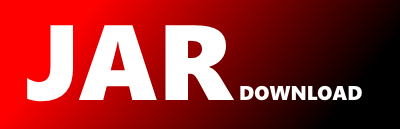
com.basistech.rosette.apimodel.SupportedLanguage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2018 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel;
import com.basistech.rosette.annotations.JacksonMixin;
import com.basistech.util.ISO15924;
import com.basistech.util.LanguageCode;
/**
* Supported language/script
*/
@JacksonMixin
@com.fasterxml.jackson.databind.annotation.JsonDeserialize(builder = SupportedLanguage.SupportedLanguageBuilder.class)
public final class SupportedLanguage {
/**
*/
private final LanguageCode language;
/**
*/
private final ISO15924 script;
/**
*/
private Boolean licensed;
@SuppressWarnings("all")
SupportedLanguage(final LanguageCode language, final ISO15924 script, final Boolean licensed) {
this.language = language;
this.script = script;
this.licensed = licensed;
}
@SuppressWarnings("all")
@com.fasterxml.jackson.databind.annotation.JsonPOJOBuilder(withPrefix = "", buildMethodName = "build")
public static class SupportedLanguageBuilder {
@SuppressWarnings("all")
private LanguageCode language;
@SuppressWarnings("all")
private ISO15924 script;
@SuppressWarnings("all")
private Boolean licensed;
@SuppressWarnings("all")
SupportedLanguageBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public SupportedLanguage.SupportedLanguageBuilder language(final LanguageCode language) {
this.language = language;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public SupportedLanguage.SupportedLanguageBuilder script(final ISO15924 script) {
this.script = script;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public SupportedLanguage.SupportedLanguageBuilder licensed(final Boolean licensed) {
this.licensed = licensed;
return this;
}
@SuppressWarnings("all")
public SupportedLanguage build() {
return new SupportedLanguage(this.language, this.script, this.licensed);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "SupportedLanguage.SupportedLanguageBuilder(language=" + this.language + ", script=" + this.script + ", licensed=" + this.licensed + ")";
}
}
@SuppressWarnings("all")
public static SupportedLanguage.SupportedLanguageBuilder builder() {
return new SupportedLanguage.SupportedLanguageBuilder();
}
/**
* @return the language code
*/
@SuppressWarnings("all")
public LanguageCode getLanguage() {
return this.language;
}
/**
* @return the script code
*/
@SuppressWarnings("all")
public ISO15924 getScript() {
return this.script;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof SupportedLanguage)) return false;
final SupportedLanguage other = (SupportedLanguage) o;
final Object this$licensed = this.getLicensed();
final Object other$licensed = other.getLicensed();
if (this$licensed == null ? other$licensed != null : !this$licensed.equals(other$licensed)) return false;
final Object this$language = this.getLanguage();
final Object other$language = other.getLanguage();
if (this$language == null ? other$language != null : !this$language.equals(other$language)) return false;
final Object this$script = this.getScript();
final Object other$script = other.getScript();
if (this$script == null ? other$script != null : !this$script.equals(other$script)) return false;
return true;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $licensed = this.getLicensed();
result = result * PRIME + ($licensed == null ? 43 : $licensed.hashCode());
final Object $language = this.getLanguage();
result = result * PRIME + ($language == null ? 43 : $language.hashCode());
final Object $script = this.getScript();
result = result * PRIME + ($script == null ? 43 : $script.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "SupportedLanguage(language=" + this.getLanguage() + ", script=" + this.getScript() + ", licensed=" + this.getLicensed() + ")";
}
/**
* @return if this language is licensed or not
*/
@SuppressWarnings("all")
public Boolean getLicensed() {
return this.licensed;
}
/**
*/
@SuppressWarnings("all")
public void setLicensed(final Boolean licensed) {
this.licensed = licensed;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy