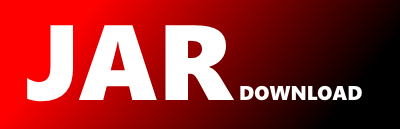
com.basistech.rosette.apimodel.SentimentOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2017 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
/**
* Sentiment options.
*/
@JacksonMixin
public final class SentimentOptions extends Options {
public static final SentimentOptions DEFAULT = SentimentOptions.builder().calculateEntityConfidence(false).calculateEntitySalience(false).build();
/**
*/
private final Boolean calculateEntityConfidence;
/**
*/
private final Boolean calculateEntitySalience;
/**
*/
private final SentimentModelType modelType;
@SuppressWarnings("all")
SentimentOptions(final Boolean calculateEntityConfidence, final Boolean calculateEntitySalience, final SentimentModelType modelType) {
this.calculateEntityConfidence = calculateEntityConfidence;
this.calculateEntitySalience = calculateEntitySalience;
this.modelType = modelType;
}
@SuppressWarnings("all")
public static class SentimentOptionsBuilder {
@SuppressWarnings("all")
private Boolean calculateEntityConfidence;
@SuppressWarnings("all")
private Boolean calculateEntitySalience;
@SuppressWarnings("all")
private SentimentModelType modelType;
@SuppressWarnings("all")
SentimentOptionsBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public SentimentOptions.SentimentOptionsBuilder calculateEntityConfidence(final Boolean calculateEntityConfidence) {
this.calculateEntityConfidence = calculateEntityConfidence;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public SentimentOptions.SentimentOptionsBuilder calculateEntitySalience(final Boolean calculateEntitySalience) {
this.calculateEntitySalience = calculateEntitySalience;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public SentimentOptions.SentimentOptionsBuilder modelType(final SentimentModelType modelType) {
this.modelType = modelType;
return this;
}
@SuppressWarnings("all")
public SentimentOptions build() {
return new SentimentOptions(this.calculateEntityConfidence, this.calculateEntitySalience, this.modelType);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "SentimentOptions.SentimentOptionsBuilder(calculateEntityConfidence=" + this.calculateEntityConfidence + ", calculateEntitySalience=" + this.calculateEntitySalience + ", modelType=" + this.modelType + ")";
}
}
@SuppressWarnings("all")
public static SentimentOptions.SentimentOptionsBuilder builder() {
return new SentimentOptions.SentimentOptionsBuilder();
}
/**
* @return the calculateEntityConfidence flag.
*/
@SuppressWarnings("all")
public Boolean getCalculateEntityConfidence() {
return this.calculateEntityConfidence;
}
/**
* @return the calculateEntitySalience flag.
*/
@SuppressWarnings("all")
public Boolean getCalculateEntitySalience() {
return this.calculateEntitySalience;
}
/**
* @return the model type.
*/
@SuppressWarnings("all")
public SentimentModelType getModelType() {
return this.modelType;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof SentimentOptions)) return false;
final SentimentOptions other = (SentimentOptions) o;
if (!other.canEqual((Object) this)) return false;
if (!super.equals(o)) return false;
final Object this$calculateEntityConfidence = this.getCalculateEntityConfidence();
final Object other$calculateEntityConfidence = other.getCalculateEntityConfidence();
if (this$calculateEntityConfidence == null ? other$calculateEntityConfidence != null : !this$calculateEntityConfidence.equals(other$calculateEntityConfidence)) return false;
final Object this$calculateEntitySalience = this.getCalculateEntitySalience();
final Object other$calculateEntitySalience = other.getCalculateEntitySalience();
if (this$calculateEntitySalience == null ? other$calculateEntitySalience != null : !this$calculateEntitySalience.equals(other$calculateEntitySalience)) return false;
final Object this$modelType = this.getModelType();
final Object other$modelType = other.getModelType();
if (this$modelType == null ? other$modelType != null : !this$modelType.equals(other$modelType)) return false;
return true;
}
@SuppressWarnings("all")
protected boolean canEqual(final Object other) {
return other instanceof SentimentOptions;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final Object $calculateEntityConfidence = this.getCalculateEntityConfidence();
result = result * PRIME + ($calculateEntityConfidence == null ? 43 : $calculateEntityConfidence.hashCode());
final Object $calculateEntitySalience = this.getCalculateEntitySalience();
result = result * PRIME + ($calculateEntitySalience == null ? 43 : $calculateEntitySalience.hashCode());
final Object $modelType = this.getModelType();
result = result * PRIME + ($modelType == null ? 43 : $modelType.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "SentimentOptions(calculateEntityConfidence=" + this.getCalculateEntityConfidence() + ", calculateEntitySalience=" + this.getCalculateEntitySalience() + ", modelType=" + this.getModelType() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy