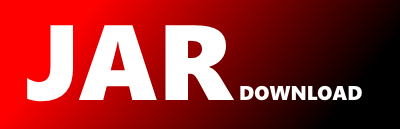
com.basistech.rosette.apimodel.SentencesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2017 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
import java.util.List;
/**
* Simple api response data model for sentence determination requests
*/
@JacksonMixin
public class SentencesResponse extends Response {
/**
*/
private final List sentences;
@SuppressWarnings("all")
SentencesResponse(final List sentences) {
this.sentences = sentences;
}
@SuppressWarnings("all")
public static class SentencesResponseBuilder {
@SuppressWarnings("all")
private List sentences;
@SuppressWarnings("all")
SentencesResponseBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public SentencesResponse.SentencesResponseBuilder sentences(final List sentences) {
this.sentences = sentences;
return this;
}
@SuppressWarnings("all")
public SentencesResponse build() {
return new SentencesResponse(this.sentences);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "SentencesResponse.SentencesResponseBuilder(sentences=" + this.sentences + ")";
}
}
@SuppressWarnings("all")
public static SentencesResponse.SentencesResponseBuilder builder() {
return new SentencesResponse.SentencesResponseBuilder();
}
/**
* @return the list of sentences
*/
@SuppressWarnings("all")
public List getSentences() {
return this.sentences;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof SentencesResponse)) return false;
final SentencesResponse other = (SentencesResponse) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$sentences = this.getSentences();
final Object other$sentences = other.getSentences();
if (this$sentences == null ? other$sentences != null : !this$sentences.equals(other$sentences)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof SentencesResponse;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $sentences = this.getSentences();
result = result * PRIME + ($sentences == null ? 43 : $sentences.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy