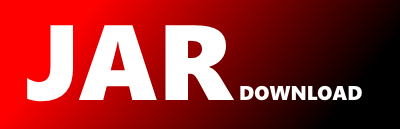
com.basistech.rosette.apimodel.AdmRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2017 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.dm.AnnotatedText;
import com.basistech.util.LanguageCode;
/**
* A request to a document-processing endpoint that supplies the input as partially-annotated
* input in a {@link com.basistech.rosette.dm.AnnotatedText} object.
*/
public final class AdmRequest extends Request {
public static final String ADM_CONTENT_TYPE = "model/vnd.rosette.annotated-data-model";
/**
*/
private final AnnotatedText text;
/**
*/
private final O options;
/**
*/
private final LanguageCode language;
// workaround for inheritance https://github.com/rzwitserloot/lombok/issues/853
public AdmRequest(String profileId, AnnotatedText text, O options, LanguageCode language) {
super(profileId);
this.text = text;
this.options = options;
this.language = language;
}
@SuppressWarnings("all")
public static class AdmRequestBuilder {
@SuppressWarnings("all")
private String profileId;
@SuppressWarnings("all")
private AnnotatedText text;
@SuppressWarnings("all")
private O options;
@SuppressWarnings("all")
private LanguageCode language;
@SuppressWarnings("all")
AdmRequestBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public AdmRequest.AdmRequestBuilder profileId(final String profileId) {
this.profileId = profileId;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public AdmRequest.AdmRequestBuilder text(final AnnotatedText text) {
this.text = text;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public AdmRequest.AdmRequestBuilder options(final O options) {
this.options = options;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public AdmRequest.AdmRequestBuilder language(final LanguageCode language) {
this.language = language;
return this;
}
@SuppressWarnings("all")
public AdmRequest build() {
return new AdmRequest(this.profileId, this.text, this.options, this.language);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "AdmRequest.AdmRequestBuilder(profileId=" + this.profileId + ", text=" + this.text + ", options=" + this.options + ", language=" + this.language + ")";
}
}
@SuppressWarnings("all")
public static AdmRequest.AdmRequestBuilder builder() {
return new AdmRequest.AdmRequestBuilder();
}
/**
* @return {@link com.basistech.rosette.dm.AnnotatedText}
*/
@SuppressWarnings("all")
public AnnotatedText getText() {
return this.text;
}
/**
* @return options
*/
@SuppressWarnings("all")
public O getOptions() {
return this.options;
}
/**
* @return language
*/
@SuppressWarnings("all")
public LanguageCode getLanguage() {
return this.language;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof AdmRequest)) return false;
final AdmRequest> other = (AdmRequest>) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$text = this.getText();
final Object other$text = other.getText();
if (this$text == null ? other$text != null : !this$text.equals(other$text)) return false;
final Object this$options = this.getOptions();
final Object other$options = other.getOptions();
if (this$options == null ? other$options != null : !this$options.equals(other$options)) return false;
final Object this$language = this.getLanguage();
final Object other$language = other.getLanguage();
if (this$language == null ? other$language != null : !this$language.equals(other$language)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof AdmRequest;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $text = this.getText();
result = result * PRIME + ($text == null ? 43 : $text.hashCode());
final Object $options = this.getOptions();
result = result * PRIME + ($options == null ? 43 : $options.hashCode());
final Object $language = this.getLanguage();
result = result * PRIME + ($language == null ? 43 : $language.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "AdmRequest(text=" + this.getText() + ", options=" + this.getOptions() + ", language=" + this.getLanguage() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy