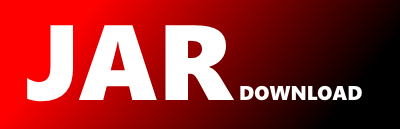
com.basistech.rosette.apimodel.Concept Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2017 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
/**
* Concepts found in a document.
*/
@JacksonMixin
public final class Concept {
/**
*/
private final String phrase;
/**
*/
private final Double salience;
/**
*/
private final String conceptId;
@SuppressWarnings("all")
Concept(final String phrase, final Double salience, final String conceptId) {
this.phrase = phrase;
this.salience = salience;
this.conceptId = conceptId;
}
@SuppressWarnings("all")
public static class ConceptBuilder {
@SuppressWarnings("all")
private String phrase;
@SuppressWarnings("all")
private Double salience;
@SuppressWarnings("all")
private String conceptId;
@SuppressWarnings("all")
ConceptBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Concept.ConceptBuilder phrase(final String phrase) {
this.phrase = phrase;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Concept.ConceptBuilder salience(final Double salience) {
this.salience = salience;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Concept.ConceptBuilder conceptId(final String conceptId) {
this.conceptId = conceptId;
return this;
}
@SuppressWarnings("all")
public Concept build() {
return new Concept(this.phrase, this.salience, this.conceptId);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "Concept.ConceptBuilder(phrase=" + this.phrase + ", salience=" + this.salience + ", conceptId=" + this.conceptId + ")";
}
}
@SuppressWarnings("all")
public static Concept.ConceptBuilder builder() {
return new Concept.ConceptBuilder();
}
/**
* @return the concept text
*/
@SuppressWarnings("all")
public String getPhrase() {
return this.phrase;
}
/**
* @return the concept salience
*/
@SuppressWarnings("all")
public Double getSalience() {
return this.salience;
}
/**
* @return the concept id
*/
@SuppressWarnings("all")
public String getConceptId() {
return this.conceptId;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Concept)) return false;
final Concept other = (Concept) o;
final Object this$salience = this.getSalience();
final Object other$salience = other.getSalience();
if (this$salience == null ? other$salience != null : !this$salience.equals(other$salience)) return false;
final Object this$phrase = this.getPhrase();
final Object other$phrase = other.getPhrase();
if (this$phrase == null ? other$phrase != null : !this$phrase.equals(other$phrase)) return false;
final Object this$conceptId = this.getConceptId();
final Object other$conceptId = other.getConceptId();
if (this$conceptId == null ? other$conceptId != null : !this$conceptId.equals(other$conceptId)) return false;
return true;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $salience = this.getSalience();
result = result * PRIME + ($salience == null ? 43 : $salience.hashCode());
final Object $phrase = this.getPhrase();
result = result * PRIME + ($phrase == null ? 43 : $phrase.hashCode());
final Object $conceptId = this.getConceptId();
result = result * PRIME + ($conceptId == null ? 43 : $conceptId.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "Concept(phrase=" + this.getPhrase() + ", salience=" + this.getSalience() + ", conceptId=" + this.getConceptId() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy