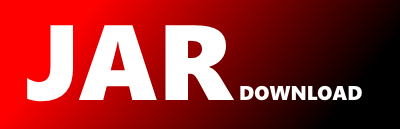
com.basistech.rosette.apimodel.NameTranslationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2017 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import java.util.List;
import com.basistech.rosette.annotations.JacksonMixin;
import com.basistech.util.ISO15924;
import com.basistech.util.LanguageCode;
import com.basistech.util.TransliterationScheme;
/**
* name translation result
*/
@JacksonMixin
public class NameTranslationResponse extends Response {
/**
*/
private final ISO15924 sourceScript;
/**
*/
private final LanguageCode sourceLanguageOfOrigin;
/**
*/
private final LanguageCode sourceLanguageOfUse;
/**
*/
private final LanguageCode targetLanguage;
/**
*/
private final ISO15924 targetScript;
/**
*/
private final TransliterationScheme targetScheme;
/**
*/
private final String translation;
/**
*/
private final Double confidence;
/**
*/
private final List translations;
@SuppressWarnings("all")
NameTranslationResponse(final ISO15924 sourceScript, final LanguageCode sourceLanguageOfOrigin, final LanguageCode sourceLanguageOfUse, final LanguageCode targetLanguage, final ISO15924 targetScript, final TransliterationScheme targetScheme, final String translation, final Double confidence, final List translations) {
this.sourceScript = sourceScript;
this.sourceLanguageOfOrigin = sourceLanguageOfOrigin;
this.sourceLanguageOfUse = sourceLanguageOfUse;
this.targetLanguage = targetLanguage;
this.targetScript = targetScript;
this.targetScheme = targetScheme;
this.translation = translation;
this.confidence = confidence;
this.translations = translations;
}
@SuppressWarnings("all")
public static class NameTranslationResponseBuilder {
@SuppressWarnings("all")
private ISO15924 sourceScript;
@SuppressWarnings("all")
private LanguageCode sourceLanguageOfOrigin;
@SuppressWarnings("all")
private LanguageCode sourceLanguageOfUse;
@SuppressWarnings("all")
private LanguageCode targetLanguage;
@SuppressWarnings("all")
private ISO15924 targetScript;
@SuppressWarnings("all")
private TransliterationScheme targetScheme;
@SuppressWarnings("all")
private String translation;
@SuppressWarnings("all")
private Double confidence;
@SuppressWarnings("all")
private List translations;
@SuppressWarnings("all")
NameTranslationResponseBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder sourceScript(final ISO15924 sourceScript) {
this.sourceScript = sourceScript;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder sourceLanguageOfOrigin(final LanguageCode sourceLanguageOfOrigin) {
this.sourceLanguageOfOrigin = sourceLanguageOfOrigin;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder sourceLanguageOfUse(final LanguageCode sourceLanguageOfUse) {
this.sourceLanguageOfUse = sourceLanguageOfUse;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder targetLanguage(final LanguageCode targetLanguage) {
this.targetLanguage = targetLanguage;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder targetScript(final ISO15924 targetScript) {
this.targetScript = targetScript;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder targetScheme(final TransliterationScheme targetScheme) {
this.targetScheme = targetScheme;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder translation(final String translation) {
this.translation = translation;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder confidence(final Double confidence) {
this.confidence = confidence;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public NameTranslationResponse.NameTranslationResponseBuilder translations(final List translations) {
this.translations = translations;
return this;
}
@SuppressWarnings("all")
public NameTranslationResponse build() {
return new NameTranslationResponse(this.sourceScript, this.sourceLanguageOfOrigin, this.sourceLanguageOfUse, this.targetLanguage, this.targetScript, this.targetScheme, this.translation, this.confidence, this.translations);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "NameTranslationResponse.NameTranslationResponseBuilder(sourceScript=" + this.sourceScript + ", sourceLanguageOfOrigin=" + this.sourceLanguageOfOrigin + ", sourceLanguageOfUse=" + this.sourceLanguageOfUse + ", targetLanguage=" + this.targetLanguage + ", targetScript=" + this.targetScript + ", targetScheme=" + this.targetScheme + ", translation=" + this.translation + ", confidence=" + this.confidence + ", translations=" + this.translations + ")";
}
}
@SuppressWarnings("all")
public static NameTranslationResponse.NameTranslationResponseBuilder builder() {
return new NameTranslationResponse.NameTranslationResponseBuilder();
}
/**
* @return the code for the script of the name to translate
*/
@SuppressWarnings("all")
public ISO15924 getSourceScript() {
return this.sourceScript;
}
/**
* @return the source name's language of origin code
*/
@SuppressWarnings("all")
public LanguageCode getSourceLanguageOfOrigin() {
return this.sourceLanguageOfOrigin;
}
/**
* @return the source name's language of use code
*/
@SuppressWarnings("all")
public LanguageCode getSourceLanguageOfUse() {
return this.sourceLanguageOfUse;
}
/**
* @return the target name's language code
*/
@SuppressWarnings("all")
public LanguageCode getTargetLanguage() {
return this.targetLanguage;
}
/**
* @return the target name's script code
*/
@SuppressWarnings("all")
public ISO15924 getTargetScript() {
return this.targetScript;
}
/**
* @return the transliteration scheme used
*/
@SuppressWarnings("all")
public TransliterationScheme getTargetScheme() {
return this.targetScheme;
}
/**
* @return the translation
*/
@SuppressWarnings("all")
public String getTranslation() {
return this.translation;
}
/**
* @return the translation confidence (0.0-1.0)
*/
@SuppressWarnings("all")
public Double getConfidence() {
return this.confidence;
}
/**
* @return the translation
*/
@SuppressWarnings("all")
public List getTranslations() {
return this.translations;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof NameTranslationResponse)) return false;
final NameTranslationResponse other = (NameTranslationResponse) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$confidence = this.getConfidence();
final Object other$confidence = other.getConfidence();
if (this$confidence == null ? other$confidence != null : !this$confidence.equals(other$confidence)) return false;
final Object this$sourceScript = this.getSourceScript();
final Object other$sourceScript = other.getSourceScript();
if (this$sourceScript == null ? other$sourceScript != null : !this$sourceScript.equals(other$sourceScript)) return false;
final Object this$sourceLanguageOfOrigin = this.getSourceLanguageOfOrigin();
final Object other$sourceLanguageOfOrigin = other.getSourceLanguageOfOrigin();
if (this$sourceLanguageOfOrigin == null ? other$sourceLanguageOfOrigin != null : !this$sourceLanguageOfOrigin.equals(other$sourceLanguageOfOrigin)) return false;
final Object this$sourceLanguageOfUse = this.getSourceLanguageOfUse();
final Object other$sourceLanguageOfUse = other.getSourceLanguageOfUse();
if (this$sourceLanguageOfUse == null ? other$sourceLanguageOfUse != null : !this$sourceLanguageOfUse.equals(other$sourceLanguageOfUse)) return false;
final Object this$targetLanguage = this.getTargetLanguage();
final Object other$targetLanguage = other.getTargetLanguage();
if (this$targetLanguage == null ? other$targetLanguage != null : !this$targetLanguage.equals(other$targetLanguage)) return false;
final Object this$targetScript = this.getTargetScript();
final Object other$targetScript = other.getTargetScript();
if (this$targetScript == null ? other$targetScript != null : !this$targetScript.equals(other$targetScript)) return false;
final Object this$targetScheme = this.getTargetScheme();
final Object other$targetScheme = other.getTargetScheme();
if (this$targetScheme == null ? other$targetScheme != null : !this$targetScheme.equals(other$targetScheme)) return false;
final Object this$translation = this.getTranslation();
final Object other$translation = other.getTranslation();
if (this$translation == null ? other$translation != null : !this$translation.equals(other$translation)) return false;
final Object this$translations = this.getTranslations();
final Object other$translations = other.getTranslations();
if (this$translations == null ? other$translations != null : !this$translations.equals(other$translations)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof NameTranslationResponse;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $confidence = this.getConfidence();
result = result * PRIME + ($confidence == null ? 43 : $confidence.hashCode());
final Object $sourceScript = this.getSourceScript();
result = result * PRIME + ($sourceScript == null ? 43 : $sourceScript.hashCode());
final Object $sourceLanguageOfOrigin = this.getSourceLanguageOfOrigin();
result = result * PRIME + ($sourceLanguageOfOrigin == null ? 43 : $sourceLanguageOfOrigin.hashCode());
final Object $sourceLanguageOfUse = this.getSourceLanguageOfUse();
result = result * PRIME + ($sourceLanguageOfUse == null ? 43 : $sourceLanguageOfUse.hashCode());
final Object $targetLanguage = this.getTargetLanguage();
result = result * PRIME + ($targetLanguage == null ? 43 : $targetLanguage.hashCode());
final Object $targetScript = this.getTargetScript();
result = result * PRIME + ($targetScript == null ? 43 : $targetScript.hashCode());
final Object $targetScheme = this.getTargetScheme();
result = result * PRIME + ($targetScheme == null ? 43 : $targetScheme.hashCode());
final Object $translation = this.getTranslation();
result = result * PRIME + ($translation == null ? 43 : $translation.hashCode());
final Object $translations = this.getTranslations();
result = result * PRIME + ($translations == null ? 43 : $translations.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy