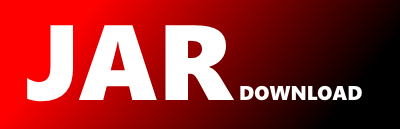
com.basistech.rosette.apimodel.ConfigurationRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*******************************************************************************
* Copyright 2019 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
******************************************************************************/
package com.basistech.rosette.apimodel;
import com.basistech.util.LanguageCode;
public final class ConfigurationRequest extends Request {
private final LanguageCode language;
private final T configuration;
public ConfigurationRequest(String profileId, LanguageCode language, T configuration) {
super(profileId);
this.language = language;
this.configuration = configuration;
}
@SuppressWarnings("all")
public static class ConfigurationRequestBuilder {
@SuppressWarnings("all")
private String profileId;
@SuppressWarnings("all")
private LanguageCode language;
@SuppressWarnings("all")
private T configuration;
@SuppressWarnings("all")
ConfigurationRequestBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public ConfigurationRequest.ConfigurationRequestBuilder profileId(final String profileId) {
this.profileId = profileId;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public ConfigurationRequest.ConfigurationRequestBuilder language(final LanguageCode language) {
this.language = language;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public ConfigurationRequest.ConfigurationRequestBuilder configuration(final T configuration) {
this.configuration = configuration;
return this;
}
@SuppressWarnings("all")
public ConfigurationRequest build() {
return new ConfigurationRequest(this.profileId, this.language, this.configuration);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "ConfigurationRequest.ConfigurationRequestBuilder(profileId=" + this.profileId + ", language=" + this.language + ", configuration=" + this.configuration + ")";
}
}
@SuppressWarnings("all")
public static ConfigurationRequest.ConfigurationRequestBuilder builder() {
return new ConfigurationRequest.ConfigurationRequestBuilder();
}
@SuppressWarnings("all")
public LanguageCode getLanguage() {
return this.language;
}
@SuppressWarnings("all")
public T getConfiguration() {
return this.configuration;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ConfigurationRequest)) return false;
final ConfigurationRequest> other = (ConfigurationRequest>) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$language = this.getLanguage();
final Object other$language = other.getLanguage();
if (this$language == null ? other$language != null : !this$language.equals(other$language)) return false;
final Object this$configuration = this.getConfiguration();
final Object other$configuration = other.getConfiguration();
if (this$configuration == null ? other$configuration != null : !this$configuration.equals(other$configuration)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof ConfigurationRequest;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $language = this.getLanguage();
result = result * PRIME + ($language == null ? 43 : $language.hashCode());
final Object $configuration = this.getConfiguration();
result = result * PRIME + ($configuration == null ? 43 : $configuration.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "ConfigurationRequest(language=" + this.getLanguage() + ", configuration=" + this.getConfiguration() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy