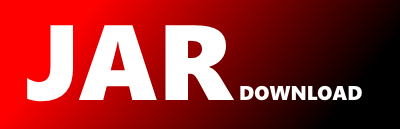
com.basistech.rosette.apimodel.ErrorResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2024 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
/**
* Analytics API response error data
*/
@JacksonMixin
public class ErrorResponse extends Response {
/**
*/
private String code;
/**
*/
private String message;
@SuppressWarnings("all")
ErrorResponse(final String code, final String message) {
this.code = code;
this.message = message;
}
@SuppressWarnings("all")
public static class ErrorResponseBuilder {
@SuppressWarnings("all")
private String code;
@SuppressWarnings("all")
private String message;
@SuppressWarnings("all")
ErrorResponseBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public ErrorResponse.ErrorResponseBuilder code(final String code) {
this.code = code;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public ErrorResponse.ErrorResponseBuilder message(final String message) {
this.message = message;
return this;
}
@SuppressWarnings("all")
public ErrorResponse build() {
return new ErrorResponse(this.code, this.message);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "ErrorResponse.ErrorResponseBuilder(code=" + this.code + ", message=" + this.message + ")";
}
}
@SuppressWarnings("all")
public static ErrorResponse.ErrorResponseBuilder builder() {
return new ErrorResponse.ErrorResponseBuilder();
}
/**
* @return the error code
*/
@SuppressWarnings("all")
public String getCode() {
return this.code;
}
/**
* @return the error message
*/
@SuppressWarnings("all")
public String getMessage() {
return this.message;
}
/**
*/
@SuppressWarnings("all")
public void setCode(final String code) {
this.code = code;
}
/**
*/
@SuppressWarnings("all")
public void setMessage(final String message) {
this.message = message;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "ErrorResponse(code=" + this.getCode() + ", message=" + this.getMessage() + ")";
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof ErrorResponse)) return false;
final ErrorResponse other = (ErrorResponse) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$code = this.getCode();
final Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final Object this$message = this.getMessage();
final Object other$message = other.getMessage();
if (this$message == null ? other$message != null : !this$message.equals(other$message)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof ErrorResponse;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final Object $message = this.getMessage();
result = result * PRIME + ($message == null ? 43 : $message.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy