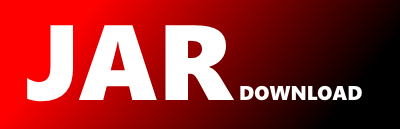
com.basistech.rosette.apimodel.LanguageOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2022 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
import com.basistech.rosette.util.EncodingCode;
import com.basistech.util.LanguageCode;
import jakarta.validation.Valid;
import jakarta.validation.constraints.DecimalMax;
import jakarta.validation.constraints.DecimalMin;
import jakarta.validation.constraints.Min;
import java.util.Set;
/**
* Languages detection options
*/
@JacksonMixin
public final class LanguageOptions extends Options {
/**
*/
private final Boolean multilingual;
/**
*/
@Min(1)
private final Integer minValidChars;
/**
*/
@Min(1)
private final Integer profileDepth;
/**
*/
@DecimalMin("0.0")
@DecimalMax("100.0")
private final Double ambiguityThreshold;
/**
*/
@DecimalMin("0.0")
@DecimalMax("100.0")
private final Double invalidityThreshold;
/**
*/
private final LanguageCode languageHint;
/**
*/
@DecimalMin("1.0")
@DecimalMax("99.0")
private final Double languageHintWeight;
/**
*/
private final EncodingCode encodingHint;
/**
*/
@DecimalMin("1.0")
@DecimalMax("100.0")
private final Double encodingHintWeight;
/**
*/
@Valid
private final Set languageWeightAdjustments;
/**
*/
private final Boolean koreanDialects;
@SuppressWarnings("all")
LanguageOptions(final Boolean multilingual, final Integer minValidChars, final Integer profileDepth, final Double ambiguityThreshold, final Double invalidityThreshold, final LanguageCode languageHint, final Double languageHintWeight, final EncodingCode encodingHint, final Double encodingHintWeight, final Set languageWeightAdjustments, final Boolean koreanDialects) {
this.multilingual = multilingual;
this.minValidChars = minValidChars;
this.profileDepth = profileDepth;
this.ambiguityThreshold = ambiguityThreshold;
this.invalidityThreshold = invalidityThreshold;
this.languageHint = languageHint;
this.languageHintWeight = languageHintWeight;
this.encodingHint = encodingHint;
this.encodingHintWeight = encodingHintWeight;
this.languageWeightAdjustments = languageWeightAdjustments;
this.koreanDialects = koreanDialects;
}
@SuppressWarnings("all")
public static class LanguageOptionsBuilder {
@SuppressWarnings("all")
private Boolean multilingual;
@SuppressWarnings("all")
private Integer minValidChars;
@SuppressWarnings("all")
private Integer profileDepth;
@SuppressWarnings("all")
private Double ambiguityThreshold;
@SuppressWarnings("all")
private Double invalidityThreshold;
@SuppressWarnings("all")
private LanguageCode languageHint;
@SuppressWarnings("all")
private Double languageHintWeight;
@SuppressWarnings("all")
private EncodingCode encodingHint;
@SuppressWarnings("all")
private Double encodingHintWeight;
@SuppressWarnings("all")
private Set languageWeightAdjustments;
@SuppressWarnings("all")
private Boolean koreanDialects;
@SuppressWarnings("all")
LanguageOptionsBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder multilingual(final Boolean multilingual) {
this.multilingual = multilingual;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder minValidChars(final Integer minValidChars) {
this.minValidChars = minValidChars;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder profileDepth(final Integer profileDepth) {
this.profileDepth = profileDepth;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder ambiguityThreshold(final Double ambiguityThreshold) {
this.ambiguityThreshold = ambiguityThreshold;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder invalidityThreshold(final Double invalidityThreshold) {
this.invalidityThreshold = invalidityThreshold;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder languageHint(final LanguageCode languageHint) {
this.languageHint = languageHint;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder languageHintWeight(final Double languageHintWeight) {
this.languageHintWeight = languageHintWeight;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder encodingHint(final EncodingCode encodingHint) {
this.encodingHint = encodingHint;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder encodingHintWeight(final Double encodingHintWeight) {
this.encodingHintWeight = encodingHintWeight;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder languageWeightAdjustments(final Set languageWeightAdjustments) {
this.languageWeightAdjustments = languageWeightAdjustments;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageOptions.LanguageOptionsBuilder koreanDialects(final Boolean koreanDialects) {
this.koreanDialects = koreanDialects;
return this;
}
@SuppressWarnings("all")
public LanguageOptions build() {
return new LanguageOptions(this.multilingual, this.minValidChars, this.profileDepth, this.ambiguityThreshold, this.invalidityThreshold, this.languageHint, this.languageHintWeight, this.encodingHint, this.encodingHintWeight, this.languageWeightAdjustments, this.koreanDialects);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "LanguageOptions.LanguageOptionsBuilder(multilingual=" + this.multilingual + ", minValidChars=" + this.minValidChars + ", profileDepth=" + this.profileDepth + ", ambiguityThreshold=" + this.ambiguityThreshold + ", invalidityThreshold=" + this.invalidityThreshold + ", languageHint=" + this.languageHint + ", languageHintWeight=" + this.languageHintWeight + ", encodingHint=" + this.encodingHint + ", encodingHintWeight=" + this.encodingHintWeight + ", languageWeightAdjustments=" + this.languageWeightAdjustments + ", koreanDialects=" + this.koreanDialects + ")";
}
}
@SuppressWarnings("all")
public static LanguageOptions.LanguageOptionsBuilder builder() {
return new LanguageOptions.LanguageOptionsBuilder();
}
/**
* @return whether to detect language regions
*/
@SuppressWarnings("all")
public Boolean getMultilingual() {
return this.multilingual;
}
/**
* @return minimum number of valid characters
*/
@SuppressWarnings("all")
public Integer getMinValidChars() {
return this.minValidChars;
}
/**
* @return the most frequent n-grams to consider in detection, smaller depth results in faster operation but
* lower detection accuracy
*/
@SuppressWarnings("all")
public Integer getProfileDepth() {
return this.profileDepth;
}
/**
* @return number of n-gram distance ambiguity threshold (0.0-100.0), input profile distances closer to each
* other than this threshold are deemed ambiguous
*/
@SuppressWarnings("all")
public Double getAmbiguityThreshold() {
return this.ambiguityThreshold;
}
/**
* @return number of n-gram distance invalidity threshold (0.0-100.0), input profile distance exceeding this
* threshold will be deemed invalid
*/
@SuppressWarnings("all")
public Double getInvalidityThreshold() {
return this.invalidityThreshold;
}
/**
* @return language hint
*/
@SuppressWarnings("all")
public LanguageCode getLanguageHint() {
return this.languageHint;
}
/**
* @return the language hint weight (1.0-99.0) used to help resolve ambiguous results.
* A value of N reduces the distance of correctly hinted ambiguous result by N%.
* Value of 1.0 is the lightest hint, value of 99.0 the strongest.
*/
@SuppressWarnings("all")
public Double getLanguageHintWeight() {
return this.languageHintWeight;
}
/**
* @return the encoding hint used to help resolve ambiguous results
*/
@SuppressWarnings("all")
public EncodingCode getEncodingHint() {
return this.encodingHint;
}
/**
* @return the encoding hint weight (1.0-100.0) used to help resolve ambiguous results.
* A value of N reduces the distance of correctly hinted result by N%.
* A value of 100 forces the detector to consider only the results with the hinted encoding.
*/
@SuppressWarnings("all")
public Double getEncodingHintWeight() {
return this.encodingHintWeight;
}
/**
* @return languageWeightAdjustments the set of language weight adjustments
*/
@SuppressWarnings("all")
public Set getLanguageWeightAdjustments() {
return this.languageWeightAdjustments;
}
/**
* @return whether to distinguish between North Korean and South Korean
*/
@SuppressWarnings("all")
public Boolean getKoreanDialects() {
return this.koreanDialects;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof LanguageOptions)) return false;
final LanguageOptions other = (LanguageOptions) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$multilingual = this.getMultilingual();
final Object other$multilingual = other.getMultilingual();
if (this$multilingual == null ? other$multilingual != null : !this$multilingual.equals(other$multilingual)) return false;
final Object this$minValidChars = this.getMinValidChars();
final Object other$minValidChars = other.getMinValidChars();
if (this$minValidChars == null ? other$minValidChars != null : !this$minValidChars.equals(other$minValidChars)) return false;
final Object this$profileDepth = this.getProfileDepth();
final Object other$profileDepth = other.getProfileDepth();
if (this$profileDepth == null ? other$profileDepth != null : !this$profileDepth.equals(other$profileDepth)) return false;
final Object this$ambiguityThreshold = this.getAmbiguityThreshold();
final Object other$ambiguityThreshold = other.getAmbiguityThreshold();
if (this$ambiguityThreshold == null ? other$ambiguityThreshold != null : !this$ambiguityThreshold.equals(other$ambiguityThreshold)) return false;
final Object this$invalidityThreshold = this.getInvalidityThreshold();
final Object other$invalidityThreshold = other.getInvalidityThreshold();
if (this$invalidityThreshold == null ? other$invalidityThreshold != null : !this$invalidityThreshold.equals(other$invalidityThreshold)) return false;
final Object this$languageHintWeight = this.getLanguageHintWeight();
final Object other$languageHintWeight = other.getLanguageHintWeight();
if (this$languageHintWeight == null ? other$languageHintWeight != null : !this$languageHintWeight.equals(other$languageHintWeight)) return false;
final Object this$encodingHintWeight = this.getEncodingHintWeight();
final Object other$encodingHintWeight = other.getEncodingHintWeight();
if (this$encodingHintWeight == null ? other$encodingHintWeight != null : !this$encodingHintWeight.equals(other$encodingHintWeight)) return false;
final Object this$koreanDialects = this.getKoreanDialects();
final Object other$koreanDialects = other.getKoreanDialects();
if (this$koreanDialects == null ? other$koreanDialects != null : !this$koreanDialects.equals(other$koreanDialects)) return false;
final Object this$languageHint = this.getLanguageHint();
final Object other$languageHint = other.getLanguageHint();
if (this$languageHint == null ? other$languageHint != null : !this$languageHint.equals(other$languageHint)) return false;
final Object this$encodingHint = this.getEncodingHint();
final Object other$encodingHint = other.getEncodingHint();
if (this$encodingHint == null ? other$encodingHint != null : !this$encodingHint.equals(other$encodingHint)) return false;
final Object this$languageWeightAdjustments = this.getLanguageWeightAdjustments();
final Object other$languageWeightAdjustments = other.getLanguageWeightAdjustments();
if (this$languageWeightAdjustments == null ? other$languageWeightAdjustments != null : !this$languageWeightAdjustments.equals(other$languageWeightAdjustments)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof LanguageOptions;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $multilingual = this.getMultilingual();
result = result * PRIME + ($multilingual == null ? 43 : $multilingual.hashCode());
final Object $minValidChars = this.getMinValidChars();
result = result * PRIME + ($minValidChars == null ? 43 : $minValidChars.hashCode());
final Object $profileDepth = this.getProfileDepth();
result = result * PRIME + ($profileDepth == null ? 43 : $profileDepth.hashCode());
final Object $ambiguityThreshold = this.getAmbiguityThreshold();
result = result * PRIME + ($ambiguityThreshold == null ? 43 : $ambiguityThreshold.hashCode());
final Object $invalidityThreshold = this.getInvalidityThreshold();
result = result * PRIME + ($invalidityThreshold == null ? 43 : $invalidityThreshold.hashCode());
final Object $languageHintWeight = this.getLanguageHintWeight();
result = result * PRIME + ($languageHintWeight == null ? 43 : $languageHintWeight.hashCode());
final Object $encodingHintWeight = this.getEncodingHintWeight();
result = result * PRIME + ($encodingHintWeight == null ? 43 : $encodingHintWeight.hashCode());
final Object $koreanDialects = this.getKoreanDialects();
result = result * PRIME + ($koreanDialects == null ? 43 : $koreanDialects.hashCode());
final Object $languageHint = this.getLanguageHint();
result = result * PRIME + ($languageHint == null ? 43 : $languageHint.hashCode());
final Object $encodingHint = this.getEncodingHint();
result = result * PRIME + ($encodingHint == null ? 43 : $encodingHint.hashCode());
final Object $languageWeightAdjustments = this.getLanguageWeightAdjustments();
result = result * PRIME + ($languageWeightAdjustments == null ? 43 : $languageWeightAdjustments.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "LanguageOptions(multilingual=" + this.getMultilingual() + ", minValidChars=" + this.getMinValidChars() + ", profileDepth=" + this.getProfileDepth() + ", ambiguityThreshold=" + this.getAmbiguityThreshold() + ", invalidityThreshold=" + this.getInvalidityThreshold() + ", languageHint=" + this.getLanguageHint() + ", languageHintWeight=" + this.getLanguageHintWeight() + ", encodingHint=" + this.getEncodingHint() + ", encodingHintWeight=" + this.getEncodingHintWeight() + ", languageWeightAdjustments=" + this.getLanguageWeightAdjustments() + ", koreanDialects=" + this.getKoreanDialects() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy