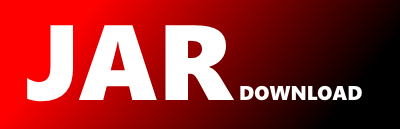
com.basistech.rosette.apimodel.EntitiesOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2022 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
/**
* EntityMention extraction options
*/
@JacksonMixin
public final class EntitiesOptions extends Options {
/**
* Default options
*/
public static final EntitiesOptions DEFAULT = EntitiesOptions.builder().calculateConfidence(false).calculateSalience(false).linkEntities(true).modelType("statistical").enableStructuredRegion(false).build();
/**
*/
private final Boolean calculateConfidence;
/**
*/
private final Boolean calculateSalience;
/**
*/
private final Boolean linkEntities;
/**
*/
private final String linkMentionMode;
/**
*/
private final String modelType;
/**
* @since 1.14.0 (19.08)
*/
private final Boolean includeDBpediaTypes;
/**
*/
private final Boolean includePermID;
/**
*/
private final String rtsDecoder;
/**
*/
private final String caseSensitivity;
/**
*/
private final Boolean enableStructuredRegion;
/**
*/
private final String structuredRegionProcessingType;
/**
*/
private final Boolean regexCurrencySplit;
/**
*/
private final Boolean useIndocServer;
@SuppressWarnings("all")
EntitiesOptions(final Boolean calculateConfidence, final Boolean calculateSalience, final Boolean linkEntities, final String linkMentionMode, final String modelType, final Boolean includeDBpediaTypes, final Boolean includePermID, final String rtsDecoder, final String caseSensitivity, final Boolean enableStructuredRegion, final String structuredRegionProcessingType, final Boolean regexCurrencySplit, final Boolean useIndocServer) {
this.calculateConfidence = calculateConfidence;
this.calculateSalience = calculateSalience;
this.linkEntities = linkEntities;
this.linkMentionMode = linkMentionMode;
this.modelType = modelType;
this.includeDBpediaTypes = includeDBpediaTypes;
this.includePermID = includePermID;
this.rtsDecoder = rtsDecoder;
this.caseSensitivity = caseSensitivity;
this.enableStructuredRegion = enableStructuredRegion;
this.structuredRegionProcessingType = structuredRegionProcessingType;
this.regexCurrencySplit = regexCurrencySplit;
this.useIndocServer = useIndocServer;
}
@SuppressWarnings("all")
public static class EntitiesOptionsBuilder {
@SuppressWarnings("all")
private Boolean calculateConfidence;
@SuppressWarnings("all")
private Boolean calculateSalience;
@SuppressWarnings("all")
private Boolean linkEntities;
@SuppressWarnings("all")
private String linkMentionMode;
@SuppressWarnings("all")
private String modelType;
@SuppressWarnings("all")
private Boolean includeDBpediaTypes;
@SuppressWarnings("all")
private Boolean includePermID;
@SuppressWarnings("all")
private String rtsDecoder;
@SuppressWarnings("all")
private String caseSensitivity;
@SuppressWarnings("all")
private Boolean enableStructuredRegion;
@SuppressWarnings("all")
private String structuredRegionProcessingType;
@SuppressWarnings("all")
private Boolean regexCurrencySplit;
@SuppressWarnings("all")
private Boolean useIndocServer;
@SuppressWarnings("all")
EntitiesOptionsBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder calculateConfidence(final Boolean calculateConfidence) {
this.calculateConfidence = calculateConfidence;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder calculateSalience(final Boolean calculateSalience) {
this.calculateSalience = calculateSalience;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder linkEntities(final Boolean linkEntities) {
this.linkEntities = linkEntities;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder linkMentionMode(final String linkMentionMode) {
this.linkMentionMode = linkMentionMode;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder modelType(final String modelType) {
this.modelType = modelType;
return this;
}
/**
* @since 1.14.0 (19.08)
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder includeDBpediaTypes(final Boolean includeDBpediaTypes) {
this.includeDBpediaTypes = includeDBpediaTypes;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder includePermID(final Boolean includePermID) {
this.includePermID = includePermID;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder rtsDecoder(final String rtsDecoder) {
this.rtsDecoder = rtsDecoder;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder caseSensitivity(final String caseSensitivity) {
this.caseSensitivity = caseSensitivity;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder enableStructuredRegion(final Boolean enableStructuredRegion) {
this.enableStructuredRegion = enableStructuredRegion;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder structuredRegionProcessingType(final String structuredRegionProcessingType) {
this.structuredRegionProcessingType = structuredRegionProcessingType;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder regexCurrencySplit(final Boolean regexCurrencySplit) {
this.regexCurrencySplit = regexCurrencySplit;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public EntitiesOptions.EntitiesOptionsBuilder useIndocServer(final Boolean useIndocServer) {
this.useIndocServer = useIndocServer;
return this;
}
@SuppressWarnings("all")
public EntitiesOptions build() {
return new EntitiesOptions(this.calculateConfidence, this.calculateSalience, this.linkEntities, this.linkMentionMode, this.modelType, this.includeDBpediaTypes, this.includePermID, this.rtsDecoder, this.caseSensitivity, this.enableStructuredRegion, this.structuredRegionProcessingType, this.regexCurrencySplit, this.useIndocServer);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "EntitiesOptions.EntitiesOptionsBuilder(calculateConfidence=" + this.calculateConfidence + ", calculateSalience=" + this.calculateSalience + ", linkEntities=" + this.linkEntities + ", linkMentionMode=" + this.linkMentionMode + ", modelType=" + this.modelType + ", includeDBpediaTypes=" + this.includeDBpediaTypes + ", includePermID=" + this.includePermID + ", rtsDecoder=" + this.rtsDecoder + ", caseSensitivity=" + this.caseSensitivity + ", enableStructuredRegion=" + this.enableStructuredRegion + ", structuredRegionProcessingType=" + this.structuredRegionProcessingType + ", regexCurrencySplit=" + this.regexCurrencySplit + ", useIndocServer=" + this.useIndocServer + ")";
}
}
@SuppressWarnings("all")
public static EntitiesOptions.EntitiesOptionsBuilder builder() {
return new EntitiesOptions.EntitiesOptionsBuilder();
}
/**
* @return the calculateConfidence flag.
*/
@SuppressWarnings("all")
public Boolean getCalculateConfidence() {
return this.calculateConfidence;
}
/**
* @return the calculateSalience flag.
*/
@SuppressWarnings("all")
public Boolean getCalculateSalience() {
return this.calculateSalience;
}
/**
* @return the linkEntities flag.
*/
@SuppressWarnings("all")
public Boolean getLinkEntities() {
return this.linkEntities;
}
/**
* @return the linkMentionMode mode.
*/
@SuppressWarnings("all")
public String getLinkMentionMode() {
return this.linkMentionMode;
}
/**
* @return the modelType to use.
*/
@SuppressWarnings("all")
public String getModelType() {
return this.modelType;
}
/**
* @since 1.14.0 (19.08)
* @return the includeDBpediaType flag.
*/
@SuppressWarnings("all")
public Boolean getIncludeDBpediaTypes() {
return this.includeDBpediaTypes;
}
/**
* @return the includePermID flag.
*/
@SuppressWarnings("all")
public Boolean getIncludePermID() {
return this.includePermID;
}
/**
* @return the RTS workspace id.
*/
@SuppressWarnings("all")
public String getRtsDecoder() {
return this.rtsDecoder;
}
/**
* @return case sensitivity of model to use. Can be one of caseSensitive, caseInsensitive or automatic.
*/
@SuppressWarnings("all")
public String getCaseSensitivity() {
return this.caseSensitivity;
}
/**
* @return the enableStructuredRegion flag.
*/
@SuppressWarnings("all")
public Boolean getEnableStructuredRegion() {
return this.enableStructuredRegion;
}
/**
* @return the structuredRegionProcessingType flag. Can be one of none, nerModel, nameClassifier
*/
@SuppressWarnings("all")
public String getStructuredRegionProcessingType() {
return this.structuredRegionProcessingType;
}
/**
* @return the regexCurrencySplit flag.
* If enabled, will cause MONEY regular expression entity extractions to be split into two:
* CURRENCY:AMT and CURRENCY:TYPE
*/
@SuppressWarnings("all")
public Boolean getRegexCurrencySplit() {
return this.regexCurrencySplit;
}
/**
* @return useIndocServer flag. If true, REX will make request to indoc-coref-server and merge
* results with existing entities.
* default false
*/
@SuppressWarnings("all")
public Boolean getUseIndocServer() {
return this.useIndocServer;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof EntitiesOptions)) return false;
final EntitiesOptions other = (EntitiesOptions) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$calculateConfidence = this.getCalculateConfidence();
final Object other$calculateConfidence = other.getCalculateConfidence();
if (this$calculateConfidence == null ? other$calculateConfidence != null : !this$calculateConfidence.equals(other$calculateConfidence)) return false;
final Object this$calculateSalience = this.getCalculateSalience();
final Object other$calculateSalience = other.getCalculateSalience();
if (this$calculateSalience == null ? other$calculateSalience != null : !this$calculateSalience.equals(other$calculateSalience)) return false;
final Object this$linkEntities = this.getLinkEntities();
final Object other$linkEntities = other.getLinkEntities();
if (this$linkEntities == null ? other$linkEntities != null : !this$linkEntities.equals(other$linkEntities)) return false;
final Object this$includeDBpediaTypes = this.getIncludeDBpediaTypes();
final Object other$includeDBpediaTypes = other.getIncludeDBpediaTypes();
if (this$includeDBpediaTypes == null ? other$includeDBpediaTypes != null : !this$includeDBpediaTypes.equals(other$includeDBpediaTypes)) return false;
final Object this$includePermID = this.getIncludePermID();
final Object other$includePermID = other.getIncludePermID();
if (this$includePermID == null ? other$includePermID != null : !this$includePermID.equals(other$includePermID)) return false;
final Object this$enableStructuredRegion = this.getEnableStructuredRegion();
final Object other$enableStructuredRegion = other.getEnableStructuredRegion();
if (this$enableStructuredRegion == null ? other$enableStructuredRegion != null : !this$enableStructuredRegion.equals(other$enableStructuredRegion)) return false;
final Object this$regexCurrencySplit = this.getRegexCurrencySplit();
final Object other$regexCurrencySplit = other.getRegexCurrencySplit();
if (this$regexCurrencySplit == null ? other$regexCurrencySplit != null : !this$regexCurrencySplit.equals(other$regexCurrencySplit)) return false;
final Object this$useIndocServer = this.getUseIndocServer();
final Object other$useIndocServer = other.getUseIndocServer();
if (this$useIndocServer == null ? other$useIndocServer != null : !this$useIndocServer.equals(other$useIndocServer)) return false;
final Object this$linkMentionMode = this.getLinkMentionMode();
final Object other$linkMentionMode = other.getLinkMentionMode();
if (this$linkMentionMode == null ? other$linkMentionMode != null : !this$linkMentionMode.equals(other$linkMentionMode)) return false;
final Object this$modelType = this.getModelType();
final Object other$modelType = other.getModelType();
if (this$modelType == null ? other$modelType != null : !this$modelType.equals(other$modelType)) return false;
final Object this$rtsDecoder = this.getRtsDecoder();
final Object other$rtsDecoder = other.getRtsDecoder();
if (this$rtsDecoder == null ? other$rtsDecoder != null : !this$rtsDecoder.equals(other$rtsDecoder)) return false;
final Object this$caseSensitivity = this.getCaseSensitivity();
final Object other$caseSensitivity = other.getCaseSensitivity();
if (this$caseSensitivity == null ? other$caseSensitivity != null : !this$caseSensitivity.equals(other$caseSensitivity)) return false;
final Object this$structuredRegionProcessingType = this.getStructuredRegionProcessingType();
final Object other$structuredRegionProcessingType = other.getStructuredRegionProcessingType();
if (this$structuredRegionProcessingType == null ? other$structuredRegionProcessingType != null : !this$structuredRegionProcessingType.equals(other$structuredRegionProcessingType)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof EntitiesOptions;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $calculateConfidence = this.getCalculateConfidence();
result = result * PRIME + ($calculateConfidence == null ? 43 : $calculateConfidence.hashCode());
final Object $calculateSalience = this.getCalculateSalience();
result = result * PRIME + ($calculateSalience == null ? 43 : $calculateSalience.hashCode());
final Object $linkEntities = this.getLinkEntities();
result = result * PRIME + ($linkEntities == null ? 43 : $linkEntities.hashCode());
final Object $includeDBpediaTypes = this.getIncludeDBpediaTypes();
result = result * PRIME + ($includeDBpediaTypes == null ? 43 : $includeDBpediaTypes.hashCode());
final Object $includePermID = this.getIncludePermID();
result = result * PRIME + ($includePermID == null ? 43 : $includePermID.hashCode());
final Object $enableStructuredRegion = this.getEnableStructuredRegion();
result = result * PRIME + ($enableStructuredRegion == null ? 43 : $enableStructuredRegion.hashCode());
final Object $regexCurrencySplit = this.getRegexCurrencySplit();
result = result * PRIME + ($regexCurrencySplit == null ? 43 : $regexCurrencySplit.hashCode());
final Object $useIndocServer = this.getUseIndocServer();
result = result * PRIME + ($useIndocServer == null ? 43 : $useIndocServer.hashCode());
final Object $linkMentionMode = this.getLinkMentionMode();
result = result * PRIME + ($linkMentionMode == null ? 43 : $linkMentionMode.hashCode());
final Object $modelType = this.getModelType();
result = result * PRIME + ($modelType == null ? 43 : $modelType.hashCode());
final Object $rtsDecoder = this.getRtsDecoder();
result = result * PRIME + ($rtsDecoder == null ? 43 : $rtsDecoder.hashCode());
final Object $caseSensitivity = this.getCaseSensitivity();
result = result * PRIME + ($caseSensitivity == null ? 43 : $caseSensitivity.hashCode());
final Object $structuredRegionProcessingType = this.getStructuredRegionProcessingType();
result = result * PRIME + ($structuredRegionProcessingType == null ? 43 : $structuredRegionProcessingType.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "EntitiesOptions(calculateConfidence=" + this.getCalculateConfidence() + ", calculateSalience=" + this.getCalculateSalience() + ", linkEntities=" + this.getLinkEntities() + ", linkMentionMode=" + this.getLinkMentionMode() + ", modelType=" + this.getModelType() + ", includeDBpediaTypes=" + this.getIncludeDBpediaTypes() + ", includePermID=" + this.getIncludePermID() + ", rtsDecoder=" + this.getRtsDecoder() + ", caseSensitivity=" + this.getCaseSensitivity() + ", enableStructuredRegion=" + this.getEnableStructuredRegion() + ", structuredRegionProcessingType=" + this.getStructuredRegionProcessingType() + ", regexCurrencySplit=" + this.getRegexCurrencySplit() + ", useIndocServer=" + this.getUseIndocServer() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy