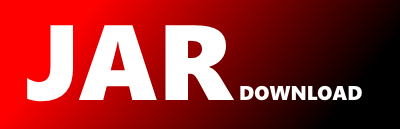
com.basistech.rosette.apimodel.Relationship Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2017 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
import java.util.List;
import java.util.Set;
/**
* Relationship extracted by the relationship extractor
*/
@JacksonMixin
public final class Relationship {
/**
*/
private final String predicate;
/**
*/
private final String predicateId;
/**
*/
private final String arg1;
/**
*/
private final String arg1Id;
/**
*/
private final String arg2;
/**
*/
private final String arg2Id;
/**
*/
private final String arg3;
/**
*/
private final String arg3Id;
/**
*/
private final List adjuncts;
/**
*/
private final List locatives;
/**
*/
private final List temporals;
/**
*/
private final Set modalities;
/**
*/
private final Double confidence;
@SuppressWarnings("all")
Relationship(final String predicate, final String predicateId, final String arg1, final String arg1Id, final String arg2, final String arg2Id, final String arg3, final String arg3Id, final List adjuncts, final List locatives, final List temporals, final Set modalities, final Double confidence) {
this.predicate = predicate;
this.predicateId = predicateId;
this.arg1 = arg1;
this.arg1Id = arg1Id;
this.arg2 = arg2;
this.arg2Id = arg2Id;
this.arg3 = arg3;
this.arg3Id = arg3Id;
this.adjuncts = adjuncts;
this.locatives = locatives;
this.temporals = temporals;
this.modalities = modalities;
this.confidence = confidence;
}
@SuppressWarnings("all")
public static class RelationshipBuilder {
@SuppressWarnings("all")
private String predicate;
@SuppressWarnings("all")
private String predicateId;
@SuppressWarnings("all")
private String arg1;
@SuppressWarnings("all")
private String arg1Id;
@SuppressWarnings("all")
private String arg2;
@SuppressWarnings("all")
private String arg2Id;
@SuppressWarnings("all")
private String arg3;
@SuppressWarnings("all")
private String arg3Id;
@SuppressWarnings("all")
private List adjuncts;
@SuppressWarnings("all")
private List locatives;
@SuppressWarnings("all")
private List temporals;
@SuppressWarnings("all")
private Set modalities;
@SuppressWarnings("all")
private Double confidence;
@SuppressWarnings("all")
RelationshipBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder predicate(final String predicate) {
this.predicate = predicate;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder predicateId(final String predicateId) {
this.predicateId = predicateId;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder arg1(final String arg1) {
this.arg1 = arg1;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder arg1Id(final String arg1Id) {
this.arg1Id = arg1Id;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder arg2(final String arg2) {
this.arg2 = arg2;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder arg2Id(final String arg2Id) {
this.arg2Id = arg2Id;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder arg3(final String arg3) {
this.arg3 = arg3;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder arg3Id(final String arg3Id) {
this.arg3Id = arg3Id;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder adjuncts(final List adjuncts) {
this.adjuncts = adjuncts;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder locatives(final List locatives) {
this.locatives = locatives;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder temporals(final List temporals) {
this.temporals = temporals;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder modalities(final Set modalities) {
this.modalities = modalities;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public Relationship.RelationshipBuilder confidence(final Double confidence) {
this.confidence = confidence;
return this;
}
@SuppressWarnings("all")
public Relationship build() {
return new Relationship(this.predicate, this.predicateId, this.arg1, this.arg1Id, this.arg2, this.arg2Id, this.arg3, this.arg3Id, this.adjuncts, this.locatives, this.temporals, this.modalities, this.confidence);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "Relationship.RelationshipBuilder(predicate=" + this.predicate + ", predicateId=" + this.predicateId + ", arg1=" + this.arg1 + ", arg1Id=" + this.arg1Id + ", arg2=" + this.arg2 + ", arg2Id=" + this.arg2Id + ", arg3=" + this.arg3 + ", arg3Id=" + this.arg3Id + ", adjuncts=" + this.adjuncts + ", locatives=" + this.locatives + ", temporals=" + this.temporals + ", modalities=" + this.modalities + ", confidence=" + this.confidence + ")";
}
}
@SuppressWarnings("all")
public static Relationship.RelationshipBuilder builder() {
return new Relationship.RelationshipBuilder();
}
/**
* @return the relationship predicate
*/
@SuppressWarnings("all")
public String getPredicate() {
return this.predicate;
}
/**
* @return the relationship predicate'sID
*/
@SuppressWarnings("all")
public String getPredicateId() {
return this.predicateId;
}
/**
* @return the first arg
*/
@SuppressWarnings("all")
public String getArg1() {
return this.arg1;
}
/**
* @return the first arg's ID
*/
@SuppressWarnings("all")
public String getArg1Id() {
return this.arg1Id;
}
/**
* @return the second arg
*/
@SuppressWarnings("all")
public String getArg2() {
return this.arg2;
}
/**
* @return the second arg's ID
*/
@SuppressWarnings("all")
public String getArg2Id() {
return this.arg2Id;
}
/**
* @return the third arg
*/
@SuppressWarnings("all")
public String getArg3() {
return this.arg3;
}
/**
* @return the third arg's ID
*/
@SuppressWarnings("all")
public String getArg3Id() {
return this.arg3Id;
}
/**
* @return a list of adjuncts
*/
@SuppressWarnings("all")
public List getAdjuncts() {
return this.adjuncts;
}
/**
* @return a list of locatives
*/
@SuppressWarnings("all")
public List getLocatives() {
return this.locatives;
}
/**
* @return a list of temporals
*/
@SuppressWarnings("all")
public List getTemporals() {
return this.temporals;
}
/**
* @return modalities
*/
@SuppressWarnings("all")
public Set getModalities() {
return this.modalities;
}
/**
* @return the confidence (0.0-1.0)
*/
@SuppressWarnings("all")
public Double getConfidence() {
return this.confidence;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Relationship)) return false;
final Relationship other = (Relationship) o;
final Object this$confidence = this.getConfidence();
final Object other$confidence = other.getConfidence();
if (this$confidence == null ? other$confidence != null : !this$confidence.equals(other$confidence)) return false;
final Object this$predicate = this.getPredicate();
final Object other$predicate = other.getPredicate();
if (this$predicate == null ? other$predicate != null : !this$predicate.equals(other$predicate)) return false;
final Object this$predicateId = this.getPredicateId();
final Object other$predicateId = other.getPredicateId();
if (this$predicateId == null ? other$predicateId != null : !this$predicateId.equals(other$predicateId)) return false;
final Object this$arg1 = this.getArg1();
final Object other$arg1 = other.getArg1();
if (this$arg1 == null ? other$arg1 != null : !this$arg1.equals(other$arg1)) return false;
final Object this$arg1Id = this.getArg1Id();
final Object other$arg1Id = other.getArg1Id();
if (this$arg1Id == null ? other$arg1Id != null : !this$arg1Id.equals(other$arg1Id)) return false;
final Object this$arg2 = this.getArg2();
final Object other$arg2 = other.getArg2();
if (this$arg2 == null ? other$arg2 != null : !this$arg2.equals(other$arg2)) return false;
final Object this$arg2Id = this.getArg2Id();
final Object other$arg2Id = other.getArg2Id();
if (this$arg2Id == null ? other$arg2Id != null : !this$arg2Id.equals(other$arg2Id)) return false;
final Object this$arg3 = this.getArg3();
final Object other$arg3 = other.getArg3();
if (this$arg3 == null ? other$arg3 != null : !this$arg3.equals(other$arg3)) return false;
final Object this$arg3Id = this.getArg3Id();
final Object other$arg3Id = other.getArg3Id();
if (this$arg3Id == null ? other$arg3Id != null : !this$arg3Id.equals(other$arg3Id)) return false;
final Object this$adjuncts = this.getAdjuncts();
final Object other$adjuncts = other.getAdjuncts();
if (this$adjuncts == null ? other$adjuncts != null : !this$adjuncts.equals(other$adjuncts)) return false;
final Object this$locatives = this.getLocatives();
final Object other$locatives = other.getLocatives();
if (this$locatives == null ? other$locatives != null : !this$locatives.equals(other$locatives)) return false;
final Object this$temporals = this.getTemporals();
final Object other$temporals = other.getTemporals();
if (this$temporals == null ? other$temporals != null : !this$temporals.equals(other$temporals)) return false;
final Object this$modalities = this.getModalities();
final Object other$modalities = other.getModalities();
if (this$modalities == null ? other$modalities != null : !this$modalities.equals(other$modalities)) return false;
return true;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $confidence = this.getConfidence();
result = result * PRIME + ($confidence == null ? 43 : $confidence.hashCode());
final Object $predicate = this.getPredicate();
result = result * PRIME + ($predicate == null ? 43 : $predicate.hashCode());
final Object $predicateId = this.getPredicateId();
result = result * PRIME + ($predicateId == null ? 43 : $predicateId.hashCode());
final Object $arg1 = this.getArg1();
result = result * PRIME + ($arg1 == null ? 43 : $arg1.hashCode());
final Object $arg1Id = this.getArg1Id();
result = result * PRIME + ($arg1Id == null ? 43 : $arg1Id.hashCode());
final Object $arg2 = this.getArg2();
result = result * PRIME + ($arg2 == null ? 43 : $arg2.hashCode());
final Object $arg2Id = this.getArg2Id();
result = result * PRIME + ($arg2Id == null ? 43 : $arg2Id.hashCode());
final Object $arg3 = this.getArg3();
result = result * PRIME + ($arg3 == null ? 43 : $arg3.hashCode());
final Object $arg3Id = this.getArg3Id();
result = result * PRIME + ($arg3Id == null ? 43 : $arg3Id.hashCode());
final Object $adjuncts = this.getAdjuncts();
result = result * PRIME + ($adjuncts == null ? 43 : $adjuncts.hashCode());
final Object $locatives = this.getLocatives();
result = result * PRIME + ($locatives == null ? 43 : $locatives.hashCode());
final Object $temporals = this.getTemporals();
result = result * PRIME + ($temporals == null ? 43 : $temporals.hashCode());
final Object $modalities = this.getModalities();
result = result * PRIME + ($modalities == null ? 43 : $modalities.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "Relationship(predicate=" + this.getPredicate() + ", predicateId=" + this.getPredicateId() + ", arg1=" + this.getArg1() + ", arg1Id=" + this.getArg1Id() + ", arg2=" + this.getArg2() + ", arg2Id=" + this.getArg2Id() + ", arg3=" + this.getArg3() + ", arg3Id=" + this.getArg3Id() + ", adjuncts=" + this.getAdjuncts() + ", locatives=" + this.getLocatives() + ", temporals=" + this.getTemporals() + ", modalities=" + this.getModalities() + ", confidence=" + this.getConfidence() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy