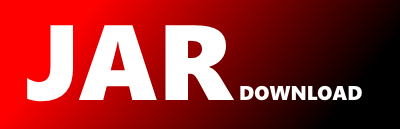
com.basistech.rosette.apimodel.LanguageWeight Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2017 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
import com.basistech.util.ISO15924;
import com.basistech.util.LanguageCode;
import jakarta.validation.constraints.Max;
import jakarta.validation.constraints.Min;
/**
* language weight used to resolve ambiguous results
*/
@JacksonMixin
public final class LanguageWeight {
/**
*/
private final LanguageCode language;
/**
*/
private final ISO15924 script;
/**
*/
@Min(0)
@Max(100)
private final Integer weight;
@SuppressWarnings("all")
LanguageWeight(final LanguageCode language, final ISO15924 script, final Integer weight) {
this.language = language;
this.script = script;
this.weight = weight;
}
@SuppressWarnings("all")
public static class LanguageWeightBuilder {
@SuppressWarnings("all")
private LanguageCode language;
@SuppressWarnings("all")
private ISO15924 script;
@SuppressWarnings("all")
private Integer weight;
@SuppressWarnings("all")
LanguageWeightBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageWeight.LanguageWeightBuilder language(final LanguageCode language) {
this.language = language;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageWeight.LanguageWeightBuilder script(final ISO15924 script) {
this.script = script;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public LanguageWeight.LanguageWeightBuilder weight(final Integer weight) {
this.weight = weight;
return this;
}
@SuppressWarnings("all")
public LanguageWeight build() {
return new LanguageWeight(this.language, this.script, this.weight);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "LanguageWeight.LanguageWeightBuilder(language=" + this.language + ", script=" + this.script + ", weight=" + this.weight + ")";
}
}
@SuppressWarnings("all")
public static LanguageWeight.LanguageWeightBuilder builder() {
return new LanguageWeight.LanguageWeightBuilder();
}
/**
* @return the language
*/
@SuppressWarnings("all")
public LanguageCode getLanguage() {
return this.language;
}
/**
* @return the script
*/
@SuppressWarnings("all")
public ISO15924 getScript() {
return this.script;
}
/**
* @return the weight used to resolve ambiguous results
*/
@SuppressWarnings("all")
public Integer getWeight() {
return this.weight;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof LanguageWeight)) return false;
final LanguageWeight other = (LanguageWeight) o;
final Object this$weight = this.getWeight();
final Object other$weight = other.getWeight();
if (this$weight == null ? other$weight != null : !this$weight.equals(other$weight)) return false;
final Object this$language = this.getLanguage();
final Object other$language = other.getLanguage();
if (this$language == null ? other$language != null : !this$language.equals(other$language)) return false;
final Object this$script = this.getScript();
final Object other$script = other.getScript();
if (this$script == null ? other$script != null : !this$script.equals(other$script)) return false;
return true;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $weight = this.getWeight();
result = result * PRIME + ($weight == null ? 43 : $weight.hashCode());
final Object $language = this.getLanguage();
result = result * PRIME + ($language == null ? 43 : $language.hashCode());
final Object $script = this.getScript();
result = result * PRIME + ($script == null ? 43 : $script.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "LanguageWeight(language=" + this.getLanguage() + ", script=" + this.getScript() + ", weight=" + this.getWeight() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy