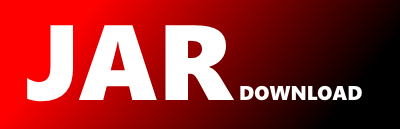
com.basistech.rosette.apimodel.batch.BatchRequestItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rosette-api-all Show documentation
Show all versions of rosette-api-all Show documentation
Babel Street Analytics API all modules combined
The newest version!
// Generated by delombok at Fri Nov 15 11:56:46 CST 2024
/*
* Copyright 2017 Basis Technology Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.basistech.rosette.apimodel.batch;
import com.basistech.rosette.annotations.JacksonMixin;
import com.basistech.rosette.apimodel.Request;
/**
* One item in a batch request. Each item consists of an input
* to process and an endpoint to apply to the item.
* The inputs are specified by {@link Request} objects.
* If the request type is {@link com.basistech.rosette.apimodel.DocumentRequest}, the special content type
* {@code application/vnd.basistech-multiple-inputs}
* used in {@link com.basistech.rosette.apimodel.DocumentRequest#contentType} identifies
* a json file consisting of an array of strings; each string being treated as an
* input. The string length in this case is limited to 200 characters.
*/
@JacksonMixin
public final class BatchRequestItem {
private final String endpoint;
private final Request request;
private final String id;
@SuppressWarnings("all")
BatchRequestItem(final String endpoint, final Request request, final String id) {
this.endpoint = endpoint;
this.request = request;
this.id = id;
}
@SuppressWarnings("all")
public static class BatchRequestItemBuilder {
@SuppressWarnings("all")
private String endpoint;
@SuppressWarnings("all")
private Request request;
@SuppressWarnings("all")
private String id;
@SuppressWarnings("all")
BatchRequestItemBuilder() {
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public BatchRequestItem.BatchRequestItemBuilder endpoint(final String endpoint) {
this.endpoint = endpoint;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public BatchRequestItem.BatchRequestItemBuilder request(final Request request) {
this.request = request;
return this;
}
/**
* @return {@code this}.
*/
@SuppressWarnings("all")
public BatchRequestItem.BatchRequestItemBuilder id(final String id) {
this.id = id;
return this;
}
@SuppressWarnings("all")
public BatchRequestItem build() {
return new BatchRequestItem(this.endpoint, this.request, this.id);
}
@Override
@SuppressWarnings("all")
public String toString() {
return "BatchRequestItem.BatchRequestItemBuilder(endpoint=" + this.endpoint + ", request=" + this.request + ", id=" + this.id + ")";
}
}
@SuppressWarnings("all")
public static BatchRequestItem.BatchRequestItemBuilder builder() {
return new BatchRequestItem.BatchRequestItemBuilder();
}
@SuppressWarnings("all")
public String getEndpoint() {
return this.endpoint;
}
@SuppressWarnings("all")
public Request getRequest() {
return this.request;
}
@SuppressWarnings("all")
public String getId() {
return this.id;
}
@Override
@SuppressWarnings("all")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof BatchRequestItem)) return false;
final BatchRequestItem other = (BatchRequestItem) o;
final Object this$endpoint = this.getEndpoint();
final Object other$endpoint = other.getEndpoint();
if (this$endpoint == null ? other$endpoint != null : !this$endpoint.equals(other$endpoint)) return false;
final Object this$request = this.getRequest();
final Object other$request = other.getRequest();
if (this$request == null ? other$request != null : !this$request.equals(other$request)) return false;
final Object this$id = this.getId();
final Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
return true;
}
@Override
@SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $endpoint = this.getEndpoint();
result = result * PRIME + ($endpoint == null ? 43 : $endpoint.hashCode());
final Object $request = this.getRequest();
result = result * PRIME + ($request == null ? 43 : $request.hashCode());
final Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
public String toString() {
return "BatchRequestItem(endpoint=" + this.getEndpoint() + ", request=" + this.getRequest() + ", id=" + this.getId() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy