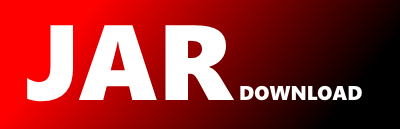
com.bazaarvoice.emodb.auth.identity.AuthIdentityModification Maven / Gradle / Ivy
package com.bazaarvoice.emodb.auth.identity;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Sets;
import java.util.Set;
import static com.google.common.base.Preconditions.checkArgument;
import static java.util.Objects.requireNonNull;
/**
* AuthIdentityModification is the base class for storing partial updates to an {@link AuthIdentity} as part of a create
* or update operation using {@link AuthIdentityManager#createIdentity(String, AuthIdentityModification)} or
* {@link AuthIdentityManager#updateIdentity(String, AuthIdentityModification)}, respectively. Subclasses should
* override to instantiate a new instance from one of the build methods, as well as add any additional modifiers
* for attributes specific to the AuthIdentity implementation if necessary.
*/
abstract public class AuthIdentityModification {
private String _owner;
private boolean _ownerPresent = false;
private String _description;
private boolean _descriptionPresent = false;
private Set _rolesAdded = Sets.newHashSet();
private Set _rolesRemoved = Sets.newHashSet();
public AuthIdentityModification withOwner(String owner) {
_owner = owner;
_ownerPresent = true;
return this;
}
public AuthIdentityModification withDescription(String description) {
_description = description;
_descriptionPresent = true;
return this;
}
public AuthIdentityModification addRoles(Set roles) {
requireNonNull(roles, "roles");
checkArgument(Sets.intersection(roles, _rolesRemoved).isEmpty(), "Cannot add and remove the same role");
_rolesAdded.addAll(roles);
return this;
}
public AuthIdentityModification addRoles(String... roles) {
return addRoles(ImmutableSet.copyOf(roles));
}
public AuthIdentityModification removeRoles(Set roles) {
requireNonNull(roles, "roles");
checkArgument(Sets.intersection(roles, _rolesAdded).isEmpty(), "Cannot add and remove the same role");
_rolesRemoved.addAll(roles);
return this;
}
public AuthIdentityModification removeRoles(String... roles) {
return removeRoles(ImmutableSet.copyOf(roles));
}
protected boolean isOwnerPresent() {
return _ownerPresent;
}
protected String getOwner() {
return _owner;
}
protected boolean isDescriptionPresent() {
return _descriptionPresent;
}
protected String getDescription() {
return _description;
}
/**
* Helper method for subclasses which, given a set of roles, returns a new set of roles with all added and removed
* roles from this modification applied.
*/
protected Set getUpdatedRolesFrom(Set roles) {
Set updatedRoles = Sets.newHashSet(roles);
updatedRoles.addAll(_rolesAdded);
updatedRoles.removeAll(_rolesRemoved);
return updatedRoles;
}
/**
* Subclasses should return a new AuthIdentity instance based only on the attributes set in this object with
* the provided ID. For example:
*
*
* AuthIdentityImpl identity = createAuthIdentityImplModification()
* .withOwner("[email protected]")
* .addRoles("role1")
* .buildNew("123");
*
* assert identity.getId().equals("123");
* assert identity.getOwner().equals("[email protected]");
* assert identity.getRoles().equals(ImmutableSet.of("role1"));
* assert identity.getDescription() == null;
*
*/
abstract public T buildNew(String id);
/**
* Subclasses should return a new AuthIdentity instance matching the provided entity plus any modifications
* set in this object. For example:
*
*
* AuthIdentityImpl before = getAuthIdentityImplFromSomewhere();
* assert before.getId().equals("123");
* assert before.getOwner.equals("[email protected]");
* assert before.getDescription.equals("Old description");
* assert before.getRoles().equals(ImmutableSet.of("role1"));
*
* AuthIdentityImpl after = createAuthIdentityImplModification()
* .withDescription("New description")
* .addRoles("role2")
* .removeRoles("role1")
* .buildFrom(before);
*
* assert after.getId().equals("123");
* assert after.getOwner.equals("[email protected]");
* assert after.getDescription.equals("New description");
* assert after.getRoles().equals(ImmutableSet.of("role2"));
*
*/
abstract public T buildFrom(T identity);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy