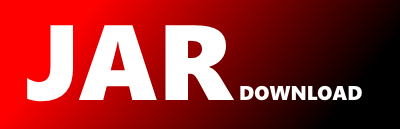
com.battcn.boot.request.utils.StringUtils Maven / Gradle / Ivy
package com.battcn.boot.request.utils;
/**
* @author Levin
* @since 2018/12/20 0020
*/
public class StringUtils {
/**
* The empty String {@code ""}.
*
* @since 2.0
*/
public static final String EMPTY = "";
public static final String DEFAULT_CHARSET = "UTF-8";
/**
* Returns either the passed in String, or if the String is
* {@code null}, the value of {@code defaultStr}.
*
*
* StringUtils.defaultString(null, "NULL") = "NULL"
* StringUtils.defaultString("", "NULL") = ""
* StringUtils.defaultString("bat", "NULL") = "bat"
*
*
* @param str the String to check, may be null
* @param defaultStr the default String to return
* if the input is {@code null}, may be null
* @return the passed in String, or the default if it was {@code null}
* @see String#valueOf(Object)
*/
public static String defaultString(final String str, final String defaultStr) {
return str == null ? defaultStr : str;
}
/**
* Joins the elements of the provided array into a single String
* containing the provided list of elements.
*
*
No separator is added to the joined String.
* Null objects or empty strings within the array are represented by
* empty strings.
*
*
* StringUtils.join(null) = null
* StringUtils.join([]) = ""
* StringUtils.join([null]) = ""
* StringUtils.join(["a", "b", "c"]) = "abc"
* StringUtils.join([null, "", "a"]) = "a"
*
*
* @param the specific type of values to join together
* @param elements the values to join together, may be null
* @return the joined String, {@code null} if null array input
* @since 3.0 Changed signature to use varargs
*/
public static String join(final T... elements) {
return join(elements, null);
}
/**
* Joins the elements of the provided array into a single String
* containing the provided list of elements.
*
*
No delimiter is added before or after the list.
* Null objects or empty strings within the array are represented by
* empty strings.
*
*
* StringUtils.join(null, *) = null
* StringUtils.join([], *) = ""
* StringUtils.join([null], *) = ""
* StringUtils.join(["a", "b", "c"], ';') = "a;b;c"
* StringUtils.join(["a", "b", "c"], null) = "abc"
* StringUtils.join([null, "", "a"], ';') = ";;a"
*
*
* @param array the array of values to join together, may be null
* @param separator the separator character to use
* @param startIndex the first index to start joining from. It is
* an error to pass in an end index past the end of the array
* @param endIndex the index to stop joining from (exclusive). It is
* an error to pass in an end index past the end of the array
* @return the joined String, {@code null} if null array input
* @since 2.0
*/
public static String join(final Object[] array, final char separator, final int startIndex, final int endIndex) {
if (array == null) {
return null;
}
final int noOfItems = endIndex - startIndex;
if (noOfItems <= 0) {
return EMPTY;
}
final StringBuilder buf = new StringBuilder(noOfItems * 16);
for (int i = startIndex; i < endIndex; i++) {
if (i > startIndex) {
buf.append(separator);
}
if (array[i] != null) {
buf.append(array[i]);
}
}
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy