interpolationsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder addAllInterpolations(
java.lang.Iterable extends com.basho.riak.protobuf.RiakPB.RpbPair> values) {
if (interpolationsBuilder_ == null) {
ensureInterpolationsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, interpolations_);
onChanged();
} else {
interpolationsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder clearInterpolations() {
if (interpolationsBuilder_ == null) {
interpolations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
interpolationsBuilder_.clear();
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public Builder removeInterpolations(int index) {
if (interpolationsBuilder_ == null) {
ensureInterpolationsIsMutable();
interpolations_.remove(index);
onChanged();
} else {
interpolationsBuilder_.remove(index);
}
return this;
}
/**
* repeated .RpbPair interpolations = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair.Builder getInterpolationsBuilder(
int index) {
return getInterpolationsFieldBuilder().getBuilder(index);
}
/**
* repeated .RpbPair interpolations = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder getInterpolationsOrBuilder(
int index) {
if (interpolationsBuilder_ == null) {
return interpolations_.get(index); } else {
return interpolationsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .RpbPair interpolations = 2;
*/
public java.util.List extends com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>
getInterpolationsOrBuilderList() {
if (interpolationsBuilder_ != null) {
return interpolationsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(interpolations_);
}
}
/**
* repeated .RpbPair interpolations = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair.Builder addInterpolationsBuilder() {
return getInterpolationsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakPB.RpbPair.getDefaultInstance());
}
/**
* repeated .RpbPair interpolations = 2;
*/
public com.basho.riak.protobuf.RiakPB.RpbPair.Builder addInterpolationsBuilder(
int index) {
return getInterpolationsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakPB.RpbPair.getDefaultInstance());
}
/**
* repeated .RpbPair interpolations = 2;
*/
public java.util.List
getInterpolationsBuilderList() {
return getInterpolationsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbPair, com.basho.riak.protobuf.RiakPB.RpbPair.Builder, com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>
getInterpolationsFieldBuilder() {
if (interpolationsBuilder_ == null) {
interpolationsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakPB.RpbPair, com.basho.riak.protobuf.RiakPB.RpbPair.Builder, com.basho.riak.protobuf.RiakPB.RpbPairOrBuilder>(
interpolations_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
interpolations_ = null;
}
return interpolationsBuilder_;
}
// @@protoc_insertion_point(builder_scope:TsInterpolation)
}
static {
defaultInstance = new TsInterpolation(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsInterpolation)
}
public interface TsColumnDescriptionOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsColumnDescription)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes name = 1;
*/
boolean hasName();
/**
* required bytes name = 1;
*/
com.google.protobuf.ByteString getName();
/**
* required .TsColumnType type = 2;
*/
boolean hasType();
/**
* required .TsColumnType type = 2;
*/
com.basho.riak.protobuf.RiakTsPB.TsColumnType getType();
}
/**
* Protobuf type {@code TsColumnDescription}
*/
public static final class TsColumnDescription extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsColumnDescription)
TsColumnDescriptionOrBuilder {
// Use TsColumnDescription.newBuilder() to construct.
private TsColumnDescription(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsColumnDescription(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsColumnDescription defaultInstance;
public static TsColumnDescription getDefaultInstance() {
return defaultInstance;
}
public TsColumnDescription getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsColumnDescription(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
com.basho.riak.protobuf.RiakTsPB.TsColumnType value = com.basho.riak.protobuf.RiakTsPB.TsColumnType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
type_ = value;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsColumnDescription_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsColumnDescription_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.class, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsColumnDescription parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsColumnDescription(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString name_;
/**
* required bytes name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes name = 1;
*/
public com.google.protobuf.ByteString getName() {
return name_;
}
public static final int TYPE_FIELD_NUMBER = 2;
private com.basho.riak.protobuf.RiakTsPB.TsColumnType type_;
/**
* required .TsColumnType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .TsColumnType type = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnType getType() {
return type_;
}
private void initFields() {
name_ = com.google.protobuf.ByteString.EMPTY;
type_ = com.basho.riak.protobuf.RiakTsPB.TsColumnType.VARCHAR;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, name_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, type_.getNumber());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, name_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_.getNumber());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsColumnDescription prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsColumnDescription}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsColumnDescription)
com.basho.riak.protobuf.RiakTsPB.TsColumnDescriptionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsColumnDescription_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsColumnDescription_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.class, com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
type_ = com.basho.riak.protobuf.RiakTsPB.TsColumnType.VARCHAR;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsColumnDescription_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription build() {
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsColumnDescription buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription result = new com.basho.riak.protobuf.RiakTsPB.TsColumnDescription(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsColumnDescription) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsColumnDescription)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsColumnDescription other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsColumnDescription.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasType()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsColumnDescription parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsColumnDescription) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes name = 1;
*/
public com.google.protobuf.ByteString getName() {
return name_;
}
/**
* required bytes name = 1;
*/
public Builder setName(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* required bytes name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakTsPB.TsColumnType type_ = com.basho.riak.protobuf.RiakTsPB.TsColumnType.VARCHAR;
/**
* required .TsColumnType type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required .TsColumnType type = 2;
*/
public com.basho.riak.protobuf.RiakTsPB.TsColumnType getType() {
return type_;
}
/**
* required .TsColumnType type = 2;
*/
public Builder setType(com.basho.riak.protobuf.RiakTsPB.TsColumnType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* required .TsColumnType type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = com.basho.riak.protobuf.RiakTsPB.TsColumnType.VARCHAR;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsColumnDescription)
}
static {
defaultInstance = new TsColumnDescription(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsColumnDescription)
}
public interface TsRowOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsRow)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .TsCell cells = 1;
*/
java.util.List
getCellsList();
/**
* repeated .TsCell cells = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsCell getCells(int index);
/**
* repeated .TsCell cells = 1;
*/
int getCellsCount();
/**
* repeated .TsCell cells = 1;
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getCellsOrBuilderList();
/**
* repeated .TsCell cells = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getCellsOrBuilder(
int index);
}
/**
* Protobuf type {@code TsRow}
*/
public static final class TsRow extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsRow)
TsRowOrBuilder {
// Use TsRow.newBuilder() to construct.
private TsRow(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsRow(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsRow defaultInstance;
public static TsRow getDefaultInstance() {
return defaultInstance;
}
public TsRow getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsRow(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
cells_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
cells_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsCell.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
cells_ = java.util.Collections.unmodifiableList(cells_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRow_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsRow.class, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsRow parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsRow(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int CELLS_FIELD_NUMBER = 1;
private java.util.List cells_;
/**
* repeated .TsCell cells = 1;
*/
public java.util.List getCellsList() {
return cells_;
}
/**
* repeated .TsCell cells = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getCellsOrBuilderList() {
return cells_;
}
/**
* repeated .TsCell cells = 1;
*/
public int getCellsCount() {
return cells_.size();
}
/**
* repeated .TsCell cells = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell getCells(int index) {
return cells_.get(index);
}
/**
* repeated .TsCell cells = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getCellsOrBuilder(
int index) {
return cells_.get(index);
}
private void initFields() {
cells_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < cells_.size(); i++) {
output.writeMessage(1, cells_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < cells_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, cells_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRow parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsRow prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsRow}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsRow)
com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRow_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRow_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsRow.class, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsRow.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getCellsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (cellsBuilder_ == null) {
cells_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
cellsBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRow_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsRow getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsRow build() {
com.basho.riak.protobuf.RiakTsPB.TsRow result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsRow buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsRow result = new com.basho.riak.protobuf.RiakTsPB.TsRow(this);
int from_bitField0_ = bitField0_;
if (cellsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
cells_ = java.util.Collections.unmodifiableList(cells_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.cells_ = cells_;
} else {
result.cells_ = cellsBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsRow) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsRow)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsRow other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance()) return this;
if (cellsBuilder_ == null) {
if (!other.cells_.isEmpty()) {
if (cells_.isEmpty()) {
cells_ = other.cells_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCellsIsMutable();
cells_.addAll(other.cells_);
}
onChanged();
}
} else {
if (!other.cells_.isEmpty()) {
if (cellsBuilder_.isEmpty()) {
cellsBuilder_.dispose();
cellsBuilder_ = null;
cells_ = other.cells_;
bitField0_ = (bitField0_ & ~0x00000001);
cellsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getCellsFieldBuilder() : null;
} else {
cellsBuilder_.addAllMessages(other.cells_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsRow parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsRow) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List cells_ =
java.util.Collections.emptyList();
private void ensureCellsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
cells_ = new java.util.ArrayList(cells_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder> cellsBuilder_;
/**
* repeated .TsCell cells = 1;
*/
public java.util.List getCellsList() {
if (cellsBuilder_ == null) {
return java.util.Collections.unmodifiableList(cells_);
} else {
return cellsBuilder_.getMessageList();
}
}
/**
* repeated .TsCell cells = 1;
*/
public int getCellsCount() {
if (cellsBuilder_ == null) {
return cells_.size();
} else {
return cellsBuilder_.getCount();
}
}
/**
* repeated .TsCell cells = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell getCells(int index) {
if (cellsBuilder_ == null) {
return cells_.get(index);
} else {
return cellsBuilder_.getMessage(index);
}
}
/**
* repeated .TsCell cells = 1;
*/
public Builder setCells(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (cellsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCellsIsMutable();
cells_.set(index, value);
onChanged();
} else {
cellsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public Builder setCells(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
cells_.set(index, builderForValue.build());
onChanged();
} else {
cellsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public Builder addCells(com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (cellsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCellsIsMutable();
cells_.add(value);
onChanged();
} else {
cellsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public Builder addCells(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell value) {
if (cellsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCellsIsMutable();
cells_.add(index, value);
onChanged();
} else {
cellsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public Builder addCells(
com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
cells_.add(builderForValue.build());
onChanged();
} else {
cellsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public Builder addCells(
int index, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder builderForValue) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
cells_.add(index, builderForValue.build());
onChanged();
} else {
cellsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public Builder addAllCells(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsCell> values) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cells_);
onChanged();
} else {
cellsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public Builder clearCells() {
if (cellsBuilder_ == null) {
cells_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
cellsBuilder_.clear();
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public Builder removeCells(int index) {
if (cellsBuilder_ == null) {
ensureCellsIsMutable();
cells_.remove(index);
onChanged();
} else {
cellsBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsCell cells = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder getCellsBuilder(
int index) {
return getCellsFieldBuilder().getBuilder(index);
}
/**
* repeated .TsCell cells = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder getCellsOrBuilder(
int index) {
if (cellsBuilder_ == null) {
return cells_.get(index); } else {
return cellsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsCell cells = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getCellsOrBuilderList() {
if (cellsBuilder_ != null) {
return cellsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(cells_);
}
}
/**
* repeated .TsCell cells = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder addCellsBuilder() {
return getCellsFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsCell.getDefaultInstance());
}
/**
* repeated .TsCell cells = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCell.Builder addCellsBuilder(
int index) {
return getCellsFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsCell.getDefaultInstance());
}
/**
* repeated .TsCell cells = 1;
*/
public java.util.List
getCellsBuilderList() {
return getCellsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>
getCellsFieldBuilder() {
if (cellsBuilder_ == null) {
cellsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCell, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder, com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder>(
cells_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
cells_ = null;
}
return cellsBuilder_;
}
// @@protoc_insertion_point(builder_scope:TsRow)
}
static {
defaultInstance = new TsRow(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsRow)
}
public interface TsCellOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsCell)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes varchar_value = 1;
*/
boolean hasVarcharValue();
/**
* optional bytes varchar_value = 1;
*/
com.google.protobuf.ByteString getVarcharValue();
/**
* optional sint64 sint64_value = 2;
*/
boolean hasSint64Value();
/**
* optional sint64 sint64_value = 2;
*/
long getSint64Value();
/**
* optional sint64 timestamp_value = 3;
*/
boolean hasTimestampValue();
/**
* optional sint64 timestamp_value = 3;
*/
long getTimestampValue();
/**
* optional bool boolean_value = 4;
*/
boolean hasBooleanValue();
/**
* optional bool boolean_value = 4;
*/
boolean getBooleanValue();
/**
* optional double double_value = 5;
*/
boolean hasDoubleValue();
/**
* optional double double_value = 5;
*/
double getDoubleValue();
}
/**
* Protobuf type {@code TsCell}
*/
public static final class TsCell extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsCell)
TsCellOrBuilder {
// Use TsCell.newBuilder() to construct.
private TsCell(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsCell(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsCell defaultInstance;
public static TsCell getDefaultInstance() {
return defaultInstance;
}
public TsCell getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsCell(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
varcharValue_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
sint64Value_ = input.readSInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
timestampValue_ = input.readSInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
booleanValue_ = input.readBool();
break;
}
case 41: {
bitField0_ |= 0x00000010;
doubleValue_ = input.readDouble();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCell_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCell_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsCell.class, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsCell parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsCell(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int VARCHAR_VALUE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString varcharValue_;
/**
* optional bytes varchar_value = 1;
*/
public boolean hasVarcharValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes varchar_value = 1;
*/
public com.google.protobuf.ByteString getVarcharValue() {
return varcharValue_;
}
public static final int SINT64_VALUE_FIELD_NUMBER = 2;
private long sint64Value_;
/**
* optional sint64 sint64_value = 2;
*/
public boolean hasSint64Value() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional sint64 sint64_value = 2;
*/
public long getSint64Value() {
return sint64Value_;
}
public static final int TIMESTAMP_VALUE_FIELD_NUMBER = 3;
private long timestampValue_;
/**
* optional sint64 timestamp_value = 3;
*/
public boolean hasTimestampValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional sint64 timestamp_value = 3;
*/
public long getTimestampValue() {
return timestampValue_;
}
public static final int BOOLEAN_VALUE_FIELD_NUMBER = 4;
private boolean booleanValue_;
/**
* optional bool boolean_value = 4;
*/
public boolean hasBooleanValue() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool boolean_value = 4;
*/
public boolean getBooleanValue() {
return booleanValue_;
}
public static final int DOUBLE_VALUE_FIELD_NUMBER = 5;
private double doubleValue_;
/**
* optional double double_value = 5;
*/
public boolean hasDoubleValue() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional double double_value = 5;
*/
public double getDoubleValue() {
return doubleValue_;
}
private void initFields() {
varcharValue_ = com.google.protobuf.ByteString.EMPTY;
sint64Value_ = 0L;
timestampValue_ = 0L;
booleanValue_ = false;
doubleValue_ = 0D;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, varcharValue_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeSInt64(2, sint64Value_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeSInt64(3, timestampValue_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, booleanValue_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeDouble(5, doubleValue_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, varcharValue_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(2, sint64Value_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(3, timestampValue_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, booleanValue_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, doubleValue_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCell parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsCell prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsCell}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsCell)
com.basho.riak.protobuf.RiakTsPB.TsCellOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCell_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCell_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsCell.class, com.basho.riak.protobuf.RiakTsPB.TsCell.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsCell.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
varcharValue_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
sint64Value_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
timestampValue_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
booleanValue_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
doubleValue_ = 0D;
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCell_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsCell getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsCell.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsCell build() {
com.basho.riak.protobuf.RiakTsPB.TsCell result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsCell buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsCell result = new com.basho.riak.protobuf.RiakTsPB.TsCell(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.varcharValue_ = varcharValue_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.sint64Value_ = sint64Value_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.timestampValue_ = timestampValue_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.booleanValue_ = booleanValue_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.doubleValue_ = doubleValue_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsCell) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsCell)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsCell other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsCell.getDefaultInstance()) return this;
if (other.hasVarcharValue()) {
setVarcharValue(other.getVarcharValue());
}
if (other.hasSint64Value()) {
setSint64Value(other.getSint64Value());
}
if (other.hasTimestampValue()) {
setTimestampValue(other.getTimestampValue());
}
if (other.hasBooleanValue()) {
setBooleanValue(other.getBooleanValue());
}
if (other.hasDoubleValue()) {
setDoubleValue(other.getDoubleValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsCell parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsCell) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString varcharValue_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes varchar_value = 1;
*/
public boolean hasVarcharValue() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes varchar_value = 1;
*/
public com.google.protobuf.ByteString getVarcharValue() {
return varcharValue_;
}
/**
* optional bytes varchar_value = 1;
*/
public Builder setVarcharValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
varcharValue_ = value;
onChanged();
return this;
}
/**
* optional bytes varchar_value = 1;
*/
public Builder clearVarcharValue() {
bitField0_ = (bitField0_ & ~0x00000001);
varcharValue_ = getDefaultInstance().getVarcharValue();
onChanged();
return this;
}
private long sint64Value_ ;
/**
* optional sint64 sint64_value = 2;
*/
public boolean hasSint64Value() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional sint64 sint64_value = 2;
*/
public long getSint64Value() {
return sint64Value_;
}
/**
* optional sint64 sint64_value = 2;
*/
public Builder setSint64Value(long value) {
bitField0_ |= 0x00000002;
sint64Value_ = value;
onChanged();
return this;
}
/**
* optional sint64 sint64_value = 2;
*/
public Builder clearSint64Value() {
bitField0_ = (bitField0_ & ~0x00000002);
sint64Value_ = 0L;
onChanged();
return this;
}
private long timestampValue_ ;
/**
* optional sint64 timestamp_value = 3;
*/
public boolean hasTimestampValue() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional sint64 timestamp_value = 3;
*/
public long getTimestampValue() {
return timestampValue_;
}
/**
* optional sint64 timestamp_value = 3;
*/
public Builder setTimestampValue(long value) {
bitField0_ |= 0x00000004;
timestampValue_ = value;
onChanged();
return this;
}
/**
* optional sint64 timestamp_value = 3;
*/
public Builder clearTimestampValue() {
bitField0_ = (bitField0_ & ~0x00000004);
timestampValue_ = 0L;
onChanged();
return this;
}
private boolean booleanValue_ ;
/**
* optional bool boolean_value = 4;
*/
public boolean hasBooleanValue() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool boolean_value = 4;
*/
public boolean getBooleanValue() {
return booleanValue_;
}
/**
* optional bool boolean_value = 4;
*/
public Builder setBooleanValue(boolean value) {
bitField0_ |= 0x00000008;
booleanValue_ = value;
onChanged();
return this;
}
/**
* optional bool boolean_value = 4;
*/
public Builder clearBooleanValue() {
bitField0_ = (bitField0_ & ~0x00000008);
booleanValue_ = false;
onChanged();
return this;
}
private double doubleValue_ ;
/**
* optional double double_value = 5;
*/
public boolean hasDoubleValue() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional double double_value = 5;
*/
public double getDoubleValue() {
return doubleValue_;
}
/**
* optional double double_value = 5;
*/
public Builder setDoubleValue(double value) {
bitField0_ |= 0x00000010;
doubleValue_ = value;
onChanged();
return this;
}
/**
* optional double double_value = 5;
*/
public Builder clearDoubleValue() {
bitField0_ = (bitField0_ & ~0x00000010);
doubleValue_ = 0D;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsCell)
}
static {
defaultInstance = new TsCell(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsCell)
}
public interface TsListKeysReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsListKeysReq)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes table = 1;
*/
boolean hasTable();
/**
* required bytes table = 1;
*/
com.google.protobuf.ByteString getTable();
/**
* optional uint32 timeout = 2;
*/
boolean hasTimeout();
/**
* optional uint32 timeout = 2;
*/
int getTimeout();
}
/**
* Protobuf type {@code TsListKeysReq}
*/
public static final class TsListKeysReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsListKeysReq)
TsListKeysReqOrBuilder {
// Use TsListKeysReq.newBuilder() to construct.
private TsListKeysReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsListKeysReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsListKeysReq defaultInstance;
public static TsListKeysReq getDefaultInstance() {
return defaultInstance;
}
public TsListKeysReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsListKeysReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
table_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
timeout_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsListKeysReq.class, com.basho.riak.protobuf.RiakTsPB.TsListKeysReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsListKeysReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsListKeysReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int TABLE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString table_;
/**
* required bytes table = 1;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes table = 1;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
public static final int TIMEOUT_FIELD_NUMBER = 2;
private int timeout_;
/**
* optional uint32 timeout = 2;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint32 timeout = 2;
*/
public int getTimeout() {
return timeout_;
}
private void initFields() {
table_ = com.google.protobuf.ByteString.EMPTY;
timeout_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTable()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, table_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(2, timeout_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, table_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, timeout_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsListKeysReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsListKeysReq}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsListKeysReq)
com.basho.riak.protobuf.RiakTsPB.TsListKeysReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsListKeysReq.class, com.basho.riak.protobuf.RiakTsPB.TsListKeysReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsListKeysReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
table_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
timeout_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysReq_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsListKeysReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsListKeysReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsListKeysReq build() {
com.basho.riak.protobuf.RiakTsPB.TsListKeysReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsListKeysReq buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsListKeysReq result = new com.basho.riak.protobuf.RiakTsPB.TsListKeysReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.table_ = table_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.timeout_ = timeout_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsListKeysReq) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsListKeysReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsListKeysReq other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsListKeysReq.getDefaultInstance()) return this;
if (other.hasTable()) {
setTable(other.getTable());
}
if (other.hasTimeout()) {
setTimeout(other.getTimeout());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTable()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsListKeysReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsListKeysReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString table_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes table = 1;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes table = 1;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
/**
* required bytes table = 1;
*/
public Builder setTable(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
table_ = value;
onChanged();
return this;
}
/**
* required bytes table = 1;
*/
public Builder clearTable() {
bitField0_ = (bitField0_ & ~0x00000001);
table_ = getDefaultInstance().getTable();
onChanged();
return this;
}
private int timeout_ ;
/**
* optional uint32 timeout = 2;
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint32 timeout = 2;
*/
public int getTimeout() {
return timeout_;
}
/**
* optional uint32 timeout = 2;
*/
public Builder setTimeout(int value) {
bitField0_ |= 0x00000002;
timeout_ = value;
onChanged();
return this;
}
/**
* optional uint32 timeout = 2;
*/
public Builder clearTimeout() {
bitField0_ = (bitField0_ & ~0x00000002);
timeout_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsListKeysReq)
}
static {
defaultInstance = new TsListKeysReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsListKeysReq)
}
public interface TsListKeysRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsListKeysResp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .TsRow keys = 1;
*/
java.util.List
getKeysList();
/**
* repeated .TsRow keys = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsRow getKeys(int index);
/**
* repeated .TsRow keys = 1;
*/
int getKeysCount();
/**
* repeated .TsRow keys = 1;
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getKeysOrBuilderList();
/**
* repeated .TsRow keys = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getKeysOrBuilder(
int index);
/**
* optional bool done = 2;
*/
boolean hasDone();
/**
* optional bool done = 2;
*/
boolean getDone();
}
/**
* Protobuf type {@code TsListKeysResp}
*/
public static final class TsListKeysResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsListKeysResp)
TsListKeysRespOrBuilder {
// Use TsListKeysResp.newBuilder() to construct.
private TsListKeysResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsListKeysResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsListKeysResp defaultInstance;
public static TsListKeysResp getDefaultInstance() {
return defaultInstance;
}
public TsListKeysResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsListKeysResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
keys_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
keys_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsRow.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
done_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
keys_ = java.util.Collections.unmodifiableList(keys_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsListKeysResp.class, com.basho.riak.protobuf.RiakTsPB.TsListKeysResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsListKeysResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsListKeysResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int KEYS_FIELD_NUMBER = 1;
private java.util.List keys_;
/**
* repeated .TsRow keys = 1;
*/
public java.util.List getKeysList() {
return keys_;
}
/**
* repeated .TsRow keys = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getKeysOrBuilderList() {
return keys_;
}
/**
* repeated .TsRow keys = 1;
*/
public int getKeysCount() {
return keys_.size();
}
/**
* repeated .TsRow keys = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow getKeys(int index) {
return keys_.get(index);
}
/**
* repeated .TsRow keys = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getKeysOrBuilder(
int index) {
return keys_.get(index);
}
public static final int DONE_FIELD_NUMBER = 2;
private boolean done_;
/**
* optional bool done = 2;
*/
public boolean hasDone() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bool done = 2;
*/
public boolean getDone() {
return done_;
}
private void initFields() {
keys_ = java.util.Collections.emptyList();
done_ = false;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < keys_.size(); i++) {
output.writeMessage(1, keys_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBool(2, done_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < keys_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, keys_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, done_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsListKeysResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsListKeysResp}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsListKeysResp)
com.basho.riak.protobuf.RiakTsPB.TsListKeysRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsListKeysResp.class, com.basho.riak.protobuf.RiakTsPB.TsListKeysResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsListKeysResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getKeysFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (keysBuilder_ == null) {
keys_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
keysBuilder_.clear();
}
done_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsListKeysResp_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsListKeysResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsListKeysResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsListKeysResp build() {
com.basho.riak.protobuf.RiakTsPB.TsListKeysResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsListKeysResp buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsListKeysResp result = new com.basho.riak.protobuf.RiakTsPB.TsListKeysResp(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (keysBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
keys_ = java.util.Collections.unmodifiableList(keys_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.keys_ = keys_;
} else {
result.keys_ = keysBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.done_ = done_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsListKeysResp) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsListKeysResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsListKeysResp other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsListKeysResp.getDefaultInstance()) return this;
if (keysBuilder_ == null) {
if (!other.keys_.isEmpty()) {
if (keys_.isEmpty()) {
keys_ = other.keys_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureKeysIsMutable();
keys_.addAll(other.keys_);
}
onChanged();
}
} else {
if (!other.keys_.isEmpty()) {
if (keysBuilder_.isEmpty()) {
keysBuilder_.dispose();
keysBuilder_ = null;
keys_ = other.keys_;
bitField0_ = (bitField0_ & ~0x00000001);
keysBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getKeysFieldBuilder() : null;
} else {
keysBuilder_.addAllMessages(other.keys_);
}
}
}
if (other.hasDone()) {
setDone(other.getDone());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsListKeysResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsListKeysResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List keys_ =
java.util.Collections.emptyList();
private void ensureKeysIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
keys_ = new java.util.ArrayList(keys_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder> keysBuilder_;
/**
* repeated .TsRow keys = 1;
*/
public java.util.List getKeysList() {
if (keysBuilder_ == null) {
return java.util.Collections.unmodifiableList(keys_);
} else {
return keysBuilder_.getMessageList();
}
}
/**
* repeated .TsRow keys = 1;
*/
public int getKeysCount() {
if (keysBuilder_ == null) {
return keys_.size();
} else {
return keysBuilder_.getCount();
}
}
/**
* repeated .TsRow keys = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow getKeys(int index) {
if (keysBuilder_ == null) {
return keys_.get(index);
} else {
return keysBuilder_.getMessage(index);
}
}
/**
* repeated .TsRow keys = 1;
*/
public Builder setKeys(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (keysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeysIsMutable();
keys_.set(index, value);
onChanged();
} else {
keysBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public Builder setKeys(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
keys_.set(index, builderForValue.build());
onChanged();
} else {
keysBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public Builder addKeys(com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (keysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeysIsMutable();
keys_.add(value);
onChanged();
} else {
keysBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public Builder addKeys(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow value) {
if (keysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureKeysIsMutable();
keys_.add(index, value);
onChanged();
} else {
keysBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public Builder addKeys(
com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
keys_.add(builderForValue.build());
onChanged();
} else {
keysBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public Builder addKeys(
int index, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder builderForValue) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
keys_.add(index, builderForValue.build());
onChanged();
} else {
keysBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public Builder addAllKeys(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsRow> values) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, keys_);
onChanged();
} else {
keysBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public Builder clearKeys() {
if (keysBuilder_ == null) {
keys_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
keysBuilder_.clear();
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public Builder removeKeys(int index) {
if (keysBuilder_ == null) {
ensureKeysIsMutable();
keys_.remove(index);
onChanged();
} else {
keysBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsRow keys = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder getKeysBuilder(
int index) {
return getKeysFieldBuilder().getBuilder(index);
}
/**
* repeated .TsRow keys = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder getKeysOrBuilder(
int index) {
if (keysBuilder_ == null) {
return keys_.get(index); } else {
return keysBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsRow keys = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getKeysOrBuilderList() {
if (keysBuilder_ != null) {
return keysBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(keys_);
}
}
/**
* repeated .TsRow keys = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder addKeysBuilder() {
return getKeysFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance());
}
/**
* repeated .TsRow keys = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsRow.Builder addKeysBuilder(
int index) {
return getKeysFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsRow.getDefaultInstance());
}
/**
* repeated .TsRow keys = 1;
*/
public java.util.List
getKeysBuilderList() {
return getKeysFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>
getKeysFieldBuilder() {
if (keysBuilder_ == null) {
keysBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRow, com.basho.riak.protobuf.RiakTsPB.TsRow.Builder, com.basho.riak.protobuf.RiakTsPB.TsRowOrBuilder>(
keys_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
keys_ = null;
}
return keysBuilder_;
}
private boolean done_ ;
/**
* optional bool done = 2;
*/
public boolean hasDone() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool done = 2;
*/
public boolean getDone() {
return done_;
}
/**
* optional bool done = 2;
*/
public Builder setDone(boolean value) {
bitField0_ |= 0x00000002;
done_ = value;
onChanged();
return this;
}
/**
* optional bool done = 2;
*/
public Builder clearDone() {
bitField0_ = (bitField0_ & ~0x00000002);
done_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsListKeysResp)
}
static {
defaultInstance = new TsListKeysResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsListKeysResp)
}
public interface TsCoverageReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsCoverageReq)
com.google.protobuf.MessageOrBuilder {
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
boolean hasQuery();
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
com.basho.riak.protobuf.RiakTsPB.TsInterpolation getQuery();
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder getQueryOrBuilder();
/**
* required bytes table = 2;
*/
boolean hasTable();
/**
* required bytes table = 2;
*/
com.google.protobuf.ByteString getTable();
/**
* optional bytes replace_cover = 3;
*
*
* For failure recovery
*
*/
boolean hasReplaceCover();
/**
* optional bytes replace_cover = 3;
*
*
* For failure recovery
*
*/
com.google.protobuf.ByteString getReplaceCover();
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
java.util.List getUnavailableCoverList();
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
int getUnavailableCoverCount();
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
com.google.protobuf.ByteString getUnavailableCover(int index);
}
/**
* Protobuf type {@code TsCoverageReq}
*
*
* Request a segmented coverage plan for this query
*
*/
public static final class TsCoverageReq extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsCoverageReq)
TsCoverageReqOrBuilder {
// Use TsCoverageReq.newBuilder() to construct.
private TsCoverageReq(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsCoverageReq(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsCoverageReq defaultInstance;
public static TsCoverageReq getDefaultInstance() {
return defaultInstance;
}
public TsCoverageReq getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsCoverageReq(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = query_.toBuilder();
}
query_ = input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsInterpolation.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(query_);
query_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
bitField0_ |= 0x00000002;
table_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
replaceCover_ = input.readBytes();
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
unavailableCover_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
unavailableCover_.add(input.readBytes());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
unavailableCover_ = java.util.Collections.unmodifiableList(unavailableCover_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsCoverageReq.class, com.basho.riak.protobuf.RiakTsPB.TsCoverageReq.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsCoverageReq parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsCoverageReq(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int QUERY_FIELD_NUMBER = 1;
private com.basho.riak.protobuf.RiakTsPB.TsInterpolation query_;
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public boolean hasQuery() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation getQuery() {
return query_;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder getQueryOrBuilder() {
return query_;
}
public static final int TABLE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString table_;
/**
* required bytes table = 2;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes table = 2;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
public static final int REPLACE_COVER_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString replaceCover_;
/**
* optional bytes replace_cover = 3;
*
*
* For failure recovery
*
*/
public boolean hasReplaceCover() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes replace_cover = 3;
*
*
* For failure recovery
*
*/
public com.google.protobuf.ByteString getReplaceCover() {
return replaceCover_;
}
public static final int UNAVAILABLE_COVER_FIELD_NUMBER = 4;
private java.util.List unavailableCover_;
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public java.util.List
getUnavailableCoverList() {
return unavailableCover_;
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public int getUnavailableCoverCount() {
return unavailableCover_.size();
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public com.google.protobuf.ByteString getUnavailableCover(int index) {
return unavailableCover_.get(index);
}
private void initFields() {
query_ = com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
table_ = com.google.protobuf.ByteString.EMPTY;
replaceCover_ = com.google.protobuf.ByteString.EMPTY;
unavailableCover_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTable()) {
memoizedIsInitialized = 0;
return false;
}
if (hasQuery()) {
if (!getQuery().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, query_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, table_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, replaceCover_);
}
for (int i = 0; i < unavailableCover_.size(); i++) {
output.writeBytes(4, unavailableCover_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, query_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, table_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, replaceCover_);
}
{
int dataSize = 0;
for (int i = 0; i < unavailableCover_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(unavailableCover_.get(i));
}
size += dataSize;
size += 1 * getUnavailableCoverList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsCoverageReq prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsCoverageReq}
*
*
* Request a segmented coverage plan for this query
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsCoverageReq)
com.basho.riak.protobuf.RiakTsPB.TsCoverageReqOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageReq_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageReq_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsCoverageReq.class, com.basho.riak.protobuf.RiakTsPB.TsCoverageReq.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsCoverageReq.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getQueryFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (queryBuilder_ == null) {
query_ = com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
} else {
queryBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
table_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
replaceCover_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
unavailableCover_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageReq_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageReq getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsCoverageReq.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageReq build() {
com.basho.riak.protobuf.RiakTsPB.TsCoverageReq result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageReq buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsCoverageReq result = new com.basho.riak.protobuf.RiakTsPB.TsCoverageReq(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (queryBuilder_ == null) {
result.query_ = query_;
} else {
result.query_ = queryBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.table_ = table_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.replaceCover_ = replaceCover_;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
unavailableCover_ = java.util.Collections.unmodifiableList(unavailableCover_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.unavailableCover_ = unavailableCover_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsCoverageReq) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsCoverageReq)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsCoverageReq other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsCoverageReq.getDefaultInstance()) return this;
if (other.hasQuery()) {
mergeQuery(other.getQuery());
}
if (other.hasTable()) {
setTable(other.getTable());
}
if (other.hasReplaceCover()) {
setReplaceCover(other.getReplaceCover());
}
if (!other.unavailableCover_.isEmpty()) {
if (unavailableCover_.isEmpty()) {
unavailableCover_ = other.unavailableCover_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureUnavailableCoverIsMutable();
unavailableCover_.addAll(other.unavailableCover_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasTable()) {
return false;
}
if (hasQuery()) {
if (!getQuery().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsCoverageReq parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsCoverageReq) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.basho.riak.protobuf.RiakTsPB.TsInterpolation query_ = com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsInterpolation, com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder, com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder> queryBuilder_;
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public boolean hasQuery() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation getQuery() {
if (queryBuilder_ == null) {
return query_;
} else {
return queryBuilder_.getMessage();
}
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public Builder setQuery(com.basho.riak.protobuf.RiakTsPB.TsInterpolation value) {
if (queryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
query_ = value;
onChanged();
} else {
queryBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public Builder setQuery(
com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder builderForValue) {
if (queryBuilder_ == null) {
query_ = builderForValue.build();
onChanged();
} else {
queryBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public Builder mergeQuery(com.basho.riak.protobuf.RiakTsPB.TsInterpolation value) {
if (queryBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
query_ != com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance()) {
query_ =
com.basho.riak.protobuf.RiakTsPB.TsInterpolation.newBuilder(query_).mergeFrom(value).buildPartial();
} else {
query_ = value;
}
onChanged();
} else {
queryBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public Builder clearQuery() {
if (queryBuilder_ == null) {
query_ = com.basho.riak.protobuf.RiakTsPB.TsInterpolation.getDefaultInstance();
onChanged();
} else {
queryBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder getQueryBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getQueryFieldBuilder().getBuilder();
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder getQueryOrBuilder() {
if (queryBuilder_ != null) {
return queryBuilder_.getMessageOrBuilder();
} else {
return query_;
}
}
/**
* optional .TsInterpolation query = 1;
*
*
* left optional to support parameterized queries in the future
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsInterpolation, com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder, com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder>
getQueryFieldBuilder() {
if (queryBuilder_ == null) {
queryBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsInterpolation, com.basho.riak.protobuf.RiakTsPB.TsInterpolation.Builder, com.basho.riak.protobuf.RiakTsPB.TsInterpolationOrBuilder>(
getQuery(),
getParentForChildren(),
isClean());
query_ = null;
}
return queryBuilder_;
}
private com.google.protobuf.ByteString table_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes table = 2;
*/
public boolean hasTable() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required bytes table = 2;
*/
public com.google.protobuf.ByteString getTable() {
return table_;
}
/**
* required bytes table = 2;
*/
public Builder setTable(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
table_ = value;
onChanged();
return this;
}
/**
* required bytes table = 2;
*/
public Builder clearTable() {
bitField0_ = (bitField0_ & ~0x00000002);
table_ = getDefaultInstance().getTable();
onChanged();
return this;
}
private com.google.protobuf.ByteString replaceCover_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes replace_cover = 3;
*
*
* For failure recovery
*
*/
public boolean hasReplaceCover() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bytes replace_cover = 3;
*
*
* For failure recovery
*
*/
public com.google.protobuf.ByteString getReplaceCover() {
return replaceCover_;
}
/**
* optional bytes replace_cover = 3;
*
*
* For failure recovery
*
*/
public Builder setReplaceCover(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
replaceCover_ = value;
onChanged();
return this;
}
/**
* optional bytes replace_cover = 3;
*
*
* For failure recovery
*
*/
public Builder clearReplaceCover() {
bitField0_ = (bitField0_ & ~0x00000004);
replaceCover_ = getDefaultInstance().getReplaceCover();
onChanged();
return this;
}
private java.util.List unavailableCover_ = java.util.Collections.emptyList();
private void ensureUnavailableCoverIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
unavailableCover_ = new java.util.ArrayList(unavailableCover_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public java.util.List
getUnavailableCoverList() {
return java.util.Collections.unmodifiableList(unavailableCover_);
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public int getUnavailableCoverCount() {
return unavailableCover_.size();
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public com.google.protobuf.ByteString getUnavailableCover(int index) {
return unavailableCover_.get(index);
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public Builder setUnavailableCover(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureUnavailableCoverIsMutable();
unavailableCover_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public Builder addUnavailableCover(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureUnavailableCoverIsMutable();
unavailableCover_.add(value);
onChanged();
return this;
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public Builder addAllUnavailableCover(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureUnavailableCoverIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, unavailableCover_);
onChanged();
return this;
}
/**
* repeated bytes unavailable_cover = 4;
*
*
* Other coverage contexts that have failed to assist Riak in deciding what nodes to avoid
*
*/
public Builder clearUnavailableCover() {
unavailableCover_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsCoverageReq)
}
static {
defaultInstance = new TsCoverageReq(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsCoverageReq)
}
public interface TsCoverageRespOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsCoverageResp)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .TsCoverageEntry entries = 1;
*/
java.util.List
getEntriesList();
/**
* repeated .TsCoverageEntry entries = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry getEntries(int index);
/**
* repeated .TsCoverageEntry entries = 1;
*/
int getEntriesCount();
/**
* repeated .TsCoverageEntry entries = 1;
*/
java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder>
getEntriesOrBuilderList();
/**
* repeated .TsCoverageEntry entries = 1;
*/
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder getEntriesOrBuilder(
int index);
}
/**
* Protobuf type {@code TsCoverageResp}
*
*
* Segmented TS coverage plan response
*
*/
public static final class TsCoverageResp extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsCoverageResp)
TsCoverageRespOrBuilder {
// Use TsCoverageResp.newBuilder() to construct.
private TsCoverageResp(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsCoverageResp(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsCoverageResp defaultInstance;
public static TsCoverageResp getDefaultInstance() {
return defaultInstance;
}
public TsCoverageResp getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsCoverageResp(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
entries_.add(input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsCoverageResp.class, com.basho.riak.protobuf.RiakTsPB.TsCoverageResp.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsCoverageResp parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsCoverageResp(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public static final int ENTRIES_FIELD_NUMBER = 1;
private java.util.List entries_;
/**
* repeated .TsCoverageEntry entries = 1;
*/
public java.util.List getEntriesList() {
return entries_;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public int getEntriesCount() {
return entries_.size();
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry getEntries(int index) {
return entries_.get(index);
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
private void initFields() {
entries_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getEntriesCount(); i++) {
if (!getEntries(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(1, entries_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, entries_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsCoverageResp prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsCoverageResp}
*
*
* Segmented TS coverage plan response
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsCoverageResp)
com.basho.riak.protobuf.RiakTsPB.TsCoverageRespOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageResp_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageResp_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsCoverageResp.class, com.basho.riak.protobuf.RiakTsPB.TsCoverageResp.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsCoverageResp.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
entriesBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageResp_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageResp getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsCoverageResp.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageResp build() {
com.basho.riak.protobuf.RiakTsPB.TsCoverageResp result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageResp buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsCoverageResp result = new com.basho.riak.protobuf.RiakTsPB.TsCoverageResp(this);
int from_bitField0_ = bitField0_;
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsCoverageResp) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsCoverageResp)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsCoverageResp other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsCoverageResp.getDefaultInstance()) return this;
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000001);
entriesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getEntriesCount(); i++) {
if (!getEntries(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsCoverageResp parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsCoverageResp) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder> entriesBuilder_;
/**
* repeated .TsCoverageEntry entries = 1;
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder setEntries(
int index, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder setEntries(
int index, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder addEntries(com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder addEntries(
int index, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder addEntries(
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder addEntries(
int index, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder addAllEntries(
java.lang.Iterable extends com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public java.util.List extends com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.getDefaultInstance());
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.getDefaultInstance());
}
/**
* repeated .TsCoverageEntry entries = 1;
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder>(
entries_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
// @@protoc_insertion_point(builder_scope:TsCoverageResp)
}
static {
defaultInstance = new TsCoverageResp(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsCoverageResp)
}
public interface TsCoverageEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsCoverageEntry)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes ip = 1;
*/
boolean hasIp();
/**
* required bytes ip = 1;
*/
com.google.protobuf.ByteString getIp();
/**
* required uint32 port = 2;
*/
boolean hasPort();
/**
* required uint32 port = 2;
*/
int getPort();
/**
* required bytes cover_context = 3;
*
*
* Opaque context to pass into follow-up request
*
*/
boolean hasCoverContext();
/**
* required bytes cover_context = 3;
*
*
* Opaque context to pass into follow-up request
*
*/
com.google.protobuf.ByteString getCoverContext();
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
boolean hasRange();
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
com.basho.riak.protobuf.RiakTsPB.TsRange getRange();
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
com.basho.riak.protobuf.RiakTsPB.TsRangeOrBuilder getRangeOrBuilder();
}
/**
* Protobuf type {@code TsCoverageEntry}
*
*
* Segment of a TS coverage plan
*
*/
public static final class TsCoverageEntry extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsCoverageEntry)
TsCoverageEntryOrBuilder {
// Use TsCoverageEntry.newBuilder() to construct.
private TsCoverageEntry(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsCoverageEntry(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsCoverageEntry defaultInstance;
public static TsCoverageEntry getDefaultInstance() {
return defaultInstance;
}
public TsCoverageEntry getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsCoverageEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
ip_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
port_ = input.readUInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
coverContext_ = input.readBytes();
break;
}
case 34: {
com.basho.riak.protobuf.RiakTsPB.TsRange.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = range_.toBuilder();
}
range_ = input.readMessage(com.basho.riak.protobuf.RiakTsPB.TsRange.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(range_);
range_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageEntry_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.class, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsCoverageEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsCoverageEntry(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int IP_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString ip_;
/**
* required bytes ip = 1;
*/
public boolean hasIp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes ip = 1;
*/
public com.google.protobuf.ByteString getIp() {
return ip_;
}
public static final int PORT_FIELD_NUMBER = 2;
private int port_;
/**
* required uint32 port = 2;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint32 port = 2;
*/
public int getPort() {
return port_;
}
public static final int COVER_CONTEXT_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString coverContext_;
/**
* required bytes cover_context = 3;
*
*
* Opaque context to pass into follow-up request
*
*/
public boolean hasCoverContext() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bytes cover_context = 3;
*
*
* Opaque context to pass into follow-up request
*
*/
public com.google.protobuf.ByteString getCoverContext() {
return coverContext_;
}
public static final int RANGE_FIELD_NUMBER = 4;
private com.basho.riak.protobuf.RiakTsPB.TsRange range_;
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public boolean hasRange() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRange getRange() {
return range_;
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRangeOrBuilder getRangeOrBuilder() {
return range_;
}
private void initFields() {
ip_ = com.google.protobuf.ByteString.EMPTY;
port_ = 0;
coverContext_ = com.google.protobuf.ByteString.EMPTY;
range_ = com.basho.riak.protobuf.RiakTsPB.TsRange.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasIp()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCoverContext()) {
memoizedIsInitialized = 0;
return false;
}
if (hasRange()) {
if (!getRange().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, ip_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(2, port_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, coverContext_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, range_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, ip_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, port_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, coverContext_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, range_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsCoverageEntry}
*
*
* Segment of a TS coverage plan
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsCoverageEntry)
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageEntry_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.class, com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getRangeFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
ip_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
coverContext_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
if (rangeBuilder_ == null) {
range_ = com.basho.riak.protobuf.RiakTsPB.TsRange.getDefaultInstance();
} else {
rangeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsCoverageEntry_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry build() {
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry result = new com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.ip_ = ip_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.port_ = port_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.coverContext_ = coverContext_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (rangeBuilder_ == null) {
result.range_ = range_;
} else {
result.range_ = rangeBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry.getDefaultInstance()) return this;
if (other.hasIp()) {
setIp(other.getIp());
}
if (other.hasPort()) {
setPort(other.getPort());
}
if (other.hasCoverContext()) {
setCoverContext(other.getCoverContext());
}
if (other.hasRange()) {
mergeRange(other.getRange());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasIp()) {
return false;
}
if (!hasPort()) {
return false;
}
if (!hasCoverContext()) {
return false;
}
if (hasRange()) {
if (!getRange().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsCoverageEntry) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString ip_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes ip = 1;
*/
public boolean hasIp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes ip = 1;
*/
public com.google.protobuf.ByteString getIp() {
return ip_;
}
/**
* required bytes ip = 1;
*/
public Builder setIp(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
ip_ = value;
onChanged();
return this;
}
/**
* required bytes ip = 1;
*/
public Builder clearIp() {
bitField0_ = (bitField0_ & ~0x00000001);
ip_ = getDefaultInstance().getIp();
onChanged();
return this;
}
private int port_ ;
/**
* required uint32 port = 2;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint32 port = 2;
*/
public int getPort() {
return port_;
}
/**
* required uint32 port = 2;
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000002;
port_ = value;
onChanged();
return this;
}
/**
* required uint32 port = 2;
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString coverContext_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes cover_context = 3;
*
*
* Opaque context to pass into follow-up request
*
*/
public boolean hasCoverContext() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bytes cover_context = 3;
*
*
* Opaque context to pass into follow-up request
*
*/
public com.google.protobuf.ByteString getCoverContext() {
return coverContext_;
}
/**
* required bytes cover_context = 3;
*
*
* Opaque context to pass into follow-up request
*
*/
public Builder setCoverContext(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
coverContext_ = value;
onChanged();
return this;
}
/**
* required bytes cover_context = 3;
*
*
* Opaque context to pass into follow-up request
*
*/
public Builder clearCoverContext() {
bitField0_ = (bitField0_ & ~0x00000004);
coverContext_ = getDefaultInstance().getCoverContext();
onChanged();
return this;
}
private com.basho.riak.protobuf.RiakTsPB.TsRange range_ = com.basho.riak.protobuf.RiakTsPB.TsRange.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRange, com.basho.riak.protobuf.RiakTsPB.TsRange.Builder, com.basho.riak.protobuf.RiakTsPB.TsRangeOrBuilder> rangeBuilder_;
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public boolean hasRange() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRange getRange() {
if (rangeBuilder_ == null) {
return range_;
} else {
return rangeBuilder_.getMessage();
}
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public Builder setRange(com.basho.riak.protobuf.RiakTsPB.TsRange value) {
if (rangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
range_ = value;
onChanged();
} else {
rangeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public Builder setRange(
com.basho.riak.protobuf.RiakTsPB.TsRange.Builder builderForValue) {
if (rangeBuilder_ == null) {
range_ = builderForValue.build();
onChanged();
} else {
rangeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public Builder mergeRange(com.basho.riak.protobuf.RiakTsPB.TsRange value) {
if (rangeBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
range_ != com.basho.riak.protobuf.RiakTsPB.TsRange.getDefaultInstance()) {
range_ =
com.basho.riak.protobuf.RiakTsPB.TsRange.newBuilder(range_).mergeFrom(value).buildPartial();
} else {
range_ = value;
}
onChanged();
} else {
rangeBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public Builder clearRange() {
if (rangeBuilder_ == null) {
range_ = com.basho.riak.protobuf.RiakTsPB.TsRange.getDefaultInstance();
onChanged();
} else {
rangeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRange.Builder getRangeBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getRangeFieldBuilder().getBuilder();
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
public com.basho.riak.protobuf.RiakTsPB.TsRangeOrBuilder getRangeOrBuilder() {
if (rangeBuilder_ != null) {
return rangeBuilder_.getMessageOrBuilder();
} else {
return range_;
}
}
/**
* optional .TsRange range = 4;
*
*
* Might be other types of coverage queries/responses
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRange, com.basho.riak.protobuf.RiakTsPB.TsRange.Builder, com.basho.riak.protobuf.RiakTsPB.TsRangeOrBuilder>
getRangeFieldBuilder() {
if (rangeBuilder_ == null) {
rangeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.basho.riak.protobuf.RiakTsPB.TsRange, com.basho.riak.protobuf.RiakTsPB.TsRange.Builder, com.basho.riak.protobuf.RiakTsPB.TsRangeOrBuilder>(
getRange(),
getParentForChildren(),
isClean());
range_ = null;
}
return rangeBuilder_;
}
// @@protoc_insertion_point(builder_scope:TsCoverageEntry)
}
static {
defaultInstance = new TsCoverageEntry(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsCoverageEntry)
}
public interface TsRangeOrBuilder extends
// @@protoc_insertion_point(interface_extends:TsRange)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes field_name = 1;
*/
boolean hasFieldName();
/**
* required bytes field_name = 1;
*/
com.google.protobuf.ByteString getFieldName();
/**
* required sint64 lower_bound = 2;
*/
boolean hasLowerBound();
/**
* required sint64 lower_bound = 2;
*/
long getLowerBound();
/**
* required bool lower_bound_inclusive = 3;
*/
boolean hasLowerBoundInclusive();
/**
* required bool lower_bound_inclusive = 3;
*/
boolean getLowerBoundInclusive();
/**
* required sint64 upper_bound = 4;
*/
boolean hasUpperBound();
/**
* required sint64 upper_bound = 4;
*/
long getUpperBound();
/**
* required bool upper_bound_inclusive = 5;
*/
boolean hasUpperBoundInclusive();
/**
* required bool upper_bound_inclusive = 5;
*/
boolean getUpperBoundInclusive();
/**
* required bytes desc = 6;
*
*
* Some human readable description of the time range
*
*/
boolean hasDesc();
/**
* required bytes desc = 6;
*
*
* Some human readable description of the time range
*
*/
com.google.protobuf.ByteString getDesc();
}
/**
* Protobuf type {@code TsRange}
*
*
* Each prospective subquery has a range of valid time values
*
*/
public static final class TsRange extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:TsRange)
TsRangeOrBuilder {
// Use TsRange.newBuilder() to construct.
private TsRange(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private TsRange(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final TsRange defaultInstance;
public static TsRange getDefaultInstance() {
return defaultInstance;
}
public TsRange getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TsRange(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
fieldName_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
lowerBound_ = input.readSInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
lowerBoundInclusive_ = input.readBool();
break;
}
case 32: {
bitField0_ |= 0x00000008;
upperBound_ = input.readSInt64();
break;
}
case 40: {
bitField0_ |= 0x00000010;
upperBoundInclusive_ = input.readBool();
break;
}
case 50: {
bitField0_ |= 0x00000020;
desc_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRange_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsRange.class, com.basho.riak.protobuf.RiakTsPB.TsRange.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public TsRange parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TsRange(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
public static final int FIELD_NAME_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString fieldName_;
/**
* required bytes field_name = 1;
*/
public boolean hasFieldName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes field_name = 1;
*/
public com.google.protobuf.ByteString getFieldName() {
return fieldName_;
}
public static final int LOWER_BOUND_FIELD_NUMBER = 2;
private long lowerBound_;
/**
* required sint64 lower_bound = 2;
*/
public boolean hasLowerBound() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required sint64 lower_bound = 2;
*/
public long getLowerBound() {
return lowerBound_;
}
public static final int LOWER_BOUND_INCLUSIVE_FIELD_NUMBER = 3;
private boolean lowerBoundInclusive_;
/**
* required bool lower_bound_inclusive = 3;
*/
public boolean hasLowerBoundInclusive() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bool lower_bound_inclusive = 3;
*/
public boolean getLowerBoundInclusive() {
return lowerBoundInclusive_;
}
public static final int UPPER_BOUND_FIELD_NUMBER = 4;
private long upperBound_;
/**
* required sint64 upper_bound = 4;
*/
public boolean hasUpperBound() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required sint64 upper_bound = 4;
*/
public long getUpperBound() {
return upperBound_;
}
public static final int UPPER_BOUND_INCLUSIVE_FIELD_NUMBER = 5;
private boolean upperBoundInclusive_;
/**
* required bool upper_bound_inclusive = 5;
*/
public boolean hasUpperBoundInclusive() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required bool upper_bound_inclusive = 5;
*/
public boolean getUpperBoundInclusive() {
return upperBoundInclusive_;
}
public static final int DESC_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString desc_;
/**
* required bytes desc = 6;
*
*
* Some human readable description of the time range
*
*/
public boolean hasDesc() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required bytes desc = 6;
*
*
* Some human readable description of the time range
*
*/
public com.google.protobuf.ByteString getDesc() {
return desc_;
}
private void initFields() {
fieldName_ = com.google.protobuf.ByteString.EMPTY;
lowerBound_ = 0L;
lowerBoundInclusive_ = false;
upperBound_ = 0L;
upperBoundInclusive_ = false;
desc_ = com.google.protobuf.ByteString.EMPTY;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasFieldName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasLowerBound()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasLowerBoundInclusive()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasUpperBound()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasUpperBoundInclusive()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasDesc()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, fieldName_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeSInt64(2, lowerBound_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBool(3, lowerBoundInclusive_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeSInt64(4, upperBound_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, upperBoundInclusive_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, desc_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, fieldName_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(2, lowerBound_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, lowerBoundInclusive_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(4, upperBound_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, upperBoundInclusive_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, desc_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.basho.riak.protobuf.RiakTsPB.TsRange parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.basho.riak.protobuf.RiakTsPB.TsRange prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code TsRange}
*
*
* Each prospective subquery has a range of valid time values
*
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:TsRange)
com.basho.riak.protobuf.RiakTsPB.TsRangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRange_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.basho.riak.protobuf.RiakTsPB.TsRange.class, com.basho.riak.protobuf.RiakTsPB.TsRange.Builder.class);
}
// Construct using com.basho.riak.protobuf.RiakTsPB.TsRange.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
fieldName_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
lowerBound_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
lowerBoundInclusive_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
upperBound_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
upperBoundInclusive_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
desc_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.basho.riak.protobuf.RiakTsPB.internal_static_TsRange_descriptor;
}
public com.basho.riak.protobuf.RiakTsPB.TsRange getDefaultInstanceForType() {
return com.basho.riak.protobuf.RiakTsPB.TsRange.getDefaultInstance();
}
public com.basho.riak.protobuf.RiakTsPB.TsRange build() {
com.basho.riak.protobuf.RiakTsPB.TsRange result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.basho.riak.protobuf.RiakTsPB.TsRange buildPartial() {
com.basho.riak.protobuf.RiakTsPB.TsRange result = new com.basho.riak.protobuf.RiakTsPB.TsRange(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.fieldName_ = fieldName_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.lowerBound_ = lowerBound_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.lowerBoundInclusive_ = lowerBoundInclusive_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.upperBound_ = upperBound_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.upperBoundInclusive_ = upperBoundInclusive_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.desc_ = desc_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.basho.riak.protobuf.RiakTsPB.TsRange) {
return mergeFrom((com.basho.riak.protobuf.RiakTsPB.TsRange)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.basho.riak.protobuf.RiakTsPB.TsRange other) {
if (other == com.basho.riak.protobuf.RiakTsPB.TsRange.getDefaultInstance()) return this;
if (other.hasFieldName()) {
setFieldName(other.getFieldName());
}
if (other.hasLowerBound()) {
setLowerBound(other.getLowerBound());
}
if (other.hasLowerBoundInclusive()) {
setLowerBoundInclusive(other.getLowerBoundInclusive());
}
if (other.hasUpperBound()) {
setUpperBound(other.getUpperBound());
}
if (other.hasUpperBoundInclusive()) {
setUpperBoundInclusive(other.getUpperBoundInclusive());
}
if (other.hasDesc()) {
setDesc(other.getDesc());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
if (!hasFieldName()) {
return false;
}
if (!hasLowerBound()) {
return false;
}
if (!hasLowerBoundInclusive()) {
return false;
}
if (!hasUpperBound()) {
return false;
}
if (!hasUpperBoundInclusive()) {
return false;
}
if (!hasDesc()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.basho.riak.protobuf.RiakTsPB.TsRange parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.basho.riak.protobuf.RiakTsPB.TsRange) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString fieldName_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes field_name = 1;
*/
public boolean hasFieldName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes field_name = 1;
*/
public com.google.protobuf.ByteString getFieldName() {
return fieldName_;
}
/**
* required bytes field_name = 1;
*/
public Builder setFieldName(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
fieldName_ = value;
onChanged();
return this;
}
/**
* required bytes field_name = 1;
*/
public Builder clearFieldName() {
bitField0_ = (bitField0_ & ~0x00000001);
fieldName_ = getDefaultInstance().getFieldName();
onChanged();
return this;
}
private long lowerBound_ ;
/**
* required sint64 lower_bound = 2;
*/
public boolean hasLowerBound() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required sint64 lower_bound = 2;
*/
public long getLowerBound() {
return lowerBound_;
}
/**
* required sint64 lower_bound = 2;
*/
public Builder setLowerBound(long value) {
bitField0_ |= 0x00000002;
lowerBound_ = value;
onChanged();
return this;
}
/**
* required sint64 lower_bound = 2;
*/
public Builder clearLowerBound() {
bitField0_ = (bitField0_ & ~0x00000002);
lowerBound_ = 0L;
onChanged();
return this;
}
private boolean lowerBoundInclusive_ ;
/**
* required bool lower_bound_inclusive = 3;
*/
public boolean hasLowerBoundInclusive() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required bool lower_bound_inclusive = 3;
*/
public boolean getLowerBoundInclusive() {
return lowerBoundInclusive_;
}
/**
* required bool lower_bound_inclusive = 3;
*/
public Builder setLowerBoundInclusive(boolean value) {
bitField0_ |= 0x00000004;
lowerBoundInclusive_ = value;
onChanged();
return this;
}
/**
* required bool lower_bound_inclusive = 3;
*/
public Builder clearLowerBoundInclusive() {
bitField0_ = (bitField0_ & ~0x00000004);
lowerBoundInclusive_ = false;
onChanged();
return this;
}
private long upperBound_ ;
/**
* required sint64 upper_bound = 4;
*/
public boolean hasUpperBound() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* required sint64 upper_bound = 4;
*/
public long getUpperBound() {
return upperBound_;
}
/**
* required sint64 upper_bound = 4;
*/
public Builder setUpperBound(long value) {
bitField0_ |= 0x00000008;
upperBound_ = value;
onChanged();
return this;
}
/**
* required sint64 upper_bound = 4;
*/
public Builder clearUpperBound() {
bitField0_ = (bitField0_ & ~0x00000008);
upperBound_ = 0L;
onChanged();
return this;
}
private boolean upperBoundInclusive_ ;
/**
* required bool upper_bound_inclusive = 5;
*/
public boolean hasUpperBoundInclusive() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* required bool upper_bound_inclusive = 5;
*/
public boolean getUpperBoundInclusive() {
return upperBoundInclusive_;
}
/**
* required bool upper_bound_inclusive = 5;
*/
public Builder setUpperBoundInclusive(boolean value) {
bitField0_ |= 0x00000010;
upperBoundInclusive_ = value;
onChanged();
return this;
}
/**
* required bool upper_bound_inclusive = 5;
*/
public Builder clearUpperBoundInclusive() {
bitField0_ = (bitField0_ & ~0x00000010);
upperBoundInclusive_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString desc_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes desc = 6;
*
*
* Some human readable description of the time range
*
*/
public boolean hasDesc() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* required bytes desc = 6;
*
*
* Some human readable description of the time range
*
*/
public com.google.protobuf.ByteString getDesc() {
return desc_;
}
/**
* required bytes desc = 6;
*
*
* Some human readable description of the time range
*
*/
public Builder setDesc(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
desc_ = value;
onChanged();
return this;
}
/**
* required bytes desc = 6;
*
*
* Some human readable description of the time range
*
*/
public Builder clearDesc() {
bitField0_ = (bitField0_ & ~0x00000020);
desc_ = getDefaultInstance().getDesc();
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:TsRange)
}
static {
defaultInstance = new TsRange(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:TsRange)
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsQueryReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsQueryReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsQueryResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsQueryResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsGetReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsGetReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsGetResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsGetResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsPutReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsPutReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsPutResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsPutResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsDelReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsDelReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsDelResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsDelResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsInterpolation_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsInterpolation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsColumnDescription_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsColumnDescription_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsRow_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsRow_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsCell_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsCell_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsListKeysReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsListKeysReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsListKeysResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsListKeysResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsCoverageReq_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsCoverageReq_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsCoverageResp_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsCoverageResp_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsCoverageEntry_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsCoverageEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_TsRange_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_TsRange_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\rriak_ts.proto\032\nriak.proto\"[\n\nTsQueryRe" +
"q\022\037\n\005query\030\001 \001(\0132\020.TsInterpolation\022\025\n\006st" +
"ream\030\002 \001(\010:\005false\022\025\n\rcover_context\030\003 \001(\014" +
"\"^\n\013TsQueryResp\022%\n\007columns\030\001 \003(\0132\024.TsCol" +
"umnDescription\022\024\n\004rows\030\002 \003(\0132\006.TsRow\022\022\n\004" +
"done\030\003 \001(\010:\004true\"@\n\010TsGetReq\022\r\n\005table\030\001 " +
"\002(\014\022\024\n\003key\030\002 \003(\0132\007.TsCell\022\017\n\007timeout\030\003 \001" +
"(\r\"H\n\tTsGetResp\022%\n\007columns\030\001 \003(\0132\024.TsCol" +
"umnDescription\022\024\n\004rows\030\002 \003(\0132\006.TsRow\"V\n\010" +
"TsPutReq\022\r\n\005table\030\001 \002(\014\022%\n\007columns\030\002 \003(\013",
"2\024.TsColumnDescription\022\024\n\004rows\030\003 \003(\0132\006.T" +
"sRow\"\013\n\tTsPutResp\"P\n\010TsDelReq\022\r\n\005table\030\001" +
" \002(\014\022\024\n\003key\030\002 \003(\0132\007.TsCell\022\016\n\006vclock\030\003 \001" +
"(\014\022\017\n\007timeout\030\004 \001(\r\"\013\n\tTsDelResp\"A\n\017TsIn" +
"terpolation\022\014\n\004base\030\001 \002(\014\022 \n\016interpolati" +
"ons\030\002 \003(\0132\010.RpbPair\"@\n\023TsColumnDescripti" +
"on\022\014\n\004name\030\001 \002(\014\022\033\n\004type\030\002 \002(\0162\r.TsColum" +
"nType\"\037\n\005TsRow\022\026\n\005cells\030\001 \003(\0132\007.TsCell\"{" +
"\n\006TsCell\022\025\n\rvarchar_value\030\001 \001(\014\022\024\n\014sint6" +
"4_value\030\002 \001(\022\022\027\n\017timestamp_value\030\003 \001(\022\022\025",
"\n\rboolean_value\030\004 \001(\010\022\024\n\014double_value\030\005 " +
"\001(\001\"/\n\rTsListKeysReq\022\r\n\005table\030\001 \002(\014\022\017\n\007t" +
"imeout\030\002 \001(\r\"4\n\016TsListKeysResp\022\024\n\004keys\030\001" +
" \003(\0132\006.TsRow\022\014\n\004done\030\002 \001(\010\"q\n\rTsCoverage" +
"Req\022\037\n\005query\030\001 \001(\0132\020.TsInterpolation\022\r\n\005" +
"table\030\002 \002(\014\022\025\n\rreplace_cover\030\003 \001(\014\022\031\n\021un" +
"available_cover\030\004 \003(\014\"3\n\016TsCoverageResp\022" +
"!\n\007entries\030\001 \003(\0132\020.TsCoverageEntry\"[\n\017Ts" +
"CoverageEntry\022\n\n\002ip\030\001 \002(\014\022\014\n\004port\030\002 \002(\r\022" +
"\025\n\rcover_context\030\003 \002(\014\022\027\n\005range\030\004 \001(\0132\010.",
"TsRange\"\223\001\n\007TsRange\022\022\n\nfield_name\030\001 \002(\014\022" +
"\023\n\013lower_bound\030\002 \002(\022\022\035\n\025lower_bound_incl" +
"usive\030\003 \002(\010\022\023\n\013upper_bound\030\004 \002(\022\022\035\n\025uppe" +
"r_bound_inclusive\030\005 \002(\010\022\014\n\004desc\030\006 \002(\014*Y\n" +
"\014TsColumnType\022\013\n\007VARCHAR\020\000\022\n\n\006SINT64\020\001\022\n" +
"\n\006DOUBLE\020\002\022\r\n\tTIMESTAMP\020\003\022\013\n\007BOOLEAN\020\004\022\010" +
"\n\004BLOB\020\005B#\n\027com.basho.riak.protobufB\010Ria" +
"kTsPB"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.basho.riak.protobuf.RiakPB.getDescriptor(),
}, assigner);
internal_static_TsQueryReq_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_TsQueryReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsQueryReq_descriptor,
new java.lang.String[] { "Query", "Stream", "CoverContext", });
internal_static_TsQueryResp_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_TsQueryResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsQueryResp_descriptor,
new java.lang.String[] { "Columns", "Rows", "Done", });
internal_static_TsGetReq_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_TsGetReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsGetReq_descriptor,
new java.lang.String[] { "Table", "Key", "Timeout", });
internal_static_TsGetResp_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_TsGetResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsGetResp_descriptor,
new java.lang.String[] { "Columns", "Rows", });
internal_static_TsPutReq_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_TsPutReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsPutReq_descriptor,
new java.lang.String[] { "Table", "Columns", "Rows", });
internal_static_TsPutResp_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_TsPutResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsPutResp_descriptor,
new java.lang.String[] { });
internal_static_TsDelReq_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_TsDelReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsDelReq_descriptor,
new java.lang.String[] { "Table", "Key", "Vclock", "Timeout", });
internal_static_TsDelResp_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_TsDelResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsDelResp_descriptor,
new java.lang.String[] { });
internal_static_TsInterpolation_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_TsInterpolation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsInterpolation_descriptor,
new java.lang.String[] { "Base", "Interpolations", });
internal_static_TsColumnDescription_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_TsColumnDescription_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsColumnDescription_descriptor,
new java.lang.String[] { "Name", "Type", });
internal_static_TsRow_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_TsRow_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsRow_descriptor,
new java.lang.String[] { "Cells", });
internal_static_TsCell_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_TsCell_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsCell_descriptor,
new java.lang.String[] { "VarcharValue", "Sint64Value", "TimestampValue", "BooleanValue", "DoubleValue", });
internal_static_TsListKeysReq_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_TsListKeysReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsListKeysReq_descriptor,
new java.lang.String[] { "Table", "Timeout", });
internal_static_TsListKeysResp_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_TsListKeysResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsListKeysResp_descriptor,
new java.lang.String[] { "Keys", "Done", });
internal_static_TsCoverageReq_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_TsCoverageReq_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsCoverageReq_descriptor,
new java.lang.String[] { "Query", "Table", "ReplaceCover", "UnavailableCover", });
internal_static_TsCoverageResp_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_TsCoverageResp_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsCoverageResp_descriptor,
new java.lang.String[] { "Entries", });
internal_static_TsCoverageEntry_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_TsCoverageEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsCoverageEntry_descriptor,
new java.lang.String[] { "Ip", "Port", "CoverContext", "Range", });
internal_static_TsRange_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_TsRange_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_TsRange_descriptor,
new java.lang.String[] { "FieldName", "LowerBound", "LowerBoundInclusive", "UpperBound", "UpperBoundInclusive", "Desc", });
com.basho.riak.protobuf.RiakPB.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy