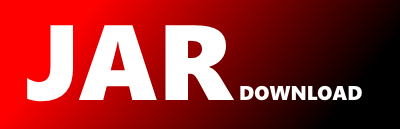
com.basistech.rosette.apimodel.FieldedAddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2020 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
public final class FieldedAddress implements IAddress {
/**
*/
private final String house;
/**
*/
private final String houseNumber;
/**
*/
private final String road;
/**
*/
private final String unit;
/**
*/
private final String level;
/**
*/
private final String staircase;
/**
*/
private final String entrance;
/**
*/
private final String suburb;
/**
*/
private final String cityDistrict;
/**
*/
private final String city;
/**
*/
private final String island;
/**
*/
private final String stateDistrict;
/**
*/
private final String state;
/**
*/
private final String countryRegion;
/**
*/
private final String country;
/**
*/
private final String worldRegion;
/**
*/
private final String postCode;
/**
*/
private final String poBox;
@Override
public boolean fielded() {
return true;
}
@SuppressWarnings("all")
FieldedAddress(final String house, final String houseNumber, final String road, final String unit, final String level, final String staircase, final String entrance, final String suburb, final String cityDistrict, final String city, final String island, final String stateDistrict, final String state, final String countryRegion, final String country, final String worldRegion, final String postCode, final String poBox) {
this.house = house;
this.houseNumber = houseNumber;
this.road = road;
this.unit = unit;
this.level = level;
this.staircase = staircase;
this.entrance = entrance;
this.suburb = suburb;
this.cityDistrict = cityDistrict;
this.city = city;
this.island = island;
this.stateDistrict = stateDistrict;
this.state = state;
this.countryRegion = countryRegion;
this.country = country;
this.worldRegion = worldRegion;
this.postCode = postCode;
this.poBox = poBox;
}
@SuppressWarnings("all")
public static class FieldedAddressBuilder {
@SuppressWarnings("all")
private String house;
@SuppressWarnings("all")
private String houseNumber;
@SuppressWarnings("all")
private String road;
@SuppressWarnings("all")
private String unit;
@SuppressWarnings("all")
private String level;
@SuppressWarnings("all")
private String staircase;
@SuppressWarnings("all")
private String entrance;
@SuppressWarnings("all")
private String suburb;
@SuppressWarnings("all")
private String cityDistrict;
@SuppressWarnings("all")
private String city;
@SuppressWarnings("all")
private String island;
@SuppressWarnings("all")
private String stateDistrict;
@SuppressWarnings("all")
private String state;
@SuppressWarnings("all")
private String countryRegion;
@SuppressWarnings("all")
private String country;
@SuppressWarnings("all")
private String worldRegion;
@SuppressWarnings("all")
private String postCode;
@SuppressWarnings("all")
private String poBox;
@SuppressWarnings("all")
FieldedAddressBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder house(final String house) {
this.house = house;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder houseNumber(final String houseNumber) {
this.houseNumber = houseNumber;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder road(final String road) {
this.road = road;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder unit(final String unit) {
this.unit = unit;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder level(final String level) {
this.level = level;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder staircase(final String staircase) {
this.staircase = staircase;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder entrance(final String entrance) {
this.entrance = entrance;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder suburb(final String suburb) {
this.suburb = suburb;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder cityDistrict(final String cityDistrict) {
this.cityDistrict = cityDistrict;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder city(final String city) {
this.city = city;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder island(final String island) {
this.island = island;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder stateDistrict(final String stateDistrict) {
this.stateDistrict = stateDistrict;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder state(final String state) {
this.state = state;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder countryRegion(final String countryRegion) {
this.countryRegion = countryRegion;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder country(final String country) {
this.country = country;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder worldRegion(final String worldRegion) {
this.worldRegion = worldRegion;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder postCode(final String postCode) {
this.postCode = postCode;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public FieldedAddress.FieldedAddressBuilder poBox(final String poBox) {
this.poBox = poBox;
return this;
}
@SuppressWarnings("all")
public FieldedAddress build() {
return new FieldedAddress(this.house, this.houseNumber, this.road, this.unit, this.level, this.staircase, this.entrance, this.suburb, this.cityDistrict, this.city, this.island, this.stateDistrict, this.state, this.countryRegion, this.country, this.worldRegion, this.postCode, this.poBox);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "FieldedAddress.FieldedAddressBuilder(house=" + this.house + ", houseNumber=" + this.houseNumber + ", road=" + this.road + ", unit=" + this.unit + ", level=" + this.level + ", staircase=" + this.staircase + ", entrance=" + this.entrance + ", suburb=" + this.suburb + ", cityDistrict=" + this.cityDistrict + ", city=" + this.city + ", island=" + this.island + ", stateDistrict=" + this.stateDistrict + ", state=" + this.state + ", countryRegion=" + this.countryRegion + ", country=" + this.country + ", worldRegion=" + this.worldRegion + ", postCode=" + this.postCode + ", poBox=" + this.poBox + ")";
}
}
@SuppressWarnings("all")
public static FieldedAddress.FieldedAddressBuilder builder() {
return new FieldedAddress.FieldedAddressBuilder();
}
/**
* @return the address house
*/
@SuppressWarnings("all")
public String getHouse() {
return this.house;
}
/**
* @return the address house number
*/
@SuppressWarnings("all")
public String getHouseNumber() {
return this.houseNumber;
}
/**
* @return the address road
*/
@SuppressWarnings("all")
public String getRoad() {
return this.road;
}
/**
* @return the address unit
*/
@SuppressWarnings("all")
public String getUnit() {
return this.unit;
}
/**
* @return the address level
*/
@SuppressWarnings("all")
public String getLevel() {
return this.level;
}
/**
* @return the address staircase
*/
@SuppressWarnings("all")
public String getStaircase() {
return this.staircase;
}
/**
* @return the address entrance
*/
@SuppressWarnings("all")
public String getEntrance() {
return this.entrance;
}
/**
* @return the address suburb
*/
@SuppressWarnings("all")
public String getSuburb() {
return this.suburb;
}
/**
* @return the address city district
*/
@SuppressWarnings("all")
public String getCityDistrict() {
return this.cityDistrict;
}
/**
* @return the address city
*/
@SuppressWarnings("all")
public String getCity() {
return this.city;
}
/**
* @return the address island
*/
@SuppressWarnings("all")
public String getIsland() {
return this.island;
}
/**
* @return the address state district
*/
@SuppressWarnings("all")
public String getStateDistrict() {
return this.stateDistrict;
}
/**
* @return the address state
*/
@SuppressWarnings("all")
public String getState() {
return this.state;
}
/**
* @return the address country region
*/
@SuppressWarnings("all")
public String getCountryRegion() {
return this.countryRegion;
}
/**
* @return the address country
*/
@SuppressWarnings("all")
public String getCountry() {
return this.country;
}
/**
* @return the address world region
*/
@SuppressWarnings("all")
public String getWorldRegion() {
return this.worldRegion;
}
/**
* @return the address postal code
*/
@SuppressWarnings("all")
public String getPostCode() {
return this.postCode;
}
/**
* @return the address P.O. Box
*/
@SuppressWarnings("all")
public String getPoBox() {
return this.poBox;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof FieldedAddress)) return false;
final FieldedAddress other = (FieldedAddress) o;
final Object this$house = this.getHouse();
final Object other$house = other.getHouse();
if (this$house == null ? other$house != null : !this$house.equals(other$house)) return false;
final Object this$houseNumber = this.getHouseNumber();
final Object other$houseNumber = other.getHouseNumber();
if (this$houseNumber == null ? other$houseNumber != null : !this$houseNumber.equals(other$houseNumber)) return false;
final Object this$road = this.getRoad();
final Object other$road = other.getRoad();
if (this$road == null ? other$road != null : !this$road.equals(other$road)) return false;
final Object this$unit = this.getUnit();
final Object other$unit = other.getUnit();
if (this$unit == null ? other$unit != null : !this$unit.equals(other$unit)) return false;
final Object this$level = this.getLevel();
final Object other$level = other.getLevel();
if (this$level == null ? other$level != null : !this$level.equals(other$level)) return false;
final Object this$staircase = this.getStaircase();
final Object other$staircase = other.getStaircase();
if (this$staircase == null ? other$staircase != null : !this$staircase.equals(other$staircase)) return false;
final Object this$entrance = this.getEntrance();
final Object other$entrance = other.getEntrance();
if (this$entrance == null ? other$entrance != null : !this$entrance.equals(other$entrance)) return false;
final Object this$suburb = this.getSuburb();
final Object other$suburb = other.getSuburb();
if (this$suburb == null ? other$suburb != null : !this$suburb.equals(other$suburb)) return false;
final Object this$cityDistrict = this.getCityDistrict();
final Object other$cityDistrict = other.getCityDistrict();
if (this$cityDistrict == null ? other$cityDistrict != null : !this$cityDistrict.equals(other$cityDistrict)) return false;
final Object this$city = this.getCity();
final Object other$city = other.getCity();
if (this$city == null ? other$city != null : !this$city.equals(other$city)) return false;
final Object this$island = this.getIsland();
final Object other$island = other.getIsland();
if (this$island == null ? other$island != null : !this$island.equals(other$island)) return false;
final Object this$stateDistrict = this.getStateDistrict();
final Object other$stateDistrict = other.getStateDistrict();
if (this$stateDistrict == null ? other$stateDistrict != null : !this$stateDistrict.equals(other$stateDistrict)) return false;
final Object this$state = this.getState();
final Object other$state = other.getState();
if (this$state == null ? other$state != null : !this$state.equals(other$state)) return false;
final Object this$countryRegion = this.getCountryRegion();
final Object other$countryRegion = other.getCountryRegion();
if (this$countryRegion == null ? other$countryRegion != null : !this$countryRegion.equals(other$countryRegion)) return false;
final Object this$country = this.getCountry();
final Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
final Object this$worldRegion = this.getWorldRegion();
final Object other$worldRegion = other.getWorldRegion();
if (this$worldRegion == null ? other$worldRegion != null : !this$worldRegion.equals(other$worldRegion)) return false;
final Object this$postCode = this.getPostCode();
final Object other$postCode = other.getPostCode();
if (this$postCode == null ? other$postCode != null : !this$postCode.equals(other$postCode)) return false;
final Object this$poBox = this.getPoBox();
final Object other$poBox = other.getPoBox();
if (this$poBox == null ? other$poBox != null : !this$poBox.equals(other$poBox)) return false;
return true;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $house = this.getHouse();
result = result * PRIME + ($house == null ? 43 : $house.hashCode());
final Object $houseNumber = this.getHouseNumber();
result = result * PRIME + ($houseNumber == null ? 43 : $houseNumber.hashCode());
final Object $road = this.getRoad();
result = result * PRIME + ($road == null ? 43 : $road.hashCode());
final Object $unit = this.getUnit();
result = result * PRIME + ($unit == null ? 43 : $unit.hashCode());
final Object $level = this.getLevel();
result = result * PRIME + ($level == null ? 43 : $level.hashCode());
final Object $staircase = this.getStaircase();
result = result * PRIME + ($staircase == null ? 43 : $staircase.hashCode());
final Object $entrance = this.getEntrance();
result = result * PRIME + ($entrance == null ? 43 : $entrance.hashCode());
final Object $suburb = this.getSuburb();
result = result * PRIME + ($suburb == null ? 43 : $suburb.hashCode());
final Object $cityDistrict = this.getCityDistrict();
result = result * PRIME + ($cityDistrict == null ? 43 : $cityDistrict.hashCode());
final Object $city = this.getCity();
result = result * PRIME + ($city == null ? 43 : $city.hashCode());
final Object $island = this.getIsland();
result = result * PRIME + ($island == null ? 43 : $island.hashCode());
final Object $stateDistrict = this.getStateDistrict();
result = result * PRIME + ($stateDistrict == null ? 43 : $stateDistrict.hashCode());
final Object $state = this.getState();
result = result * PRIME + ($state == null ? 43 : $state.hashCode());
final Object $countryRegion = this.getCountryRegion();
result = result * PRIME + ($countryRegion == null ? 43 : $countryRegion.hashCode());
final Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
final Object $worldRegion = this.getWorldRegion();
result = result * PRIME + ($worldRegion == null ? 43 : $worldRegion.hashCode());
final Object $postCode = this.getPostCode();
result = result * PRIME + ($postCode == null ? 43 : $postCode.hashCode());
final Object $poBox = this.getPoBox();
result = result * PRIME + ($poBox == null ? 43 : $poBox.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "FieldedAddress(house=" + this.getHouse() + ", houseNumber=" + this.getHouseNumber() + ", road=" + this.getRoad() + ", unit=" + this.getUnit() + ", level=" + this.getLevel() + ", staircase=" + this.getStaircase() + ", entrance=" + this.getEntrance() + ", suburb=" + this.getSuburb() + ", cityDistrict=" + this.getCityDistrict() + ", city=" + this.getCity() + ", island=" + this.getIsland() + ", stateDistrict=" + this.getStateDistrict() + ", state=" + this.getState() + ", countryRegion=" + this.getCountryRegion() + ", country=" + this.getCountry() + ", worldRegion=" + this.getWorldRegion() + ", postCode=" + this.getPostCode() + ", poBox=" + this.getPoBox() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy