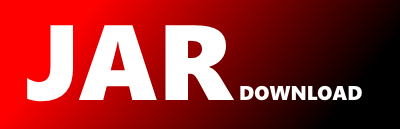
com.basistech.rosette.apimodel.MorphologyOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2018 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
import com.basistech.util.PartOfSpeechTagSet;
/**
* Morphology options
*/
@JacksonMixin
public final class MorphologyOptions extends Options {
/**
*/
private final Boolean disambiguate;
/**
* DocumentRequest query processing. Linguistics analysis may change its behavior
* to reflect the fact that query input is often not in full sentences;
* Typically, this disables disambiguation.
*/
private final Boolean query;
/**
*/
private final PartOfSpeechTagSet partOfSpeechTagSet;
/**
*/
private final MorphologyModelType modelType;
/**
*/
private final DisambiguatorType disambiguatorType;
@SuppressWarnings("all")
MorphologyOptions(final Boolean disambiguate, final Boolean query, final PartOfSpeechTagSet partOfSpeechTagSet, final MorphologyModelType modelType, final DisambiguatorType disambiguatorType) {
this.disambiguate = disambiguate;
this.query = query;
this.partOfSpeechTagSet = partOfSpeechTagSet;
this.modelType = modelType;
this.disambiguatorType = disambiguatorType;
}
@SuppressWarnings("all")
public static class MorphologyOptionsBuilder {
@SuppressWarnings("all")
private Boolean disambiguate;
@SuppressWarnings("all")
private Boolean query;
@SuppressWarnings("all")
private PartOfSpeechTagSet partOfSpeechTagSet;
@SuppressWarnings("all")
private MorphologyModelType modelType;
@SuppressWarnings("all")
private DisambiguatorType disambiguatorType;
@SuppressWarnings("all")
MorphologyOptionsBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public MorphologyOptions.MorphologyOptionsBuilder disambiguate(final Boolean disambiguate) {
this.disambiguate = disambiguate;
return this;
}
/**
* DocumentRequest query processing. Linguistics analysis may change its behavior
* to reflect the fact that query input is often not in full sentences;
* Typically, this disables disambiguation.
* @return {@code this}.
*/
@SuppressWarnings("all")
public MorphologyOptions.MorphologyOptionsBuilder query(final Boolean query) {
this.query = query;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public MorphologyOptions.MorphologyOptionsBuilder partOfSpeechTagSet(final PartOfSpeechTagSet partOfSpeechTagSet) {
this.partOfSpeechTagSet = partOfSpeechTagSet;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public MorphologyOptions.MorphologyOptionsBuilder modelType(final MorphologyModelType modelType) {
this.modelType = modelType;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public MorphologyOptions.MorphologyOptionsBuilder disambiguatorType(final DisambiguatorType disambiguatorType) {
this.disambiguatorType = disambiguatorType;
return this;
}
@SuppressWarnings("all")
public MorphologyOptions build() {
return new MorphologyOptions(this.disambiguate, this.query, this.partOfSpeechTagSet, this.modelType, this.disambiguatorType);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "MorphologyOptions.MorphologyOptionsBuilder(disambiguate=" + this.disambiguate + ", query=" + this.query + ", partOfSpeechTagSet=" + this.partOfSpeechTagSet + ", modelType=" + this.modelType + ", disambiguatorType=" + this.disambiguatorType + ")";
}
}
@SuppressWarnings("all")
public static MorphologyOptions.MorphologyOptionsBuilder builder() {
return new MorphologyOptions.MorphologyOptionsBuilder();
}
/**
* @return whether the linguistics analysis should disambiguate results
*/
@SuppressWarnings("all")
public Boolean getDisambiguate() {
return this.disambiguate;
}
/**
* DocumentRequest query processing. Linguistics analysis may change its behavior
* to reflect the fact that query input is often not in full sentences;
* Typically, this disables disambiguation.
* @return request query processing
* Linguistics analysis may change its behavior to reflect the fact that query input is often
* not in full sentences; Typically, this disables disambiguation.
*/
@SuppressWarnings("all")
public Boolean getQuery() {
return this.query;
}
/**
* @return the tag set used when returning part of speech tags. A {@code null} value
* indicates the default.
*/
@SuppressWarnings("all")
public PartOfSpeechTagSet getPartOfSpeechTagSet() {
return this.partOfSpeechTagSet;
}
/**
* @return the model type
*/
@SuppressWarnings("all")
public MorphologyModelType getModelType() {
return this.modelType;
}
/**
* @return the disambiguator type
*/
@SuppressWarnings("all")
public DisambiguatorType getDisambiguatorType() {
return this.disambiguatorType;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof MorphologyOptions)) return false;
final MorphologyOptions other = (MorphologyOptions) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$disambiguate = this.getDisambiguate();
final Object other$disambiguate = other.getDisambiguate();
if (this$disambiguate == null ? other$disambiguate != null : !this$disambiguate.equals(other$disambiguate)) return false;
final Object this$query = this.getQuery();
final Object other$query = other.getQuery();
if (this$query == null ? other$query != null : !this$query.equals(other$query)) return false;
final Object this$partOfSpeechTagSet = this.getPartOfSpeechTagSet();
final Object other$partOfSpeechTagSet = other.getPartOfSpeechTagSet();
if (this$partOfSpeechTagSet == null ? other$partOfSpeechTagSet != null : !this$partOfSpeechTagSet.equals(other$partOfSpeechTagSet)) return false;
final Object this$modelType = this.getModelType();
final Object other$modelType = other.getModelType();
if (this$modelType == null ? other$modelType != null : !this$modelType.equals(other$modelType)) return false;
final Object this$disambiguatorType = this.getDisambiguatorType();
final Object other$disambiguatorType = other.getDisambiguatorType();
if (this$disambiguatorType == null ? other$disambiguatorType != null : !this$disambiguatorType.equals(other$disambiguatorType)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof MorphologyOptions;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $disambiguate = this.getDisambiguate();
result = result * PRIME + ($disambiguate == null ? 43 : $disambiguate.hashCode());
final Object $query = this.getQuery();
result = result * PRIME + ($query == null ? 43 : $query.hashCode());
final Object $partOfSpeechTagSet = this.getPartOfSpeechTagSet();
result = result * PRIME + ($partOfSpeechTagSet == null ? 43 : $partOfSpeechTagSet.hashCode());
final Object $modelType = this.getModelType();
result = result * PRIME + ($modelType == null ? 43 : $modelType.hashCode());
final Object $disambiguatorType = this.getDisambiguatorType();
result = result * PRIME + ($disambiguatorType == null ? 43 : $disambiguatorType.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "MorphologyOptions(disambiguate=" + this.getDisambiguate() + ", query=" + this.getQuery() + ", partOfSpeechTagSet=" + this.getPartOfSpeechTagSet() + ", modelType=" + this.getModelType() + ", disambiguatorType=" + this.getDisambiguatorType() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy