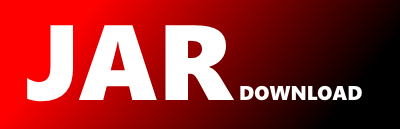
com.basistech.rosette.apimodel.batch.BatchRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2024 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel.batch;
import com.basistech.rosette.annotations.JacksonMixin;
import java.util.List;
import java.util.UUID;
/**
* Define a batch job. Serialize this object to json to create a file
* that requests a job.
*/
@JacksonMixin
public final class BatchRequest {
/**
*/
private final String batchId;
/**
* A URL for Analytics API to call to inform you the completion of the batch.
* It must be accessible on the Internet and can only be http: or https:.
* Analytics API will use the GET method so optional parameters need to be
* included as query parameters in the URL.
*/
private final String completionCallbackUrl;
/**
*/
private final List items;
/**
* Specifies where the results should be stored. Only a valid AWS S3 URL
* is supported at this time. The S3 bucket needs to have a proper policy
* statement to grant read/write permission to Analytics API within the batch
* processing time window. Analytics API's AWS account number is 625892746452.
*
* Example policy statement:
*
*
*
* {
* "Version": "2012-10-17",
* "Id": "Policy1462373421611",
* "Statement": [
* {
* "Sid": "Stmt1462373415064",
* "Effect": "Allow",
* "Principal": {
* "AWS": "arn:aws:iam::625892746452:root"
* },
* "Action": "s3:ListBucket",
* "Resource": "arn:aws:s3:::your-bucket-name",
* "Condition": {
* "StringLike": {
* "s3:prefix": [
* "output-folder-name/*"
* ]
* }
* }
* },
* {
* "Sid": "Stmt1462373415064",
* "Effect": "Allow",
* "Principal": {
* "AWS": "arn:aws:iam::625892746452:root"
* },
* "Action": [
* "s3:GetObject",
* "s3:PutObject",
* "s3:DeleteObject"
* ],
* "Resource": "arn:aws:s3:::your-bucket-name/output-folder-name/*"
* }
* ]
* }
*
*
*/
private final String batchOutputUrl;
@SuppressWarnings("all")
private static String $default$batchId() {
return UUID.randomUUID().toString();
}
@SuppressWarnings("all")
BatchRequest(final String batchId, final String completionCallbackUrl, final List items, final String batchOutputUrl) {
this.batchId = batchId;
this.completionCallbackUrl = completionCallbackUrl;
this.items = items;
this.batchOutputUrl = batchOutputUrl;
}
@SuppressWarnings("all")
public static class BatchRequestBuilder {
@SuppressWarnings("all")
private boolean batchId$set;
@SuppressWarnings("all")
private String batchId$value;
@SuppressWarnings("all")
private String completionCallbackUrl;
@SuppressWarnings("all")
private List items;
@SuppressWarnings("all")
private String batchOutputUrl;
@SuppressWarnings("all")
BatchRequestBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public BatchRequest.BatchRequestBuilder batchId(final String batchId) {
this.batchId$value = batchId;
batchId$set = true;
return this;
}
/**
* A URL for Analytics API to call to inform you the completion of the batch.
* It must be accessible on the Internet and can only be http: or https:.
* Analytics API will use the GET method so optional parameters need to be
* included as query parameters in the URL.
* @return {@code this}.
*/
@SuppressWarnings("all")
public BatchRequest.BatchRequestBuilder completionCallbackUrl(final String completionCallbackUrl) {
this.completionCallbackUrl = completionCallbackUrl;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public BatchRequest.BatchRequestBuilder items(final List items) {
this.items = items;
return this;
}
/**
* Specifies where the results should be stored. Only a valid AWS S3 URL
* is supported at this time. The S3 bucket needs to have a proper policy
* statement to grant read/write permission to Analytics API within the batch
* processing time window. Analytics API's AWS account number is 625892746452.
*
* Example policy statement:
*
*
*
* {
* "Version": "2012-10-17",
* "Id": "Policy1462373421611",
* "Statement": [
* {
* "Sid": "Stmt1462373415064",
* "Effect": "Allow",
* "Principal": {
* "AWS": "arn:aws:iam::625892746452:root"
* },
* "Action": "s3:ListBucket",
* "Resource": "arn:aws:s3:::your-bucket-name",
* "Condition": {
* "StringLike": {
* "s3:prefix": [
* "output-folder-name/*"
* ]
* }
* }
* },
* {
* "Sid": "Stmt1462373415064",
* "Effect": "Allow",
* "Principal": {
* "AWS": "arn:aws:iam::625892746452:root"
* },
* "Action": [
* "s3:GetObject",
* "s3:PutObject",
* "s3:DeleteObject"
* ],
* "Resource": "arn:aws:s3:::your-bucket-name/output-folder-name/*"
* }
* ]
* }
*
*
* @return {@code this}.
*/
@SuppressWarnings("all")
public BatchRequest.BatchRequestBuilder batchOutputUrl(final String batchOutputUrl) {
this.batchOutputUrl = batchOutputUrl;
return this;
}
@SuppressWarnings("all")
public BatchRequest build() {
String batchId$value = this.batchId$value;
if (!this.batchId$set) batchId$value = BatchRequest.$default$batchId();
return new BatchRequest(batchId$value, this.completionCallbackUrl, this.items, this.batchOutputUrl);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "BatchRequest.BatchRequestBuilder(batchId$value=" + this.batchId$value + ", completionCallbackUrl=" + this.completionCallbackUrl + ", items=" + this.items + ", batchOutputUrl=" + this.batchOutputUrl + ")";
}
}
@SuppressWarnings("all")
public static BatchRequest.BatchRequestBuilder builder() {
return new BatchRequest.BatchRequestBuilder();
}
/**
* @return id an ID string.
*/
@SuppressWarnings("all")
public String getBatchId() {
return this.batchId;
}
/**
* A URL for Analytics API to call to inform you the completion of the batch.
* It must be accessible on the Internet and can only be http: or https:.
* Analytics API will use the GET method so optional parameters need to be
* included as query parameters in the URL.
*
* @return completionCallbackUrl a URL for Analytics API to call when batch completes
*/
@SuppressWarnings("all")
public String getCompletionCallbackUrl() {
return this.completionCallbackUrl;
}
/**
* @return list of batch request items
*/
@SuppressWarnings("all")
public List getItems() {
return this.items;
}
/**
* Specifies where the results should be stored. Only a valid AWS S3 URL
* is supported at this time. The S3 bucket needs to have a proper policy
* statement to grant read/write permission to Analytics API within the batch
* processing time window. Analytics API's AWS account number is 625892746452.
*
* Example policy statement:
*
*
*
* {
* "Version": "2012-10-17",
* "Id": "Policy1462373421611",
* "Statement": [
* {
* "Sid": "Stmt1462373415064",
* "Effect": "Allow",
* "Principal": {
* "AWS": "arn:aws:iam::625892746452:root"
* },
* "Action": "s3:ListBucket",
* "Resource": "arn:aws:s3:::your-bucket-name",
* "Condition": {
* "StringLike": {
* "s3:prefix": [
* "output-folder-name/*"
* ]
* }
* }
* },
* {
* "Sid": "Stmt1462373415064",
* "Effect": "Allow",
* "Principal": {
* "AWS": "arn:aws:iam::625892746452:root"
* },
* "Action": [
* "s3:GetObject",
* "s3:PutObject",
* "s3:DeleteObject"
* ],
* "Resource": "arn:aws:s3:::your-bucket-name/output-folder-name/*"
* }
* ]
* }
*
*
*
* @return batchOutputUrl AWS S3 URL where results will be saved
*/
@SuppressWarnings("all")
public String getBatchOutputUrl() {
return this.batchOutputUrl;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof BatchRequest)) return false;
final BatchRequest other = (BatchRequest) o;
final Object this$batchId = this.getBatchId();
final Object other$batchId = other.getBatchId();
if (this$batchId == null ? other$batchId != null : !this$batchId.equals(other$batchId)) return false;
final Object this$completionCallbackUrl = this.getCompletionCallbackUrl();
final Object other$completionCallbackUrl = other.getCompletionCallbackUrl();
if (this$completionCallbackUrl == null ? other$completionCallbackUrl != null : !this$completionCallbackUrl.equals(other$completionCallbackUrl)) return false;
final Object this$items = this.getItems();
final Object other$items = other.getItems();
if (this$items == null ? other$items != null : !this$items.equals(other$items)) return false;
final Object this$batchOutputUrl = this.getBatchOutputUrl();
final Object other$batchOutputUrl = other.getBatchOutputUrl();
if (this$batchOutputUrl == null ? other$batchOutputUrl != null : !this$batchOutputUrl.equals(other$batchOutputUrl)) return false;
return true;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $batchId = this.getBatchId();
result = result * PRIME + ($batchId == null ? 43 : $batchId.hashCode());
final Object $completionCallbackUrl = this.getCompletionCallbackUrl();
result = result * PRIME + ($completionCallbackUrl == null ? 43 : $completionCallbackUrl.hashCode());
final Object $items = this.getItems();
result = result * PRIME + ($items == null ? 43 : $items.hashCode());
final Object $batchOutputUrl = this.getBatchOutputUrl();
result = result * PRIME + ($batchOutputUrl == null ? 43 : $batchOutputUrl.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "BatchRequest(batchId=" + this.getBatchId() + ", completionCallbackUrl=" + this.getCompletionCallbackUrl() + ", items=" + this.getItems() + ", batchOutputUrl=" + this.getBatchOutputUrl() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy